In C++, `endl` is used to insert a new line in the output stream and flush the output buffer, ensuring that all output is displayed immediately.
Here’s a code snippet demonstrating its use:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Does `endl` Do in C++
Definition of `endl`
In C++, `endl` is a special stream manipulator used primarily with the output stream object `cout`. It stands for "end line," and its primary role is to insert a new line in the output stream, which helps to format text more readably. When `endl` is used, it not only generates a newline in the console output but also flushes the output buffer, ensuring that all output is displayed immediately.
How `endl` Functions
To understand how `endl` operates, we first need to delve into the concept of output buffering. When you use output streams in C++, data is collected or buffered before being sent to the console. When you employ `endl`, it triggers two actions:
- Flushing the output buffer: This means that all the data waiting to be outputted is sent to the console immediately. This ensures that users see the output at that exact moment, which can be crucial in situations where timing matters.
- Moving the cursor to a new line: This adds a line break, which helps in organizing output neatly.
The difference between using `endl` and simply inserting a newline character (`\n`) lies mainly in this flushing aspect. While `\n` moves to a new line, it does not flush the output buffer by default, which means it may delay output display until the buffer is full or the program concludes.
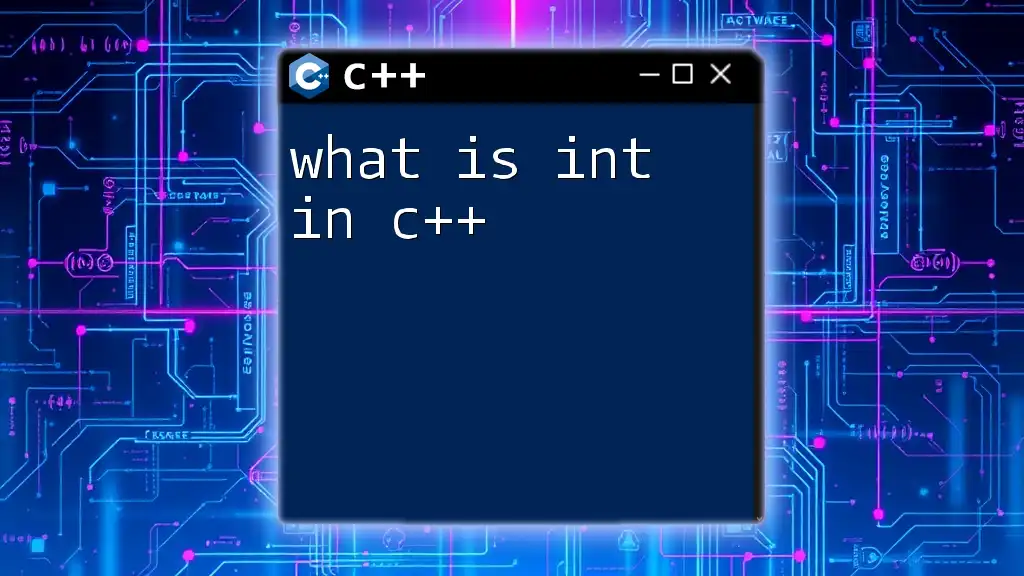
The Purpose of Using `endl`
Improving Readability of Output
Using `endl` significantly enhances the clarity and readability of the output generated by a program. Proper formatting improves user experience, especially in console applications where textual outputs are dense. Using `endl` can visually separate different outputs, making it easier to distinguish between various sections or logs in your program.
For example:
#include <iostream>
using namespace std;
int main() {
cout << "Step 1 complete" << endl;
cout << "Step 2 initiated" << endl;
cout << "Step 2 complete" << endl;
return 0;
}
In this snippet, `endl` causes a new line after each message, creating a well-formatted output that is easy to follow.
Managing Output Buffering
Output buffering plays a vital role in how inputs and outputs are processed in a C++ program. When you print messages to the console or a file, they often don’t appear instantly. The output is stored in a buffer first and sent when the buffer fills up or the program comes to an end. Using `endl` is an effective way of managing this behavior because it forces the output to flush every time it is called.
Consider this example:
#include <iostream>
#include <unistd.h>
using namespace std;
int main() {
cout << "Processing..." << flush; // Flushes without newline
sleep(2); // Simulate processing
cout << "Done!" << endl; // Flushing + newline
return 0;
}
In this code, the `flush` function ensures "Processing..." is shown immediately, while `endl` confirms "Done!" appears after a two-second delay.
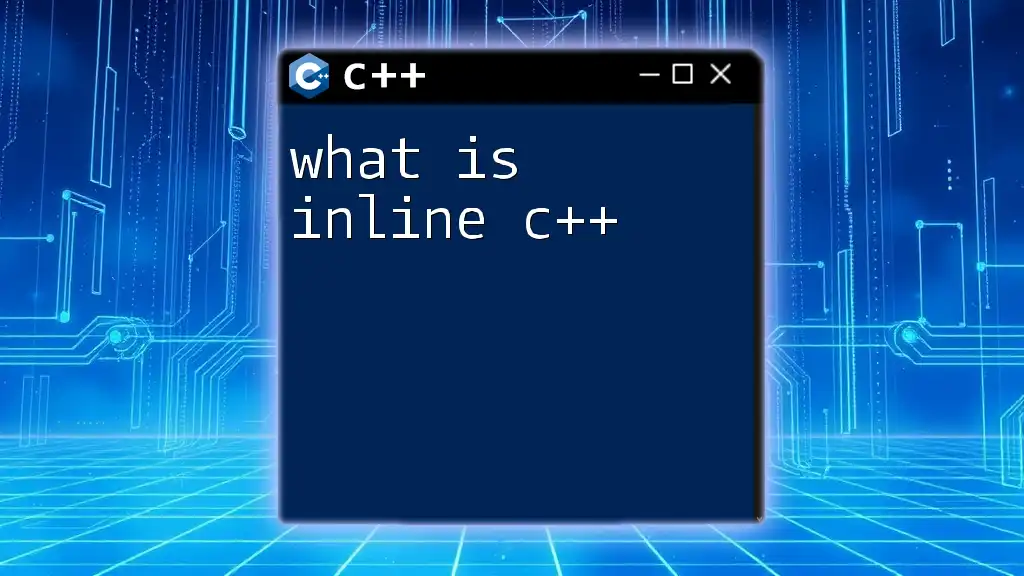
Practical Examples of `endl`
Basic Example
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this straightforward example, "Hello, World!" is printed to the console and followed by a new line due to `endl`. The program execution ends seamlessly, with the output appearing at once.
Advanced Use Case with Multiple `endl`
#include <iostream>
using namespace std;
int main() {
cout << "First line" << endl << "Second line" << endl;
return 0;
}
This snippet demonstrates that `endl` can be chained together across multiple outputs. Each `endl` call triggers both a new line and a buffer flush, enhancing clarity in the displayed output.
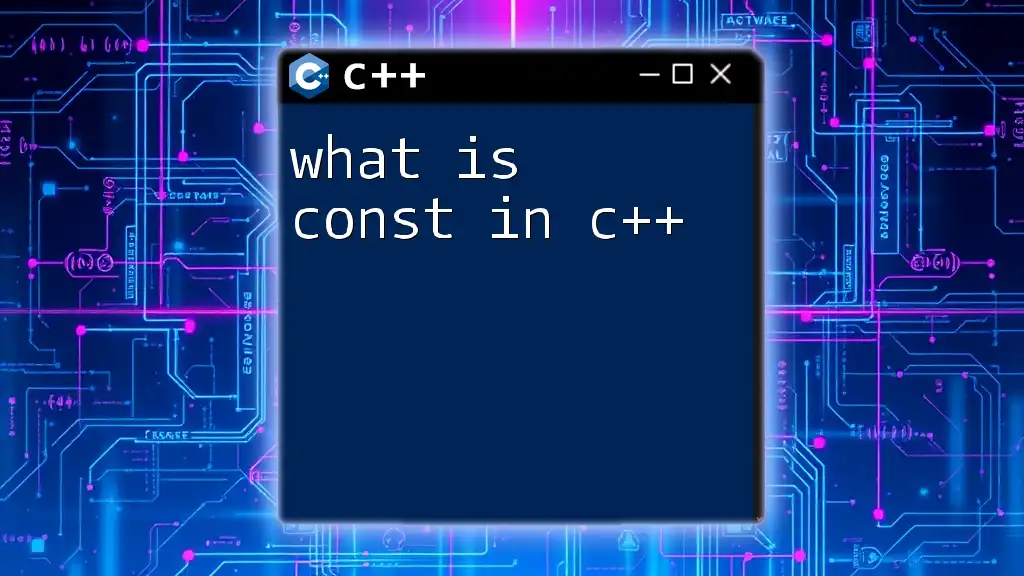
Alternatives to `endl`
Using `\n`
While `endl` has its advantages, there are situations where using `\n` is more appropriate. The newline character can be more performance-friendly in high-frequency output situations where flushing is less desirable:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!\n"; // Just a newline, no flush
return 0;
}
In scenarios where performance is critical, especially inside loops, opting for `\n` can yield better results, as it avoids unnecessary flushing, maintaining faster execution.
Other Output Manipulators
C++ offers several other manipulators; some examples include:
- `std::flush`: Flushes the output buffer but does not add a new line.
- `std::setw()`: Sets the width of the next output field. Choosing the right manipulator based on your needs can optimize code readability and performance.
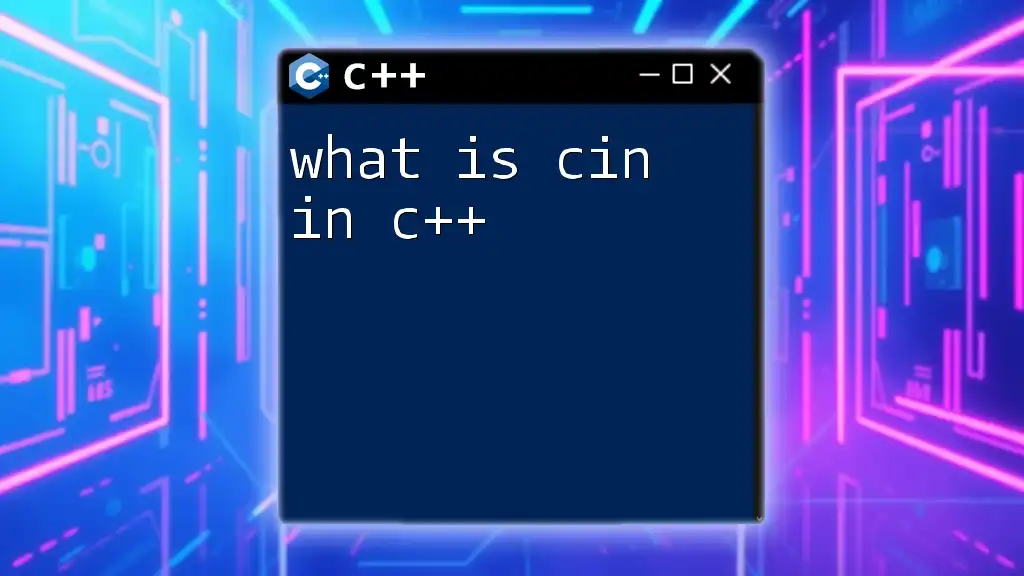
Common Mistakes with `endl`
Overusing `endl`
A common error is overusing `endl` in places where a simple newline would suffice. Excessive use can lead to performance drawbacks, primarily due to the unwanted frequent flushing of the output buffer. Maintain awareness of when it is genuinely necessary to flush the output.
Misunderstanding Buffering Behavior
Many beginners misconstrue how output buffering works. Properly understanding when the buffer flushes allows programmers to control the timing of their output more effectively, enhancing debugging efforts and program flow.
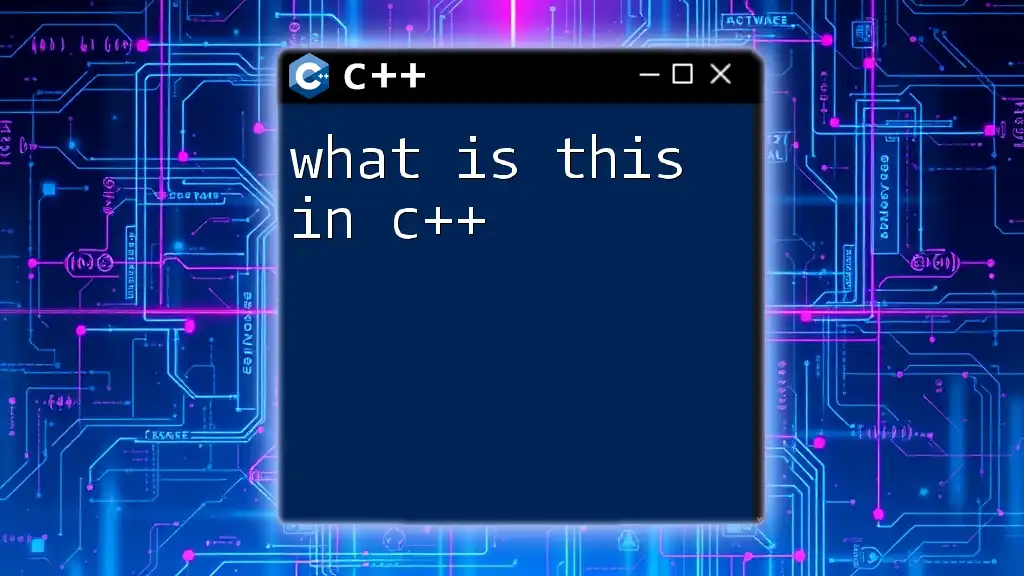
Best Practices for Using `endl`
When to Use `endl`
Use `endl` primarily in cases where immediate visibility of output is needed or when moving to a new line enhances readability significantly. It is particularly useful in situations where console logs are likely to be followed closely by user interaction or further processing steps.
Alternatives for More Control
If you're working in a performance-sensitive environment or inside loops, consider alternative approaches such as using `std::flush`, which provides more control over the output flow without inserting an additional line break.
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 3; i++) {
cout << "Count: " << i << flush; // Just flushing, no newline
}
cout << endl; // End line after the loop
return 0;
}
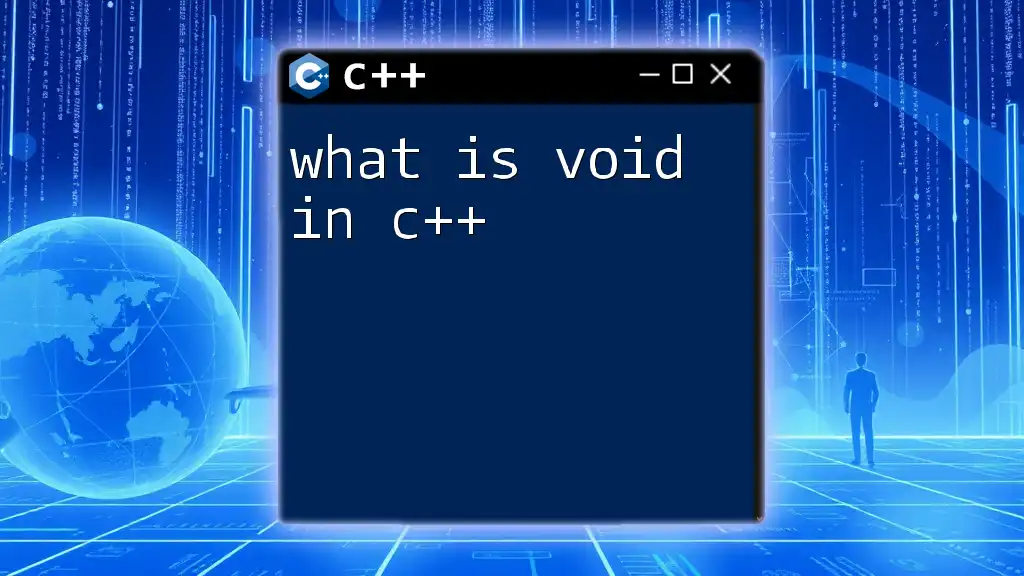
Conclusion
Understanding what is `endl` in C++ is essential for effective output management. By learning its functionality, benefits, and appropriate usage, you can enhance both the readability and performance of your console applications. Mastering output operations is a crucial aspect of becoming proficient in C++, and practices like adjusting your use of output manipulators will play a vital role in your programming journey.
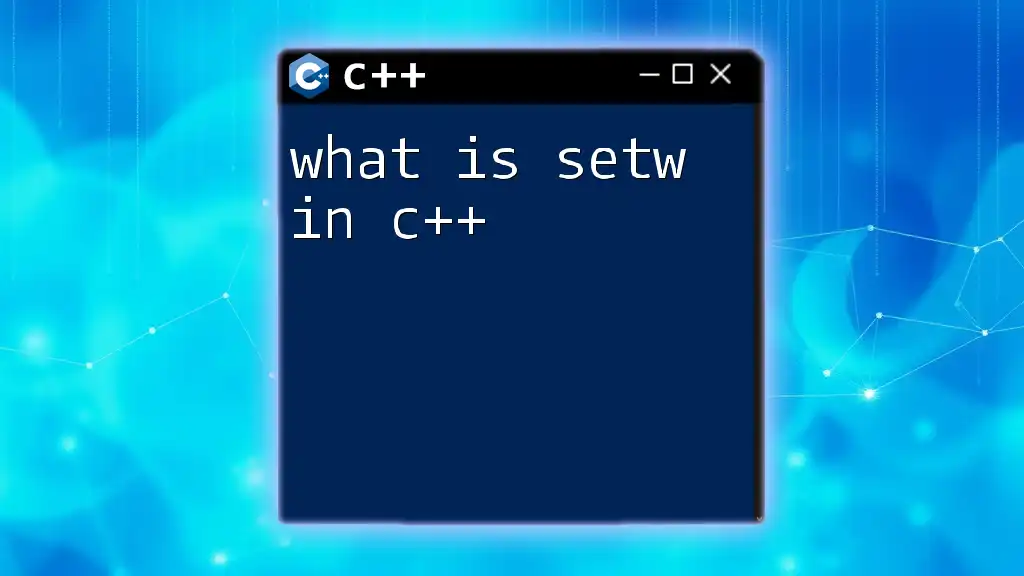
Additional Resources
For further learning, explore official C++ documentation, online courses, and community forums where you can elevate your knowledge and practical skills regarding C++ input and output operations.