To open a file in C++, you can use an `ifstream` (input file stream) object, specifying the filename, as shown in the following code snippet:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (!file) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
// File opened successfully
file.close();
return 0;
}
Understanding File Streams in C++
What are File Streams?
In C++, a file stream is an abstraction for reading from or writing to files. They allow you to treat files as streams of data, enabling you to perform file operations just like you would with console input and output. The file stream classes available in C++ are `ifstream`, `ofstream`, and `fstream`, each serving different purposes in file handling.
The Standard Library
To leverage the file stream capabilities, you must include the `<fstream>` header at the beginning of your program. This header contains the definitions necessary for file input and output operations. Here's how you can include it:
#include <fstream>
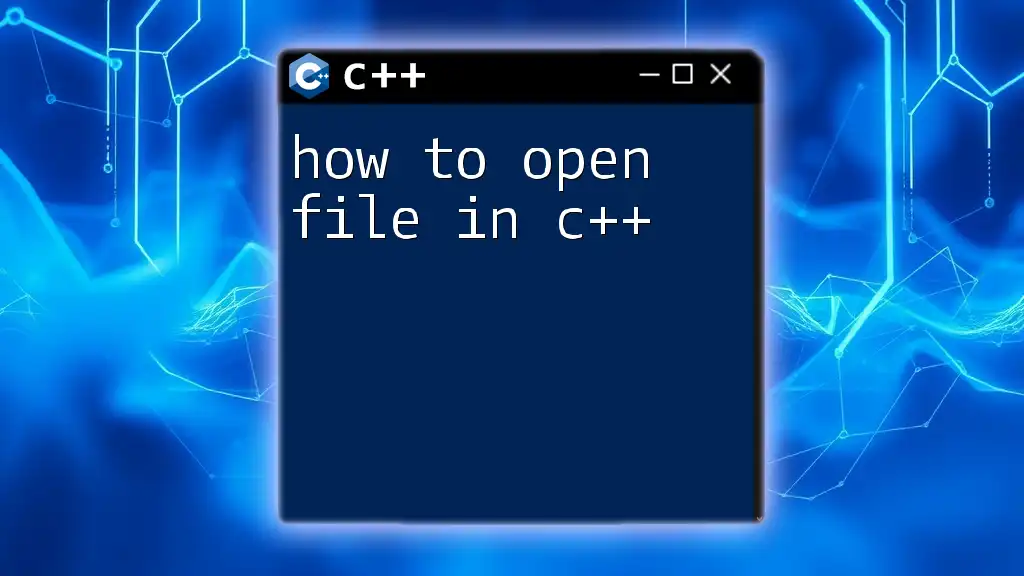
Basic Steps to Open a File
Choosing the Right Stream Type
When learning how to open a file in C++, selecting the appropriate stream type is paramount.
- Input Stream: Use `ifstream` when you want to read data from a file.
- Output Stream: Use `ofstream` if you intend to write data to a file.
- Input/Output Stream: Use `fstream` when you need both read and write functionality.
Syntax for Opening a File
The syntax for opening a file is straightforward. You can open a file by creating an instance of the appropriate stream object and passing the name of the file as a parameter. Here’s an example for both input and output files:
std::ifstream inputFile("example.txt");
std::ofstream outputFile("example.txt");
By default, opening an `ofstream` will overwrite the file if it already exists, while an `ifstream` will require the file to be present.
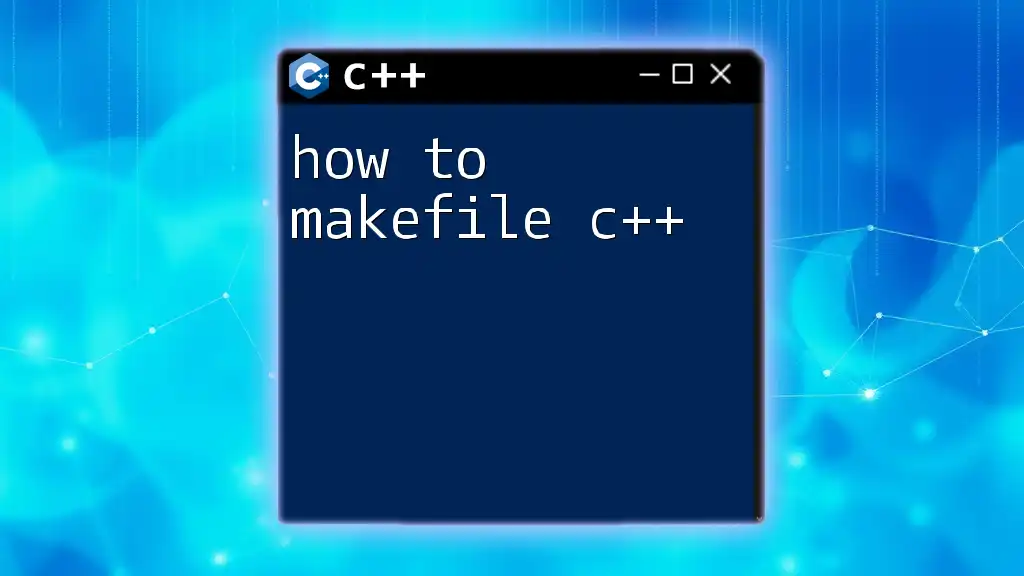
Opening a File for Reading
Using `ifstream`
To open a file for reading, utilize `ifstream`. This object allows you to read data from the file effectively. It’s crucial to check whether the file opened successfully to avoid runtime errors.
Here's an example of how to open a file and check if it was successful:
std::ifstream inputFile("example.txt");
if (!inputFile) {
std::cerr << "Error opening file." << std::endl;
}
Reading Data from the File
Once you've successfully opened a file, you can begin reading from it. The common methods to read data include `getline` for reading entire lines and the extraction operator (`>>`) for reading formatted data.
Here's an example of using `getline`:
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
This code reads each line from the file until the end and outputs it to the console.
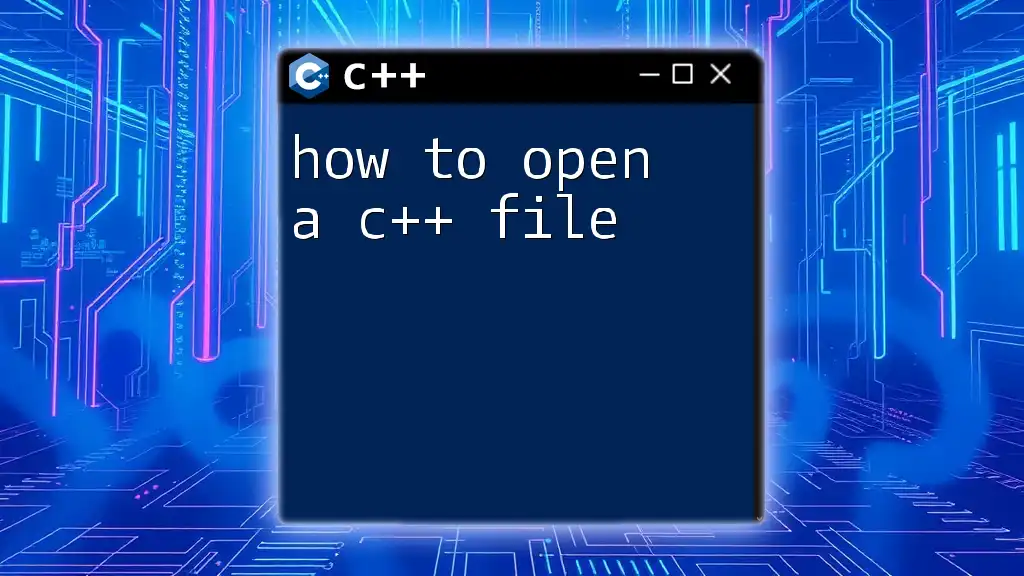
Opening a File for Writing
Using `ofstream`
To open a file for writing, you need to instantiate an `ofstream` object. If the specified file does not exist, it will be created; if it does exist, it will be overwritten.
std::ofstream outputFile("output.txt");
if (!outputFile) {
std::cerr << "Error creating file." << std::endl;
}
Writing Data to the File
You can write data using the insertion operator (`<<`) or the `write` method for binary files.
Here’s an example illustrating simple text writing:
outputFile << "Hello, World!" << std::endl;
This code writes the string "Hello, World!" to the file followed by a new line.
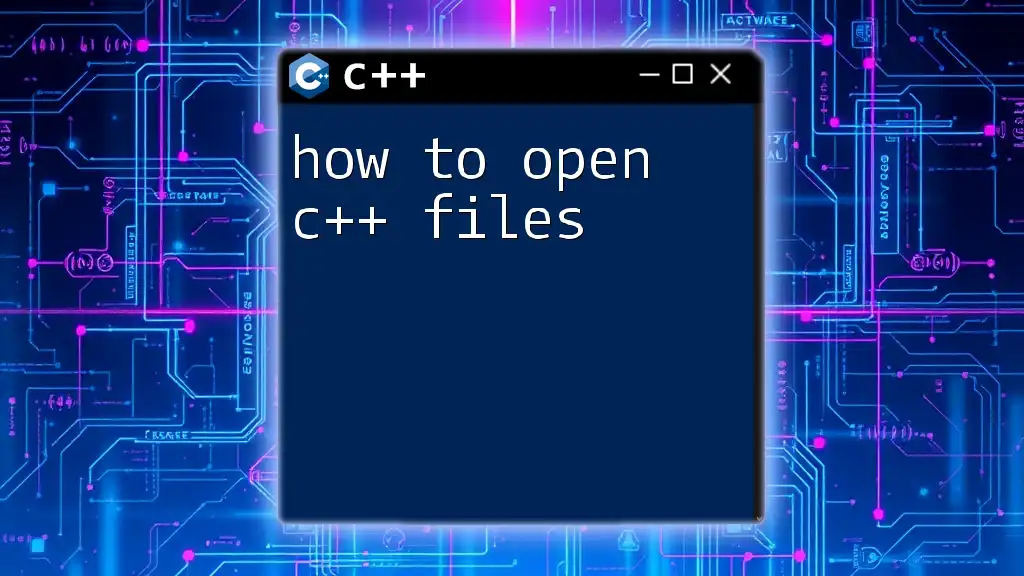
Opening a File for Both Reading and Writing
Using `fstream`
When you need to both read from and write to a file, using `fstream` is the way to go. This allows you to perform read/write operations in the same file stream.
std::fstream file("example.txt", std::ios::in | std::ios::out | std::ios::app);
if (!file) {
std::cerr << "Error opening file." << std::endl;
}
In this example, the `ios::app` flag appends the data to the end of the file, preserving existing content.
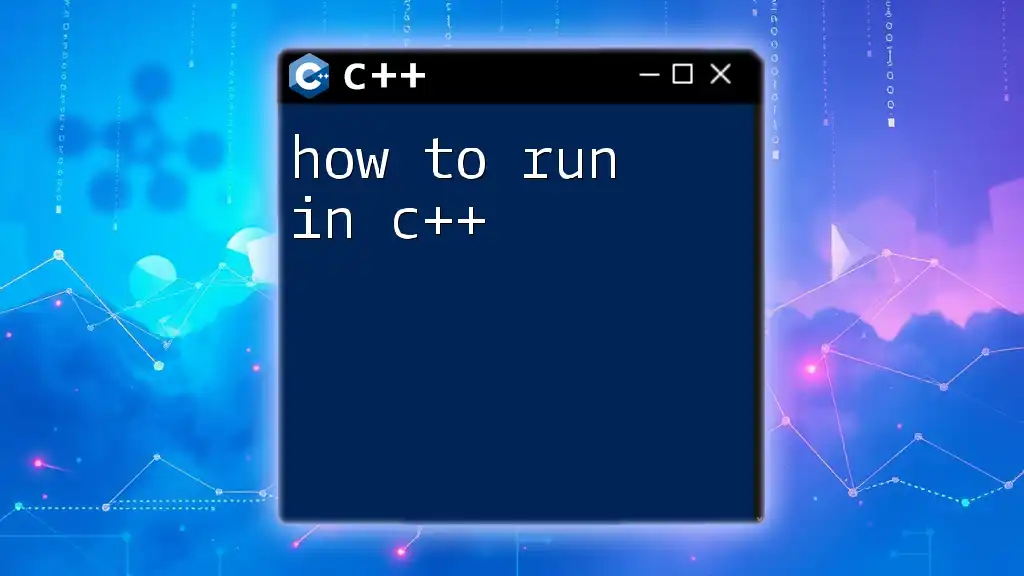
Handling File Errors
Checking File State
Error handling is a significant aspect of working with files in C++. It’s important to check the state of your file stream during and after file operations to ensure that everything worked correctly.
You can utilize various member functions to check the state of the file like `fail()` or `good()`, which indicate if the last operation was successful or if there are errors.
Example:
if (inputFile.fail()) {
std::cerr << "File operation failed." << std::endl;
}
Closing the File
Once you're finished with file operations, it’s essential to close the file using the `close()` method. This step helps prevent memory leaks and ensures all data is properly written or flushed.
inputFile.close();
outputFile.close();
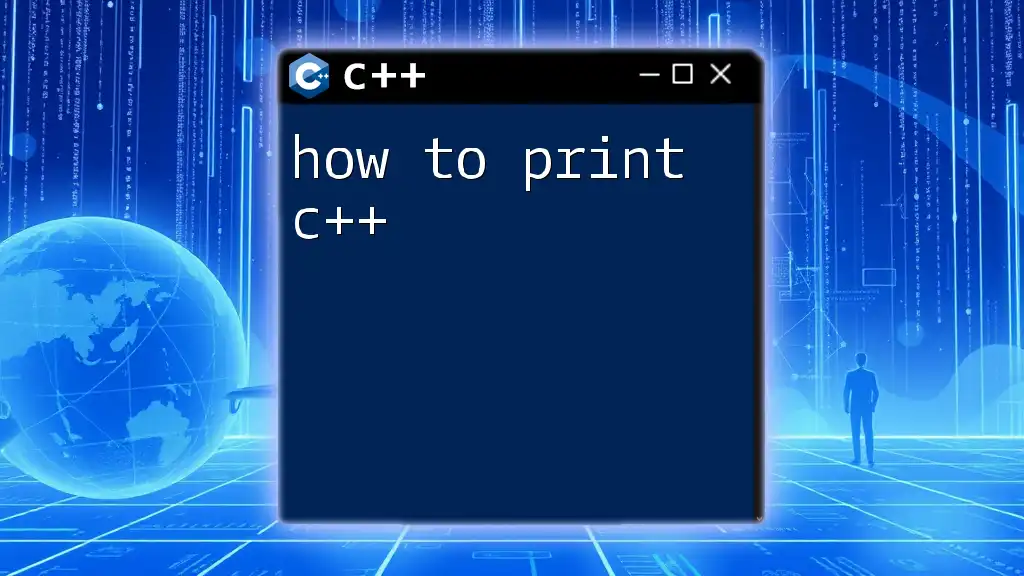
Conclusion
Now you have a comprehensive understanding of how to open a file in C++, including the different stream types, how to read from and write to files, and the importance of error handling. Mastering these concepts will significantly enhance your file manipulation skills in C++. Practice with different file operations to familiarize yourself further with the nuances of file handling in C++.
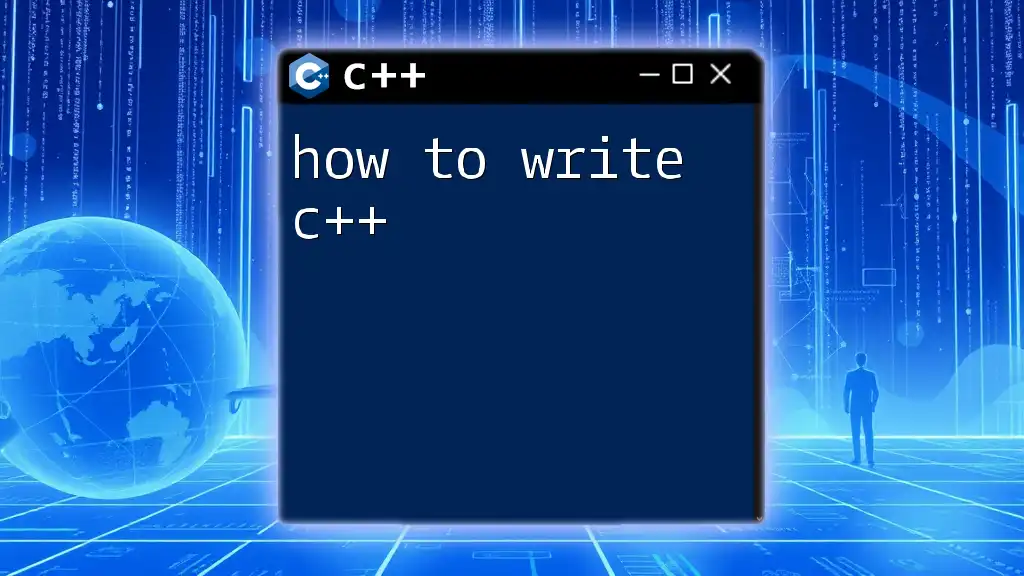
Further Resources
For deeper learning, consider consulting the C++ official documentation or exploring books and online platforms that dive into advanced file I/O techniques. These resources can provide valuable insights and additional examples for enhancing your programming prowess.