To obtain the value of π (pi) in C++, you can use the constant provided by the `<cmath>` library, which is defined as `M_PI`.
#include <iostream>
#include <cmath>
int main() {
std::cout << "Value of pi: " << M_PI << std::endl;
return 0;
}
Understanding the Pi Constant in C++
The concept of Pi (π) is fundamental in mathematics, representing the ratio of a circle's circumference to its diameter. In C++, Pi can be utilized in various applications, including geometry, engineering, and physics problems. It is essential to understand how to obtain and utilize Pi in your C++ code effectively.
Definition of a Constant
In programming, a constant is a value that remains unchanged during the execution of a program. Using constants like Pi improves readability and maintainability in your code. When you define mathematical constants, you can leverage them for accurate calculations without hardcoding values throughout your application.
Value of Pi
The value of Pi is approximately 3.14159265358979323846, but for most applications, a precision of 3.14 or 3.14159 is sufficient. When declaring Pi in your C++ code, it is crucial to maintain this precision to avoid errors in calculations.
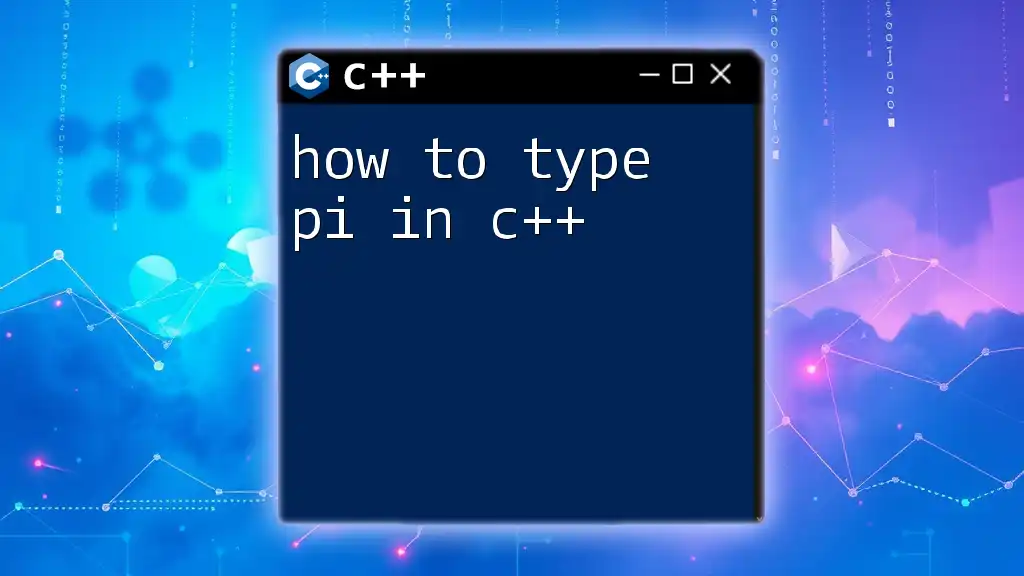
Accessing Pi in the cmath Library
Overview of cmath Library
The `cmath` library in C++ provides a collection of mathematical functions and constants. It is included in the C++ standard and is widely used for mathematical operations. Leveraging this library ensures consistency and provides built-in support for commonly used constants and functions.
Using the Pi Constant in cmath
In C++, the constant representing Pi can be referenced from the `cmath` library. From C++11 onward, you can access Pi using the `M_PI` constant. Below is a simple code snippet to illustrate this:
#include <iostream>
#include <cmath>
int main() {
std::cout << "Value of Pi: " << M_PI << std::endl;
return 0;
}
When you run this code, it will output the value of Pi, demonstrating how easy it is to work with this constant in C++.
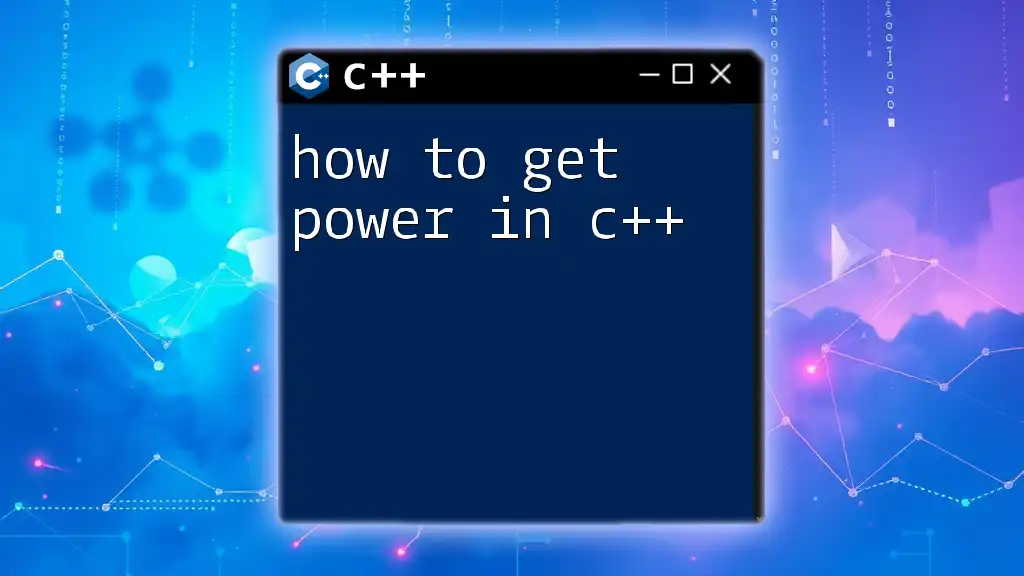
How to Declare Pi in C++
Manually Defining Pi
In some cases, you may want to define Pi manually instead of relying on the `cmath` library. This might be necessary if you're working with older C++ standards or prefer explicit definitions. You can define your Pi constant as follows:
const double MY_PI = 3.14159265358979323846;
Using Pi in Formulas
Once you have defined Pi, it can be effectively used in calculations. For example, using your manually defined Pi to calculate the area of a circle can be done as follows:
double radius = 5.0;
double area = MY_PI * radius * radius; // Area of a circle
std::cout << "Area: " << area << std::endl;
This code takes a radius of 5.0 units and calculates the area of the circle, illustrating the practical use of the Pi constant in your calculations.
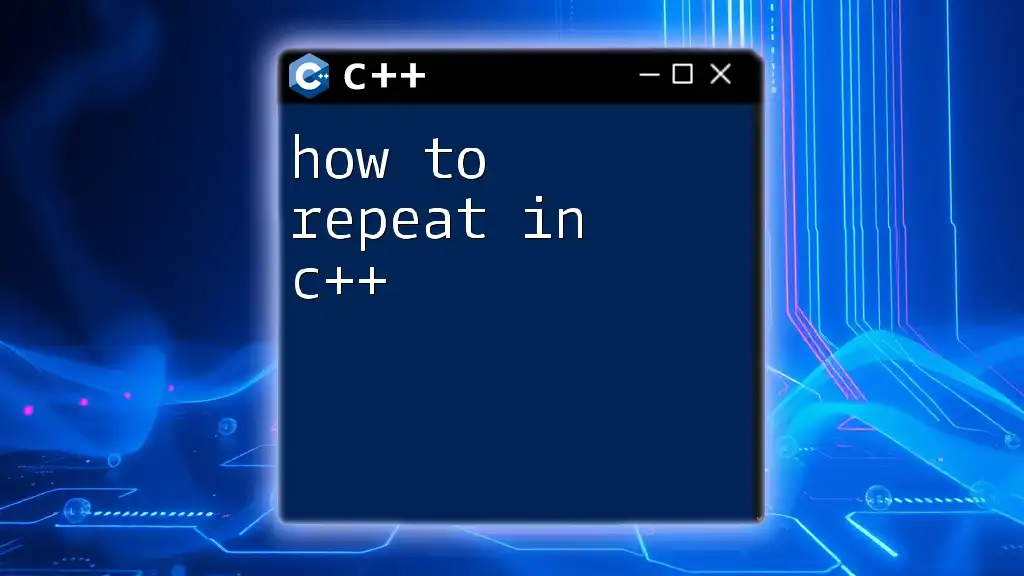
Applying Pi in C++ Calculations
Calculating the Circumference of a Circle
Understanding how to apply Pi in C++ calculations is essential. For example, the formula to calculate the circumference of a circle is:
Circumference = 2 * Pi * radius
Here is an example code snippet demonstrating this calculation:
double radius = 7.0;
double circumference = 2 * M_PI * radius;
std::cout << "Circumference: " << circumference << std::endl;
This code evaluates the circumference of a circle with a radius of 7.0 units, utilizing the value of Pi sourced from `cmath`.
Using Pi for Trigonometric Functions
Pi also plays a pivotal role in trigonometric functions. For instance, when working with angles in radians, Pi is essential for accurate computations. Here’s how you might calculate the sine and cosine of a 45-degree angle based on Pi:
double angle = M_PI / 4; // 45 degrees in radians
std::cout << "sin(45 degrees): " << sin(angle) << std::endl;
std::cout << "cos(45 degrees): " << cos(angle) << std::endl;
By using M_PI for angle conversion, you ensure that your calculations yield accurate results.
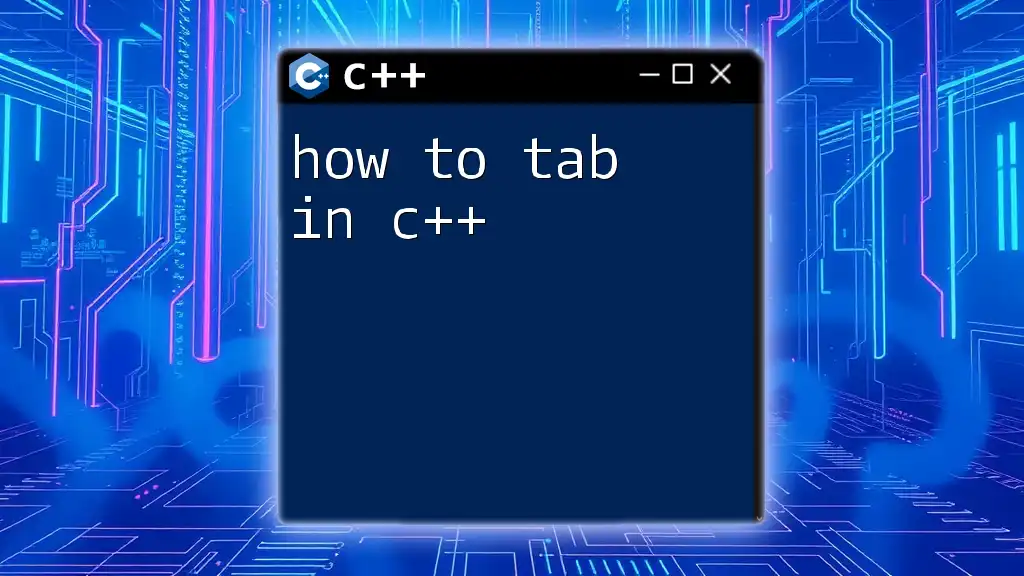
How to Use Pi in C++
Creating Functions that Use Pi
Encapsulating the use of Pi within functions can enhance code organization and reduce redundancy. For example, you can create a function to calculate the volume of a sphere:
double sphereVolume(double radius) {
return (4.0 / 3.0) * M_PI * pow(radius, 3);
}
This function utilizes the `pow` function from the `cmath` library to compute the volume based on the radius input. It also showcases the efficiency of defining reusable code in your C++ programs.
Best Practices for Using Pi in C++
To maximize the effectiveness of your programming with Pi, consider the following best practices:
-
Avoid Magic Numbers: Instead of hardcoding the value of Pi throughout your code, always use a constant (either from `cmath` or your defined value). This practice enhances readability and reduces the risk of errors.
-
Precision and Accuracy: Be mindful of floating-point inaccuracies when using Pi in calculations. It’s always good practice to test your functions for edge cases and ensure your results are correct.
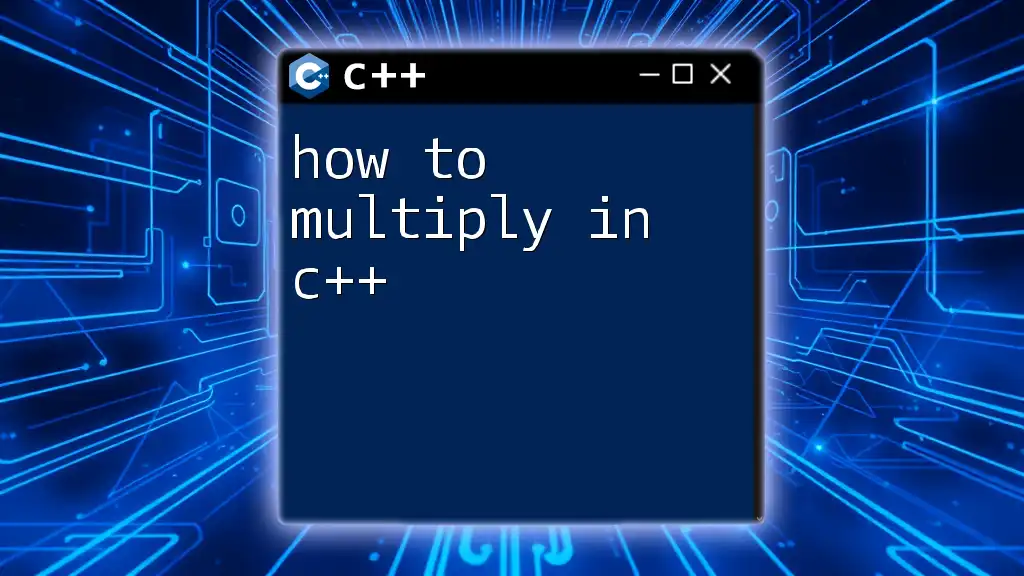
FAQs about Pi in C++
Common Pitfalls
When learning how to get Pi in C++, programmers often encounter issues such as:
- Overlooking the inclusion of the `cmath` header, which defines M_PI and other mathematical functions.
- Misunderstanding the need for using radians in trigonometric calculations rather than degrees.
Why Use the cmath Library for Pi?
Using the `cmath` library for Pi brings many advantages. It ensures consistency with other standard math functions and leverages any compiler optimizations on math operations, resulting in potentially better performance and reliability.
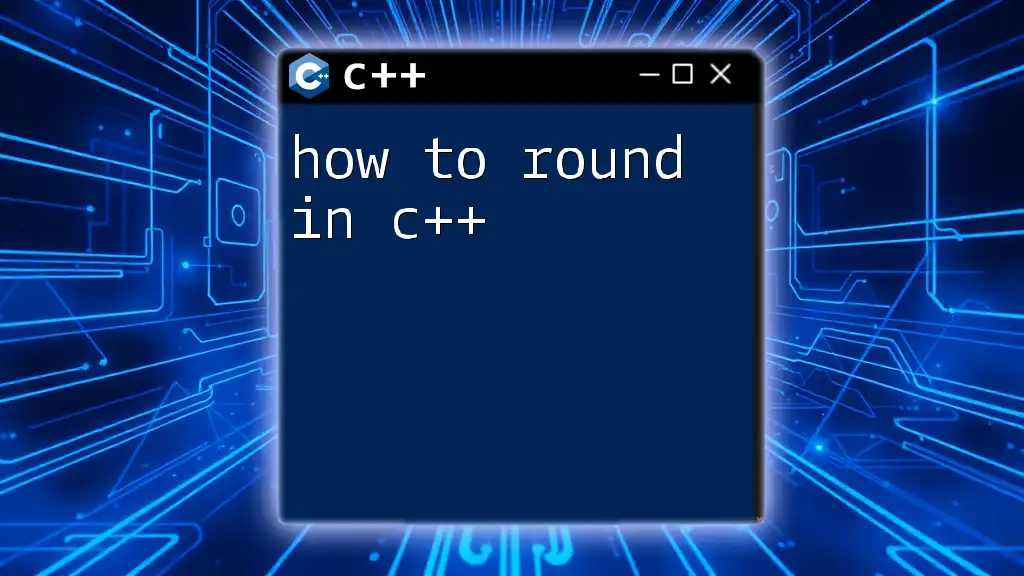
Conclusion
Incorporating Pi into your C++ programs is straightforward and essential for various mathematical computations. Whether you leverage the built-in `M_PI` from the `cmath` library or choose to define your constant, understanding how to get Pi in C++ lays the groundwork for accurate calculations in geometry and beyond. Practice using Pi in various programming scenarios, and you’ll find it becomes an invaluable tool in your coding toolkit.