In C++, `void` is a keyword used to indicate that a function does not return a value.
Here’s a simple example of a function using `void`:
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
What Does Void Mean in C++?
The term `void` in C++ signifies the absence of a value. It's a fundamental keyword that plays a pivotal role in defining the actions of functions. Unlike other data types, such as `int`, `float`, or `char`, which have specific value ranges, `void` denotes no data at all.
For instance, when compared to an `int`, which can hold numeric values, a void type essentially states, "There is nothing to see here." This distinction is critical in situations where you are designing functions that perform tasks but do not return any data to the caller.
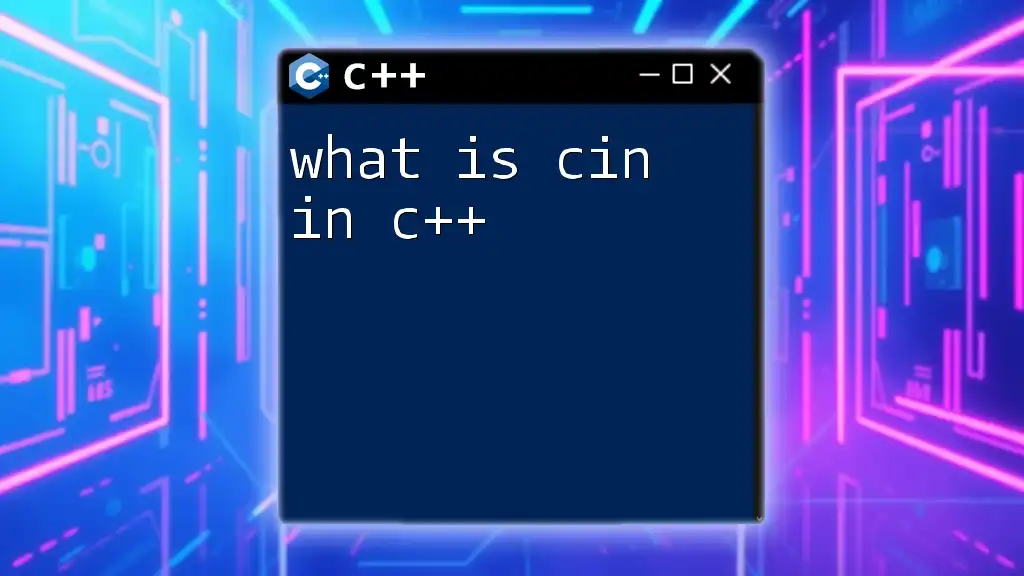
Understanding C++ Void Functions
What is a Void Function?
A void function is a type of function that does not return any value after its execution. It essentially executes a block of code and completes its task without handing back a result. This is particularly useful when a function's primary role is to perform an action rather than compute and return a value.
Syntax of a Void Function
The syntax for defining a void function is straightforward and can be summarized as follows:
void functionName() {
// function body
}
When you declare a function with `void`, you are informing the compiler that there will be no return value.
Example of a Void Function
Here’s a simple example to illustrate a void function in action:
#include <iostream>
void printHello() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
printHello();
return 0;
}
In this example, when `printHello()` is called within the `main()` function, "Hello, World!" is printed to the console. The function does not return any value, highlighting how void functions can effectively perform actions without expecting something in return.
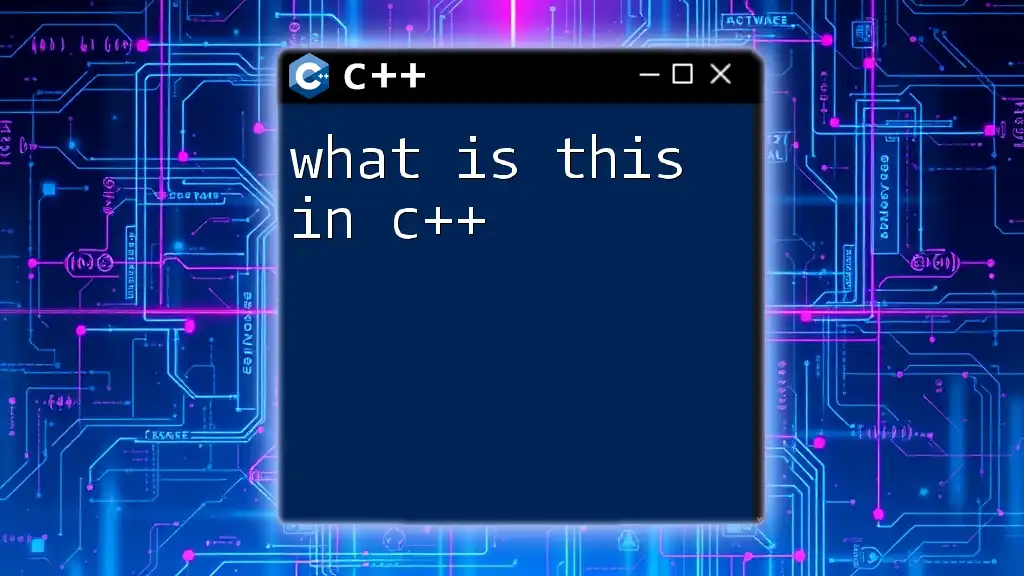
When to Use Void in C++
Situations for Choosing Void Functions
You will often choose to define a function as `void` in circumstances where a return value is not necessary. For example, if your function is modifying a global variable, updating user interface elements, or logging information, a void function is appropriate because the outcome does not need to be returned to the caller.
Benefits of Using Void Functions
Using void functions can lead to several benefits, including:
-
Simplicity: Void functions create clear and easy-to-understand procedures. When reading through the code, it is immediately clear that certain functions are designed solely for carrying out actions rather than returning values.
-
Readability: By indicating that no return value is expected, the intention behind your code becomes more straightforward, which is beneficial during team collaborations or when other developers revisit your code later.
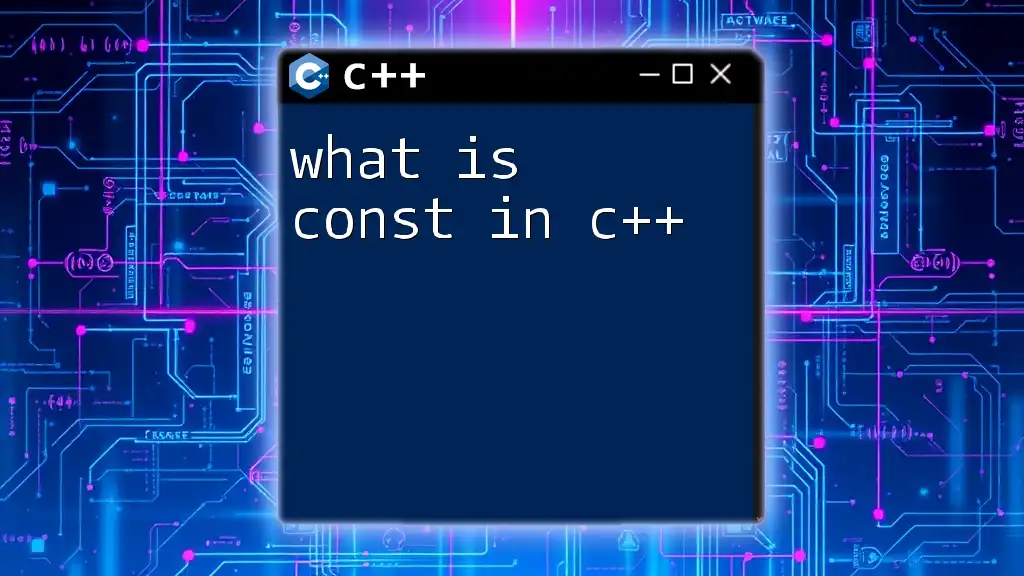
What It Does: Detailed Breakdown
How Void Affects Function Return Types
Using `void` as a return type carries specific implications for error handling and communications within your program. Since void functions cannot return a value, you must find alternative methods for indicating success or failure of operations. Common practices include utilizing global state variables, throwing exceptions, or utilizing output parameters (though output parameters can also blur the lines of function clarity).
Void as a Parameter Type
The `void` keyword can also be effectively applied as a parameter type. This is particularly valuable when you need to pass a pointer to an unspecified type. Here's a simple example:
void displayMessage(void* message) {
// function that takes a pointer to void
}
In the above code snippet, `displayMessage()` can accept a pointer to any type of data. However, keep in mind that additional typecasting will be needed within the function, which can lead to potential complications if not managed carefully.
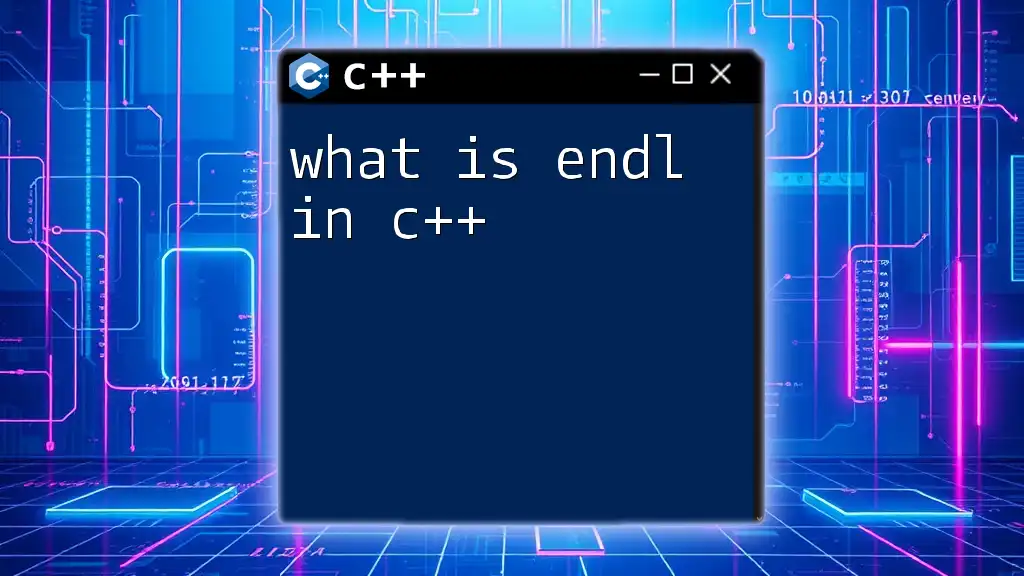
Common Mistakes with Void in C++
While `void` is an essential part of C++ programming, there are common pitfalls to avoid:
-
Forgetting to Call Void Functions: When developing a program, ensure you call your void functions; otherwise, their actions will not take place.
-
Misunderstanding That a Void Function Cannot Return a Value: It's a frequent misconception that one can accidentally return a value from a void function. Doing so will lead to compilation errors, as the function is designed explicitly to avoid returning any value.
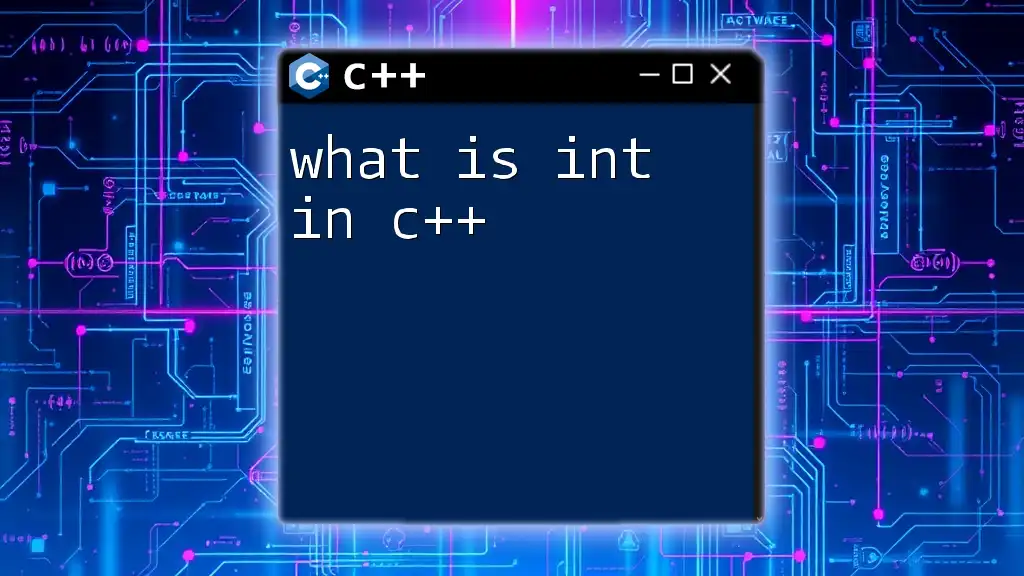
Conclusion
Understanding what is void in C++ is fundamental for any aspiring programmer. The `void` keyword plays a significant role in defining specific functions that perform tasks without expecting to return a value. By effectively utilizing void functions, you can enhance code clarity and maintainability, especially when dealing with actions that don't involve a return type.
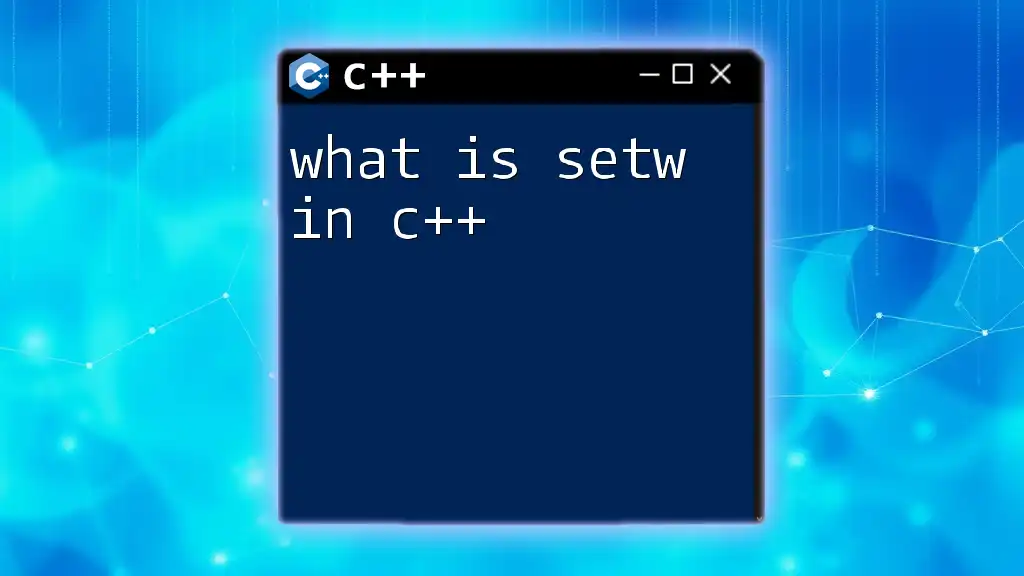
Call to Action
We encourage you to practice using void functions and experiment with different scenarios to solidify your understanding. If you have questions or want to share your experiences with using void in C++, feel free to leave a comment below! Additionally, check out the resources and exercises available through our company for a deeper dive into mastering C++ programming.
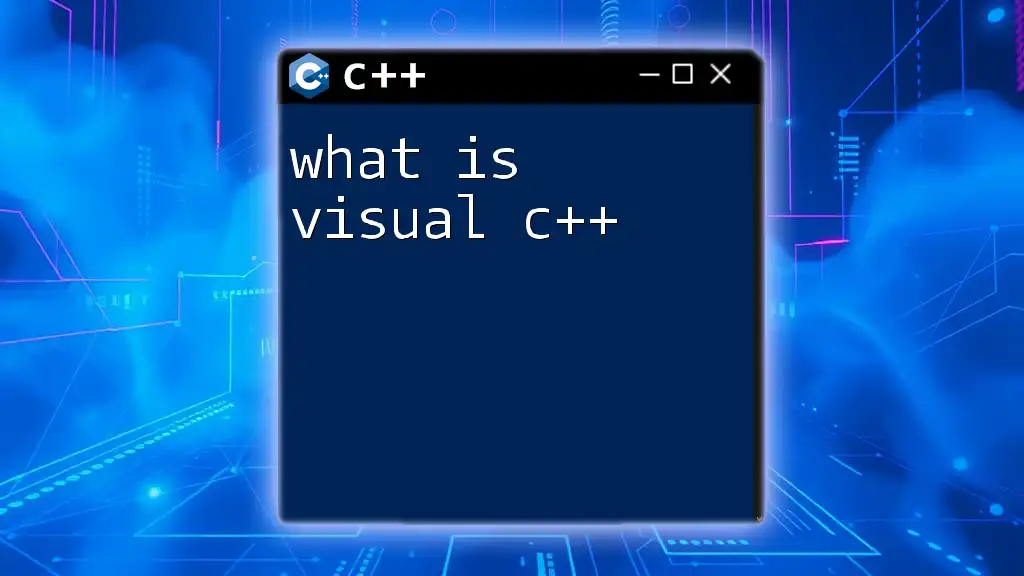
Additional Resources
For further exploration of related topics, consider reading more about C++ functions, data types, and coding best practices. Each topic will help enhance your programming skills and understanding of C++. Happy coding!