In C++, you can add elements to a list using the `push_back` method provided by the `std::list` container from the Standard Library.
#include <iostream>
#include <list>
int main() {
std::list<int> myList;
myList.push_back(10); // Adds 10 to the end of the list
myList.push_back(20); // Adds 20 to the end of the list
for (int num : myList) {
std::cout << num << " "; // Outputs: 10 20
}
return 0;
}
Understanding Lists in C++
What is a List in C++?
A list in C++ refers to a container that holds a collection of elements. It is part of the Standard Template Library (STL) and provides a flexible way to manage data without worrying about the storage size in advance. Lists are particularly useful when you need a sequence of elements that may require frequent insertions and deletions, making them more efficient in these scenarios compared to other data structures, such as arrays.
C++ Standard Template Library (STL)
The Standard Template Library (STL) in C++ offers a variety of data structures and algorithms that simplify coding tasks. Lists in STL, specifically `std::list`, implement a doubly linked list, which allows easy manipulation of elements from both ends. The primary advantages of using STL lists include:
- Dynamic Size: Lists grow and shrink as needed without requiring explicit memory allocation.
- Ease of Insertion and Deletion: Operations to add or remove elements from a list are performed in constant time (O(1)), provided you have the iterator to the relevant position.
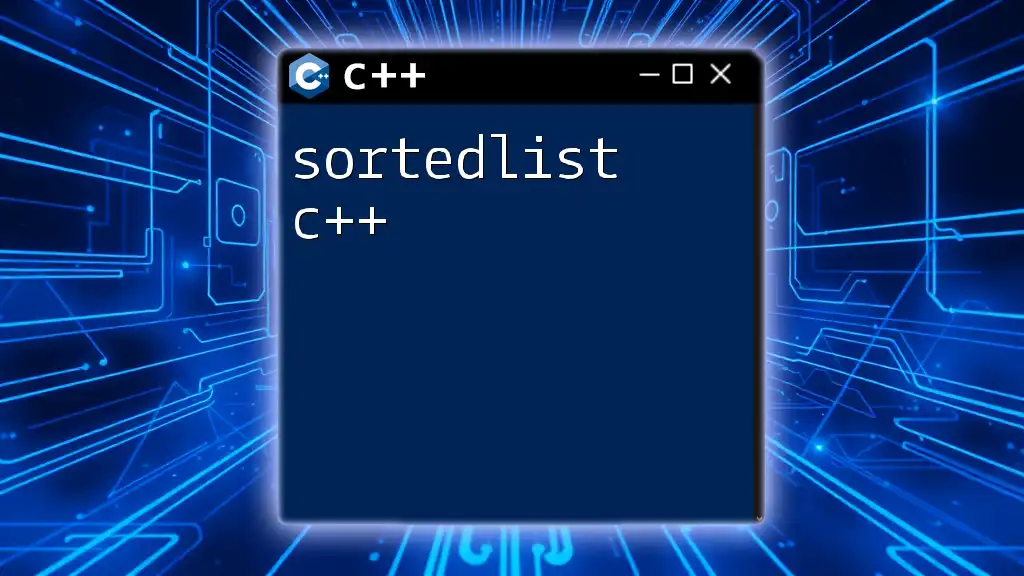
Creating a List in C++
Using `std::list`
To begin utilizing lists in C++, you first need to include the list header:
#include <list>
Once included, you can declare a list using the following syntax:
std::list<type> listName;
Where `type` can be any data type, such as `int`, `string`, or even user-defined types.
Example: Declaring a List
Here’s a simple code snippet that demonstrates how to declare a list of integers:
#include <iostream>
#include <list>
int main() {
std::list<int> myList; // Declaration of a list
return 0;
}
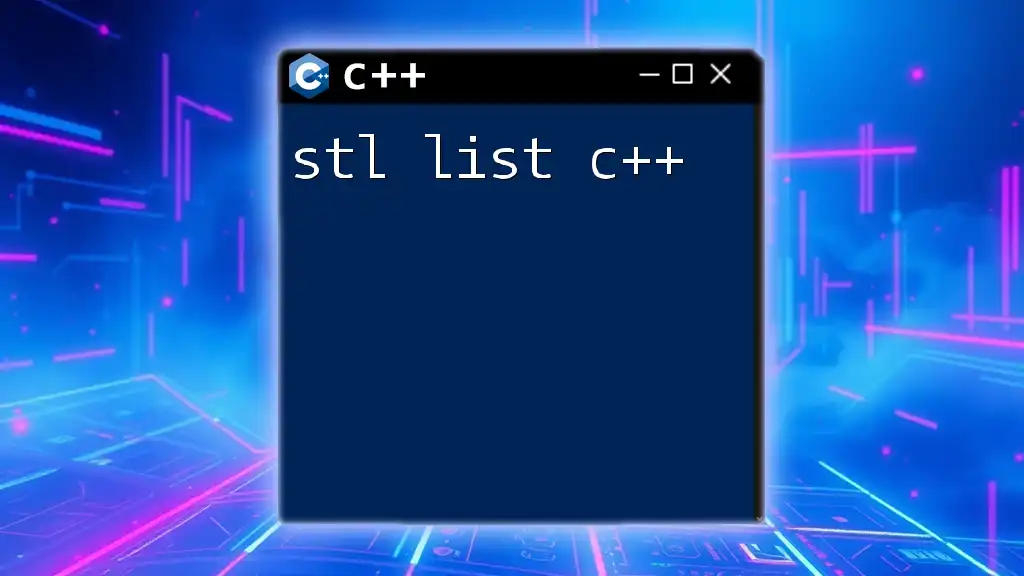
Adding Elements to a List in C++
Using `push_back()`
The `push_back()` function is employed to add an element to the end of the list. This is best used when you want to maintain the order of insertion and don't require immediate access to the front of the list.
Code Example: Adding Elements with `push_back()`
Here’s how you can use `push_back()` to add items to a list:
myList.push_back(10);
myList.push_back(20);
In this example, `10` is added first, followed by `20`, making the list look like this: `[10, 20]`.
Using `push_front()`
Conversely, the `push_front()` function adds an element to the beginning of the list. This function is particularly useful when you need to insert items at the front for priority reasons.
Code Example: Adding Elements with `push_front()`
Here’s an example:
myList.push_front(5);
After this operation, the list will appear as: `[5, 10, 20]`.
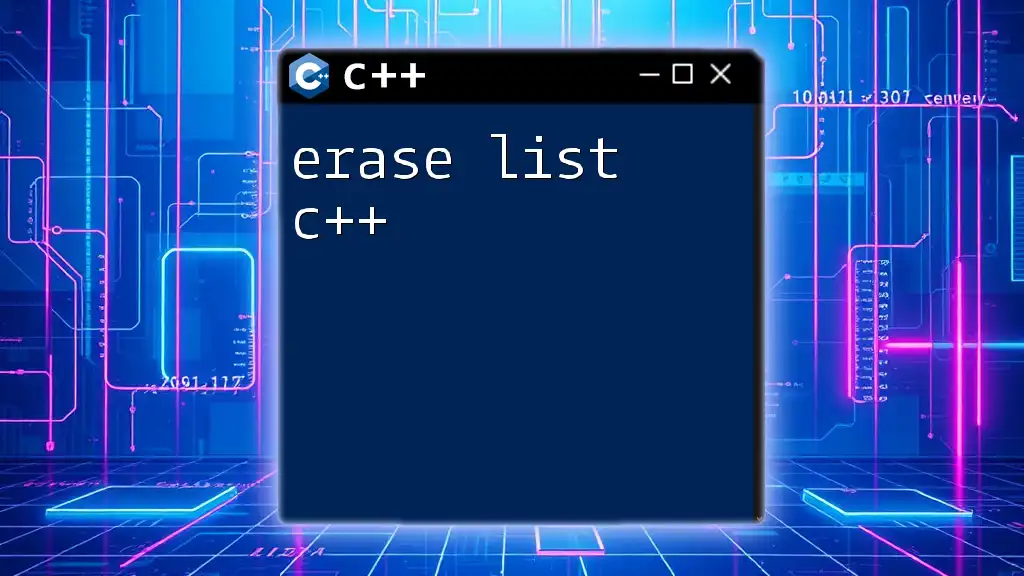
Inserting Elements in a List
Using `insert()`
The `insert()` function enables more precise control over where elements are added in the list. It allows you to specify the position in the list where the new element should be placed.
Code Example: Inserting at a Specific Position
Here's how to use the `insert()` function:
std::list<int>::iterator it = myList.begin();
myList.insert(it, 15); // Inserts 15 at the beginning
In this case, `15` is inserted at the very front, resulting in: `[15, 5, 10, 20]`.
Inserting Multiple Elements
You can also insert multiple elements at once using `insert()`. This is particularly handy when you have a collection of elements that you want to add at once.
Code Example: Inserting Multiple Elements
Here's how it works with a vector:
std::vector<int> vec = {30, 40, 50};
myList.insert(myList.end(), vec.begin(), vec.end()); // Inserts elements from vector
This snippet adds the elements `30`, `40`, and `50` to the end of the list, resulting in: `[15, 5, 10, 20, 30, 40, 50]`.
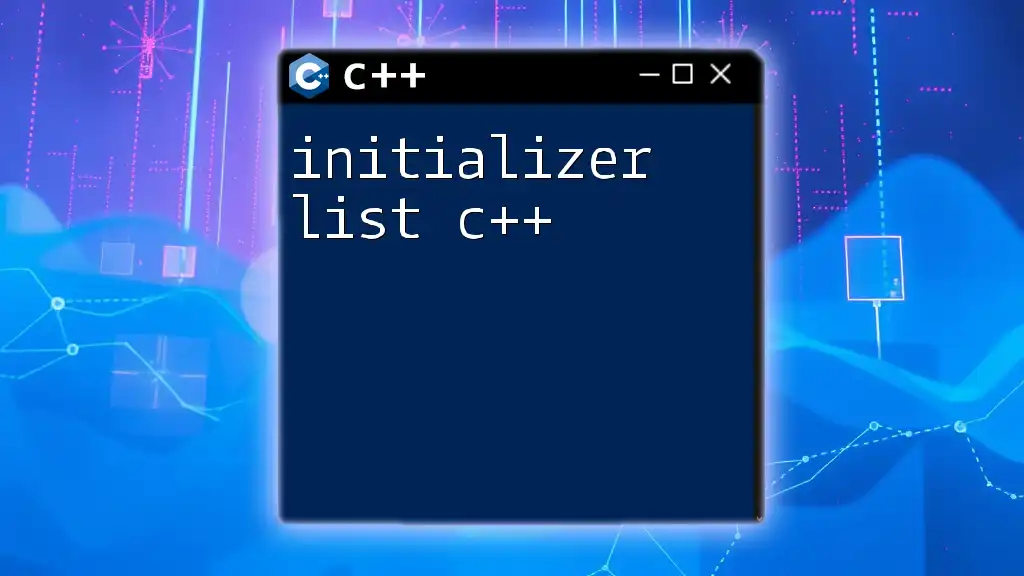
Best Practices for Adding to a List in C++
Choosing the Right Method
Understanding when to use which method can enhance the efficiency of your code. Use `push_back()` when you need to add items to the end, `push_front()` for adding to the beginning, and `insert()` when you need to place an element somewhere in between. Choosing the right method can save both time and computational resources.
Error Handling
When adding elements to a list, it is crucial to ensure that you handle potential issues, such as attempting to insert at an invalid iterator position. Remember to check the validity of iterators before using them.
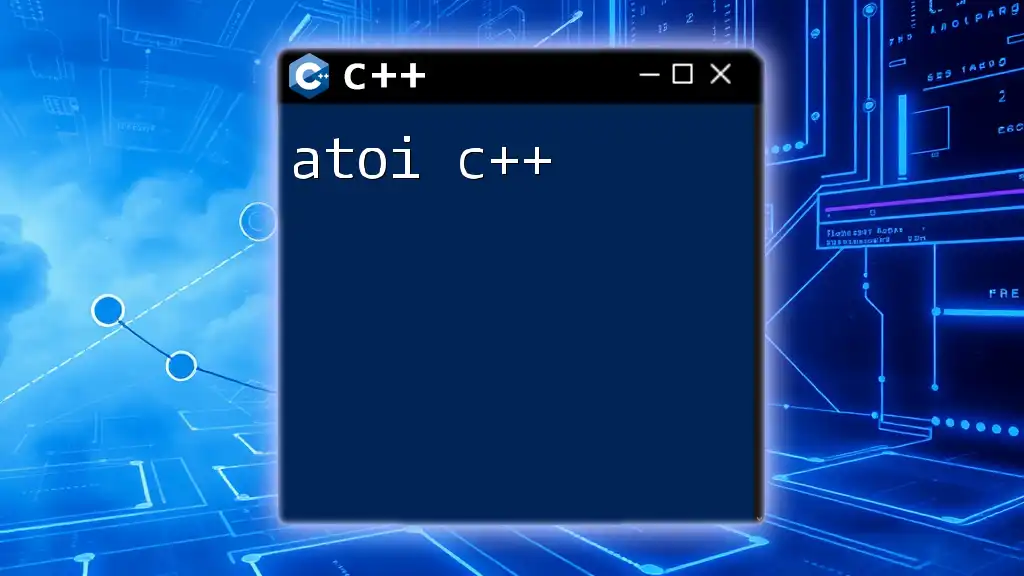
Practical Applications of Lists in C++
Use Cases for Lists
Lists can often be preferred over arrays in scenarios that require dynamic sizing or frequent changes—such as implementing queues, stacks, or any data structure involving frequent insertions and deletions. They are often employed in applications like scheduling, task management, and even gaming for managing collections of objects dynamically.
Real-World Example: Managing a Simple To-Do List
A practical implementation of C++ lists can be found in managing a to-do list application. Below is an example that showcases how easily you can manage tasks using a list.
Code Example: Interactive To-Do List Application
#include <iostream>
#include <list>
#include <string>
int main() {
std::list<std::string> todoList;
todoList.push_back("Learn C++");
todoList.push_back("Write a blog post");
// Display the list
for (const auto& item : todoList) {
std::cout << item << std::endl;
}
return 0;
}
This simple application allows users to manage their tasks and view them dynamically.
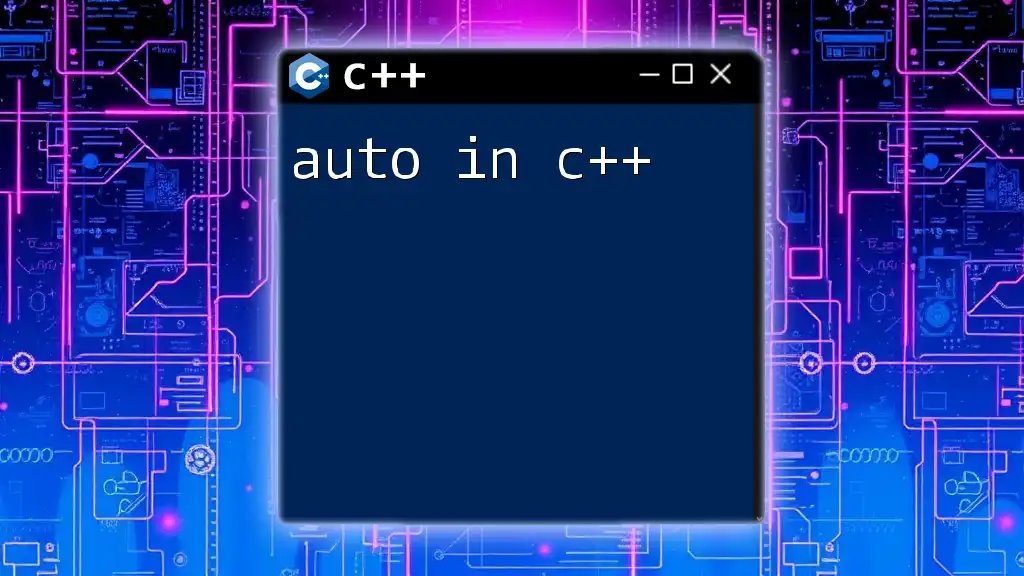
Conclusion
Recap of Key Takeaways
In this guide, we explored various methods to add to a list in C++, including `push_back()`, `push_front()`, and `insert()`. By understanding where and how to use these methods, you will be better equipped to manage collections of data efficiently.
Additional Resources
For those interested in diving deeper into C++ lists and the STL, recommended resources include books like "C++ Primer" and "Effective STL," as well as online courses that cover practical applications. Additionally, the official C++ documentation for `std::list` is an excellent place to learn more about its functionalities.
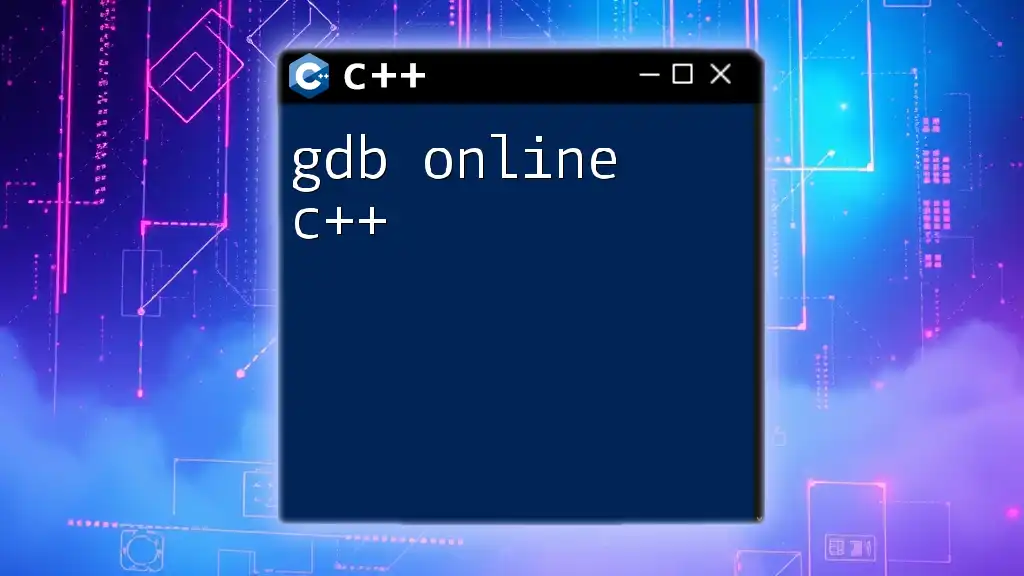
FAQs about Adding to a List in C++
Common Questions
-
What is the difference between `vector` and `list`? A vector is implemented as a dynamic array, providing fast access to elements through indexing, while a list is implemented as a linked list, offering efficient insertions and deletions.
-
Can you remove elements from a list? If so, how? Yes, elements can be removed from a list using the `remove()` method or the `erase()` function, which allows for precise targeting of the elements to be deleted.