In C++, `size_t` is an unsigned integer type used to represent the size of objects in bytes and the result of the `sizeof` operator, ensuring that it can represent the maximum size of any object in memory.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
size_t size = numbers.size(); // Using size_t to store the size of the vector
std::cout << "Size of the vector: " << size << std::endl;
return 0;
}
What is size_t in C++?
`size_t` is an unsigned integer data type defined in the C++ standard library. It represents the size of objects in memory and is used widely in various operations involving containers, dynamic memory, and calculations that involve sizes. Being an unsigned type, it is especially suited for representing sizes since memory sizes cannot be negative.
This data type is crucial for ensuring efficient use and manipulation of memory in programs. Unlike other integer types such as `int` or `unsigned int`, `size_t` is specifically optimized for the platform's memory capabilities and is utilized in standard functions that handle memory-related tasks.
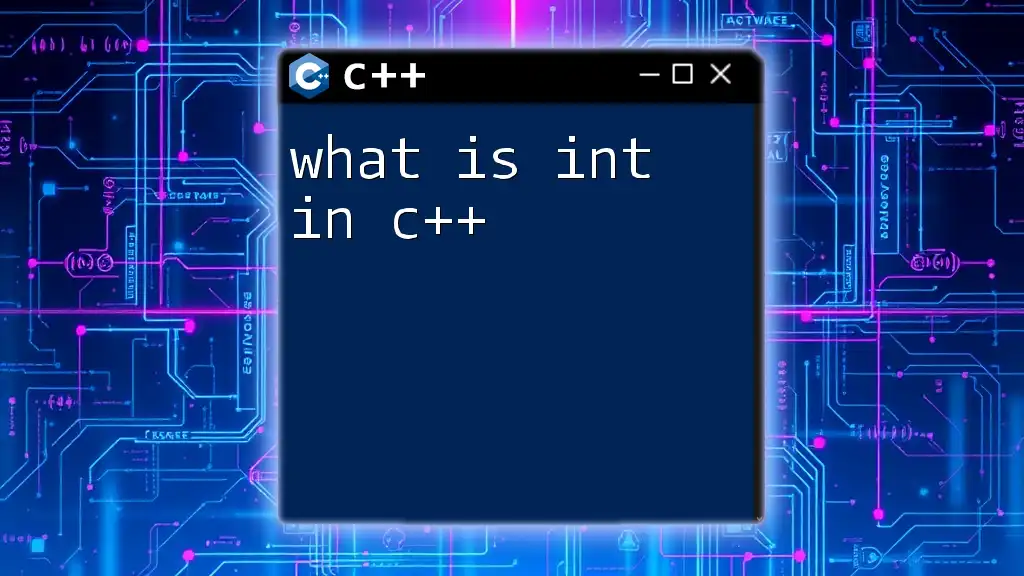
Characteristics of size_t
Data type characteristics
-
Unsigned nature: `size_t` is always an unsigned type, which guarantees that it can only represent non-negative values. This characteristic is especially vital since memory allocations and sizes cannot be negative.
-
Portability: The size of `size_t` can vary depending on the architecture of the system. On a typical 32-bit system, `size_t` is often 4 bytes, while on a 64-bit system, it can be 8 bytes. This flexibility allows the C++ standard library to efficiently accommodate different hardware capacities.
Range of values: The range of values that `size_t` can hold depends on its size. For instance, a 32-bit `size_t` can represent values from 0 to 4,294,967,295, while a 64-bit `size_t` extends this range significantly. It is essential to consider the system's architecture when performing optimizations or memory-related tasks.
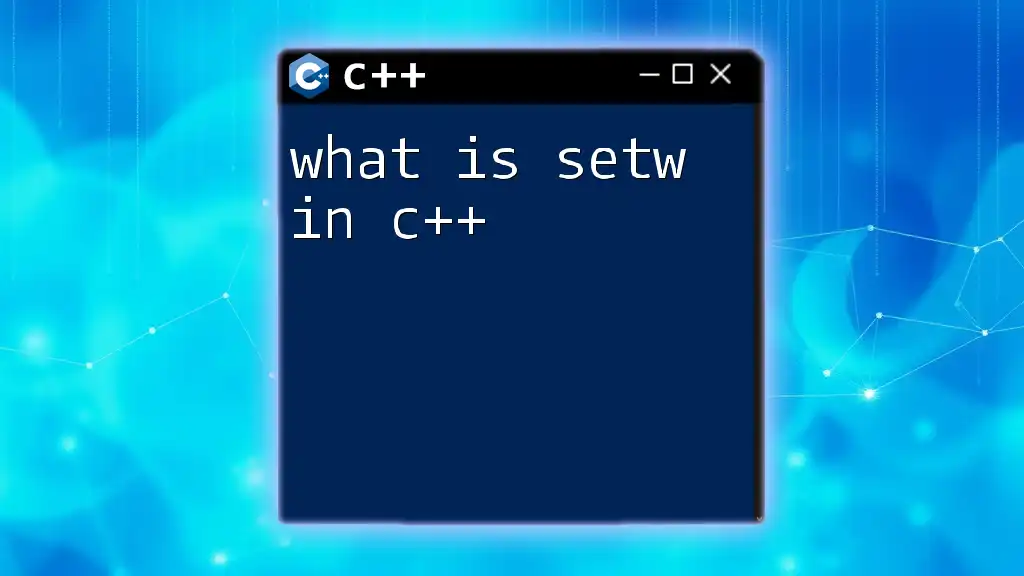
When to Use size_t
Memory Allocation
One of the primary use cases of `size_t` is in dynamic memory allocation. When utilizing functions like `malloc()` or `new`, programmers specify the number of bytes to allocate. Since memory sizes are always non-negative, using `size_t` reinforces this guarantee.
Example of memory allocation:
size_t num_elements = 100;
int* array = new int[num_elements];
In this snippet, `num_elements` is declared as `size_t`, which safely allocates memory for 100 integers, ensuring a positive count.
Array Indexing
When working with arrays, `size_t` is often the preferred type for indexing. Its unsigned nature avoids the risk of negative indices, which can lead to undefined behavior and difficult-to-track bugs.
Example code snippet:
for (size_t i = 0; i < num_elements; ++i) {
array[i] = i * 2;
}
In this loop, using `size_t` helps ensure that the index remains valid throughout the process, reinforcing robust code practices.
Looping Constructs
`size_t` is advantageous in loop conditions. By employing this data type, you reduce the risk of encountering signed/unsigned comparison issues, which are common pitfalls in C++ programming.
Example code:
for (size_t i = 0; i < array_size; ++i) {
std::cout << array[i] << std::endl;
}
By using `size_t`, you ensure that the indexing variable is appropriate for representing array sizes, making the loop more error-resistant.
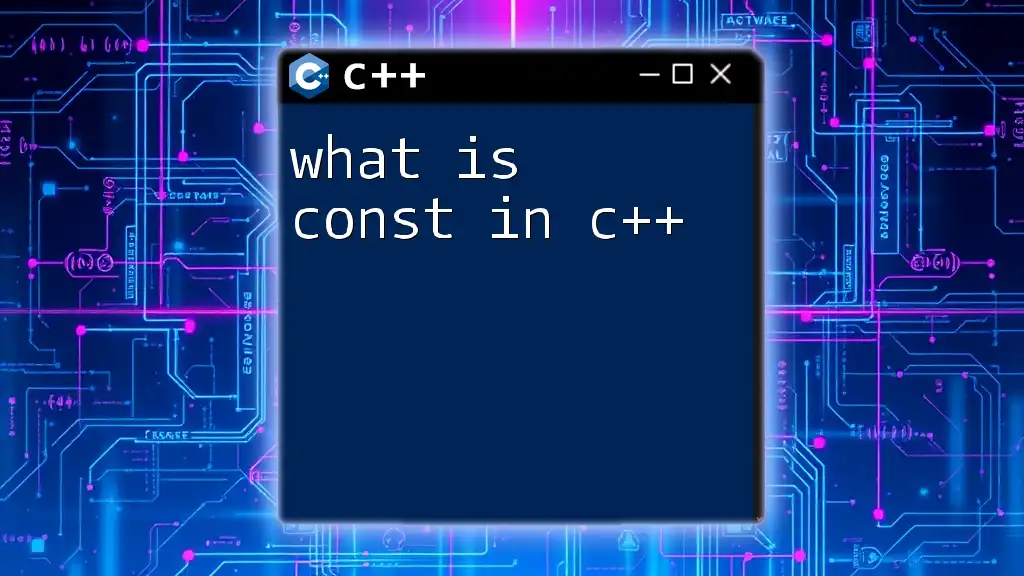
How size_t Works in Standard Library
Standard Template Library (STL)
The STL utilizes `size_t` extensively to manage container sizes and capacities. Containers like `vector`, `list`, and `map` use `size_t` to enable operations like resizing and accessing elements, reflecting the data type's importance in standard programming practices.
For instance, consider the following code snippet demonstrating the use of `size_t` in a vector:
std::vector<int> numbers(10);
for (size_t i = 0; i < numbers.size(); ++i) {
numbers[i] = i * i;
}
In this example, `numbers.size()` returns a `size_t` value indicating the number of elements in the vector, accurately reflecting the operations performed on it without risk of negativity.
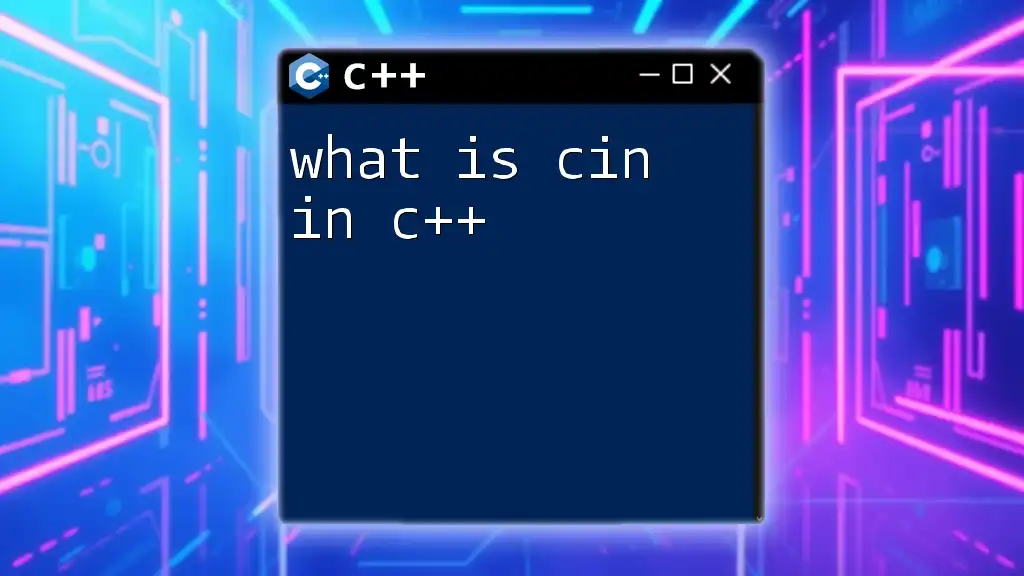
Limitations of size_t
Negative Values
While `size_t` excels in defining sizes, its unsigned trait can lead to unintended consequences when dealing with calculations. For example, subtracting a larger `size_t` from a smaller one results in an underflow, wrapping around to a very high positive number. This could introduce hard-to-detect bugs in code.
Platform Dependence
The variability of `size_t` across different system architectures presents challenges. Assumptions about the size of `size_t`—such as believing it to always be 32 bits—can lead to vulnerabilities and issues in cross-platform applications. Developers should always confirm the size of `size_t` on their target architecture to avoid problems during runtime.
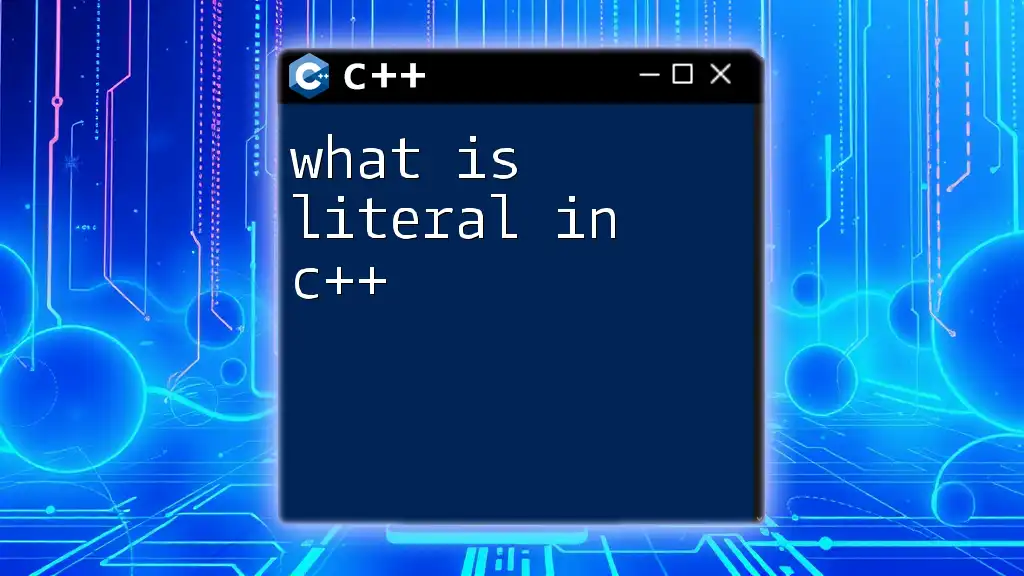
Common Mistakes with size_t
Using size_t with signed integers
A common mistake is using `size_t` in operations with signed integers. Mixing signed and unsigned types can lead to unexpected behavior and logic issues due to the way C++ handles these comparisons.
Example of a mistake:
int index = -1;
if (index < array_size) { // comparing size_t with signed int
// logic
}
Such a comparison can be misleading; the signed negative value is implicitly converted to a large positive `size_t`, resulting in unintended execution path scenarios.
Assuming size_t is always 32 bits
Developers should avoid the assumption that `size_t` is consistently 32 bits. This misconception can lead to problems, especially when writing code that operates on different hardware or when developing libraries that could be compiled on various systems.
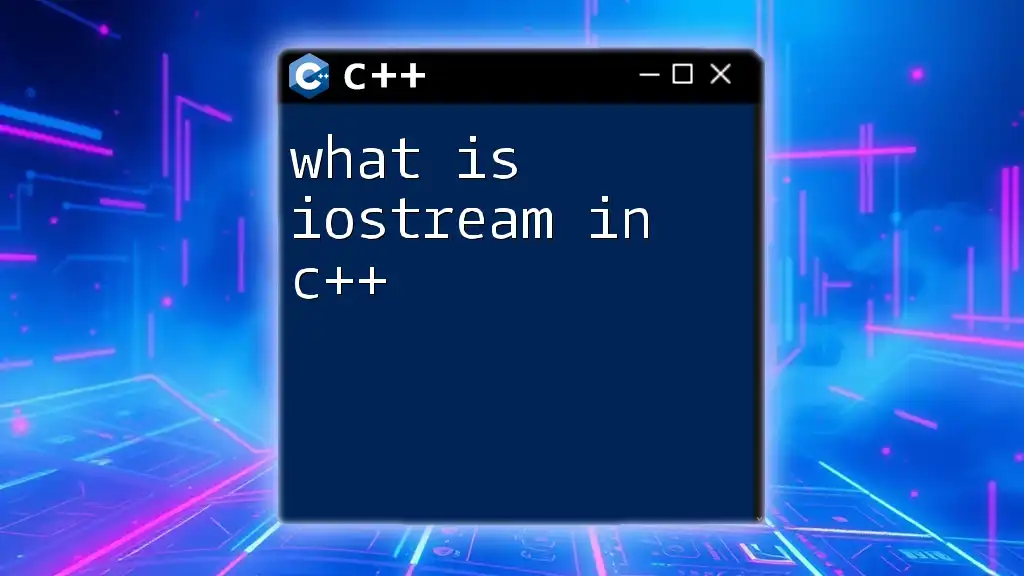
Conclusion
Understanding what is size_t in C++ sheds light on a crucial part of memory management and data handling in the language. Utilizing `size_t` while adhering to best practices can lead to more robust, portable, and error-free code.
By leveraging this data type properly, developers can enhance the performance and reliability of their applications—making the use of `size_t` a fundamental aspect of C++ programming that should not be overlooked.
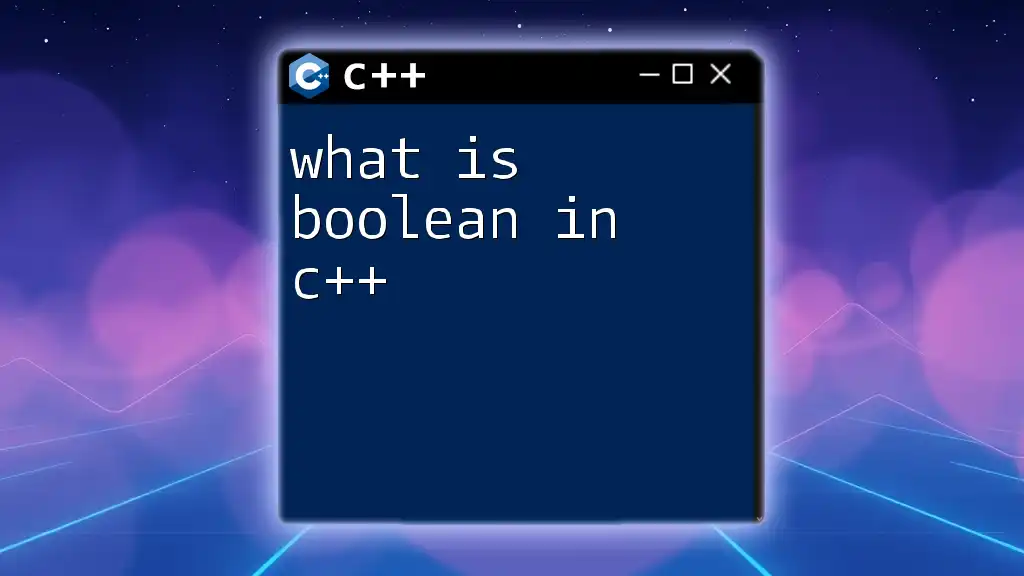
Call to Action
Continue your learning journey by exploring further resources or taking courses on C++ programming to gain deeper insights into data types and memory management in C++. Access additional articles and tutorials related to C++ data types for more enriching knowledge.
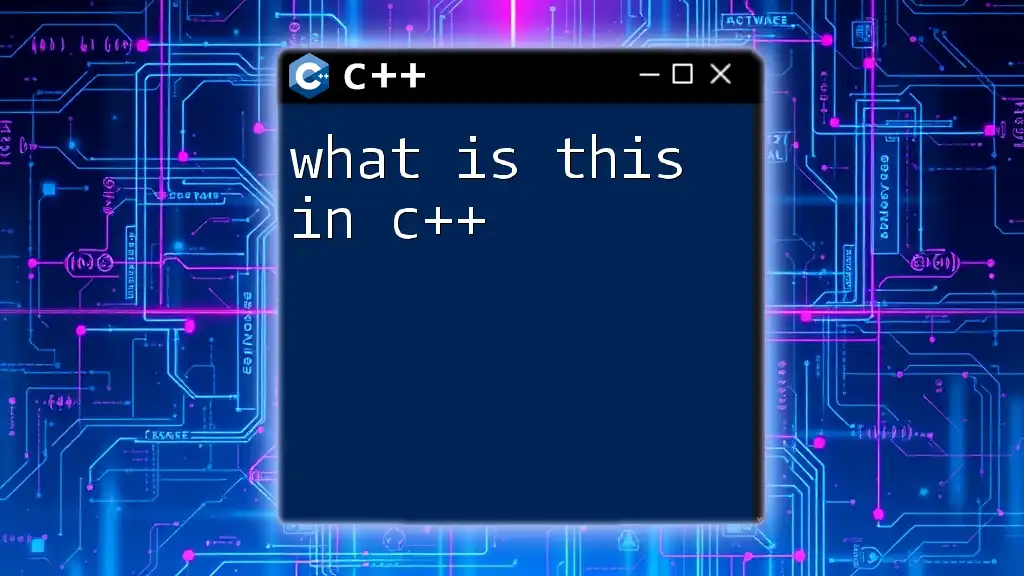
References
For further reading, consider checking reputable programming books, the C++ standard documentation, and trusted online tutorials focusing on C++ data types and memory management.