To print output in C++, you can use the `std::cout` object from the `iostream` library, as shown in the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Output in C++
In C++, output refers to the data that a program generates and displays to the user. It plays a crucial role in various programming scenarios, such as debugging, displaying results, and facilitating interaction between components. Learning how to print effectively enhances your ability to communicate the results of computations, debug issues, and create user-friendly interfaces.
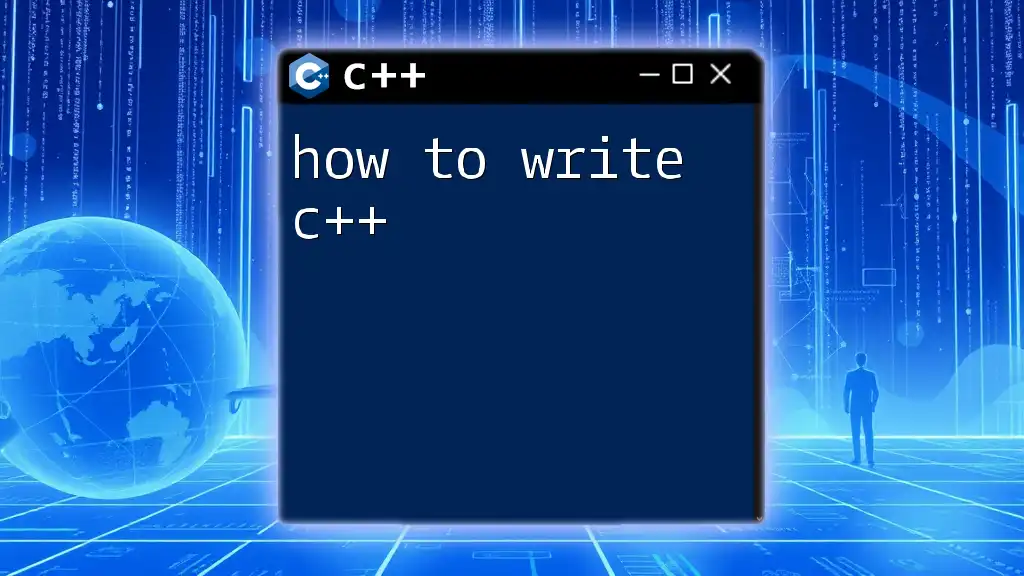
How to Print in C++: The Basics
Printing in C++ primarily involves using the standard output stream. The standard output stream is represented by `std::cout`, which is part of the `<iostream>` library. Before using `std::cout`, ensure you include the necessary header file in your code:
#include <iostream>
The Basic Print Statement
To print a message to the console, you can use the following syntax:
std::cout << "Hello, World!" << std::endl;
In this example, the text "Hello, World!" will be displayed in the console. The `<<` operator sends the string to the output stream, while `std::endl` adds a newline character and flushes the output buffer.
Understanding `std::endl`
Using `std::endl` is helpful for creating line breaks. However, you can achieve a similar effect using the newline character `\n`. For instance:
std::cout << "Hello, World!" << std::endl; // Line break
std::cout << "Hello, World!\n"; // Line break
Using `\n` is often more efficient in terms of performance since it does not flush the output buffer.
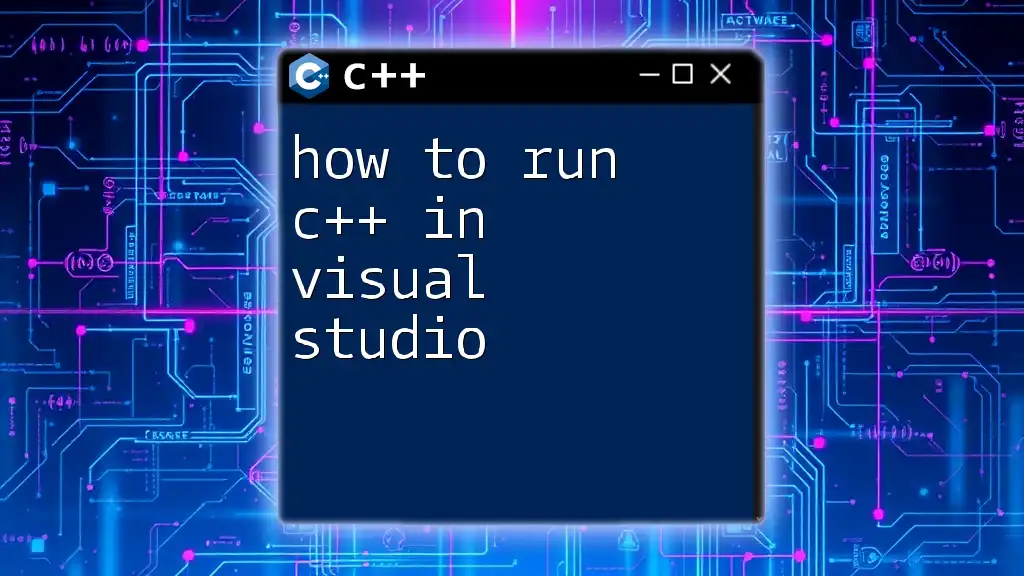
Advanced Printing Techniques in C++
Printing Different Data Types
C++ allows you to print various data types, including integers, floating-point numbers, and strings, using `std::cout`. Here's an example demonstrating how to print different types in a single line:
int number = 10;
double pi = 3.14;
std::string text = "Goodbye!";
std::cout << "Number: " << number << ", Pi: " << pi << ", Text: " << text << std::endl;
In this snippet, we print an integer, a double, and a string by chaining them with the `<<` operator.
Using Format Specifiers
C++ offers the `<iomanip>` library for finer control over formatting. You can adjust properties like decimal precision using format specifiers. For example, if you want to control how many decimal places are shown for a floating-point value:
#include <iomanip>
double value = 123.456789;
std::cout << std::fixed << std::setprecision(2) << value << std::endl; // Outputs 123.46
Using `std::fixed` ensures that the value is displayed in fixed-point notation, while `std::setprecision(2)` defines two decimal places.
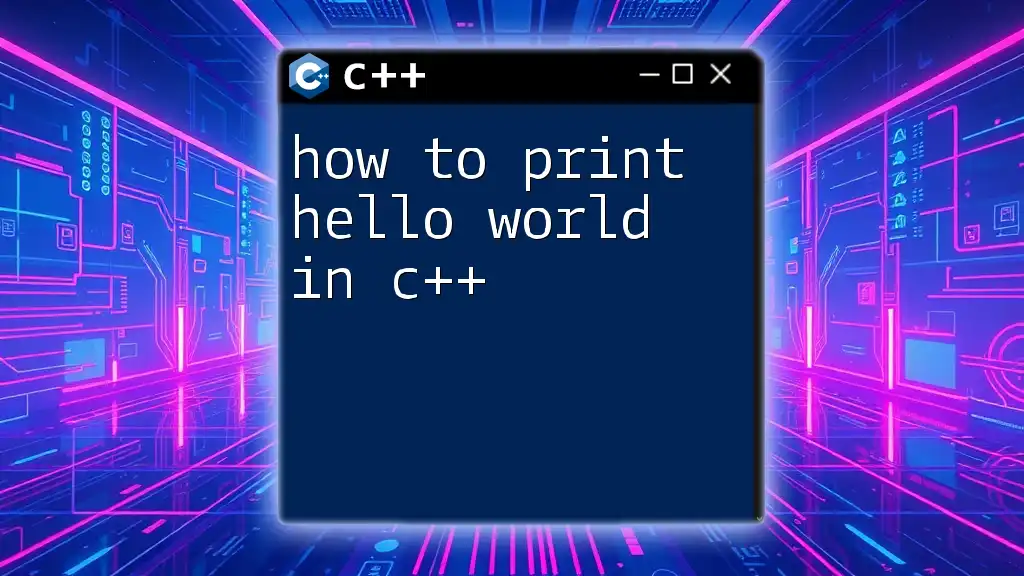
How to Print Something in C++: Practical Examples
Printing Arrays and Vectors
Printing collections such as arrays and vectors can be accomplished through loops. Here’s how to print the elements of an integer array:
int arr[] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
For vectors, the code snippet will look similar but will utilize range-based for loops for better readability:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5};
for (const auto& element : vec) {
std::cout << element << " ";
}
std::cout << std::endl;
Printing Using Loops
Loops are an excellent way to print multiple outputs. For instance, nested loops can be used to print combinations of indices:
for (int i = 1; i <= 3; ++i) {
for (int j = 1; j <= 3; ++j) {
std::cout << "(" << i << ", " << j << ") ";
}
std::cout << std::endl;
}
This generates a structured output of pairs, demonstrating the inner workings of loops in C++.
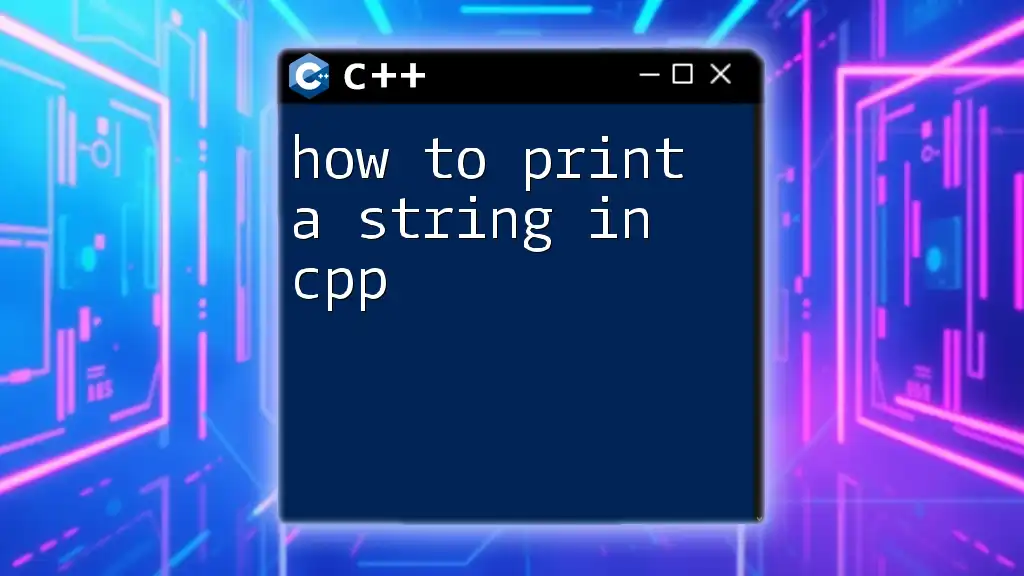
Enhancing Output in C++
Using Colors in Console Output
While C++ does not natively support colored text output, you can use ANSI escape codes or libraries like `windows.h` (on Windows systems) to enhance your console output. Here’s a basic example employing ANSI escape codes to print text in red:
std::cout << "\033[1;31mThis is red colored text\033[0m" << std::endl;
The `\033[1;31m` sequence changes the text color to red, while `\033[0m` resets the color back to default afterward.
Handling Output with Functions
Creating dedicated print functions can streamline your code and enhance readability. Here’s a simple example:
void printMessage(const std::string& message) {
std::cout << message << std::endl;
}
printMessage("Hello, this is a custom print function!");
With such functions, you can encapsulate printing logic and make your main code cleaner.
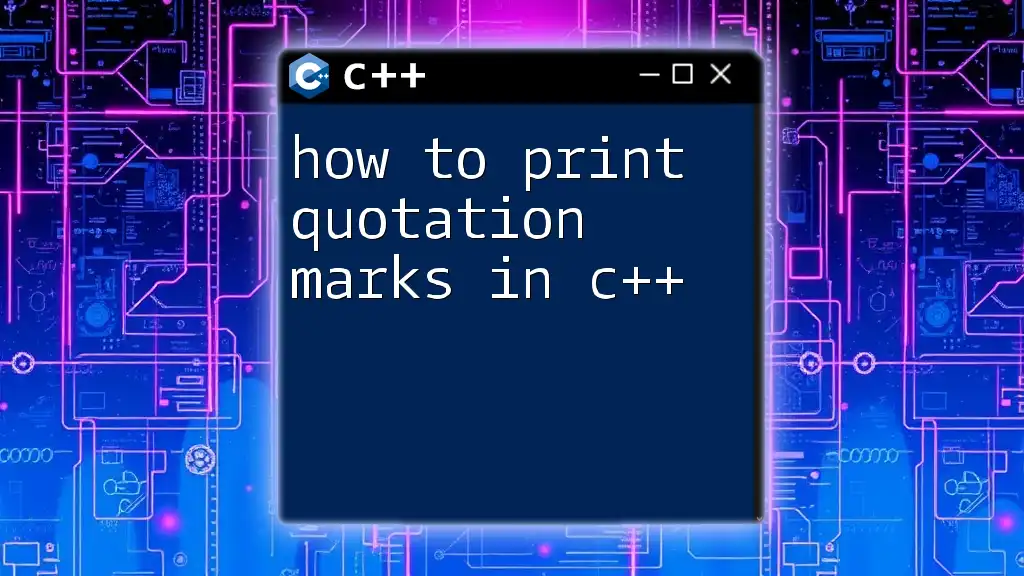
Common Mistakes in Printing
When learning how to print in C++, beginners often make a few common mistakes:
- Forgetting to Include Necessary Headers: Always include `#include <iostream>` at the beginning of your program.
- Not Properly Ending Statements: C++ statements must end with a semicolon (`;`). Omitting it will result in compilation errors.
- Printing Non-Printable Characters: Be aware that certain characters or data types (like custom classes without an overloaded `<<` operator) cannot be printed directly. Ensure proper implementation for custom objects.
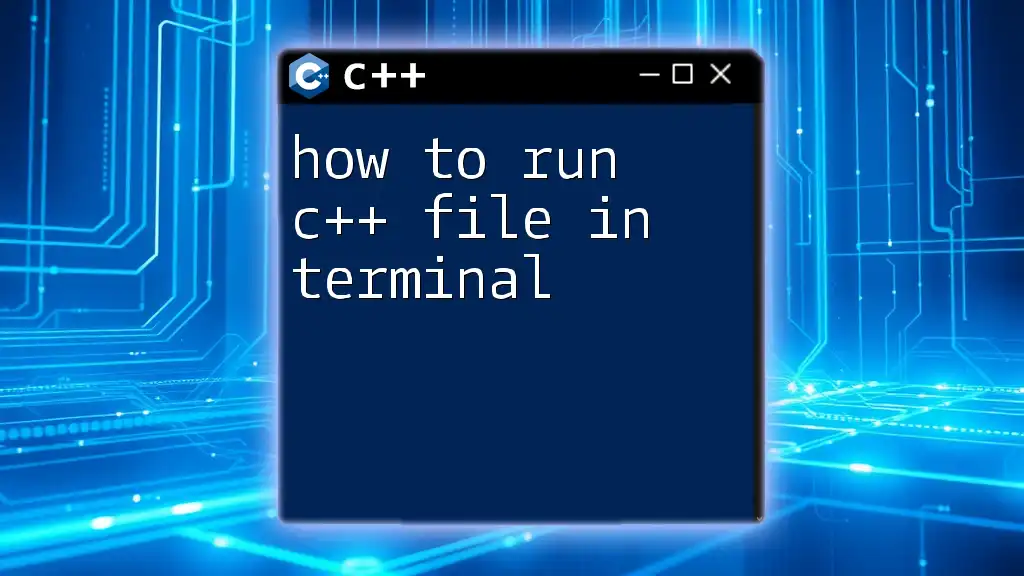
Conclusion
Understanding how to print in C++ is foundational for effective programming. It not only aids in debugging but also enhances the user experience by making outputs more informative and visually engaging. Mastering various printing techniques—from basic statements to formatting and function handling—will empower you in your programming journey.
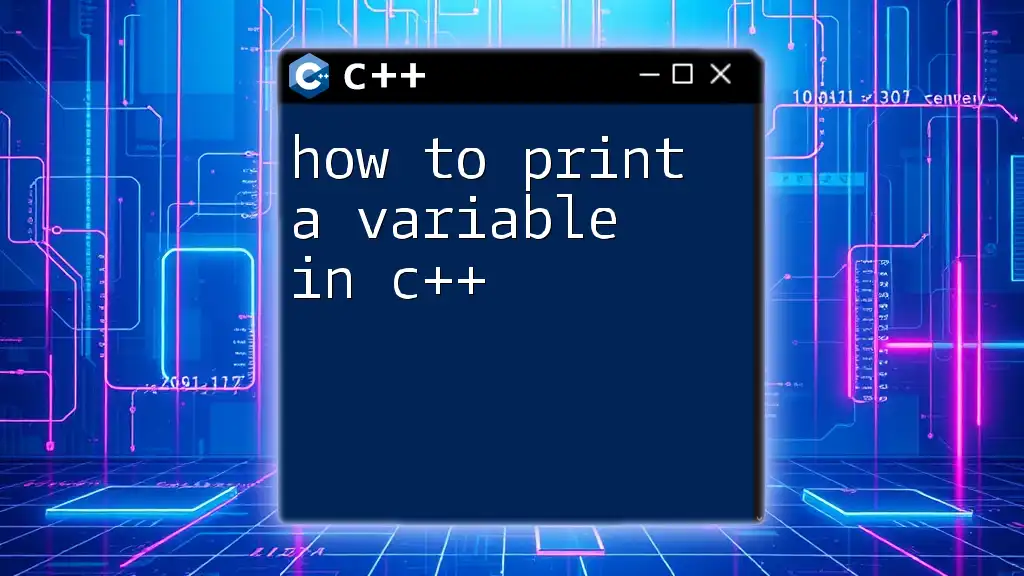
Call to Action
Join our C++ community today for more tips, tutorials, and resources. Share your own printing techniques and examples in the comments, and let’s enhance our C++ skills together!