A member initializer list in C++ is a way to initialize class member variables directly in the constructor's signature, allowing for efficient and clear assignment of values.
class MyClass {
public:
int x;
int y;
MyClass(int a, int b) : x(a), y(b) {} // Member initializer list
};
What is a Member Initializer List?
A member initializer list in C++ is a powerful feature that allows initialization of class members before the body of the constructor executes. This is crucial for efficient object creation since certain types of member variables, especially const members and references, can only be initialized using an initializer list.

Syntax of Member Initializer List
The syntax of a member initializer list follows this general structure:
ClassName::ClassName(type param1, type param2) : member1(arg1), member2(arg2) {
// Constructor body
}
Here, `ClassName` is the name of your class, and `member1` and `member2` are the class members being initialized.
Key components of the syntax:
- Constructor Name: The constructor name must match the class name.
- Member Initializer List: All member initializations are listed after the colon (`:`), separated by commas.
- Constructor Body: The constructor body follows the initializer list for additional setup or logic.
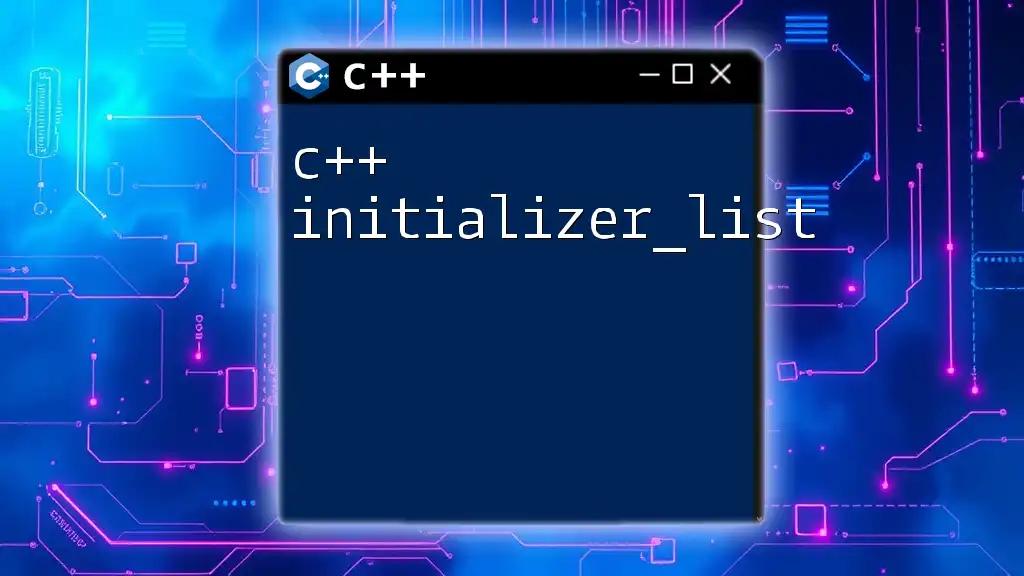
Why Use Member Initializer Lists?
Using member initializer lists provides several significant benefits over executing assignments within the constructor body.
-
Performance Improvements: For certain data types, especially complex objects, using a member initializer list can lead to better performance. Initialization happens directly rather than first calling a default constructor followed by assignment.
-
Necessity for `const` and Reference Members:
- Const Members: You must initialize constant members in the initializer list because their values cannot change once set.
- Reference Members: References must be initialized upon declaration; otherwise, a compiler error occurs.
-
Direct vs. Default Initialization: By using an initializer list, you engage in direct initialization. This avoids unnecessary default initialization followed by assignment, which can be less efficient.
Example Scenario: Using Member Initializer Lists
Consider the following example that demonstrates the use of initializer lists for `const` and reference types.
class Example {
const int value; // Constant member
int& ref; // Reference member
public:
Example(int val, int& r) : value(val), ref(r) {
// Constructor body
}
};
In this code:
- The `value` member must be set through the initializer list since it's `const`.
- The reference `ref` is also initialized directly.
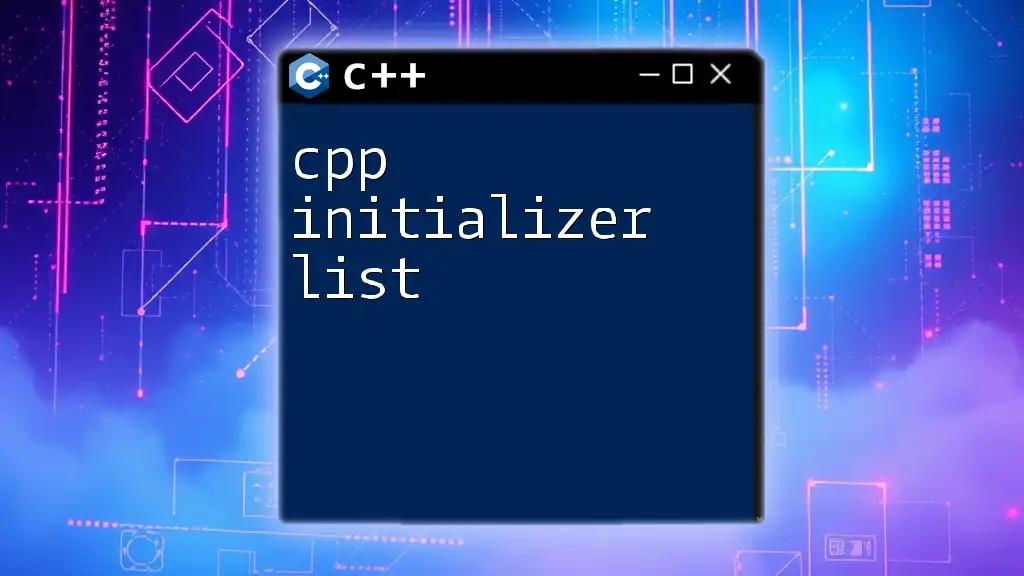
When to Use Member Initializer Lists
Member initializer lists are especially beneficial in several situations:
- Using `const` Members: Any class member declared as `const` must be initialized in the initializer list.
- Initializing Reference Members: Like `const` members, references cannot be left uninitialized. You must set them in the initializer list.
- Base Class Constructors: When deriving from a base class, you need to invoke the base class's constructor in the member initializer list.
Example Usage
Consider the following example with a base class and a derived class:
class Base {
public:
Base(int x) {
// Initialization logic for Base
}
};
class Derived : public Base {
public:
Derived(int x) : Base(x) {
// Derived class constructor body
}
};
In this scenario, the derived class `Derived` needs to call the constructor of `Base` using the member initializer list, allowing for proper initialization of both classes.

Common Mistakes and Pitfalls
When using the member initializer list, several common mistakes can lead to confusion or compilation errors:
- Incorrect Initialization: For instance, forgetting to include a member in the initializer list could lead to uninitialized members.
- Repeated Initializations: Declaring a member in the initializer list and attempting to reinitialize it in the body can cause errors or logic flaws.
Illustrative Code Snippets
Here’s an example of a common mistake regarding member initialization:
class Mistake {
int a;
int b;
public:
Mistake(int value) : a(value) {
b = value; // Wrong: should also use the initializer list for b
}
};
In this code, `b` is assigned a value in the constructor body instead of the initializer list, potentially leading to a misunderstanding of when `b` is initialized.

Best Practices
To effectively utilize member initializer lists, consider these best practices:
- Always Prefer Member Initializer Lists for `const` and Reference Members: Use initializer lists for any member that is `const` or a reference to ensure proper initialization.
- Initialize Members in their Declaration Order: C++ initializes members in the order they are declared, not in the order listed in the initializer list. Adhering to this ordering prevents confusion and potential errors.

Conclusion
In summary, understanding member initializer lists in C++ is essential for efficient programming. They allow for proper initialization of class members, enhance performance, and are necessary in specific scenarios. By leveraging the benefits of member initializer lists, you can write cleaner, more efficient C++ code.

Additional Resources
For further reading, you can explore:
- C++ initialization and constructors
- Advanced C++ features related to initialization
- Suggested exercises to practice member initializer lists for deeper understanding.