Header files in C++ are files that typically contain declarations of functions, classes, and variables, allowing code to be organized, reused, and shared across multiple source files.
Here’s a simple example of a header file and its usage:
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
void sayHello();
#endif
// main.cpp
#include <iostream>
#include "myheader.h"
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
sayHello();
return 0;
}
What is a C++ Header File?
A header file in C++ is a crucial component that plays a significant role in structuring and organizing your code. It serves as a central repository for declarations of functions, classes, and variables, enabling the code to be modular and easily maintainable. Header files help distinguish between declarations and definitions, facilitating a clearer understanding of how different parts of a program interact.
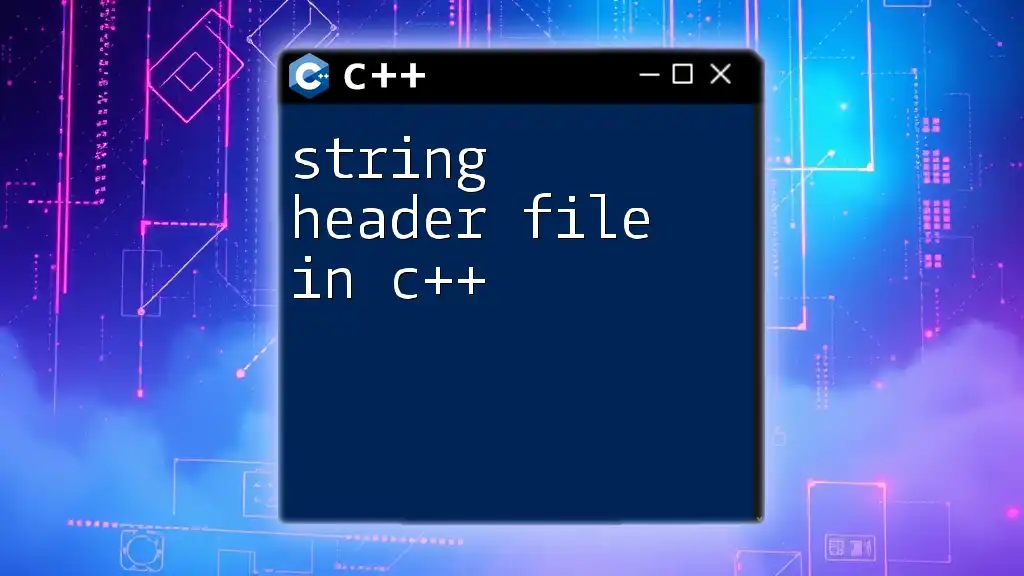
What are Header Files in C++?
Purpose of Header Files
The primary purpose of header files is to promote code reuse and modular programming. They allow different parts of a program to communicate with each other without requiring full knowledge of the implementation details. Here are the main reasons for using header files in C++:
- Cleaner Code Organization: Header files aid in segmenting different functionalities into separate files, making the codebase easier to navigate and understand.
- Encapsulation: Users can work with an interface without needing to understand the underlying implementation. This encapsulation helps manage complexity in larger software projects.
Types of Header Files
In C++, header files can be categorized into two main types:
-
Standard Library Headers: These are predefined headers that come with the C++ standard library. For example:
- `<iostream>`: Contains functionality for input and output operations.
- `<vector>`: Implements the vector container supporting dynamic array operations.
-
User-Defined Headers: Developers can create custom headers tailored to their applications. This enables encapsulation of specific functionality that can be reused across various parts of the project.
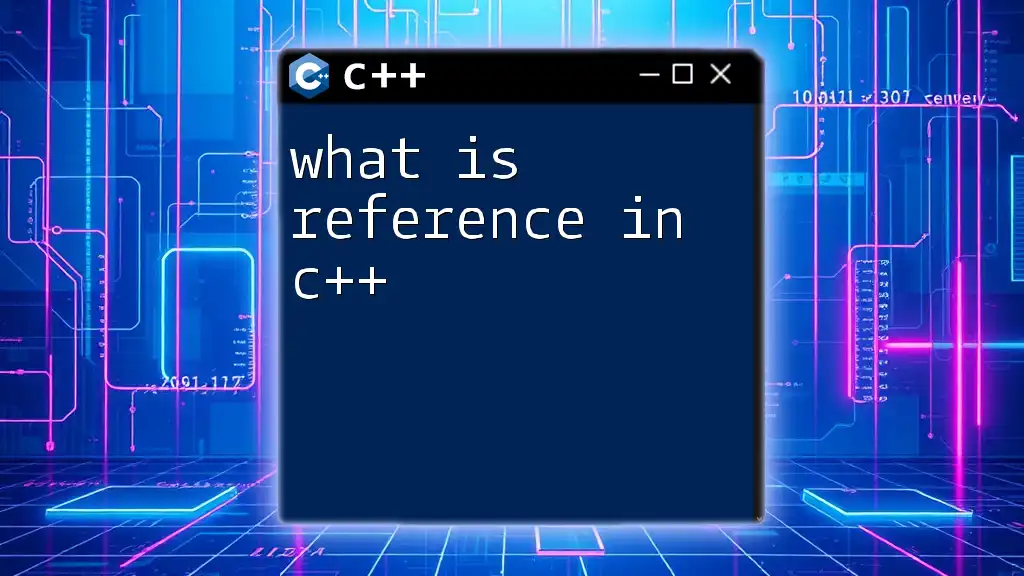
Components of a C++ Header File
Basic Structure of a Header File
A typical header file consists of function and class declarations. It’s essential to include include guards to prevent multiple inclusions, which can cause errors during compilation. The basic structure of a header file can be organized as follows:
#ifndef HEADER_NAME_H
#define HEADER_NAME_H
// Function and class declarations
#endif // HEADER_NAME_H
Syntax to Include a Header File
When you want to use the definitions from a header file, you include it in your source code using the `#include` directive.
#include "myclass.h"
Using quotes specifies that the header file is located in the current directory, while angle brackets (`< >`) are used for standard library headers.
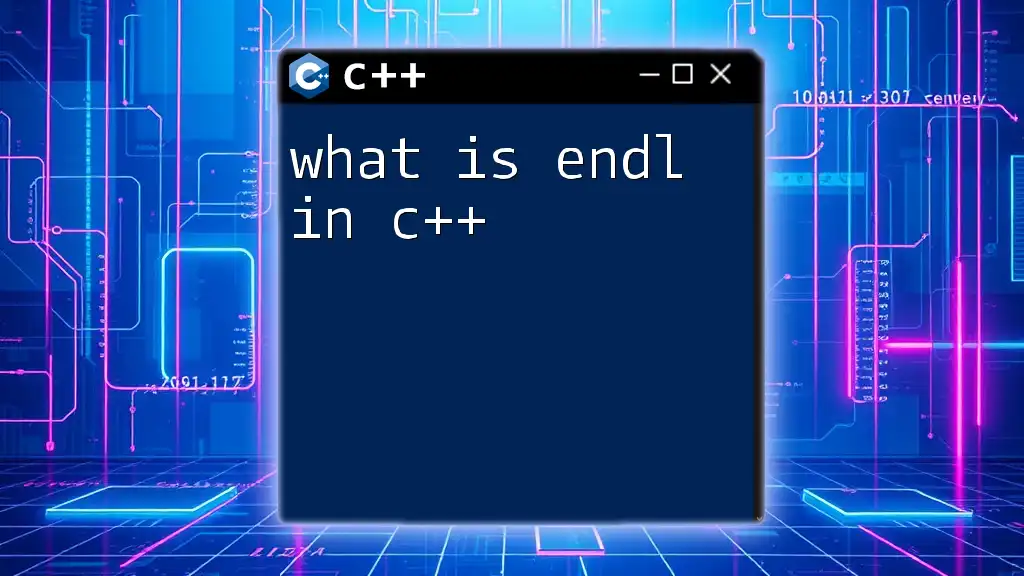
C++ Header File Example
Creating a Custom Header File
Let’s create a simple custom header file named `myclass.h` that demonstrates a class definition.
// myclass.h
#ifndef MYCLASS_H
#define MYCLASS_H
class MyClass {
public:
void display();
};
#endif // MYCLASS_H
Including and Using the Header File in a Source File
Next, we will include our custom header in a source file named `main.cpp` and implement the display method.
// main.cpp
#include "myclass.h"
#include <iostream>
void MyClass::display() {
std::cout << "Hello from MyClass!" << std::endl;
}
int main() {
MyClass obj;
obj.display();
return 0;
}
In this example, the `MyClass` class is declared in the header file, and its method `display` is defined in the source file. This separation of declaration and definition illustrates the core purpose of header files.
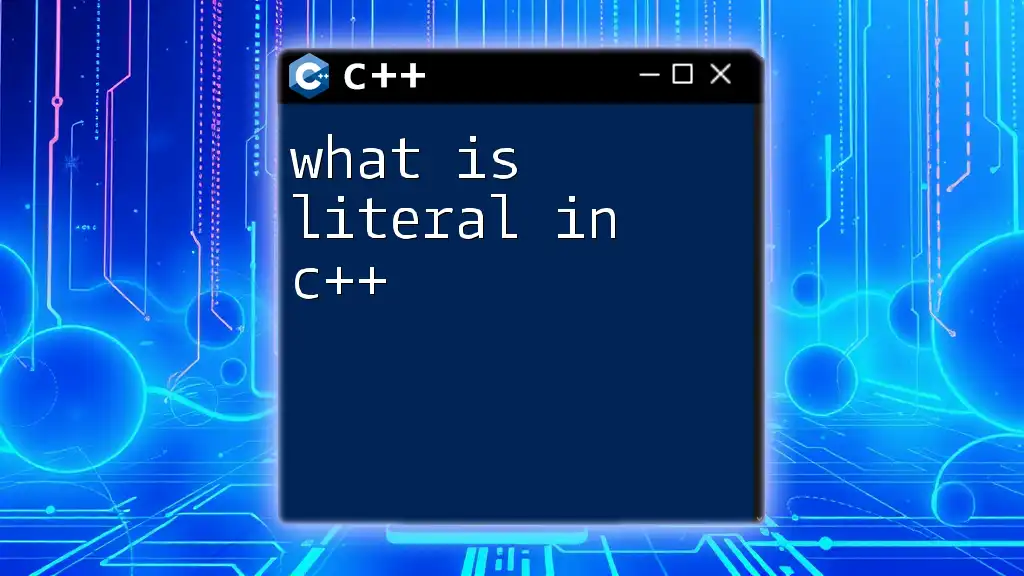
Benefits of Using Header Files in C++
Code Organization and Clarity
Using header files significantly improves the organization of your C++ code. By clearly separating declarations from definitions, it becomes easier to understand the relationships between different components. This modularity is particularly advantageous in large projects, where comprehending a singular file may become cumbersome.
Reusability
Header files bolster code reusability by allowing developers to define functions and classes only once and utilize them in multiple source files. This not only saves time but also minimizes errors, as the same definition will always remain consistent across the project.
Faster Compilation
In larger software projects, compilation can be time-consuming. Header files expedite this process by allowing the compiler to only recompile source files that have changed while maintaining compiled versions of unchanged files. This modular approach to coding translates into reduced compile times and improved overall efficiency.
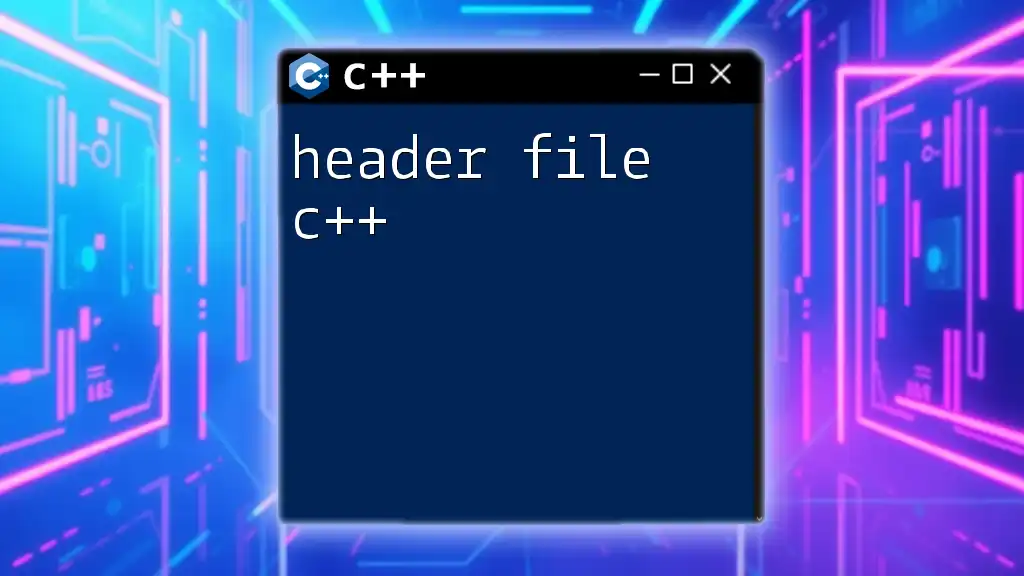
Common Practices for Managing Header Files
Naming Conventions
Establishing a clear naming convention for header files is vital for maintaining clarity. For example, using suffixes like `.h` for header files and ensuring names are descriptive helps other developers (and yourself) understand the file's content at a glance.
Avoiding Circular Dependencies
Circular dependencies occur when two header files include each other, leading to confusion and potential errors. To resolve this issue:
- Restrict includes to the necessary headers only.
- Use forward declarations if possible, where only class declarations are needed without including the full header.
Maintaining Include Order
The order of includes in a file can greatly affect the structure and behavior of your code. Adhering to a consistent include order—standard libraries first, followed by third-party libraries, and finally your own headers—can prevent a myriad of complications during compilation.
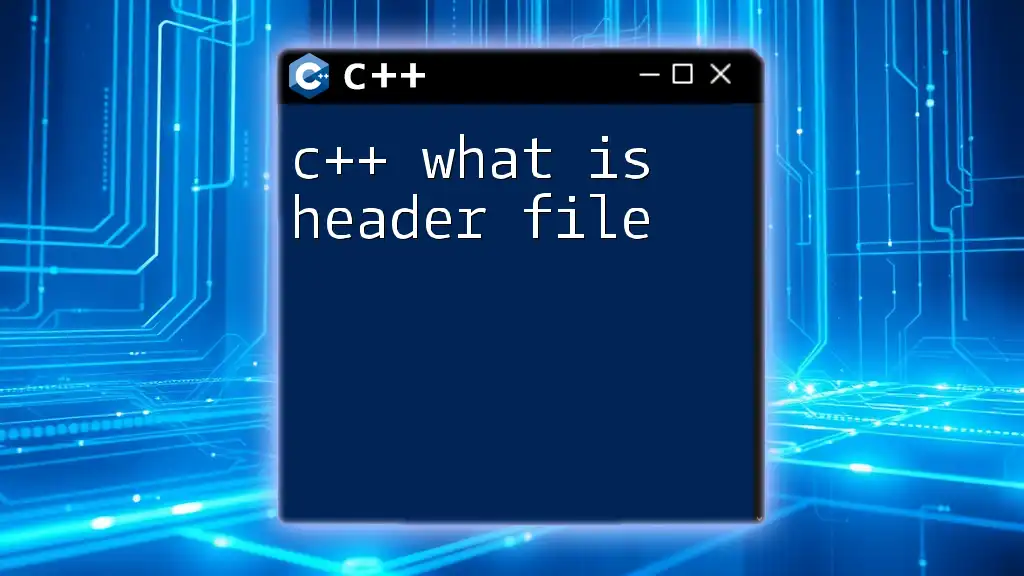
Conclusion
C++ header files are an essential feature of the language, allowing for better organization, modularity, and reusability. Understanding what header files are and their importance not only enhances your programming skills but also contributes to writing efficient, maintainable code. By implementing the best practices discussed, you can streamline your development process and ensure a robust codebase.
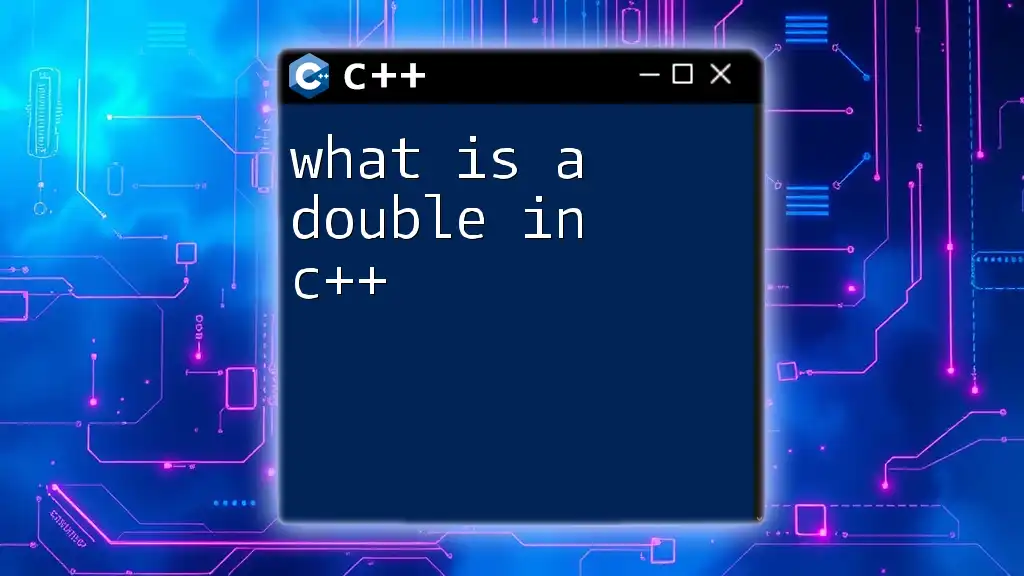
Call to Action
If you're keen to learn more about C++ programming and improve your coding practices, consider exploring additional resources or joining a community dedicated to C++. Embrace header files, and take your programming to the next level!