In C++, input files can be accessed using the `ifstream` class to read data from a file efficiently.
Here's a simple code snippet demonstrating how to use an input file in C++:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("data.txt");
if (!inputFile) {
std::cerr << "Unable to open file data.txt";
return 1; // Exit if the file cannot be opened
}
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl; // Output each line from the file
}
inputFile.close(); // Close the file
return 0;
}
Understanding File Input in C++
What is File Input?
File input in C++ refers to the process of reading data from external files so that a program can manipulate or analyze that data. This concept is crucial in many applications, such as data processing, configuration settings for applications, and more. By utilizing file input, C++ programmers can handle larger datasets that reside outside of the program itself, allowing for improved data management and versatility.
Why Use File Input?
Using file input provides several advantages:
- Persistence: Data can be saved to disk, allowing programs to read and write information over time.
- Scalability: Handling large volumes of data becomes feasible without overloading memory.
- Configuration Management: Applications can read settings from configuration files, making them more flexible and easier to manage.
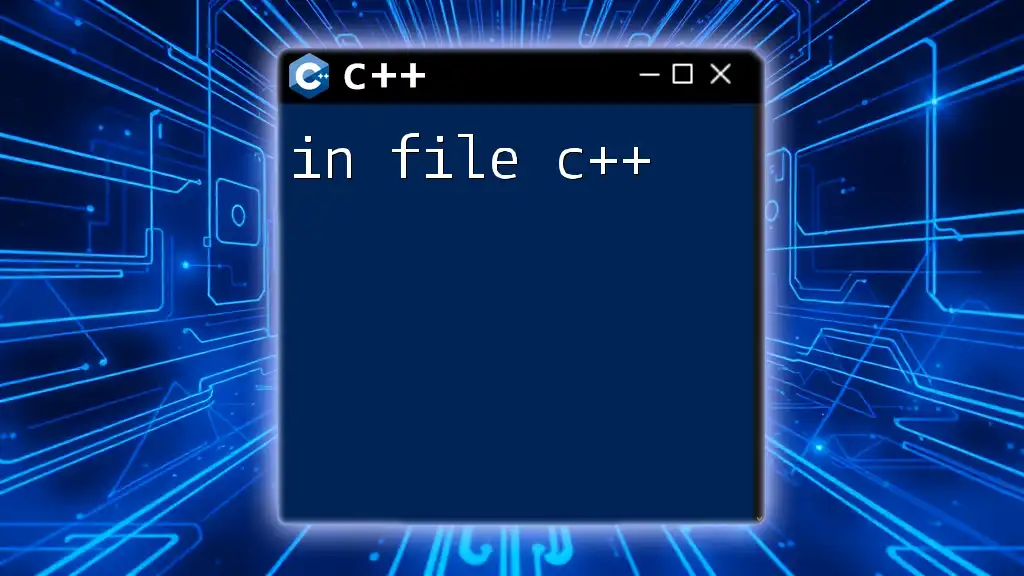
Setting Up for File Input
Including the Necessary Libraries
To work with file input in C++, you need to include the `<fstream>` library. This library provides the necessary classes for file stream operations, including reading and writing files.
#include <iostream>
#include <fstream>
Understanding File Streams
C++ employs several file stream classes, notably `ifstream`, `ofstream`, and `fstream`. Here’s a quick overview:
- ifstream: Used for reading files.
- ofstream: Used for writing to files.
- fstream: Allows both reading and writing.
Here’s a simple example showing the creation of an `ifstream` object to read from a file:
std::ifstream inputFile("example.txt");
This snippet demonstrates how to set up the file stream for input operations.
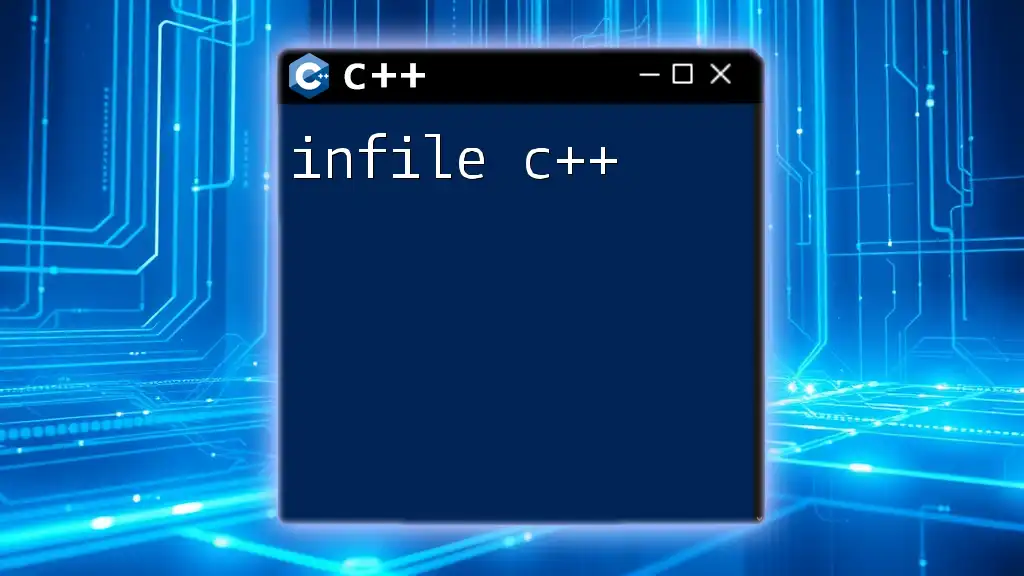
Opening a File
How to Open a File in C++
To start reading from a file, you first need to open it with an `ifstream` object. The general syntax is straightforward:
std::ifstream inputFile("filename.txt");
It’s essential to specify the correct filename while ensuring the file exists in the expected directory.
Checking for Successful Opening
After attempting to open a file, you should always check whether the operation was successful. This is important to avoid runtime errors and ensure smooth execution of your program. Here’s how you can do it:
if (!inputFile.is_open()) {
std::cerr << "Error opening file." << std::endl;
}
By checking the `is_open()` method, you can manage failure scenarios effectively.
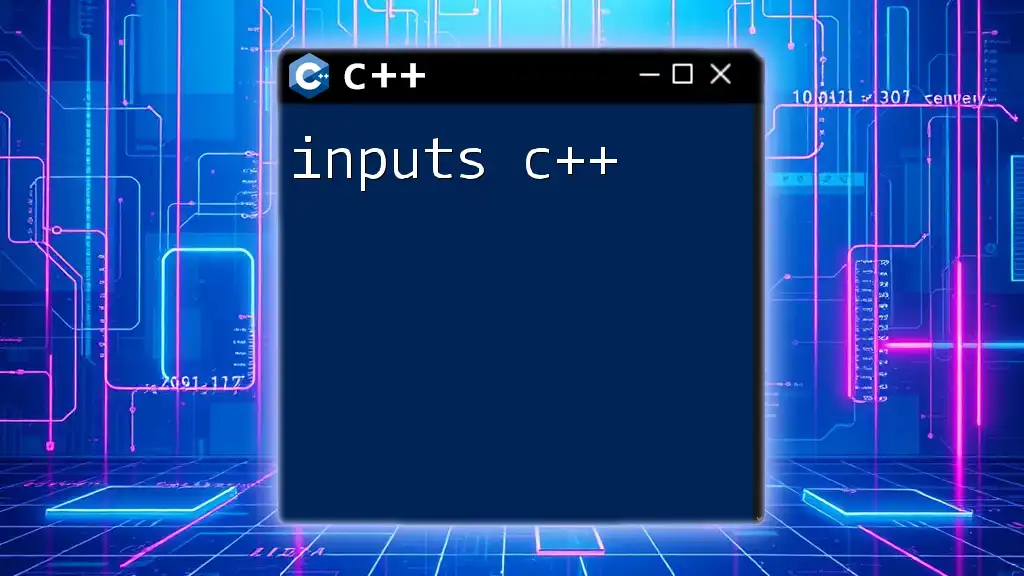
Reading Data from a File
Basic Reading Techniques
Once the file is successfully opened, you can start reading data. The basic technique in C++ for reading formatted data is using the extraction operator (`>>`). Here is a simple code snippet that reads an integer from a file:
int number;
inputFile >> number;
This operation extracts the next available integer from the file and saves it into the `number` variable.
Reading Lines of Text
For reading entire lines of text, the `std::getline()` function is a powerful alternative. This function reads all characters until a newline is encountered, making it suitable for reading lines in text files. Here’s an example:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
In this example, the program reads each line from the file until the end and prints it to the console.
Reading Multiple Data Types
In scenarios where you need to read different data types (e.g., mixing integers and strings), the extraction operator can handle this gracefully. Consider the following scenario where a file contains a name followed by an age:
std::string name;
int age;
inputFile >> name >> age;
std::cout << "Name: " << name << ", Age: " << age << std::endl;
This snippet reads a string followed by an integer, allowing for easy handling of structured data.
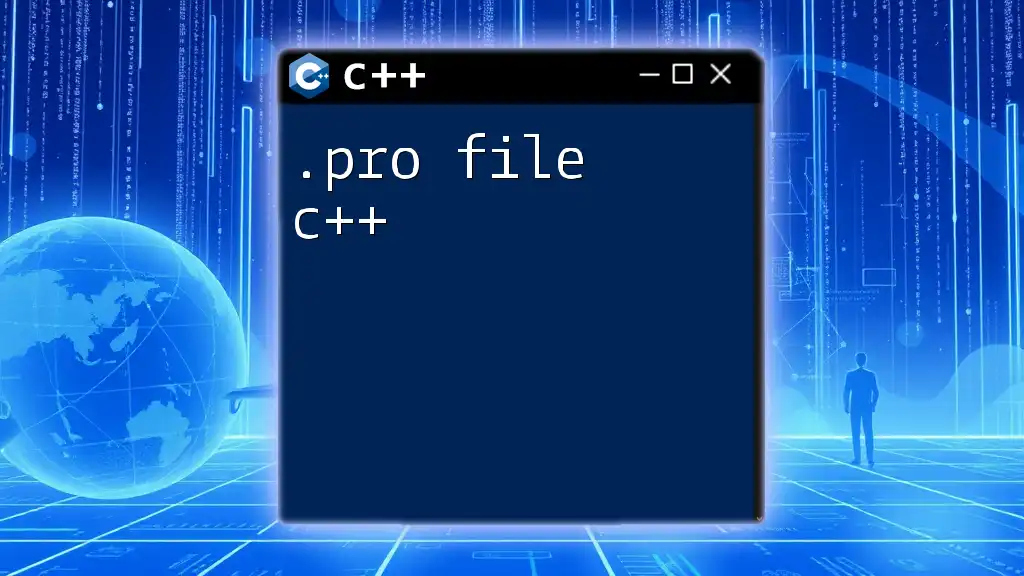
Closing the File
Importance of Closing Files
Closing files is a critical step in file handling. It frees associated resources and ensures that all buffered data is written properly. To close an open file, you simply call the `close()` method on the file stream object:
inputFile.close();
Neglecting to close files can lead to memory leaks or data corruption, particularly in long-running applications.
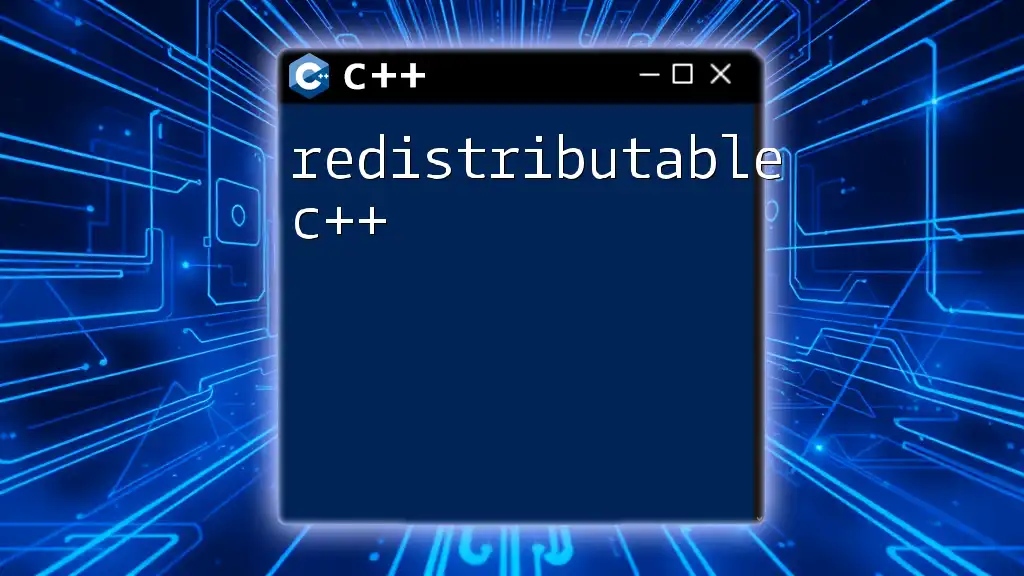
Error Handling and Exception Safety
Handling File Input Errors
Throughout file operations, it's common to encounter errors such as files that do not exist or are inaccessible. To handle these gracefully, you can check the state of the stream after reading:
if (!inputFile) {
throw std::runtime_error("Error reading the file.");
}
Implementing checks like this enhances the reliability and safety of your code.
Using `std::ifstream` with Exception Handling
To further improve robustness, using exception handling is advisable. By wrapping your file operations in a try-catch block, you can manage unexpected situations in a more controlled manner:
try {
std::ifstream inputFile("example.txt");
if (!inputFile) throw std::runtime_error("File not found");
// ... read data
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
This approach allows your program to respond appropriately to errors rather than crashing unexpectedly.
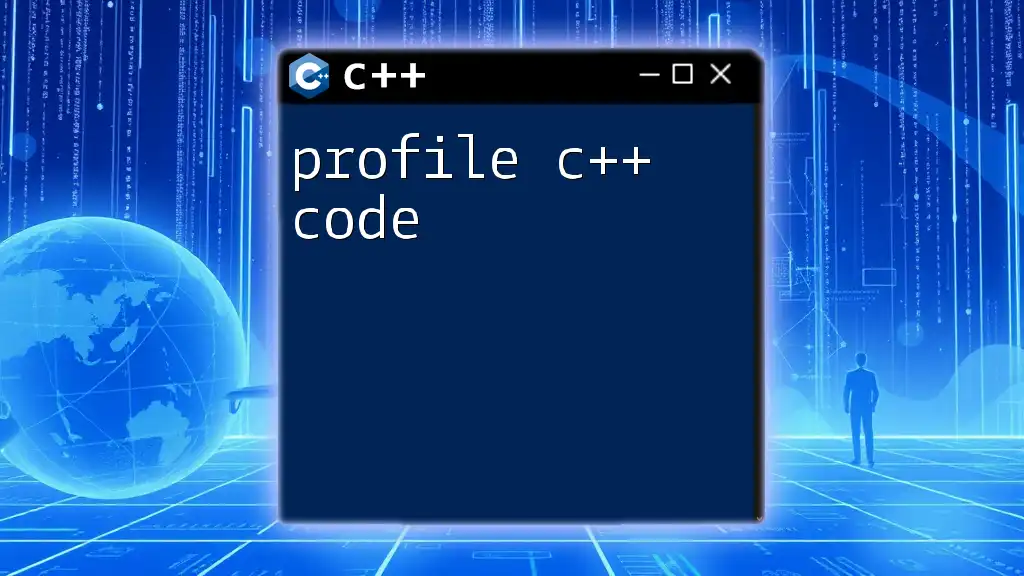
Real-world Applications of File Input in C++
Data Processing Examples
File input is heavily utilized in data processing applications, such as reading data sets from CSV files or logs. This capability allows programs to analyze data efficiently. For instance, consider a program that reads and processes numerical data from a log file, summing up entries to generate statistics.
Configuration Files
Configuration files are another essential application of file input. Applications often use these files to specify parameters that can change the behavior of the program. Here’s a simple example that reads configuration settings:
std::string configKey;
std::string configValue;
while (inputFile >> configKey >> configValue) {
std::cout << configKey << ": " << configValue << std::endl;
}
In this snippet, key-value settings can easily be processed, allowing for dynamic application behavior based on external settings.
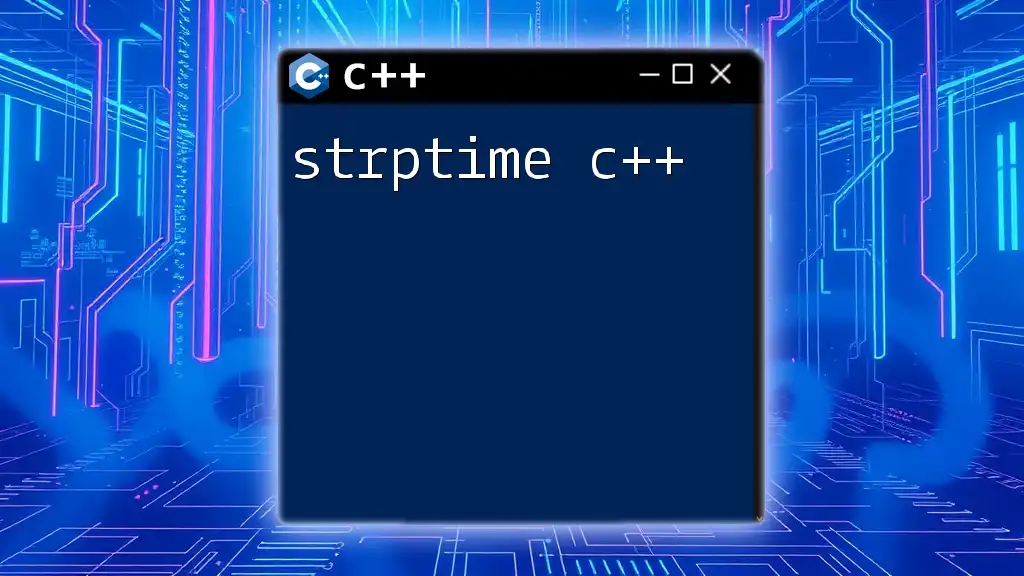
Conclusion
In summary, mastering file input in C++ is vital for any aspiring programmer. Understanding how to read data from files not only enhances your programming capabilities but also opens up numerous possibilities for creating rich applications. The knowledge you've gained in this article equips you with the foundational skills necessary for handling file input effectively. As you continue your journey in programming, remember to practice these techniques and explore further resources to deepen your understanding of file handling in C++.
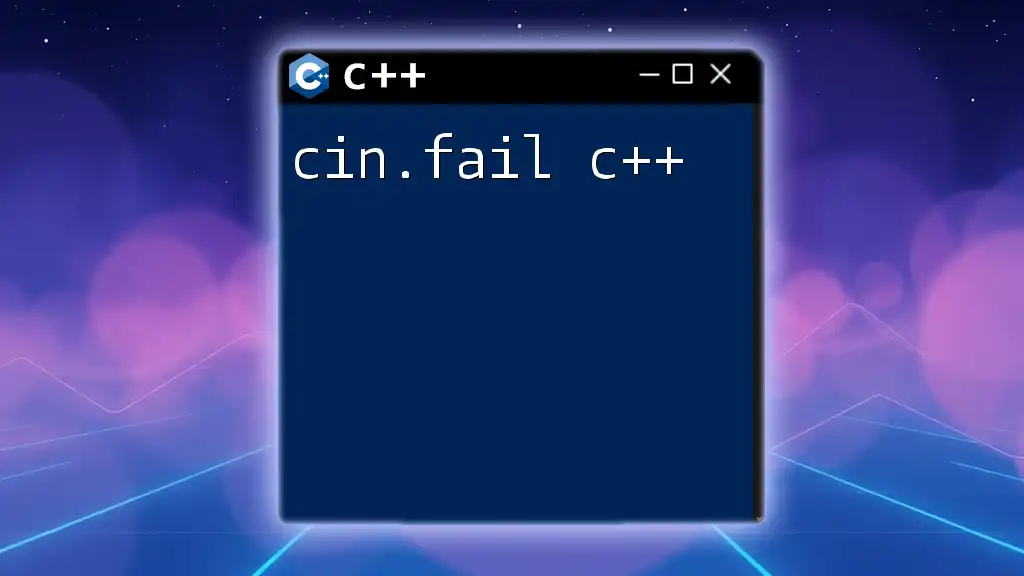
Additional Resources
For a better grasp of file handling in C++, consider exploring the following resources:
- C++ Official Documentation
- Recommended programming books that focus on C++
- Online forums where fellow programmers discuss and troubleshoot real-world coding issues
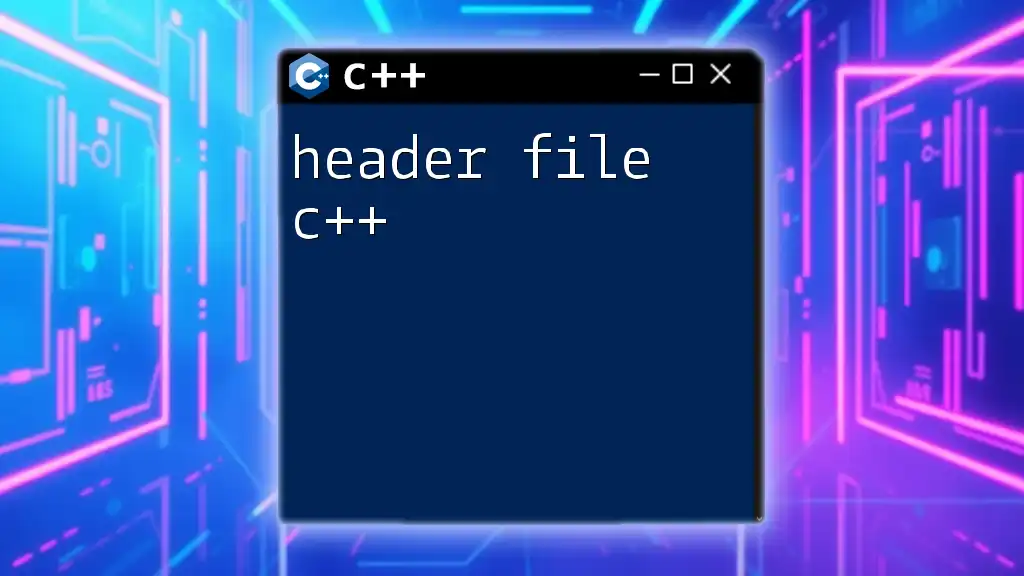
Frequently Asked Questions (FAQs)
What file formats can I read in C++?
C++ can handle a variety of text-based file formats, including CSV, TXT, and JSON. Using proper parsing techniques, you can read structured data effectively.
Is file input in C++ safe?
When implemented with proper error handling and checks, file input in C++ is safe. Always ensure to validate file existence and check stream states.
How can I improve performance when reading large files?
To enhance performance, consider using buffered reading techniques or reading data in chunks, which can significantly reduce I/O operation overhead.