In C++, you can end a line by using the `std::endl` manipulator or the newline character `\n` in your output stream. Here's how you can do it:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Using std::endl
std::cout << "Hello, again!" << '\n'; // Using newline character
return 0;
}
Understanding Output in C++
What is Output in C++?
In C++, output refers to any information that a program sends to the console or another output device for the user's observation. The primary means of producing output in C++ is through output streams, which provide a way to display data to users effectively. Output is vital in programming as it allows users to get feedback from their commands, see results of operations, or diagnose problems when they arise.
Output Streams in C++
The standard output stream in C++ is represented by `std::cout`, which is included in the `<iostream>` header file. This stream allows you to send formatted data to the console, making it essential for both debugging and user interaction.
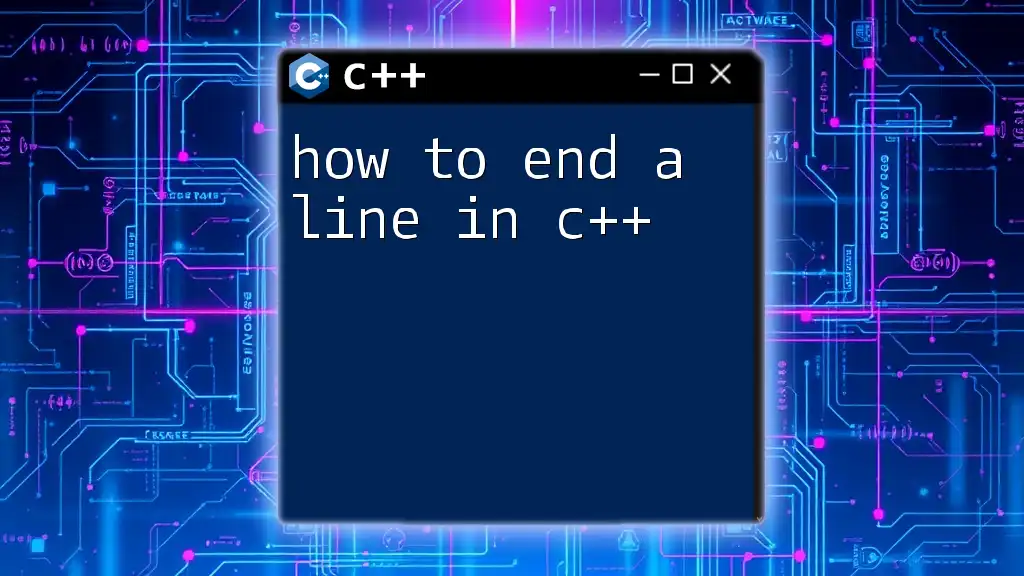
Ways to End a Line in C++
Using the Newline Character
What is the Newline Character?
The newline character is a special character in programming, represented as `\n`. When used in a string, this character will cause the output to move to the next line in the console. It is a straightforward way to achieve line breaks.
Code Example
#include <iostream>
int main() {
std::cout << "Hello, World!\n";
return 0;
}
In this example, the output displays "Hello, World!" followed by a line break. The use of `\n` ensures that any subsequent output starts on a new line.
Using std::endl
What is std::endl?
`std::endl` is a manipulator that not only inserts a newline character into the output but also flushes the output buffer. Flushing ensures that all data is written to the console immediately, which can be crucial in time-sensitive applications where you want users to see what is happening without delay.
Code Example
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this case, using `std::endl` also provides a line break, but it does more by flushing the output stream right after the message is printed. This behavior can be particularly useful when multiple output statements are close in timing.
Performance Considerations
When to Use Which Method?
When deciding between `\n` and `std::endl`, it’s important to consider performance implications. The use of `std::endl` can slow down your program because of the flushing of the output buffer, which is unnecessary in many cases. If immediate output isn't critical, using `\n` is the more efficient choice.
Code Example: Performance Testing
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < 100000; i++) {
std::cout << "Line " << i << "\n";
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "With \\n: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << " ms" << std::endl;
start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < 100000; i++) {
std::cout << "Line " << i << std::endl;
}
end = std::chrono::high_resolution_clock::now();
std::cout << "With std::endl: " << std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count() << " ms" << std::endl;
return 0;
}
This code snippet demonstrates the time taken to output 100,000 lines using both `\n` and `std::endl`. Running the program shows clear performance differences, reinforcing the recommendation to use `\n` when immediate output isn’t necessary.
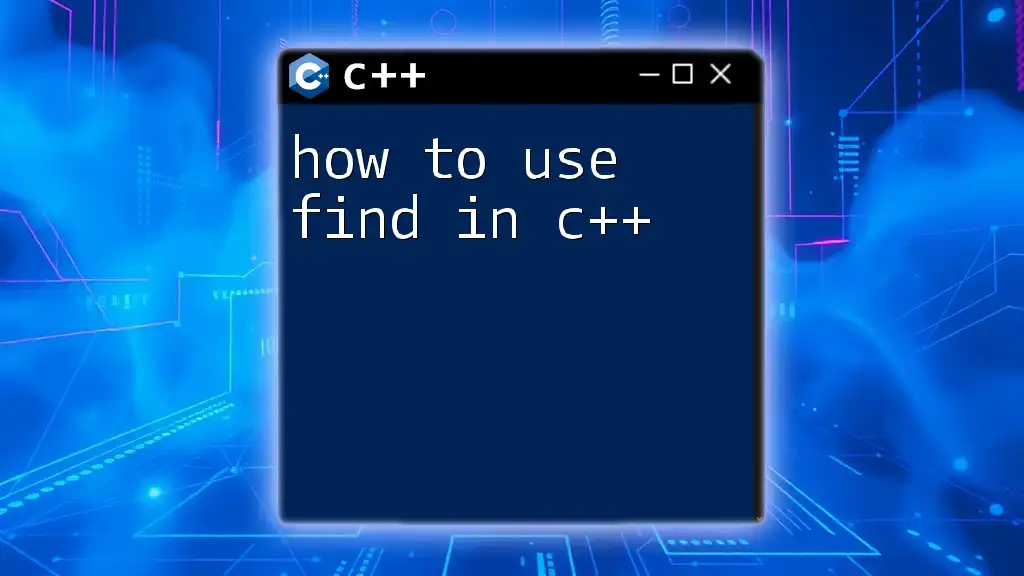
Common Errors and Troubleshooting
What to Avoid
When learning how to end line in C++, programmers often face some common pitfalls:
- Forgetting to include the `<iostream>` header file.
- Misplacing the newline character or `std::endl`, leading to unintended formatting in the output.
- Overusing `std::endl`, which may slow down the program unnecessarily.
Error Examples
An incorrect syntax, such as `std::cout << "Hello World!" std::endl;`, will lead to compilation errors. It’s essential to ensure that all function calls and operators are used correctly to avoid such issues.
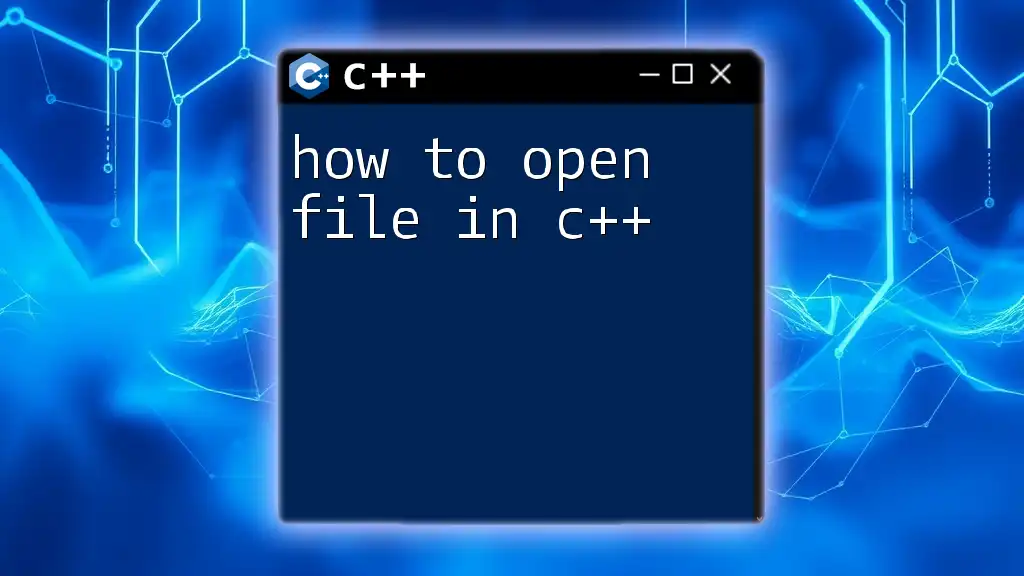
Best Practices for Formatted Output
Consistency is Key
For cleaner, more maintainable code, it’s important to maintain consistency in your formatting. Using the same method for ending lines throughout your program not only aids readability but also prevents confusion concerning the output.
Readability Matters
Clear and consistent ending of lines enhances the readability of the output, providing users with an easier way to interpret results. Strive for a structured output format that directly conveys what you want to communicate.
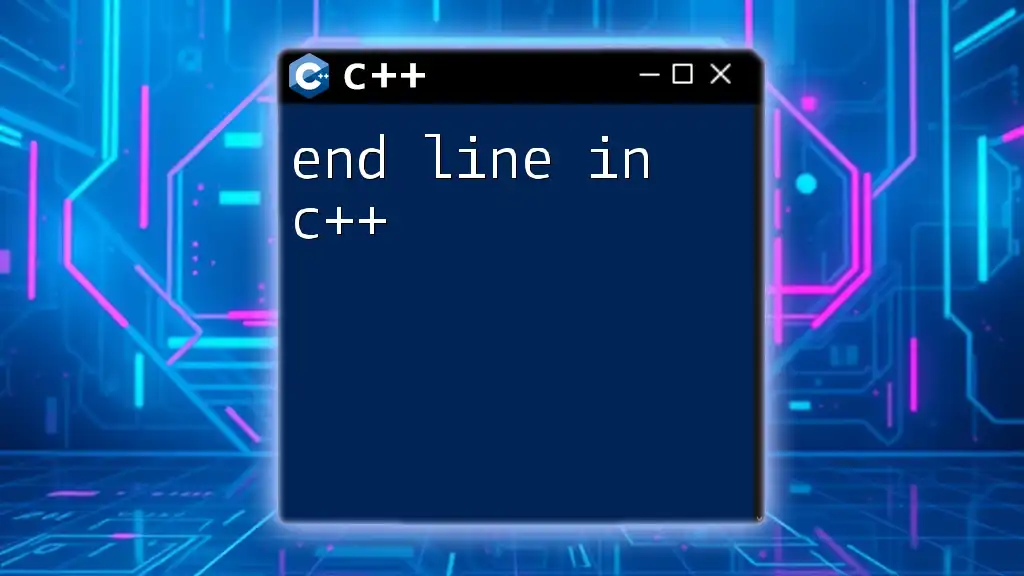
Conclusion
Understanding how to end line in C++ is fundamental in crafting user-friendly applications. Whether you choose the newline character or `std::endl`, knowing the mechanics and implications behind each choice can vastly improve both your programming skills and the experience of your users.
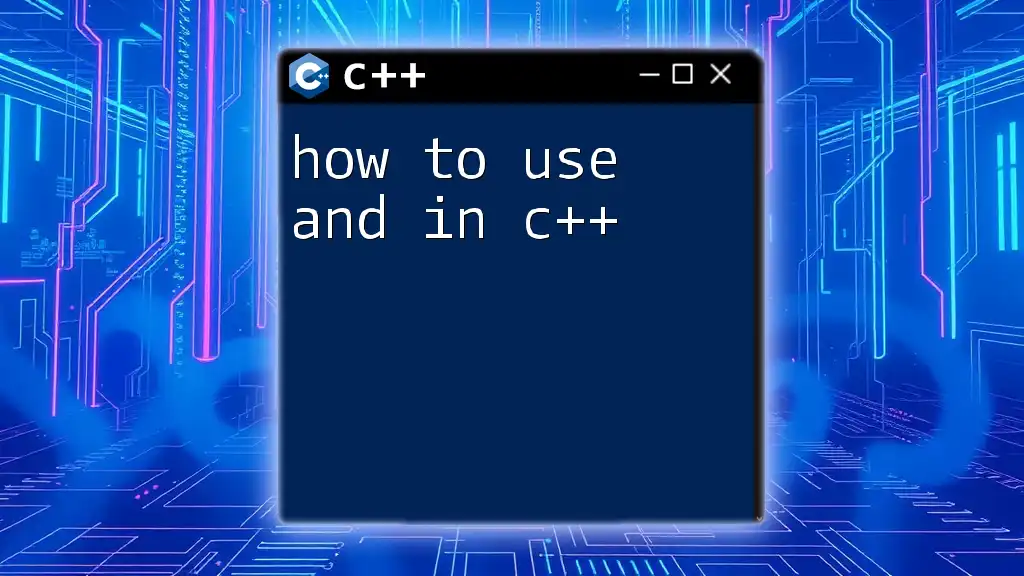
Call to Action
Take the examples discussed here and experiment in your own C++ environment. Explore further advanced concepts in output manipulation to enhance your proficiency!
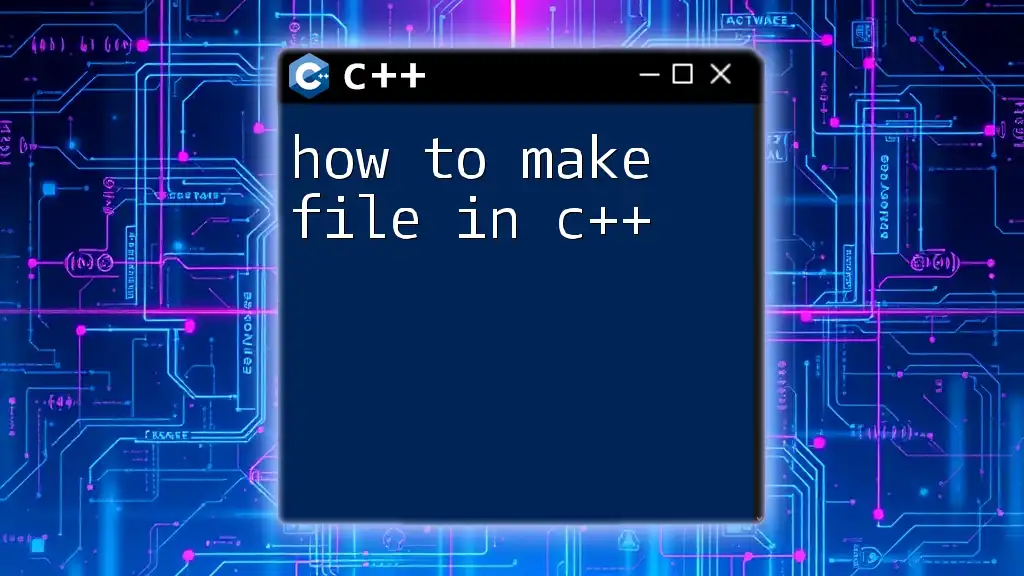
Additional Resources
For more information on output streams and formatting in C++, consider visiting the official C++ documentation and reliable coding resources that delve deeper into the nuances of C++ programming.
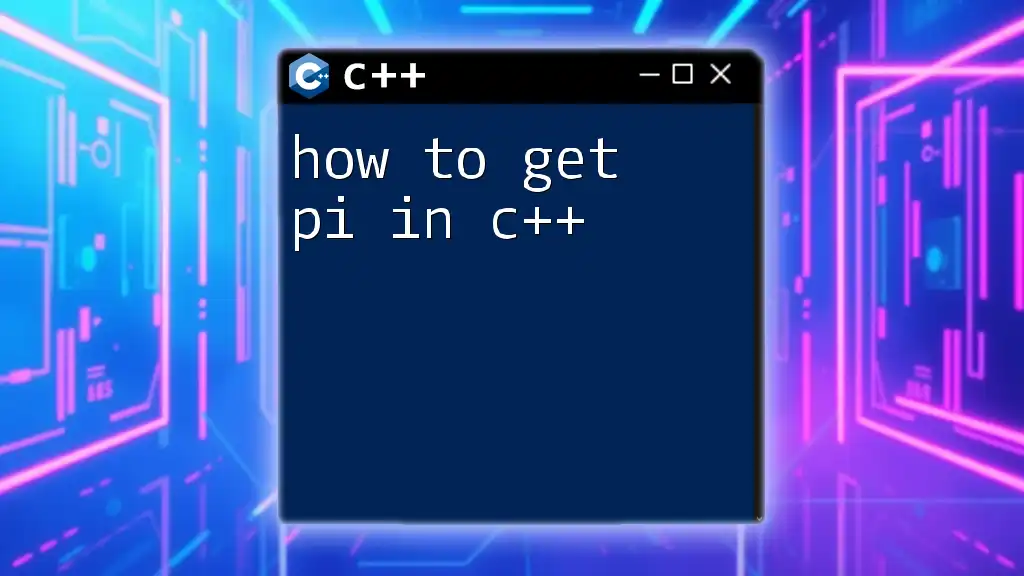
FAQ Section
What Other Formatting Options are Available in C++?
C++ offers additional manipulators such as `std::setw` for setting the width of output, `std::setprecision` for controlling the number of decimal places, and even libraries for advanced formatting options.
Can I Define My Own End Line Function?
Yes, you can define your own function to handle line endings; however, it's typically more efficient to use the built-in methods unless you have specific needs.
Why Doesn’t My Code Show New Lines As Expected?
If your code isn't generating new lines as anticipated, ensure there are no syntax errors and confirm the environment supports standard output appropriately.