In C++, the `cin.clear()` function is used to clear the error state of the input stream, allowing further input operations to proceed after an input failure.
#include <iostream>
#include <limits>
int main() {
int number;
std::cout << "Enter a number: ";
while (!(std::cin >> number)) {
std::cin.clear(); // clear error state
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
std::cout << "Invalid input. Please enter a number: ";
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
Understanding `cin` and Its Role in Input
What is `cin`?
In C++, `cin` is an instance of the `istream` class that is designed to read input from the standard input device, which is usually the keyboard. It's an essential feature in C++ that allows users to interact with programs by inputting data.
When using `cin`, programmers can read various data types, such as integers, floating-point numbers, and characters. Its flexibility is one of the key reasons for its popularity among C++ developers.
Common Types of Input with `cin`
-
Simple Data Types: `cin` can easily read `int`, `float`, and `char` variables directly from the standard input.
-
Strings and Text Input: For reading strings, `cin` can take input directly. However, developers should be cautious, as using `cin` can only capture input until a whitespace character is encountered, meaning it cannot capture entire lines containing spaces without additional handling.
Example of Basic Input with `cin`
#include <iostream>
#include <string>
int main() {
int age;
std::cout << "Enter your age: ";
std::cin >> age; // User inputs their age
std::cout << "Your age is: " << age << std::endl;
return 0;
}
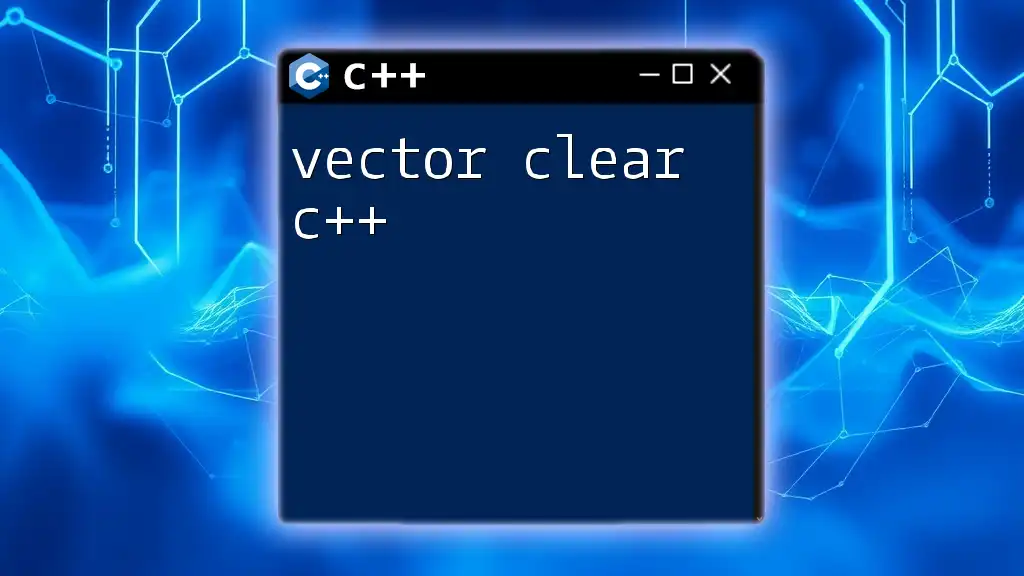
The Importance of Input Validation
Why Validate Input?
Input validation is crucial for maintaining the integrity of any program. When users enter data, there's always the possibility that the input might not match the expected format or could be of the wrong data type. This can lead to unexpected behavior, crashes, or security vulnerabilities.
Examples of Invalid Input Scenarios
Consider the following scenario where a user is prompted to enter an integer but inputs a non-integer value instead:
int number;
std::cout << "Please enter an integer: ";
std::cin >> number; // User inputs "abc"
// This will cause std::cin to enter a failure state, and 'number' remains undefined.
Without proper validation, the program can produce unintended results or behavior.
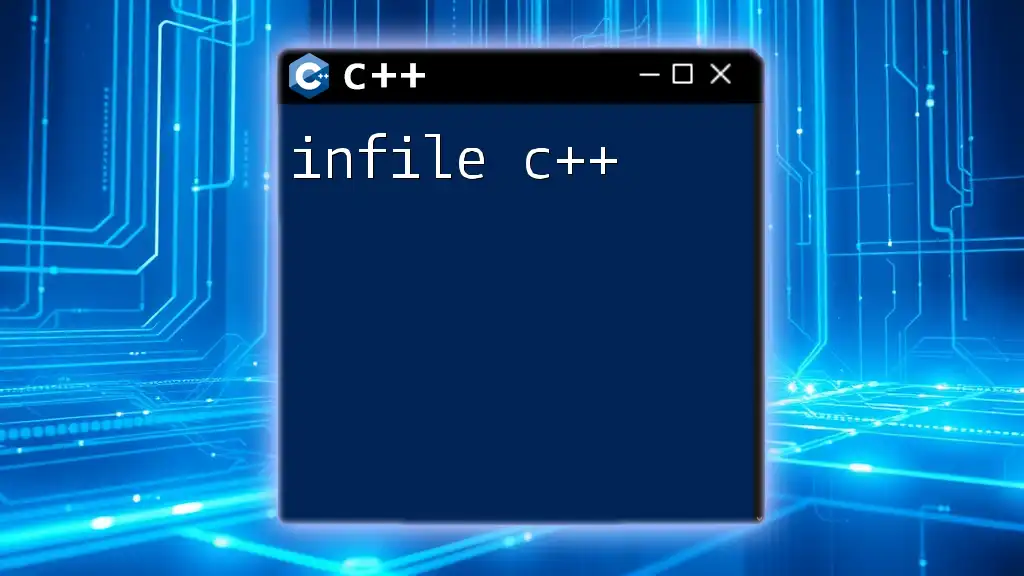
What is `cin.clear()`?
Purpose of `cin.clear()`
When `cin` encounters invalid input, it sets the stream's fail state, which prevents further input operations from functioning correctly. This is where `cin.clear()` comes into play—it resets the state of the stream, allowing the program to process new input from the user effectively.
How `cin.clear()` Works
The `cin.clear()` function clears the error flags on the input stream, resetting it so that further reading operations can proceed. Without using this command, the program might perpetually fail to read input after an error.
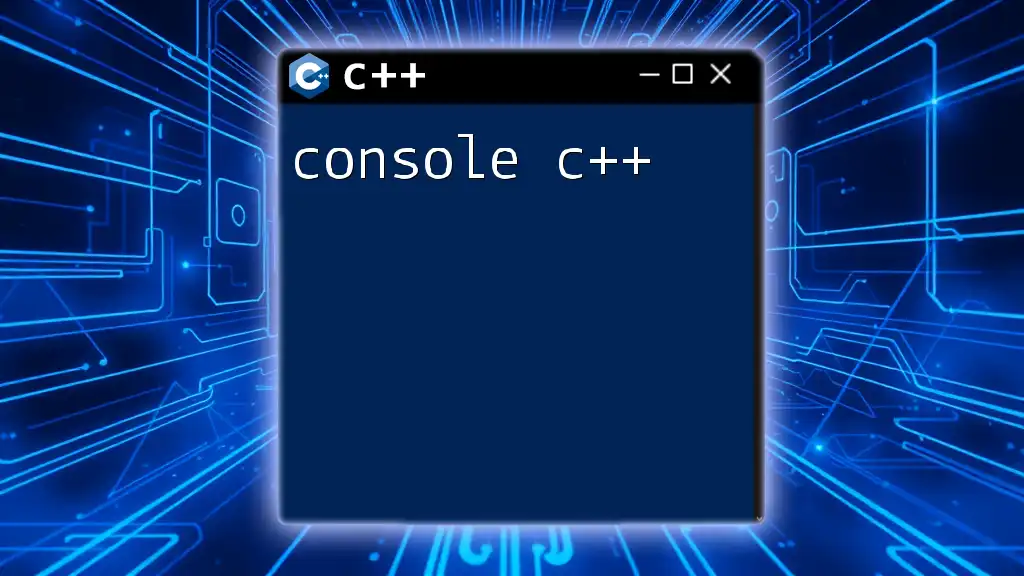
Using `cin.clear()` in Practice
Example of Using `cin.clear()`
Here’s a practical example that demonstrates how to implement input validation with `cin.clear()` in a loop. The program prompts the user to enter an integer and handles invalid input gracefully.
#include <iostream>
#include <limits>
int main() {
int number;
while (true) {
std::cout << "Please enter an integer: ";
std::cin >> number;
// Check for failure
if (std::cin.fail()) {
std::cin.clear(); // Clear the fail state
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // Discard invalid input
std::cout << "Invalid input. Try again.\n";
} else {
break; // Valid input, exit the loop
}
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
Explaining the Example
In the code above, after the user inputs a value, we check if the operation failed using `std::cin.fail()`. If it returns true, indicating that the input was invalid:
- `std::cin.clear()` is called to reset the input stream.
- `std::cin.ignore(...)` is used to discard any remaining characters in the input buffer up to the next newline. This prevents the error from being re-triggered on the next read attempt.
The loop repeats until a valid integer is entered, demonstrating how `cin.clear()` plays a critical role in enabling effective input handling.
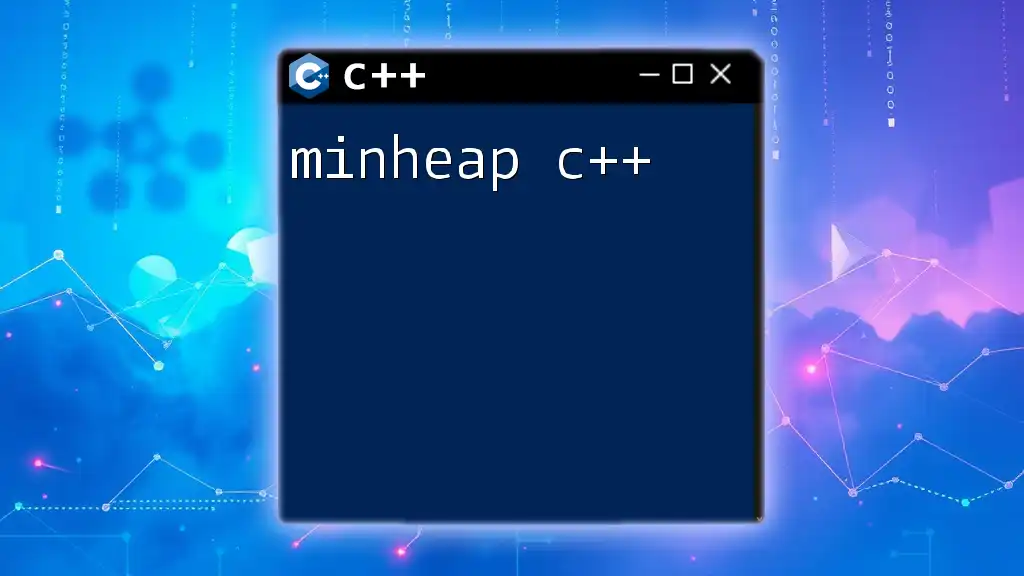
Avoiding Common Pitfalls
Common Mistakes with `cin.clear()`
Developers often make some common errors while using `cin.clear()`. One mistake includes calling `cin.clear()` without ensuring that they have also cleared the input buffer. Failing to do so could lead to the program getting stuck in an infinite loop due to remaining invalid data in the buffer.
Testing for Valid Input
It's essential to adopt a holistic approach toward input validation. In addition to using `cin.clear()`, other methods can complement user input validation:
- Use condition checks to validate the range or format.
- Utilize functions that read entire lines to process input and then convert data types.
These strategies can enhance robustness and improve overall user experience.
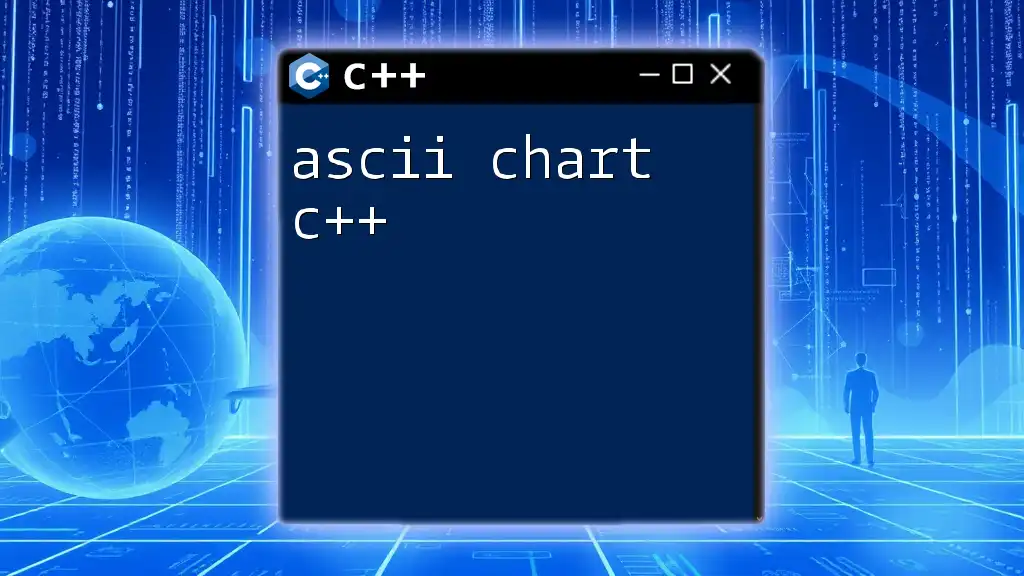
Real-World Applications of `cin.clear()`
Use Cases in C++ Applications
Input handling is a ubiquitous concern in real-world applications, especially in scenarios involving user interaction, such as:
- Command-line tools that prompt users for options.
- Games that ask for player input.
- Applications that depend on user configurations.
In all these cases, utilizing `cin` combined with a strategic use of `cin.clear()` ensures that programs remain user-friendly, allowing for efficient error recovery and maintaining flow in user interaction.
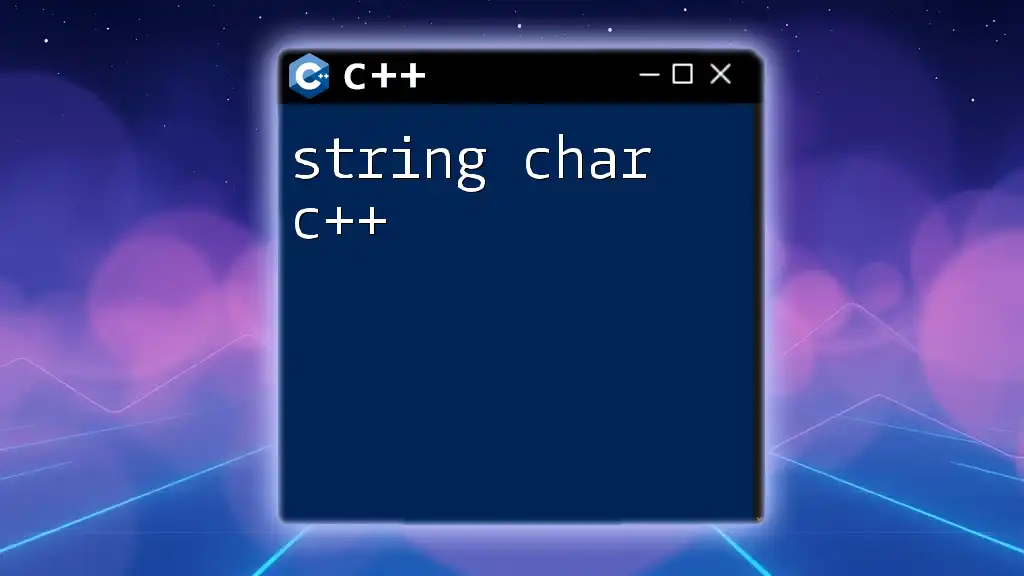
Conclusion
In summary, understanding how to effectively use `cin`, along with `cin.clear()`, is paramount for robust C++ programming. It not only ensures better user interactions but also fortifies applications against common input pitfalls. Implementing these techniques is crucial for anyone looking to build responsive and reliable C++ applications. Whether you’re a novice or an experienced developer, mastering these fundamentals will significantly enhance your coding repertoire.