To open a C++ file, you can use the `ofstream` class from the `<fstream>` library to create an output file stream object and specify the name of the file you want to open.
Here's a code snippet demonstrating this:
#include <fstream>
int main() {
std::ofstream file("example.txt");
if (file.is_open()) {
// File opened successfully
}
return 0;
}
Understanding C++ Files
What are C++ Files?
C++ files consist of source code and declarations used to create programs in the C++ programming language. These files typically contain code written by developers, and understanding their structure and purpose is essential for effective programming.
File Extensions Explained
C++ files come in various types, each serving a distinct role:
- .cpp: These are the primary source files that contain the actual C++ code that gets compiled into an executable.
- .h & .hpp: Header files that usually contain declarations for functions and classes, enabling code separation and modular programming.
- .o: Object files created when the compiler translates `.cpp` files into machine code, ready for linking.
- .exe: Executable files that your operating system can run directly after the program is compiled.
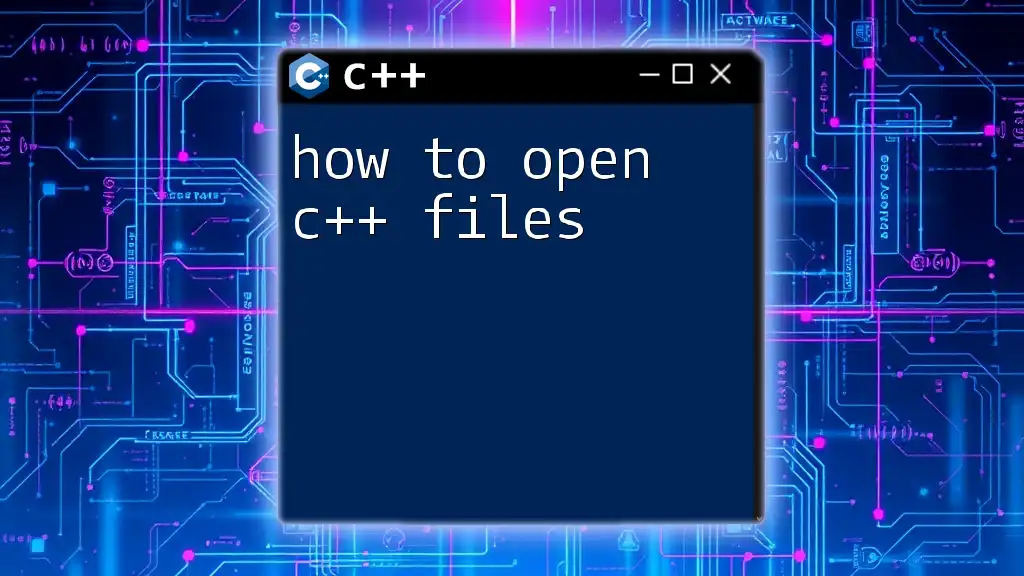
Tools Needed for Opening C++ Files
Text Editors and IDEs
When working with C++ files, choosing the right tool enhances productivity. Text editors are lightweight and great for quick edits, while Integrated Development Environments (IDEs) provide advanced features like debugging, autocompletion, and project management.
Recommended tools include:
- Visual Studio Code: Popular for its flexibility and vast array of extensions tailored for C++ programming.
- Code::Blocks: An open-source IDE tailored specifically for C and C++ development.
- CLion: A powerful IDE from JetBrains that offers deep code insight and integrates smoothly with CMake.
- Dev-C++: A simple yet effective IDE for beginners learning C++.
Installation and Setup
Setting up these tools is usually straightforward. Follow the official documentation for the selected tool, ensuring you have the necessary compilers installed, like GCC or MinGW for Windows.
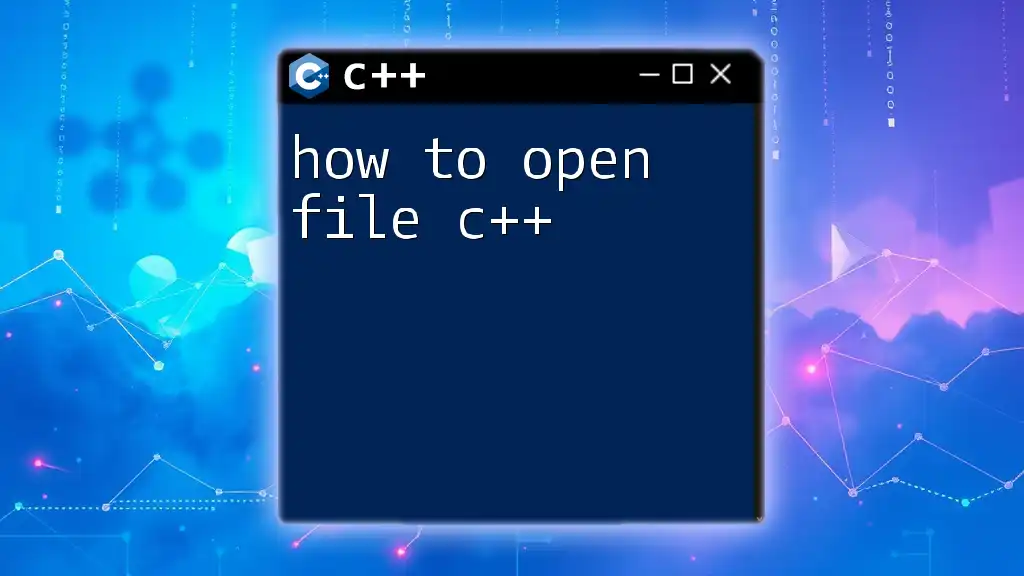
How to Open C++ Files
Using a Text Editor
To open a C++ file in a basic text editor, follow these steps. For this example, let's say you have a C++ source file named `hello.cpp` containing:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- Launch your text editor (for instance, Notepad on Windows or TextEdit on macOS).
- Navigate to the directory where `hello.cpp` is stored.
- Open the file via the editor's file menu or drag-and-drop.
Text editors often provide basic syntax highlighting, making it easier to read and edit your code.
Using an IDE
Using an IDE like Visual Studio Code enhances workflow significantly. Here’s how to open a C++ file:
- Install Visual Studio Code: Download and install from the official website.
- Install C++ Extension: Go to the Extensions view by clicking on the Extensions icon in the Activity Bar. Search for and install the C/C++ extension by Microsoft.
- Open Visual Studio Code: Launch the IDE.
- Open the File: Click on `File` > `Open File…` and navigate to `hello.cpp`.
Furthermore, the IDE provides features such as code suggestions, debugging aids, and even integration with version control systems, thereby improving the overall development experience.
Using Command Line (Terminal)
If you prefer command-line tools, here’s how to open a C++ file from the terminal.
- On Windows, you can use Notepad:
notepad hello.cpp
- On macOS/Linux, you might use Nano:
nano hello.cpp
For terminal-based text editors like Vim, you can open a file using:
vim hello.cpp
Remember, basic commands in Vim include `i` for insert mode and `:wq` to save and quit.
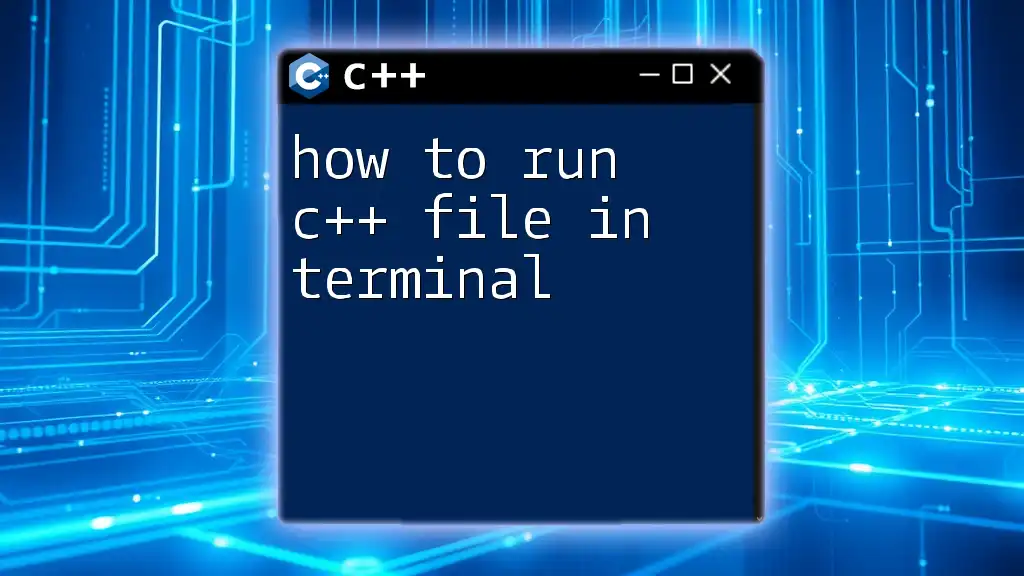
Saving Changes after Opening a C++ File
After making changes to your C++ file, saving is critical to ensure your edits are not lost.
In a text editor, you typically navigate to `File` > `Save` or use the shortcut Ctrl + S (or Cmd + S on macOS).
In IDEs like Visual Studio Code, the same save command applies, but be sure to double-check the file format. Saving as a `.cpp` file is crucial to maintain compatibility with the C++ compiler.
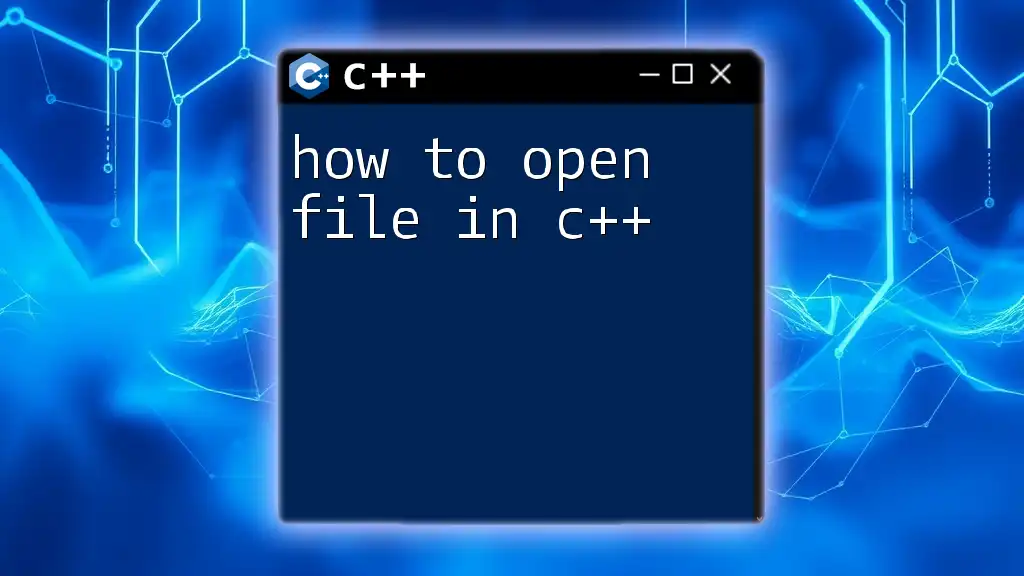
Best Practices When Working with C++ Files
Organizing Your Files
Maintaining a structured directory for your C++ projects helps streamline the development process. A well-organized structure might look like:
/project_folder
/src // for .cpp files
/include // for .h files
/bin // for executables
/lib // for libraries
This organization not only keeps your files tidy but also simplifies navigation and compilation tasks.
Version Control with Git
Using a version control system like Git is invaluable for tracking changes in your C++ files. It enables collaboration, keeps a history of modifications, and provides an easy way to revert to earlier versions.
To start using Git:
- Initialize a repository in your project folder:
git init
- Add files to the staging area:
git add .
- Commit your changes:
git commit -m "Initial commit"
- Push to a remote repository (like GitHub) when ready.
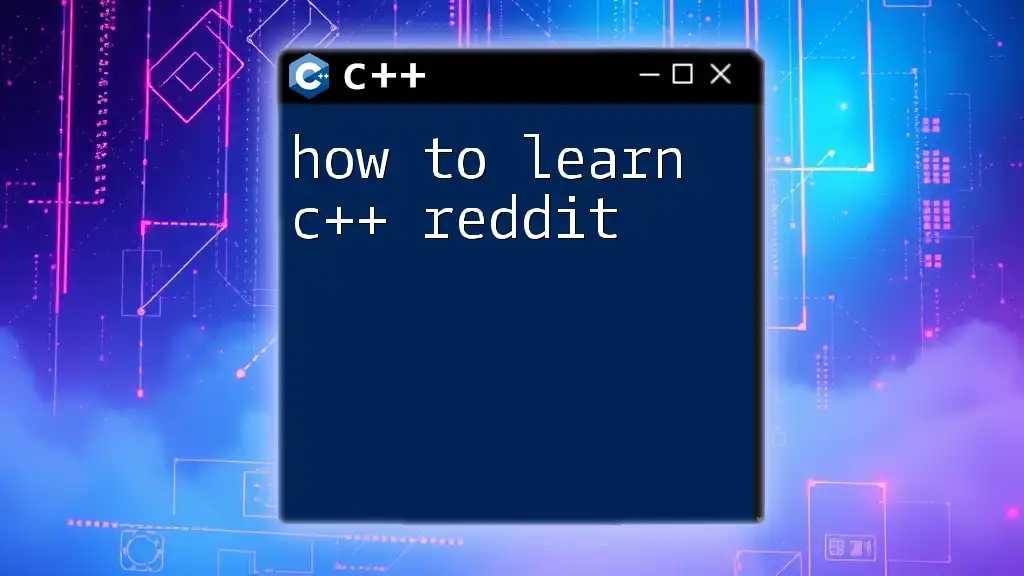
Troubleshooting Common Issues
Unable to Open a File
If you encounter issues opening a file, consider checking the following:
- File Path: Ensure that the path to the file is correct.
- Permissions: Make sure you have the necessary permissions to read the file. On Linux or macOS, you can change permissions using `chmod`.
Syntax Errors After Opening
After opening a file, syntax errors might still appear. Common issues include forgetting to close braces or missing semicolons. Therefore, it is essential to run your code through a compiler or use the built-in features of your IDE to identify problems before runtime.
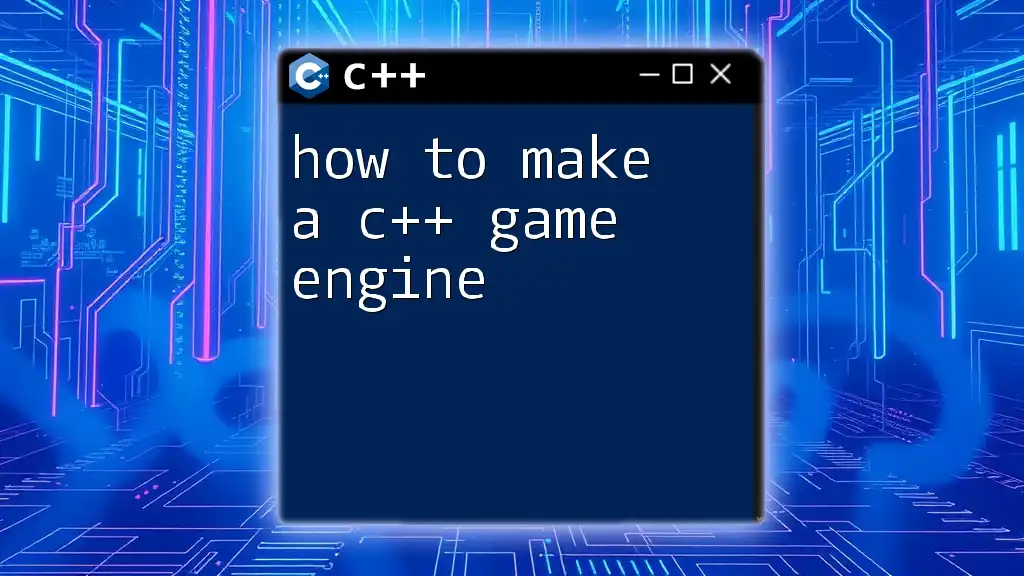
Conclusion
Knowing how to open a C++ file is a foundational skill for anyone interested in programming. By leveraging various tools, understanding file types, organizing your projects, using version control, and following best practices, you can enhance your programming workflow significantly. Dive in and start experimenting with your C++ files today!
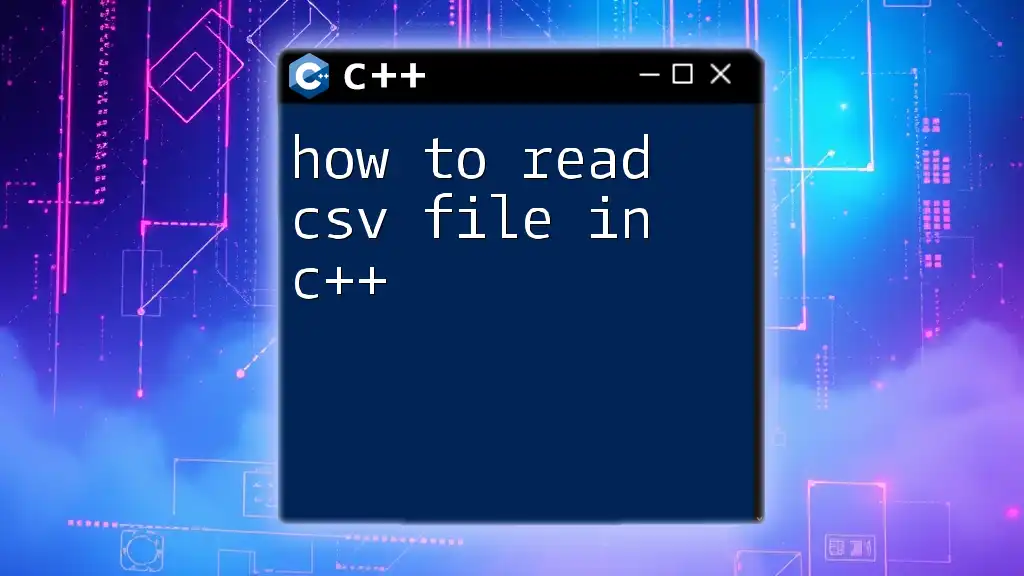
Additional Resources
For further reading and exploration, consider checking official documentation, guides, and online communities dedicated to C++ programming. These resources can provide additional support and deepen your understanding of the language.