An implementation file in C++ typically contains the definitions of functions and methods declared in a corresponding header file, allowing for separation of interface and implementation.
Here’s a simple example of an implementation file named `example.cpp` that defines a function declared in a header file `example.h`:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
void greet();
#endif // EXAMPLE_H
// example.cpp
#include <iostream>
#include "example.h"
void greet() {
std::cout << "Hello, World!" << std::endl;
}
What is an Implementation File?
An implementation file in C++ serves as a repository for the functional code that corresponds to the declarations made in a header file. The typical extension for such files is `.cpp`. The primary role of an implementation file is to define the logic behind functions, classes, and methods that have been declared in the associated header file (`.h`). This separation of concerns is crucial to organizing the codebase, making it easier to read, maintain, and debug.
Distinction Between Header Files and Implementation Files
While header files primarily contain declarations and interface information, implementation files focus on the actual code processing. This distinction allows developers to modify implementation details without altering the interface, thereby preventing the need to recompile all dependent files.
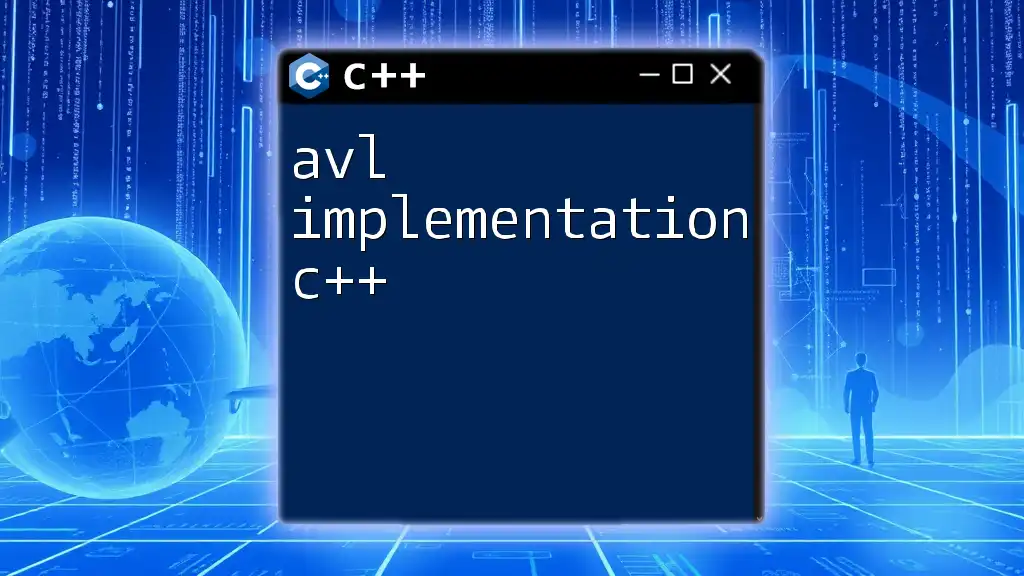
Basic Components of a C++ Implementation File
Understanding the structure of an implementation file is essential for any C++ developer. The basic layout includes:
- Preprocessor Directives: The `#include` directive is used to include the necessary header files.
- Function Definitions: Code segments where the actual functionality of declared methods is defined.
Example of a Simple Implementation File
Here’s a basic example of an implementation file:
#include "example.h"
void exampleFunction() {
// Implementation code goes here
std::cout << "Example function called!" << std::endl;
}
This code snippet demonstrates the simplicity of an implementation file. The `exampleFunction()` prints a message when called, showcasing how a function is defined outside of the header file.
File Naming Conventions
Using clear and meaningful names for implementation files can significantly enhance code clarity. Typically, the implementation file shares the same base name as its corresponding header file. For instance, if your header file is named `example.h`, the implementation should be `example.cpp`. This consistency reduces confusion and improves maintainability.
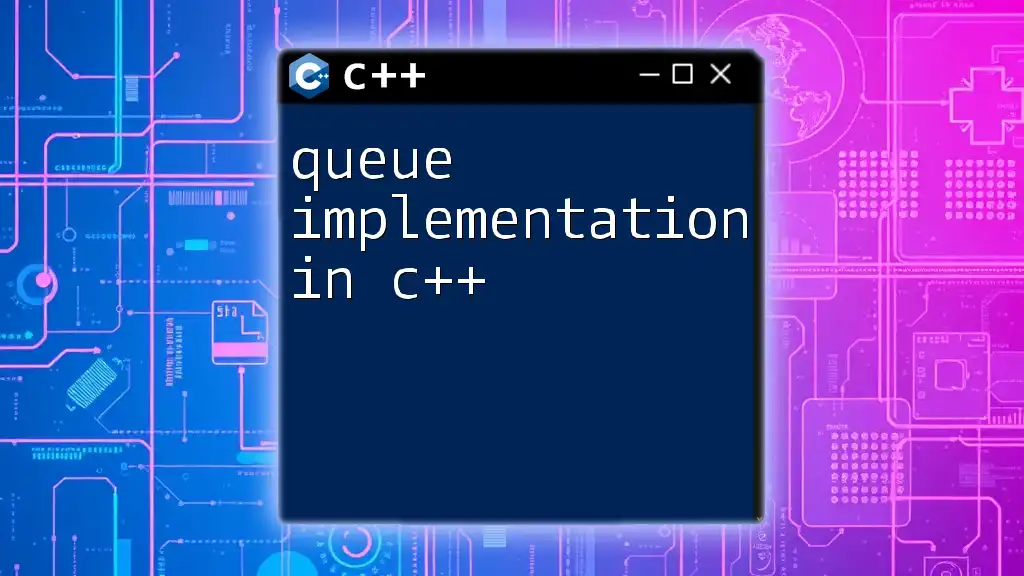
Writing a C++ Implementation File
Creating an implementation file is straightforward if you follow a logical procedure.
Step-by-Step Guide
- Choosing a Descriptive Name: The file name should reflect its contents for easier identification.
- Creating Appropriate Function Definitions: Ensure the implementations align perfectly with their declarations in the header file.
Code Snippet: Writing a Basic Class Implementation
Here’s how you can implement a class in C++:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
class Example {
public:
void showMessage();
};
#endif // EXAMPLE_H
// example.cpp
#include "example.h"
#include <iostream>
void Example::showMessage() {
std::cout << "Hello, World!" << std::endl;
}
In this example, the class `Example` is defined in the header file with a single method, `showMessage()`, which is implemented in the corresponding `.cpp` file. This structure not only maintains a clean organization but also enhances reusability across projects.
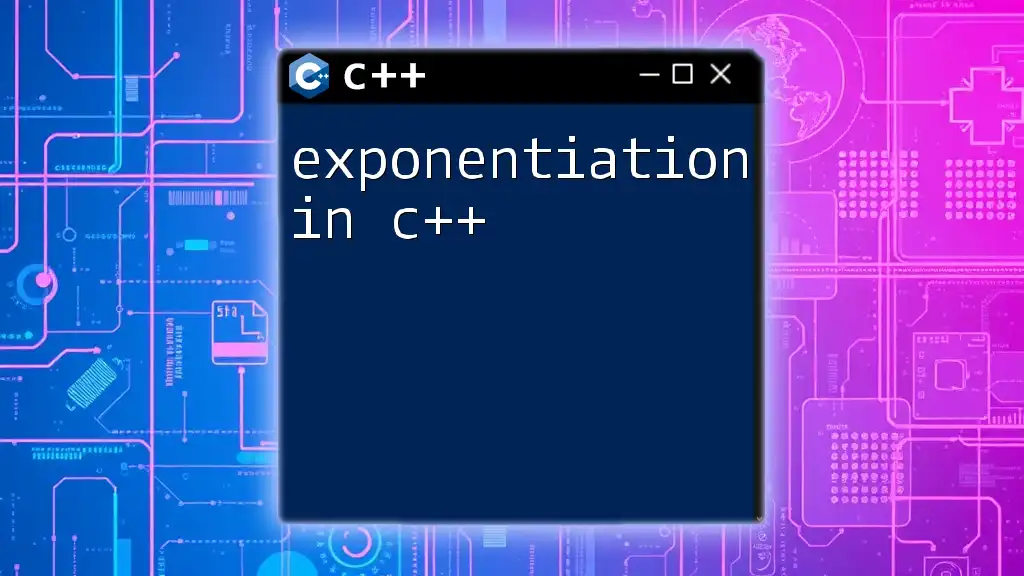
Organizing Code within Implementation Files
Well-organized code within implementation files can vastly improve your workflow and collaboration with other developers.
Grouping Related Functions and Classes
When developing, keep related functions and classes together. This logical grouping makes it easy to navigate your code and find relevant parts when necessary. Separating functionality into different files can also be beneficial, especially for larger projects.
Use of Namespaces
Namespaces are useful for managing code scope and avoiding naming conflicts. Here's an example:
namespace exampleNamespace {
void functionOne() {
std::cout << "Inside function one." << std::endl;
}
}
Using namespaces can help maintain a clean global environment, particularly in large applications where multiple developers might create similar function names.
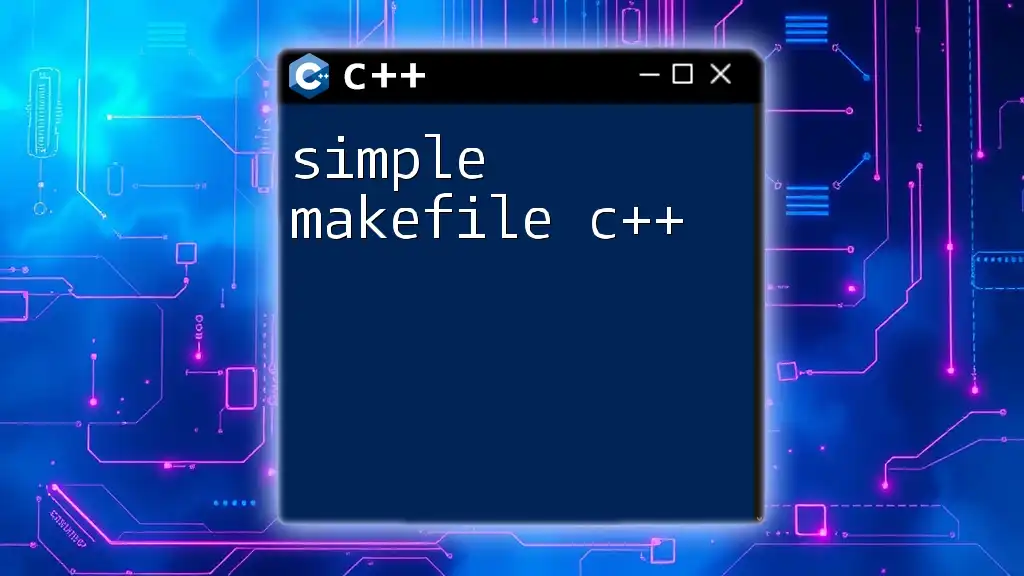
Common Mistakes in Implementation Files
Even seasoned developers may stumble upon common pitfalls while creating implementation files.
Overlooking Header Guards
Header guards are critical for preventing multiple inclusions of the same header file, which could lead to compilation errors. Always include header guards like so:
#ifndef EXAMPLE_H
#define EXAMPLE_H
// Declarations...
#endif // EXAMPLE_H
This pattern ensures that your header file's contents are included only once during compilation, thereby preventing potential issues.
Not Including Required Libraries
Always make sure to include all necessary libraries. Failing to do so can result in compiler errors when the implementation file references functions from those libraries.
Failing to Match Function Definitions with Declarations
It's crucial that function signatures in the implementation file match their declarations in the header file, including the name of the method, return type, and parameters. Mismatched signatures can lead to frustrating errors that are often difficult to trace.
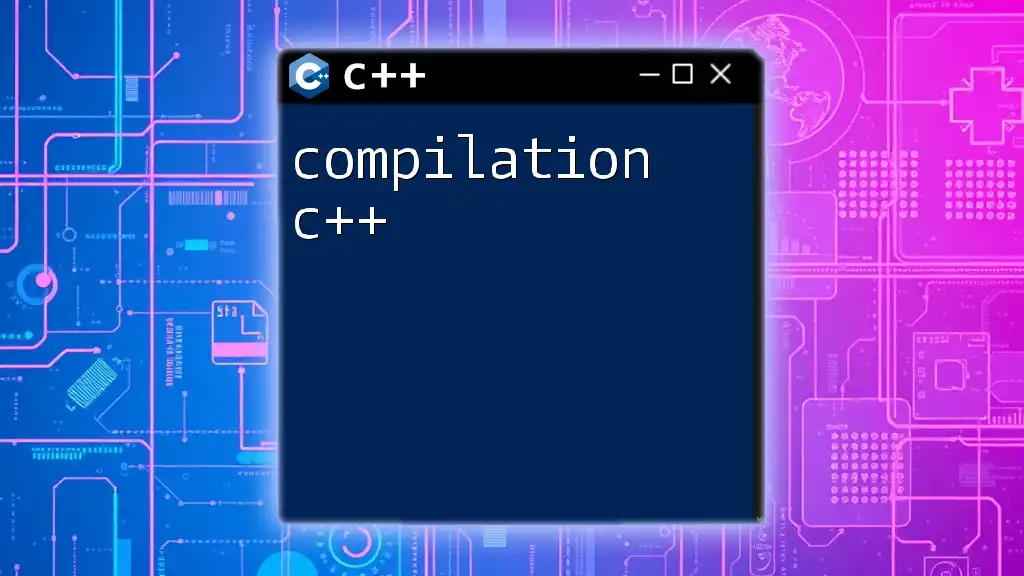
Best Practices for C++ Implementation Files
Implementing best practices in writing your implementation files can significantly affect the maintainability of your code.
Consistent Indentation and Formatting
Consistent indentation is essential not just for aesthetics but for readability as well. It helps developers understand the hierarchical structure of the code at a glance.
Commenting and Documentation
Providing comments within your code can help future developers (or even you) understand your logic when revisiting the project. Using tools like Doxygen can automate the generation of documentation, keeping it concise and up-to-date.
Testing and Debugging Your Implementation
Testing your functions is paramount for ensuring their correctness. Use unit tests and debugging tools to identify and resolve issues efficiently.
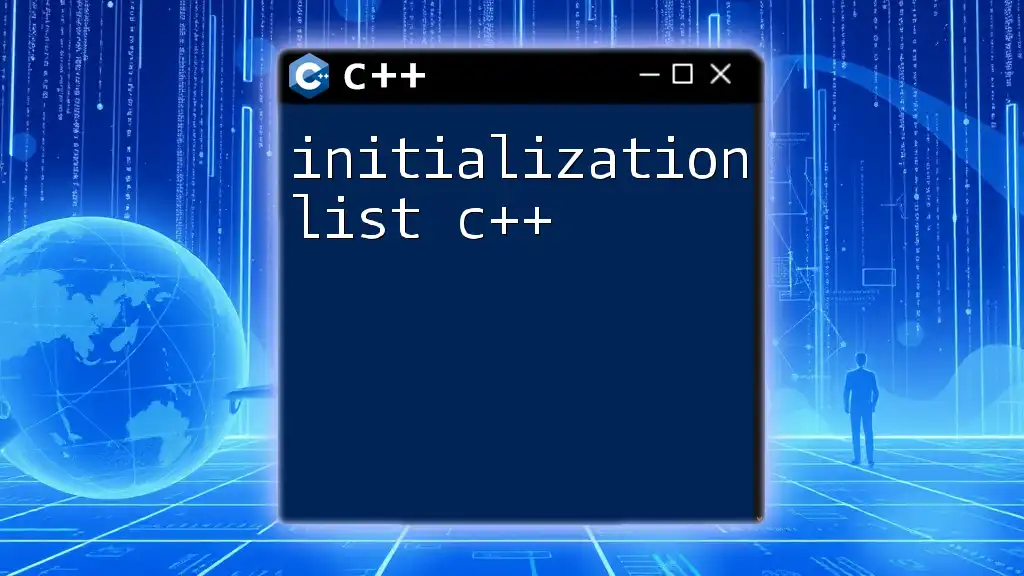
Advanced Topics in C++ Implementation Files
Once you’re comfortable with the basics, you might delve into more advanced features of C++ implementation files.
Implementing Inline Functions
Inline functions can provide performance benefits by reducing function call overhead. You declare an inline function as follows:
inline int add(int a, int b) {
return a + b;
}
These functions are typically small and are defined in the header file directly for efficiency reasons.
Using Templates in Implementation Files
Templates allow for more generic and reusable code, enabling the same function or class to work with different data types. Here's a quick example:
template <typename T>
T add(T a, T b) {
return a + b;
}
Incorporating templates expands the versatility of your C++ code significantly.
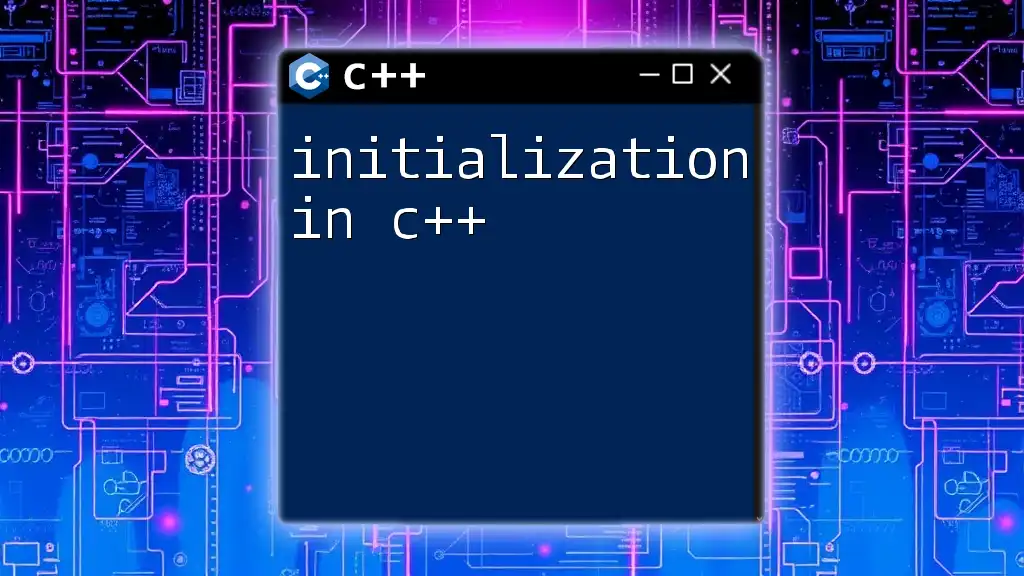
Conclusion
Having a solid understanding of what an implementation file in C++ encapsulates is crucial for any developer aiming to write clean and efficient code. By following organized structures, maintaining best practices, and avoiding common pitfalls, your implementation files can greatly enhance your overall programming efficiency.
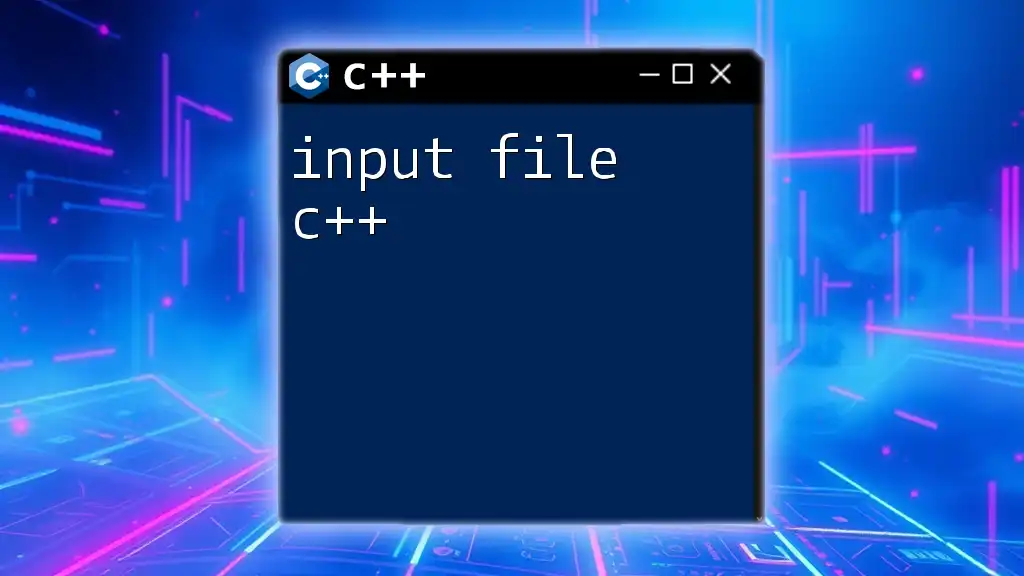
Additional Resources
To further your knowledge, seek out reputable C++ documentation and learning platforms. Engaging with these resources can provide deeper insights into the nuances of C++ implementation files and advanced coding practices, assisting you on your journey to become a proficient C++ developer.