To read a CSV file in C++, you can use the `<fstream>` library to open the file and parse its contents line by line using the `getline` function.
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
int main() {
std::ifstream file("data.csv");
std::string line, value;
while (std::getline(file, line)) {
std::stringstream s(line);
while (std::getline(s, value, ',')) {
std::cout << value << " ";
}
std::cout << std::endl;
}
return 0;
}
Understanding CSV Files
What is a CSV file?
CSV stands for Comma-Separated Values. It is a simple file format used to store tabular data, such as spreadsheets or databases. A CSV file consists of one or more lines, each containing values separated by commas. For example:
Name, Age, Occupation
Alice, 30, Developer
Bob, 25, Designer
Charlie, 35, Manager
In this format, each line represents a record, and each record consists of fields separated by commas. This structure makes it easy for both humans and machines to read and parse.
Why Use CSV Files?
CSV files are widely used due to their simplicity. Here are some of the key advantages:
- Human-readable: The plain text format makes it easy to view and understand the data.
- Lightweight: CSV files have a small size, making them efficient for data storage and transfer.
- Ease of use: Many applications, including Excel, can read and write CSV files, facilitating data exchange between systems.
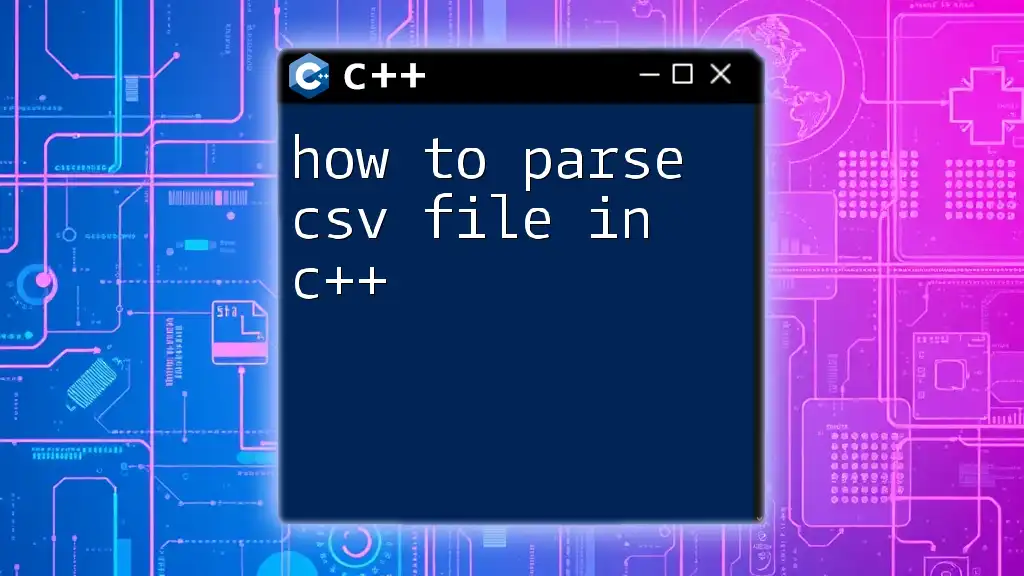
Getting Started with C++ for CSV File Handling
Setting Up Your C++ Environment
Before diving into code, ensure you have a suitable development environment. Recommended IDEs include Visual Studio, Code::Blocks, or CLion. You can also use a simple text editor alongside a terminal.
- Install a C++ compiler like GCC or use an IDE that includes one.
- Create a new C++ project or file where you'll write your code.
Necessary Libraries and Headers
For reading CSV files in C++, you will primarily need the following headers:
- `fstream`: For file input and output.
- `string`: To handle string manipulations conveniently.
These headers are essential for file operations and processing textual data.
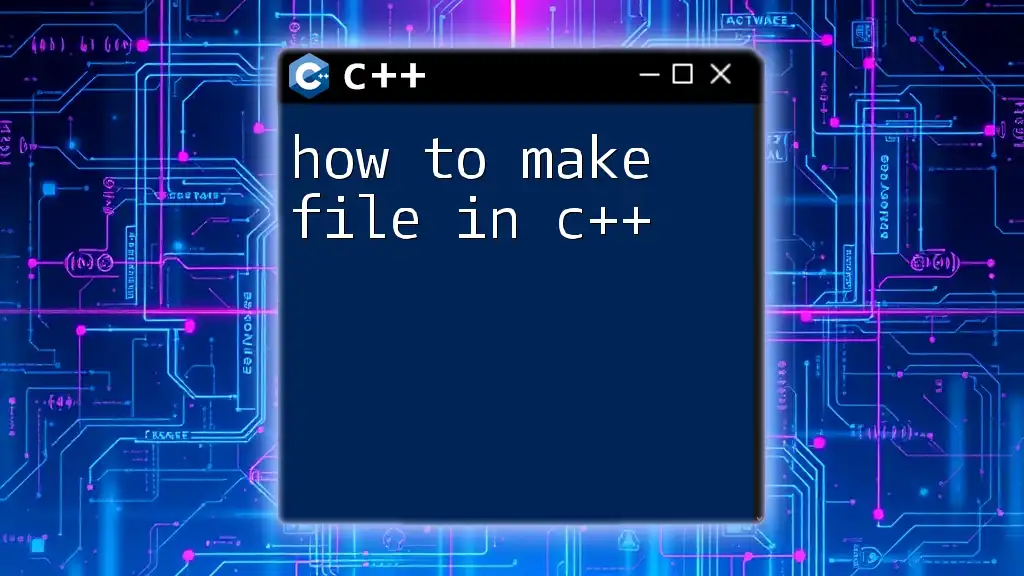
How to Read a CSV File in C++
Open the CSV File
To read a CSV file, the first step is to open it using an `ifstream` object. The following code snippet demonstrates how to do this:
#include <fstream>
#include <string>
std::ifstream file("data.csv");
if (!file.is_open()) {
// Handle error
std::cerr << "Error opening file!" << std::endl;
return 1;
}
Here, we attempt to open the "data.csv" file. It’s crucial to check if the file opened successfully; if not, handle the error gracefully.
Reading Data from the CSV File
Using `getline` for Reading Lines
Once the file is open, you can read it line by line using the `getline()` function. This function reads a line from the file and stores it in a string. Here’s how it works:
std::string line;
while (getline(file, line)) {
// Process line
}
In this loop, each line from the CSV file is read until the end of the file is reached. You can then process each line as needed.
Tokenizing the Line
To extract individual values from each line, you’ll need to tokenize it. A common way to do this in C++ is by utilizing `std::stringstream`. Here’s an example of the tokenization process:
#include <sstream>
std::stringstream ss(line);
std::string value;
while (getline(ss, value, ',')) {
// Process value
}
In this snippet, we create a `stringstream` object from the line. The `getline()` function is then used again, but this time to split the line by commas, allowing you to process each value independently.
Handling Different Data Types
CSV files often contain a mix of data types. When reading values, you typically start as strings and may need to convert them to other types for calculations or logic. This can be done using functions like `std::stoi` for integers or `std::stof` for floats. For instance:
int intValue = std::stoi(value); // Convert string to int
When using these functions, ensure that the string indeed holds a valid representation of the desired type to avoid errors.
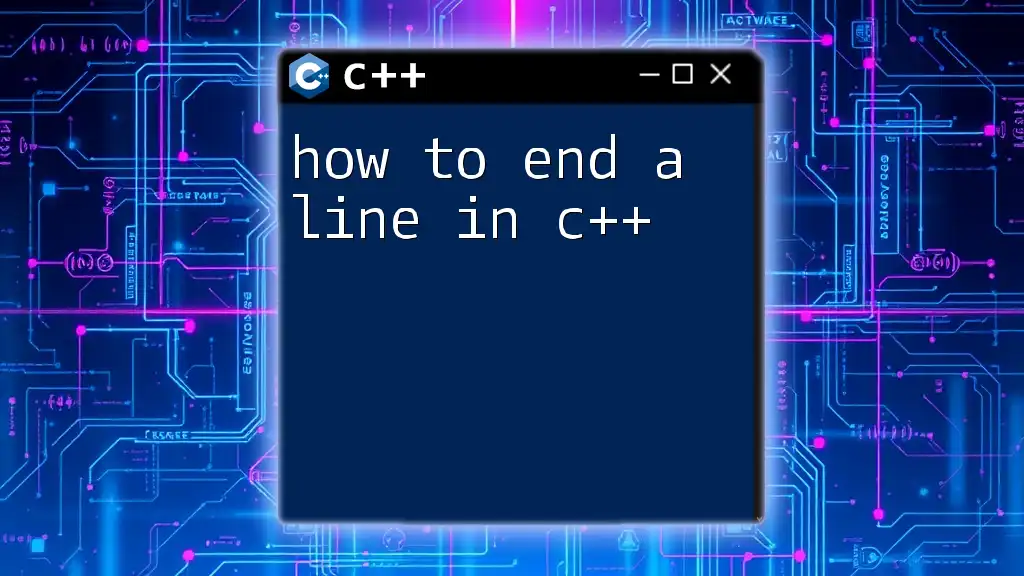
Complete Example of Reading a CSV File in C++
Here’s a complete program that demonstrates how to read data from a CSV file. This program reads data from "data.csv", tokenizes it, and prints each value to the console:
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
int main() {
std::ifstream file("data.csv");
if (!file.is_open()) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
std::string line;
while (getline(file, line)) {
std::stringstream ss(line);
std::string value;
while (getline(ss, value, ',')) {
std::cout << value << " "; // Output each value
}
std::cout << std::endl;
}
file.close();
return 0;
}
Explanation of Code Structure
- File opening: The program attempts to open "data.csv".
- Line reading: It reads each line one by one.
- Value extraction: Each line is split into individual values and printed to the console.
- File closing: Finally, the file is closed to release resources.
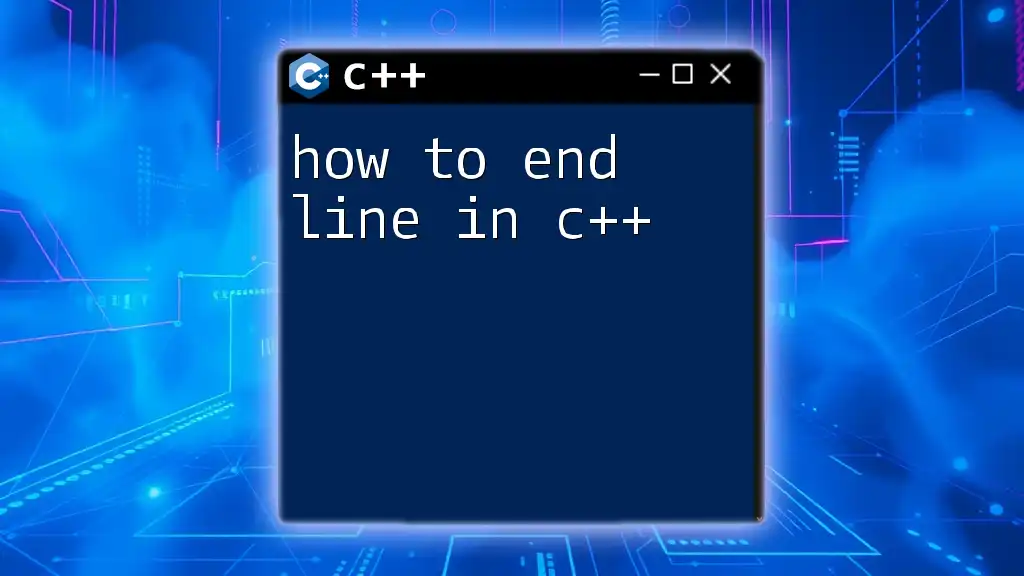
Common Issues When Reading CSV Files in C++
File Not Found
One common issue when attempting to read a CSV file is the file not being found. Ensure the path is correct relative to the execution directory of your program. A simple debug point is to print the path or directly use an absolute path for testing.
Handling Different Delimiters
While commas are standard, sometimes CSV files can use different delimiters, such as semicolons or tabs. You can easily adapt your tokenization code to change the delimiter:
while (getline(ss, value, ';')) { // Change ',' to ';'
// Process value
}
Managing Large CSV Files
When dealing with larger CSV files that may not fit into memory, consider reading them line by line as demonstrated. You can also implement buffer reading techniques, or if needed, memory-mapped files for advanced performance optimizations.
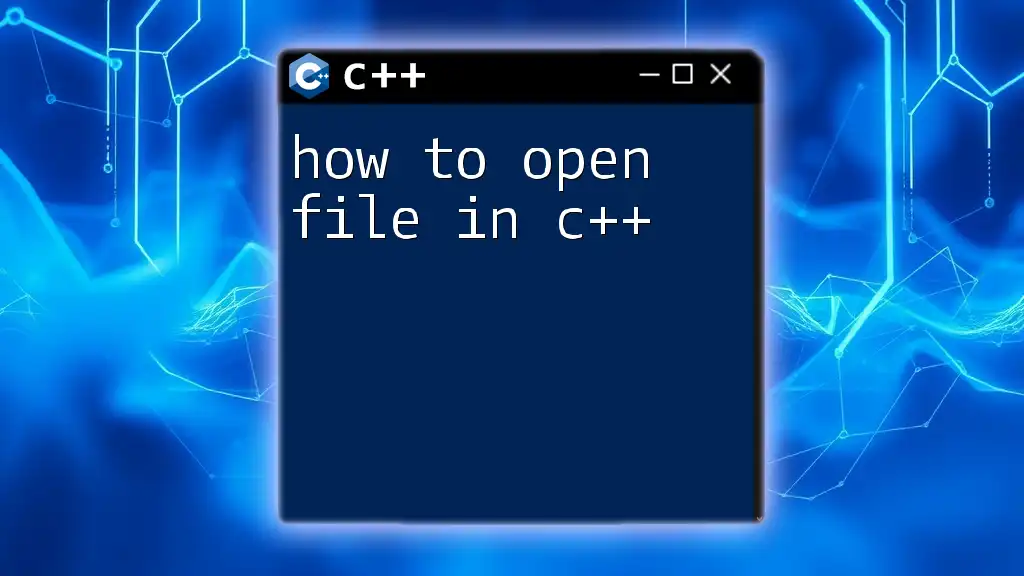
Conclusion
Understanding how to read CSV files in C++ opens up opportunities for effective data management and processing. By mastering file handling, string manipulation, and basic data type handling, you're equipped to efficiently manage CSV files in your applications. Practice with real CSV files will solidify your skills, and diving into further resources will help you grow even more proficient in C++.