To calculate the power of a number in C++, you can use the `pow()` function from the `<cmath>` library.
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Understanding Exponents in C++
What are Exponents?
Exponents represent the number of times a base is multiplied by itself. For instance, in the expression \(a^b\), \(a\) is the base, and \(b\) is the exponent. Understanding how to handle exponents is crucial in programming, particularly for calculations requiring power dynamics, such as simulations and scientific computations.
Why Use Powers and Exponents in C++?
In C++, powers and exponents find application in various domains, including scientific calculations, game development, and algorithms influenced by mathematical principles. For example, calculating trajectories in physics engines or modeling growth patterns in biological simulations heavily depends on exponentiation.
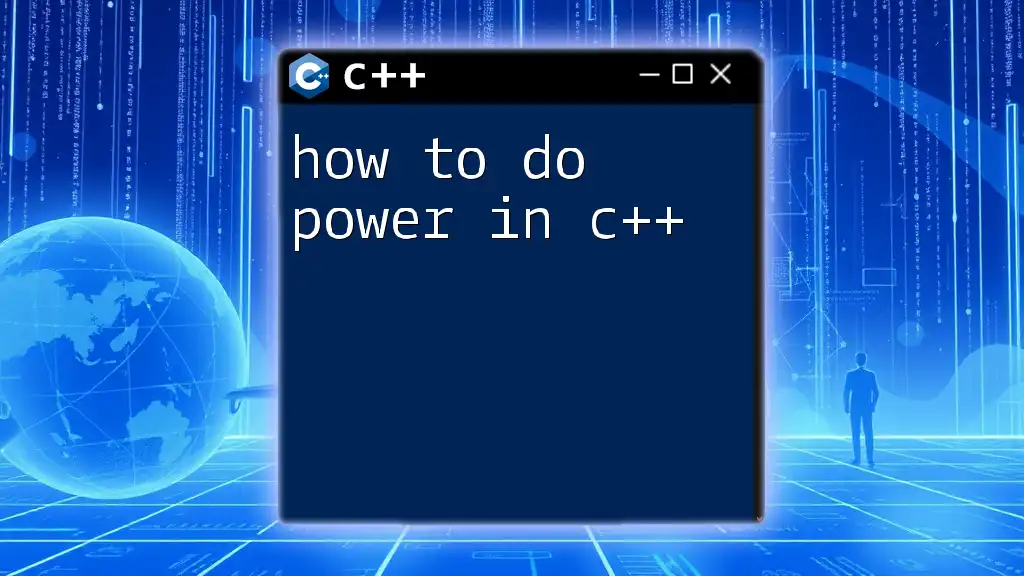
How to Write Exponents in C++
Using the Standard Library’s pow() Function
C++ provides a straightforward way to calculate powers via the `pow()` function, defined in the `<cmath>` library. This function allows you to compute base-to-the-exponent values efficiently. Its syntax resembles:
double pow(double base, double exponent);
Where `base` refers to the number to be raised to a power, and `exponent` is the power itself.
Example of Using pow() Function
To illustrate the usage of the `pow()` function, consider the following code snippet:
#include <iostream>
#include <cmath>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
Explaining the Example
- We include the `<cmath>` library to access the `pow()` function.
- We declare `base` as 2 and `exponent` as 3.
- The `pow()` function then computes \(2^3\), which is stored in the `result` variable.
- Finally, we print the outcome, which will output "2 raised to the power of 3 is 8".
Common Mistakes with pow()
When using the `pow()` function, one typical pitfall is overlooking type precision. The `pow()` function can return a floating-point number, which might lead to unexpected truncation or rounding errors if integers are expected. Always ensure that your types match the needs of your application; otherwise, you may experience accuracy issues.
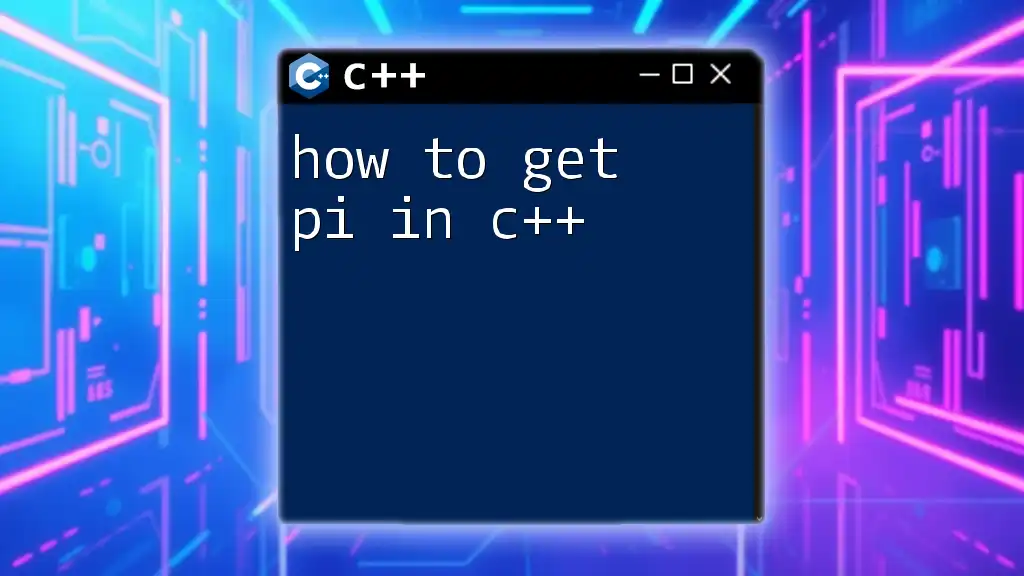
How to Use Power in C++
Working with Integer Powers Without Using pow()
Sometimes, especially in performance-critical applications, you might avoid using `pow()` for integer-based exponentiation. Instead, you can manually compute powers using iterative methods. Below is a simple implementation using loops:
Example of Manual Power Calculation
#include <iostream>
int power(int base, int exponent) {
int result = 1;
for(int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
int base = 3;
int exponent = 4;
std::cout << base << " raised to the power of " << exponent << " is " << power(base, exponent) << std::endl;
return 0;
}
Understanding the Loop in the Example
In this snippet, the `power` function iteratively multiplies the `base` for `exponent` times, effectively computing \(3^4\). When run, it will display "3 raised to the power of 4 is 81". This method is efficient for integer calculations and avoids potential pitfalls with floating-point arithmetic.
When to Use Manual Methods vs. pow()
Utilizing manual loops provides excellent performance benefits when you are working solely with integers, especially with small exponent values. In contrast, for complex calculations involving floating points or when leveraging performance optimizations, it is advisable to use the `pow()` function.
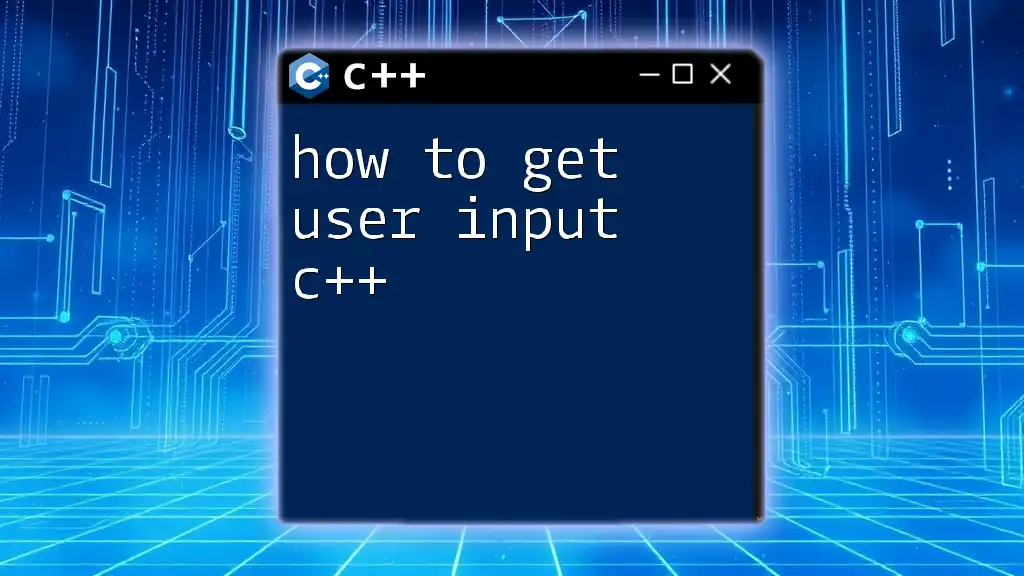
More Advanced Topics on Power in C++
Negative Exponents and Fractional Powers
In mathematics, a negative exponent represents the reciprocal of the base raised to the positive exponent, while fractional exponents denote roots. For example, \(a^{-b}\) results in \(\frac{1}{a^b}\), and \(a^{\frac{1}{b}}\) computes the \(b\)th root of \(a\).
Example with Negative and Fractional Powers
Consider this example that illustrates handling negative and fractional exponents using `pow()`:
#include <iostream>
#include <cmath>
int main() {
// Negative exponent
double result1 = pow(2.0, -3.0);
std::cout << "2 raised to the power of -3 is " << result1 << std::endl;
// Fractional exponent
double result2 = pow(27.0, 1.0/3.0);
std::cout << "27 raised to the power of 1/3 is " << result2 << std::endl;
return 0;
}
Breakdown of the Code
- The first computation demonstrates calculating \(2^{-3}\), which yields 0.125, or \(\frac{1}{8}\).
- The second computation finds the cube root of 27, producing 3, as \(27^{\frac{1}{3}} = 3\). This clearly shows how `pow()` accommodates both negative and fractional exponents seamlessly.
Error Handling with Exponents
It’s essential to be vigilant with exponent inputs, particularly regarding negative or zero bases with negative exponents, which can yield runtime errors or undefined behavior. Implementing checks for these conditions is crucial to robust code.
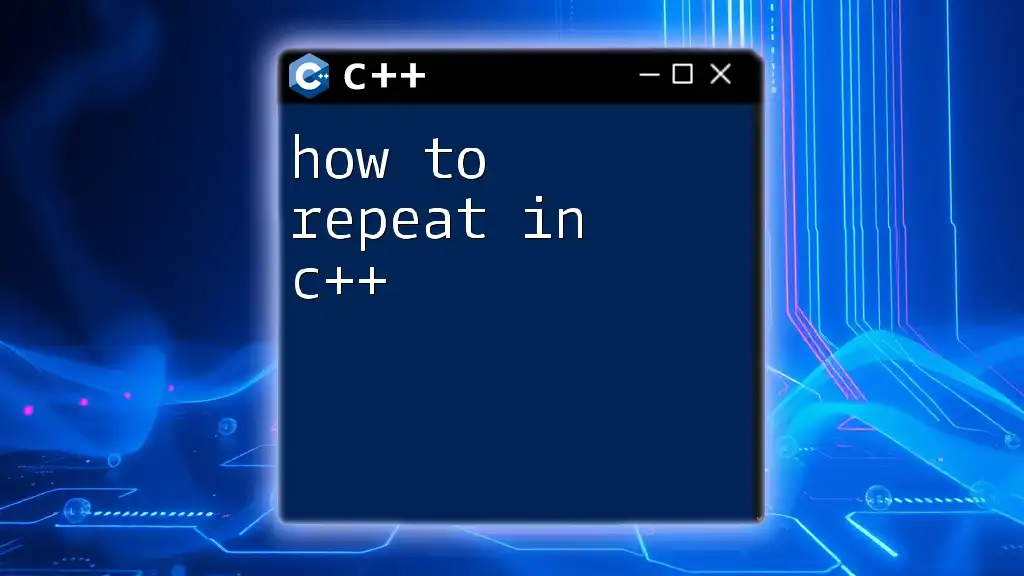
Conclusion
In summary, understanding how to get power in C++ opens the door to a wide array of programming possibilities. The `pow()` function from the `<cmath>` library provides a powerful yet simple method for computing powers, while manual calculations can offer performance benefits when working solely with integers. With knowledge of negative and fractional exponents and an awareness of potential pitfalls, you are well-equipped to tackle exponentiation in your C++ projects.
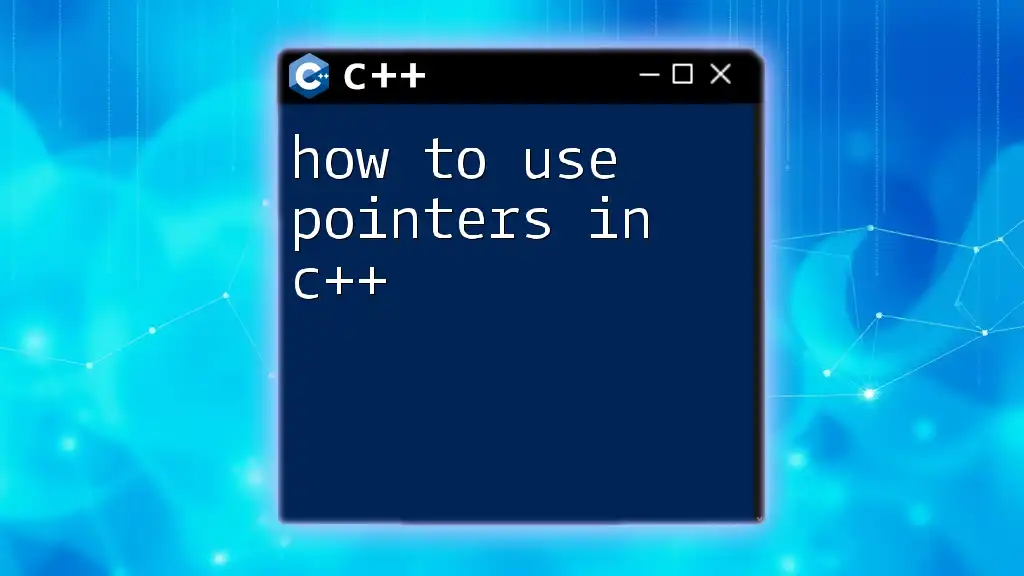
Additional Resources
Continued learning is vital for improving your skills. Explore books, websites, and tutorials dedicated to advanced C++ programming. Many communities and forums are available for discussion and collaboration; joining one can provide valuable insights and assistance.
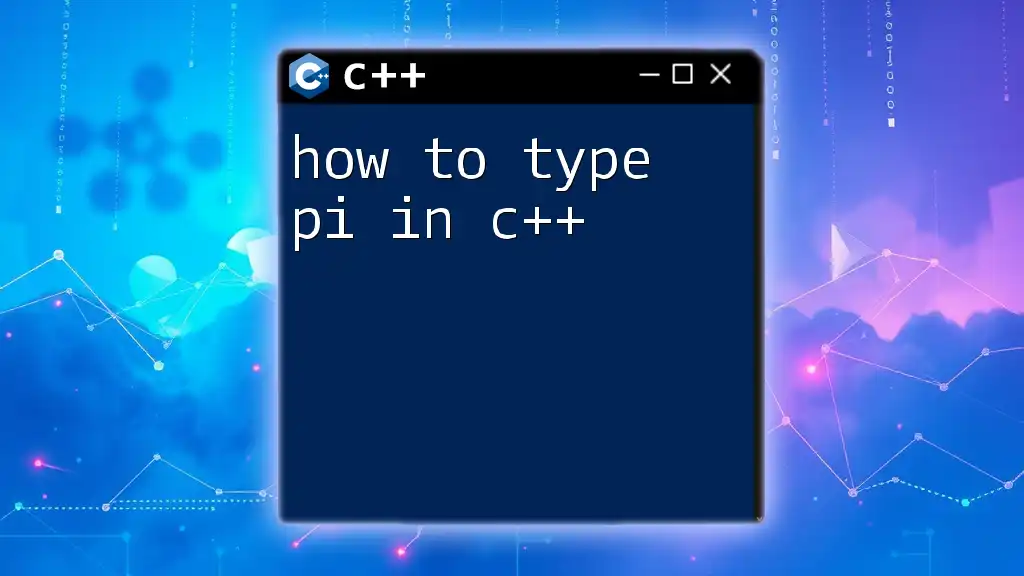
Call to Action
As you delve deeper into C++, consider enrolling in courses that can expand your understanding of this versatile language. Share your experiences and challenges in using power calculations in your projects, and embrace the journey of mastering C++. Your insights and questions can help foster discussions that benefit your fellow developers!