In C++, the `#include` directive allows you to include the contents of a file, such as a library or header file, into your program, enabling code reuse and modularization.
Here's a simple code snippet demonstrating how to include a header file:
#include <iostream> // This includes the standard input-output stream library
int main() {
std::cout << "Hello, World!" << std::endl; // Prints a message to the console
return 0;
}
Understanding File Management in C++
File management refers to the way programs interact with the file system, enabling users to create, read, write, and modify files. In C++, file management becomes efficient through the `<fstream>` library, which provides a powerful interface for working with files.
The C++ File Handling Library
The `<fstream>` library includes three key classes:
- `ifstream`: Used for reading files.
- `ofstream`: Used for writing files.
- `fstream`: Used for both reading and writing files.
Choosing between these classes depends on the task at hand: utilize `ifstream` for reading, `ofstream` for writing, and `fstream` when you need both functionalities.
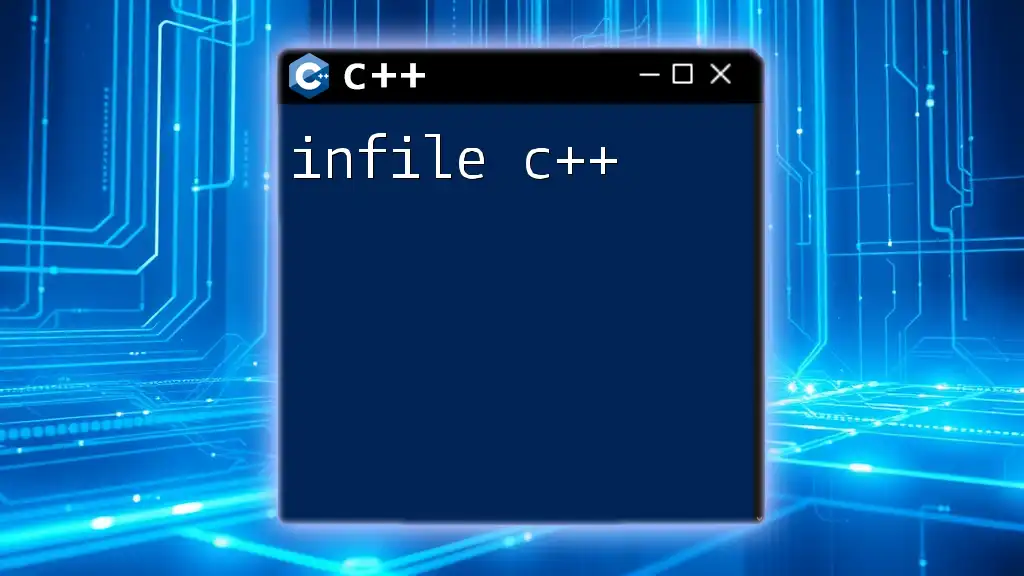
Writing to a File in C++
Opening a File
To write to a file in C++, you must first open it using the `ofstream` class. The opening syntax is straightforward:
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
if (!outFile) {
std::cerr << "Error opening the file!" << std::endl;
return 1;
}
// Continue writing...
}
In the above example, if the file can’t be opened (for instance, if the directory is incorrect or write permissions are not available), the program prints an error message and terminates.
Writing Data to a File
Once the file is opened successfully, you can write data to it using the `<<` operator. Here’s how it can be done:
outFile << "Hello, World!" << std::endl;
outFile << "Learning C++ file handling." << std::endl;
This snippet demonstrates writing two lines to the file "example.txt". Each line is followed by `std::endl`, which adds a newline character and flushes the output buffer.
Closing a File
After finishing your writing operations, it’s essential to close the file. This ensures that all data is written and releases the resources associated with the file. To close a file, simply call:
outFile.close();
Failing to close a file can lead to data loss, as the output buffer may not be fully written to the file.
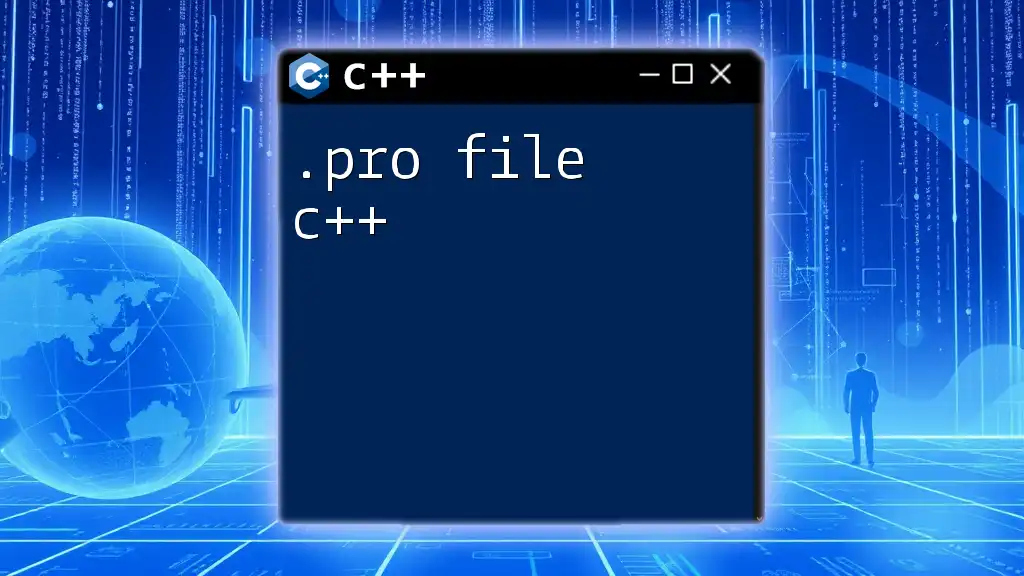
Appending Data to a File
Difference Between Writing and Appending
When writing to a file, any existing content will be overwritten unless the file is opened in append mode. Appending adds new content to the end of an existing file without affecting the current data.
How to Append Data
To append data, you open the file in append mode by using `std::ios::app`:
std::ofstream outFile("example.txt", std::ios::app);
outFile << "New line added." << std::endl;
outFile.close();
In this case, the line “New line added.” is written to the end of "example.txt", preserving all other existing content.
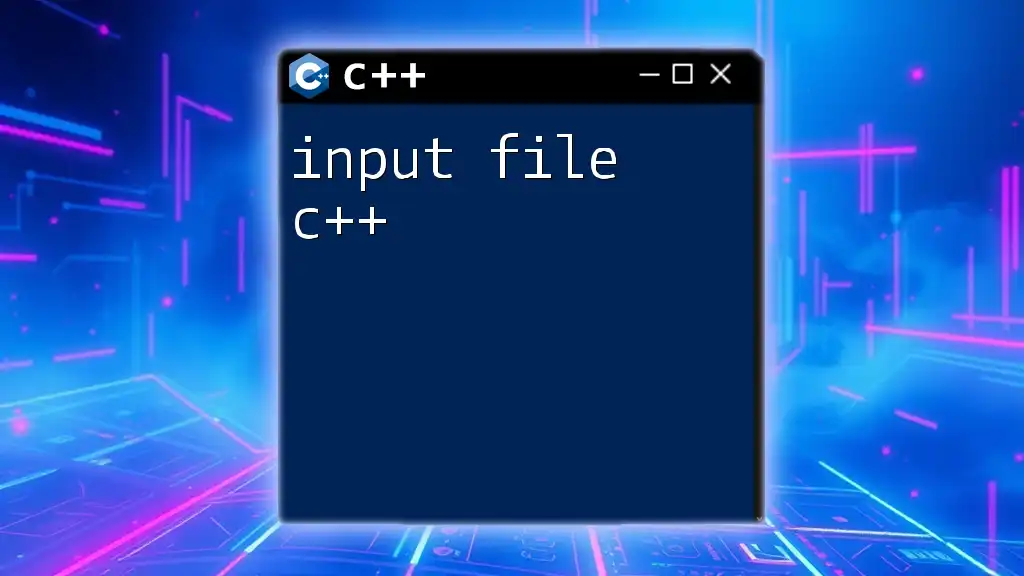
Reading from a File in C++
Opening a File for Reading
To read from a file, you’ll use the `ifstream` class. Here’s how you can open a file for reading:
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
This loop reads each line from "example.txt" until the end of the file is reached, outputting each line to the console.
Handling Errors While Reading
When working with files, it’s essential to handle errors that may occur. Use the following code snippet to ensure that the file opens correctly:
if (!inFile) {
std::cerr << "Error opening the file for reading!" << std::endl;
return 1;
}
This check prevents further operations on a file that wasn’t opened properly, avoiding potential runtime errors.
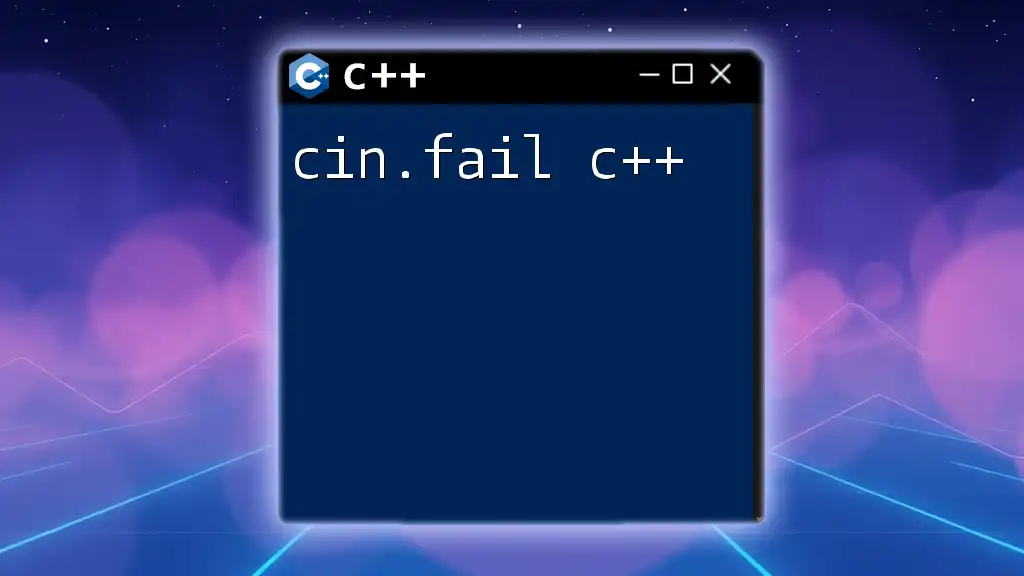
Error Handling in File Operations
Common Errors in File Handling
When performing file operations, common issues include:
- Permission denials (especially when writing)
- The specified file does not exist (when reading)
- General I/O errors while accessing the file
Best Practices for Error Handling
Utilizing exception handling can significantly simplify error management during file operations. Here’s an example of how to implement exception handling when working with files:
try {
std::ofstream outFile("example.txt");
// Writing operations...
} catch (const std::ios_base::failure& e) {
std::cerr << "File operation failed: " << e.what() << std::endl;
}
By wrapping file operations in a try-catch block, you can capture `ios_base::failure` exceptions, which handle file-related errors gracefully.
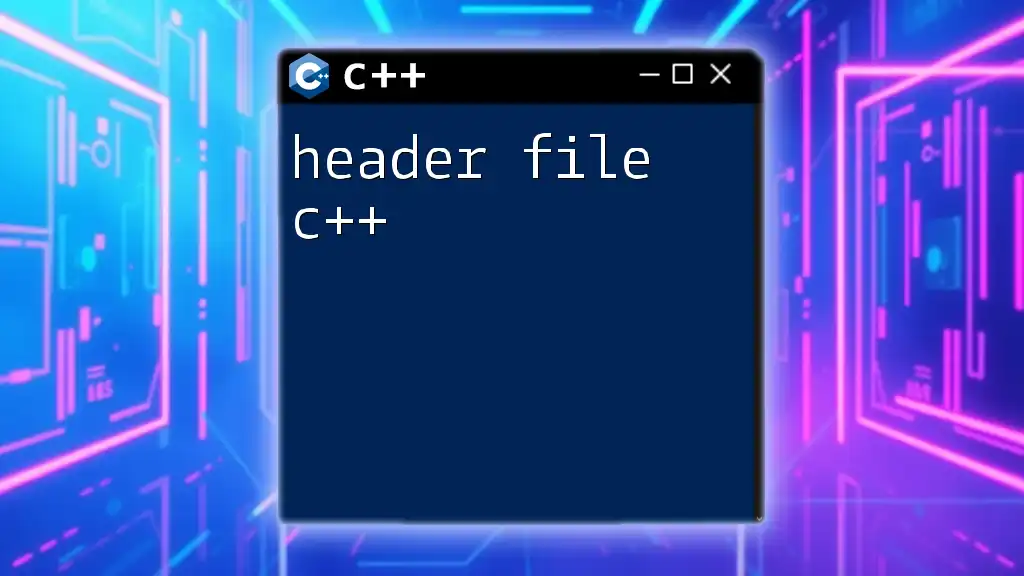
Advanced File Handling Techniques
Binary File Handling
In C++, binary files store data in a non-readable format, which can lead to more efficient I/O operations, especially for complex data structures. The approach to handling binary files differs slightly from text files.
To write to a binary file, you would use `std::ios::binary`:
std::ofstream outFile("example.bin", std::ios::binary);
int data = 12345;
outFile.write(reinterpret_cast<char*>(&data), sizeof(data));
outFile.close();
To read a binary file, the `ifstream` must also be opened in binary mode:
std::ifstream inFile("example.bin", std::ios::binary);
int data;
inFile.read(reinterpret_cast<char*>(&data), sizeof(data));
inFile.close();
Using File Streams Effectively
C++ provides the `seekg` and `seekp` functions to manipulate the position of the get and put pointers in your file streams, enabling you to read or write data at specific positions.
For example, to seek a specific position in a file:
outFile.seekp(0, std::ios::end); // Moves to the end for appending
Utilizing these functions allows for more sophisticated file manipulation, such as editing specific parts of a file without rewriting the entire contents.
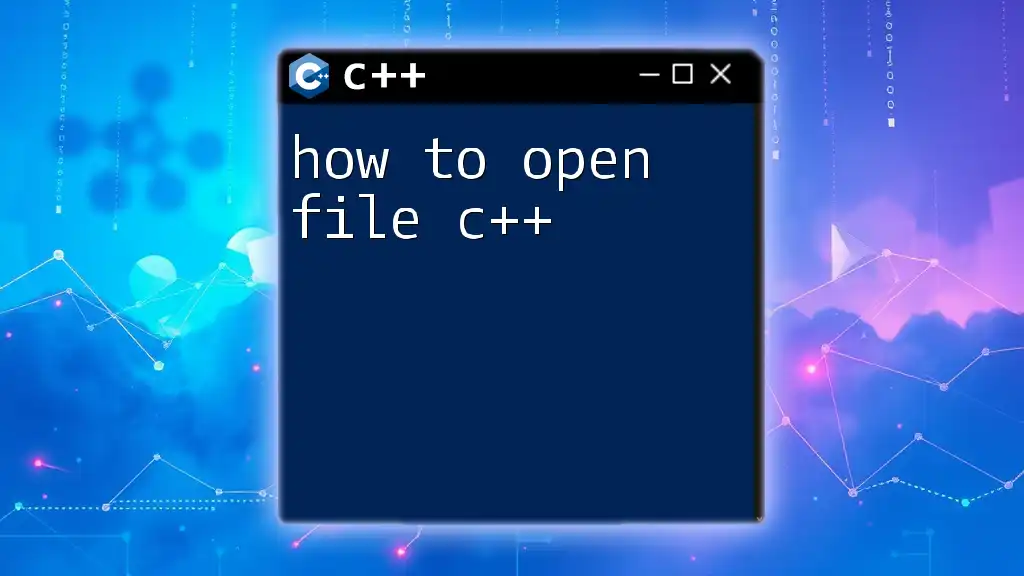
Conclusion
Through this guide, you've gained insights into the essential aspects of file management in C++. Understanding how to open, write, append, read, and handle errors effectively prepares you for a wide range of tasks involving file operations. Mastering these skills is paramount for any C++ developer, as file handling is a fundamental component of software functionality.
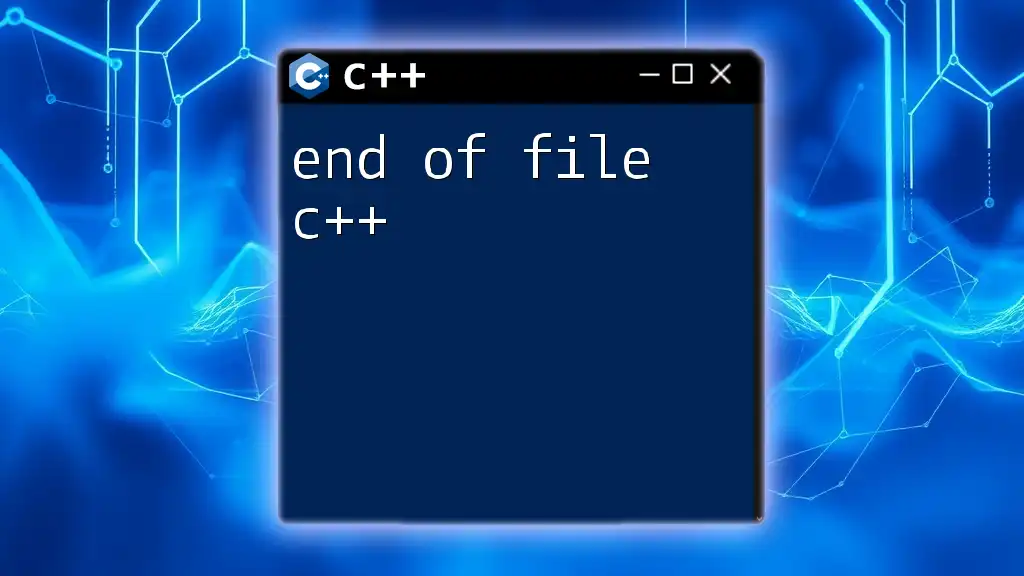
Additional Resources
For further exploration of file handling techniques in C++, consider checking out recommended books and online courses dedicated to advanced C++ programming. Continuous practice and exploration will enrich your file management skills and overall programming expertise.