The `clear()` function in C++ is used to remove all elements from a vector, effectively resetting its size to zero.
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.clear(); // All elements are removed
std::cout << "Vector size after clear: " << myVector.size() << std::endl; // Output: 0
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can resize itself automatically when elements are added or removed. Vectors are part of the Standard Template Library (STL) and provide the flexibility that traditional arrays do not. They are defined in the `<vector>` header and can hold elements of any data type, including user-defined types.
Why Use Vectors?
Vectors offer several advantages:
- Dynamic Size: Unlike arrays, vectors can grow or shrink as needed, making them a suitable choice in scenarios where the number of elements is unknown at compile time.
- Memory Management: Vectors manage memory automatically, reducing the burden on developers when it comes to allocating and deallocating memory manually.
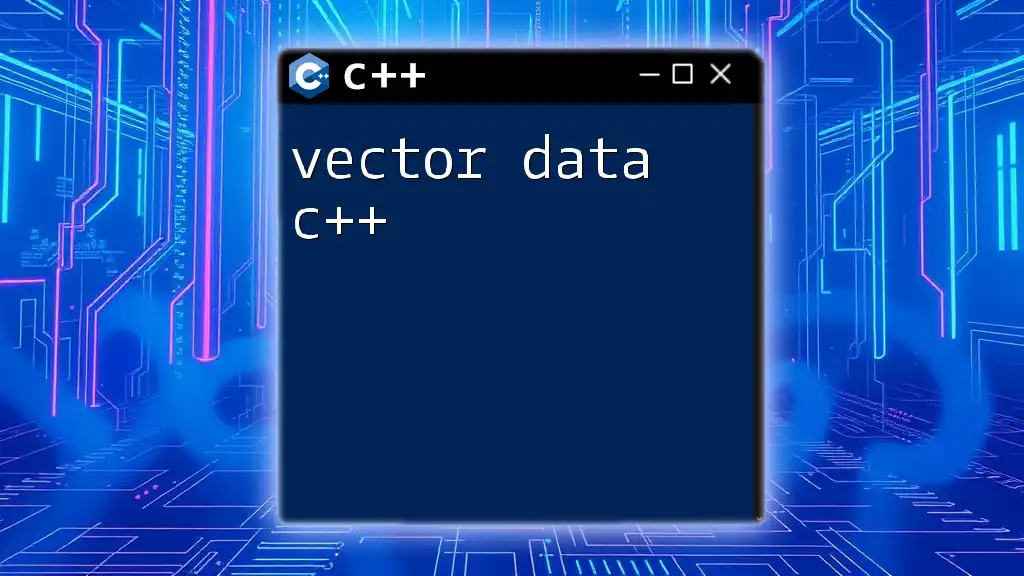
How to Clear a Vector in C++
Understanding the `clear()` Function
The `clear()` function is a member function provided by C++ vectors that removes all elements from the vector, effectively setting its size to zero. The syntax for using this method is straightforward:
vector_name.clear();
Basic Usage of `clear`
Using the `clear()` method is simple and efficient. Consider the following example that demonstrates how to clear a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.clear();
std::cout << "Vector size after clear: " << vec.size() << std::endl; // Output: 0
return 0;
}
In this example, we first declare and initialize a vector with five integers. After calling `vec.clear()`, we check the size of the vector, which confirms that it is now empty.
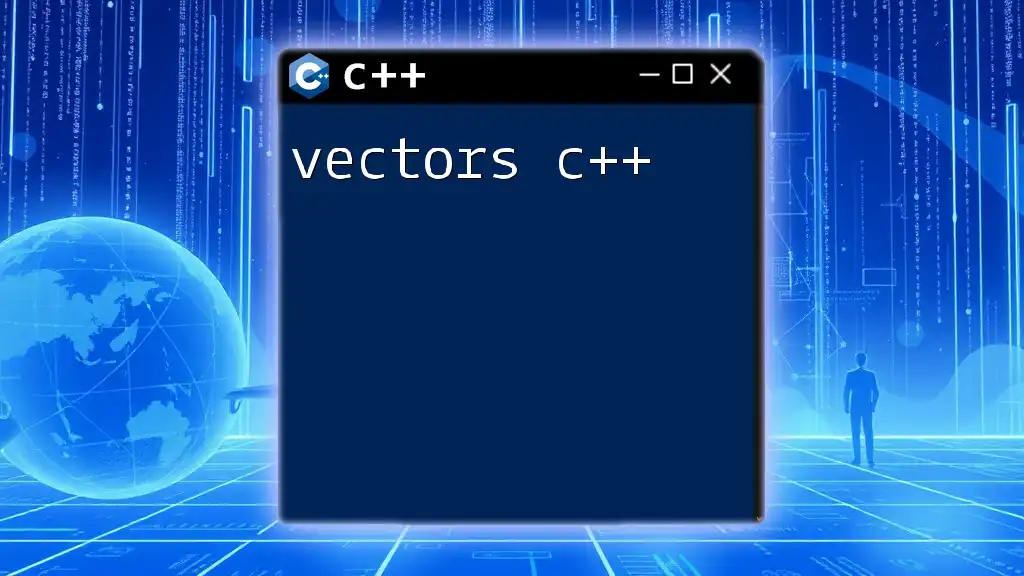
Handling Memory and Clearing Vectors
Memory Management with Vectors
When you call the `clear()` method, the vector still retains its allocated memory. This is important because if you plan to add elements back to the vector afterward, it won't need to reallocate memory unless it has to expand beyond its current capacity.
After Clearing a Vector
After a vector has been cleared, its size is zero, but its capacity remains unchanged. If you would like to free the unused memory, you can use the `shrink_to_fit()` method:
vec.clear();
vec.shrink_to_fit(); // optional
Using `shrink_to_fit()` suggests that the vector should reduce its capacity to fit its current size. This could free up unused memory resources, but it's important to note that its behavior is implementation-specific.
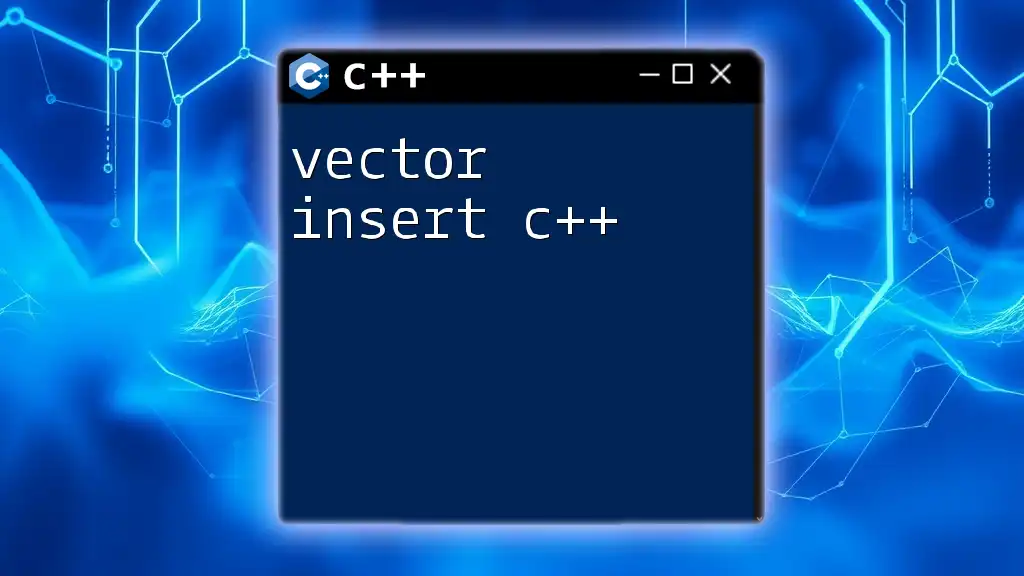
Alternatives to Clear a Vector in C++
Removing Elements with `erase()`
You can also clear a vector by removing its elements individually or in bulk using the `erase()` method. Unlike `clear()`, which removes all elements, `erase()` allows you to remove specific elements or a range:
vec.erase(vec.begin(), vec.end()); // equivalent to clear()
This method is effectively the same as `clear()`, but it provides more control. However, calling `erase()` on a vector is generally less efficient than `clear()` because it processes each element, whereas `clear()` simply resets the internal size.
Reinitializing the Vector
Another alternative to clear a vector is by reinitializing it. This completely resets the vector, effectively clearing it:
vec = std::vector<int>(); // reinitialize
This method constructs a new vector, replacing the old one. While it works, it may be less efficient than using `clear()` or `erase()` because it creates a new object and may require additional memory allocation.
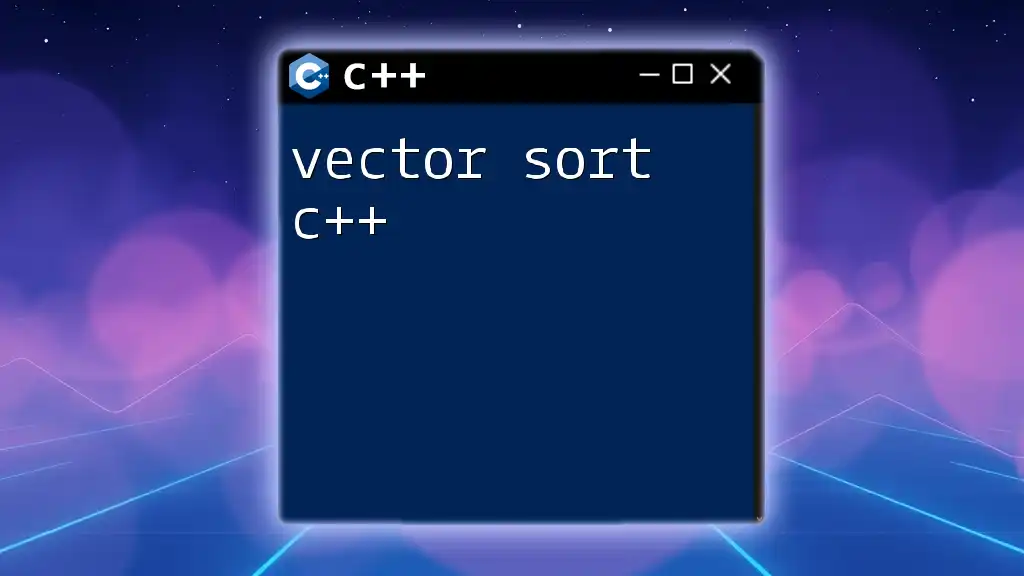
Common Mistakes and Tips
Avoiding Mistakes When Using `clear()`
When working with `clear()`, ensure that you do not attempt to access elements after clearing the vector without re-adding them. Accessing elements in a cleared vector will lead to undefined behavior, as the vector no longer holds valid data.
Performance Considerations
In terms of performance, the `clear()` function operates with a constant time complexity, or O(1). It does not, however, affect the capacity of the vector, which is a crucial distinction when considering memory management and performance in your applications.
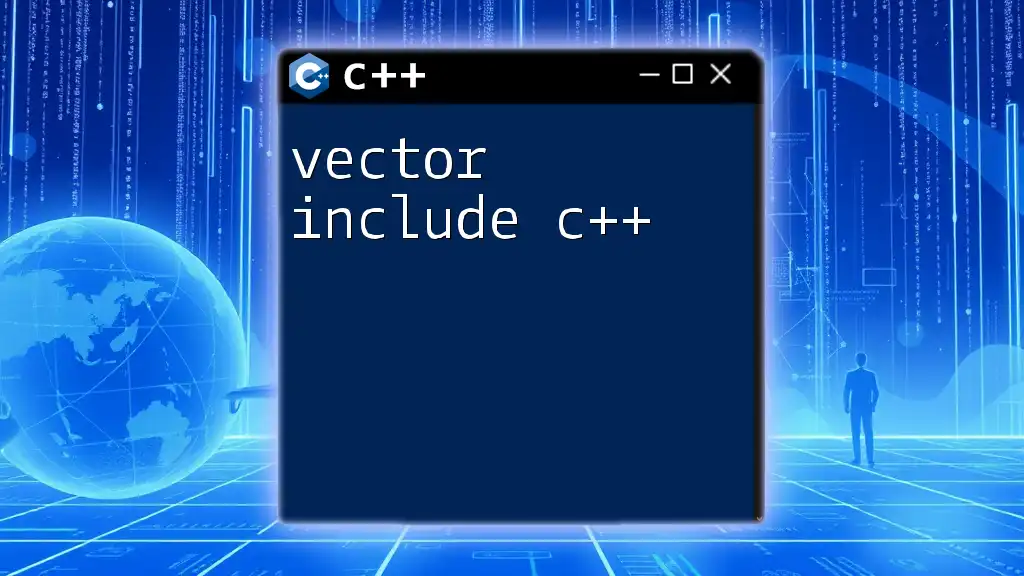
Conclusion
In summary, the `vector clear c++` operation is an essential tool for managing dynamic arrays effectively. By understanding how to use the `clear()` method, the implications of memory management, and the alternatives available, you can handle C++ vectors with confidence and efficiency. Practicing these techniques and experimenting with examples will deepen your understanding and proficiency with vectors in C++.