An initialization list in C++ is a member initializer syntax used in constructors to initialize class member variables directly before the body of the constructor executes.
Here's a code snippet demonstrating an initialization list:
#include <iostream>
class Rectangle {
public:
int width, height;
Rectangle(int w, int h) : width(w), height(h) {} // Initialization list
};
int main() {
Rectangle rect(5, 10);
std::cout << "Width: " << rect.width << ", Height: " << rect.height << std::endl;
return 0;
}
Understanding Initialization in C++
What is Initialization?
In C++, initialization refers to the process of assigning an initial value to a variable or object. It is crucial because proper initialization prevents undefined behavior in your programs. There are several types of initialization, including direct initialization, copy initialization, and list initialization. Understanding these methods is essential for writing robust and efficient code.
Why Use Initialization Lists?
Initialization lists are particularly useful for constructors. They allow you to directly initialize member variables of classes and structures before the body of the constructor is executed. The benefits of using initialization lists are significant:
-
Performance Improvements: By using initialization lists, you avoid the overhead of default construction followed by assignment. This is especially important for complex types or when passing objects by reference.
-
Ensures Correct Initialization: For constant variables and references, it is mandatory to use an initialization list. If you try to initialize these types in the body of the constructor, the compiler will generate an error.
-
Clarity: Initialization lists can enhance the readability of your code by making it clear which variables are being initialized.

Syntax of Initialization Lists
Basic Syntax
The basic syntax of an initialization list involves using a colon followed by member initializations after the constructor’s parameter list. Here’s a simple example:
class Example {
public:
int a;
float b;
Example(int x, float y) : a(x), b(y) {}
};
In this example, `a` is initialized directly with the value of `x`, and `b` is initialized with `y`.
Key Components
The `:` symbol signifies the start of the initialization list. Each member variable assignment is separated by a comma. Understanding this format is crucial for mastering the use of initialization lists in C++.

Using Initialization Lists with Member Variables
Initializing Built-in Types
Initialization lists can be predominantly used with built-in types like integers and floating-point numbers. Consider the following example:
class MyData {
public:
int val;
MyData(int v) : val(v) {}
};
Here, `val` is initialized with the value of `v` via the initialization list, making the code both efficient and straightforward.
Initializing Objects of Other Classes
It’s not just primitive types that can be initialized through an initialization list; you can also initialize objects of other classes. Below is a complete example:
class AnotherClass {
public:
AnotherClass() {}
};
class MyClass {
public:
AnotherClass obj;
MyClass() : obj() {} // using an initialization list for an object
};
In this example, the `AnotherClass` object `obj` is initialized using the constructor initialization list, ensuring it is ready for use as soon as `MyClass` is constructed.
Initializing Constants and References
Constants and references must be initialized at the moment of their declaration, which makes the initialization list practically indispensable. Here’s how it looks:
class ConstRefExample {
public:
const int c;
int &ref;
ConstRefExample(int x, int &y) : c(x), ref(y) {}
};
In this example, `c` (a constant) and `ref` (a reference) are both initialized through the initialization list, conforming to the constraints imposed by their types.

Best Practices for Using Initialization Lists
When to Use Initialization Lists
Knowing when to utilize an initialization list can dramatically affect the performance and correctness of your code. Always prefer using initialization lists when:
- You are initializing member variables of class types.
- Working with class members that are references or constants.
- Base class constructors require parameters.
Common Pitfalls to Avoid
While initialization lists are powerful, there are common mistakes that programmers may encounter:
-
Forgetting to initialize references or constants: This will lead to compilation errors.
-
Relying solely on assignment in the constructor body: This can lead to unnecessary overhead and missed optimizations.
Formatting and Readability
For improved readability, especially in lengthy constructors, maintain clear formatting. Use spaces and indentation thoughtfully. Adding comments can also help explain the purpose behind specific initializations.

Advanced Topics in Initialization Lists
Mixing Initialization Lists with Default Parameters
Combining default constructor parameters with initialization lists can be a powerful technique. Here’s how that might look in practice:
class MyClass {
public:
int a;
float b;
MyClass(int x = 10, float y = 5.0) : a(x), b(y) {}
};
In this case, if no arguments are passed to `MyClass`, it will default to initializing `a` to 10 and `b` to 5.0.
Virtual Base Classes and Initialization Lists
When dealing with virtual inheritance, you may find that initialization lists become crucial for ensuring that the base class is properly constructed. Here's an example to clarify this concept:
class Base {
public:
Base(int x) {}
};
class Derived1 : virtual public Base {
public:
Derived1(int x) : Base(x) {}
};
class Derived2 : virtual public Base {
public:
Derived2(int x) : Base(x) {}
};
class Final : public Derived1, public Derived2 {
public:
Final(int x) : Base(x), Derived1(x), Derived2(x) {}
};
In this structure, `Base` is a virtual base class, and the initialization list in `Final` ensures that it is constructed appropriately.
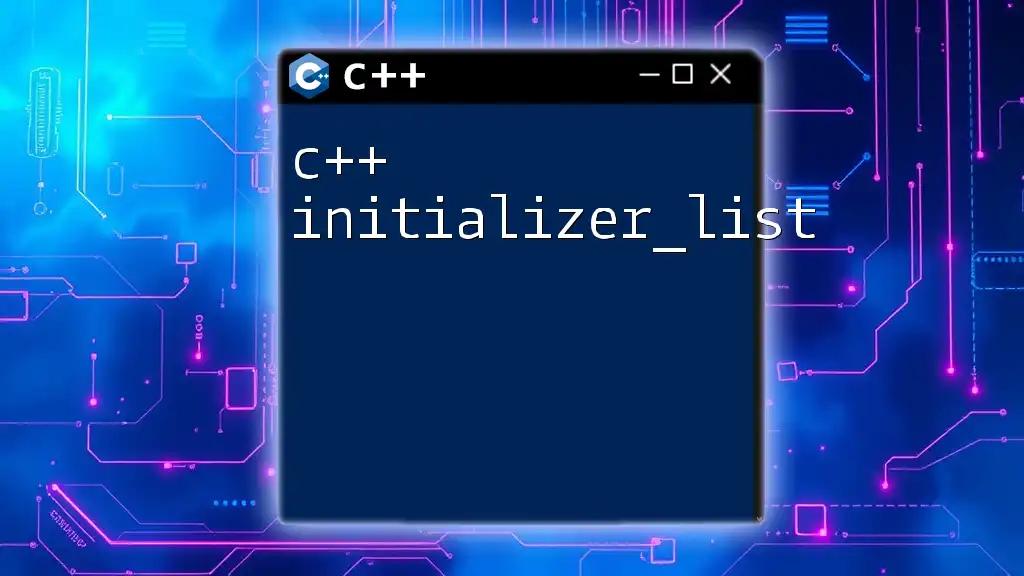
Conclusion
In conclusion, initialization lists in C++ are a vital component for efficient class design and robust object-oriented programming. Using them correctly can enhance your code’s performance, readability, and maintainability. Engaging with this topic will undoubtedly deepen your understanding of C++ and elevate your programming skills. Practice using initialization lists in your own projects and observe how they can improve your coding practices. Connect with the community to share your experiences and learn from others as you continue your journey in mastering C++.
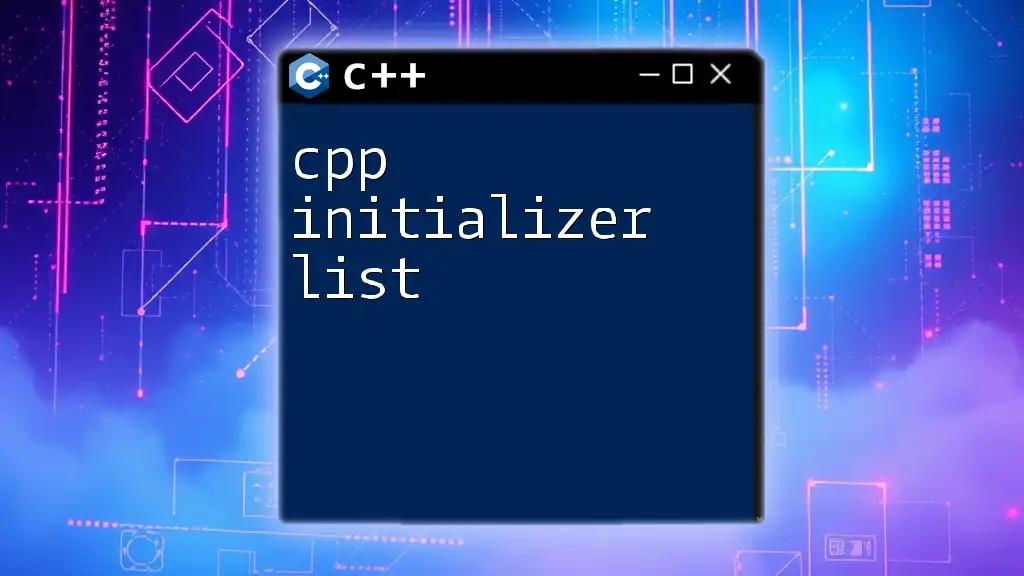
Further Resources
Explore recommended books, online tutorials, and active forums to expand your understanding and proficiency in C++. Engaging with fellow C++ enthusiasts can help solidify your knowledge of concepts like initialization lists, ultimately leading to mastery of this powerful programming language.