In C++, `endl` is used to insert a new line into the output stream and flush the output buffer, ensuring that all output is displayed immediately.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
std::cout << "This is a new line." << std::endl;
return 0;
}
Understanding endl in C++
What is endl in C++?
`endl` is a manipulator in C++ that is used with output streams, particularly with `std::cout`. It serves two primary functions: it inserts a newline character into the output stream and flushes the stream's buffer. This means that it not only moves the cursor to the next line for subsequent outputs but also ensures that all previously outputted data is written to the device (such as the console).
Historically, `endl` has been part of C++ since its inception, providing a straightforward method for formatting output. It is essential to understand its utility, especially for those new to programming in C++.
Why Use endl?
Using `endl` is beneficial for several reasons. First, it enhances the readability of the output by clearly separating lines of output, making logs and user interfaces more user-friendly. Additionally, flushing the output buffer is critical in situations where you need to ensure that all information has been displayed before a significant event occurs, such as an error message or a prompt waiting for user input.
It’s essential to note the difference between `endl` and the newline escape character `\n`. While both can create a new line in the output, `endl` flushes the buffer, which can have performance implications in tight loops or intensive output scenarios.
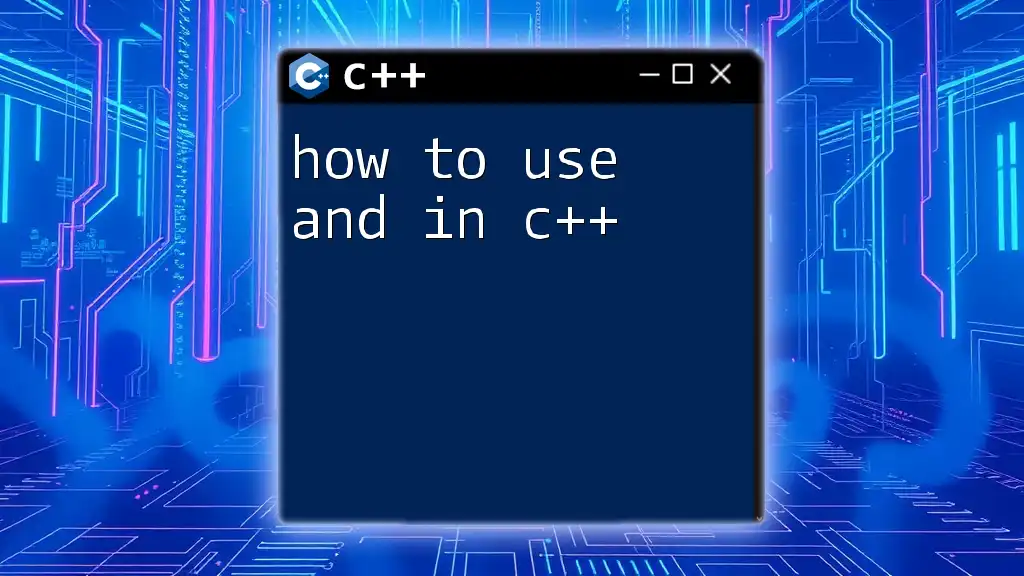
How to Use endl in C++
Basic Syntax of endl
The syntax for using `endl` is straightforward. You incorporate it into an output stream to move to the next line and flush the output buffer:
std::cout << "Hello, World!" << std::endl;
In this line, `std::cout` is the output stream, "Hello, World!" is the string being printed, and `std::endl` signifies the end of the line.
Examples of Using endl
Example 1: Simple Output
Here’s a basic program that prints two lines using `endl`:
#include <iostream>
int main() {
std::cout << "Line 1" << std::endl;
std::cout << "Line 2" << std::endl;
return 0;
}
In this example, the output will be:
Line 1
Line 2
Each call to `std::cout` followed by `std::endl` ensures that the lines are separated for clarity.
Example 2: Combining with Other Outputs
You can also combine `endl` with other output commands seamlessly. Consider the following example:
#include <iostream>
int main() {
int a = 5, b = 10;
std::cout << "The sum of " << a << " and " << b << " is " << (a + b) << std::endl;
}
The program outputs:
The sum of 5 and 10 is 15
Here, `endl` is used again to consolidate multiple outputs into a cohesive message, making it readable.
Example 3: Looping with endl
Using `endl` in loops showcases its utility in formatting repeated output:
#include <iostream>
int main() {
for(int i = 1; i <= 5; i++) {
std::cout << "This is line " << i << std::endl;
}
}
The output will be:
This is line 1
This is line 2
This is line 3
This is line 4
This is line 5
This structure aids in keeping each line distinct, enhancing the overall readability, especially when dealing with a larger set of outputs.
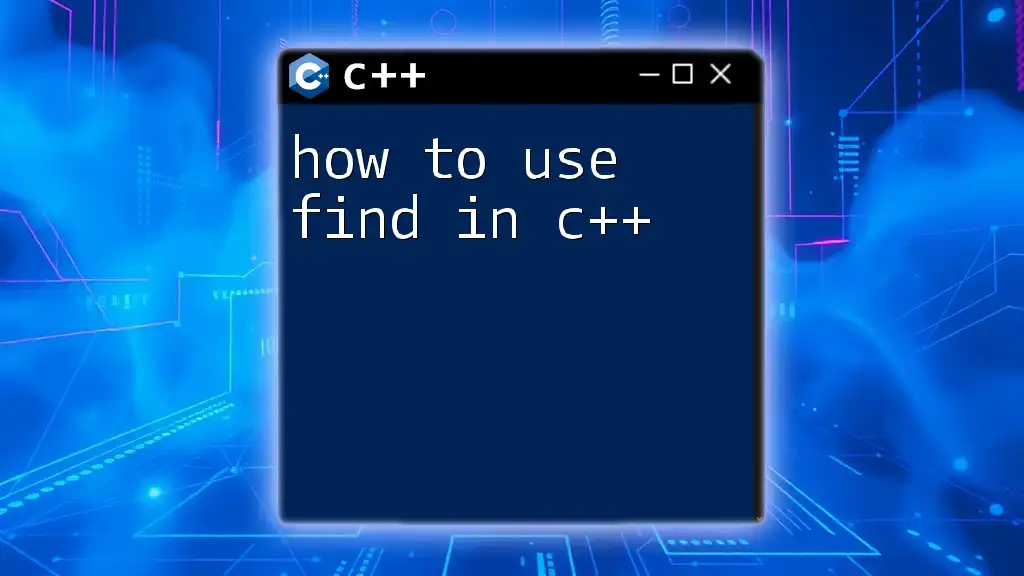
Performance Considerations
When to Use endl vs. \n
Although `endl` is handy, it is essential to recognize when to use it versus the newline character `\n`. The key difference lies in performance. Since `endl` flushes the buffer, it may introduce unnecessary delays in scenarios where frequent output occurs, such as in loops that run many times. Conversely, using `\n` does not flush the buffer, making it a faster option in scenarios where flushing is not required for the output to function correctly:
std::cout << "Line with a newline character\n"; // Does not flush the buffer
Best Practices
A recommended best practice is to use `endl` when:
- You need to ensure that output appears immediately, such as before waiting for user input.
- Logging error messages where immediate feedback is necessary.
However, consider using `\n` for regular output within performance-critical sections of your code. This approach allows you to maintain efficiency while still achieving a clear format.
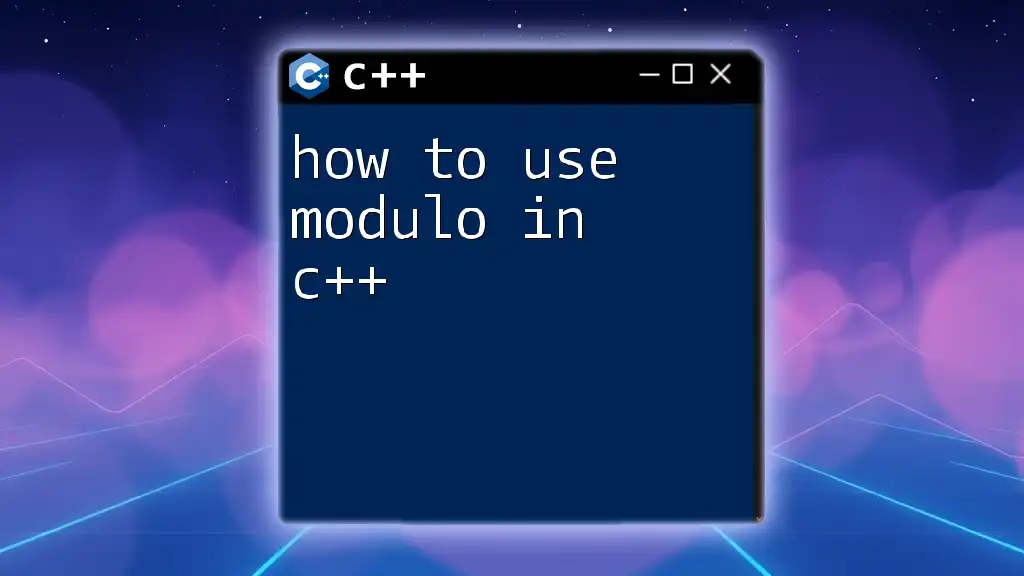
Troubleshooting Common Issues
Common Problems with endl
One common issue that beginners may encounter is the unexpected behavior of output when `endl` is used within certain contexts. For example, using `endl` excessively in tight loops can slow down the program dramatically due to frequent flushing.
Solution Tips
To mitigate performance issues, consider these strategies:
- Limit the use of `endl` in high-frequency output scenarios.
- Utilize `\n` where appropriate, reserving `endl` for situations demanding an immediate write to the output device.
- Gather multiple output statements together and call `std::endl` only once at the end to flush the output more efficiently.
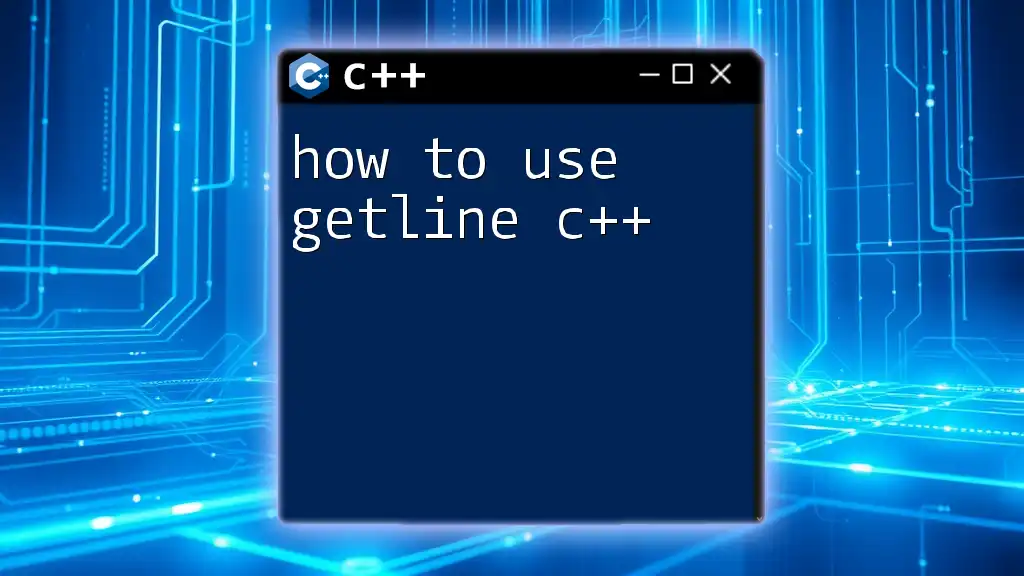
Conclusion
Understanding how to use `endl` in C++ effectively is crucial for anyone looking to improve their C++ programming skills. The considerations of readability, output buffering, and performance are all essential components that can enhance your coding practice.
Remember to practice implementing `endl` in various scenarios to solidify your understanding. This hands-on experience, along with awareness of when to use alternative methods like `\n`, will ultimately make you a more proficient C++ programmer.
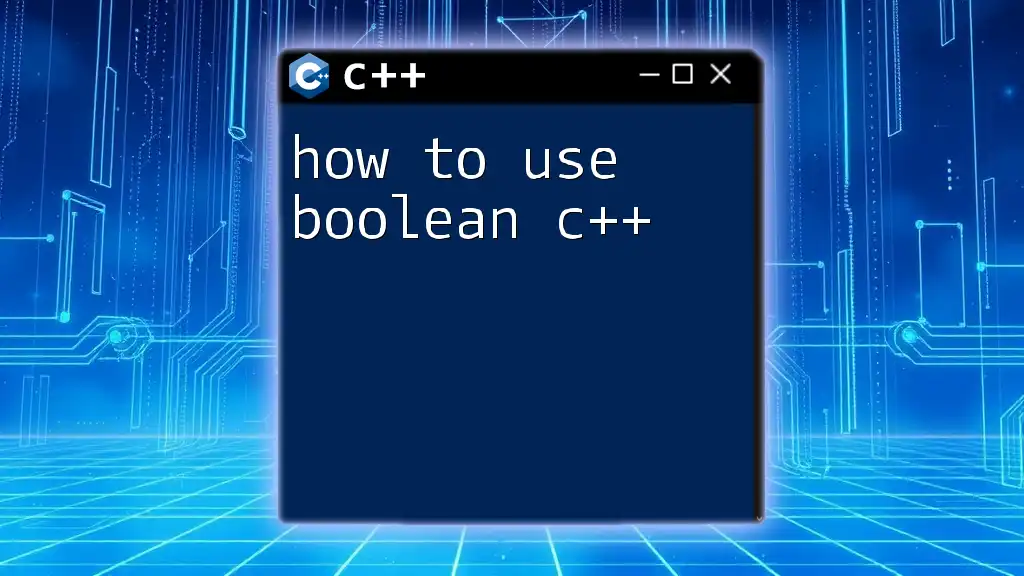
Additional Resources
For more insight into C++ output streams and to further expand your knowledge, look for resources that delve into advanced aspects of C++ programming, including output formatting and stream manipulators.
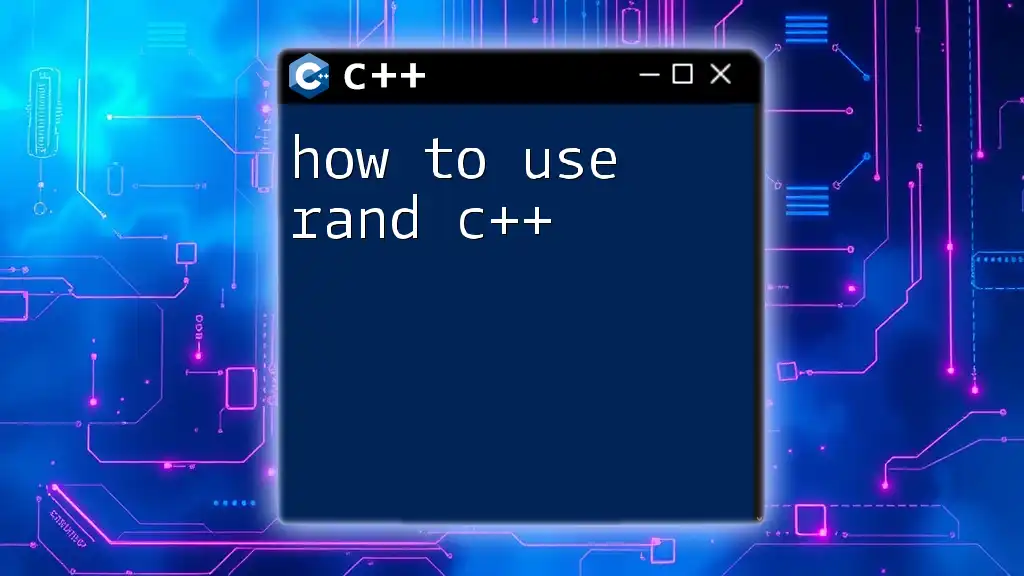
Call to Action
Now it's your turn! Try incorporating `endl` into your own C++ projects and experiment with different outputs. Share your experiences or ask your questions in the comments section below!