The `random_shuffle` function in C++ randomly rearranges the elements in a given range, producing a new order of the elements. Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
#include <ctime>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::srand(static_cast<unsigned int>(std::time(nullptr))); // Seed for random number generation
std::random_shuffle(vec.begin(), vec.end());
for(int num : vec) {
std::cout << num << " ";
}
return 0;
}
Understanding `random_shuffle`
`random_shuffle` is a function in C++ that provides a straightforward way to randomly rearrange elements in a sequence, such as an array or a vector. The importance of randomization in algorithms cannot be overstated; it plays a critical role in various applications, including simulations, games, and randomized algorithms which provide robustness and security against predictable patterns.
How `random_shuffle` Works
The `random_shuffle` function is part of the `<algorithm>` library and operates on iterators. It takes a range defined by two iterators and rearranges the elements in that range in a random order. The shuffling process typically follows a mechanism that ensures each permutation of the sequence is equally likely.
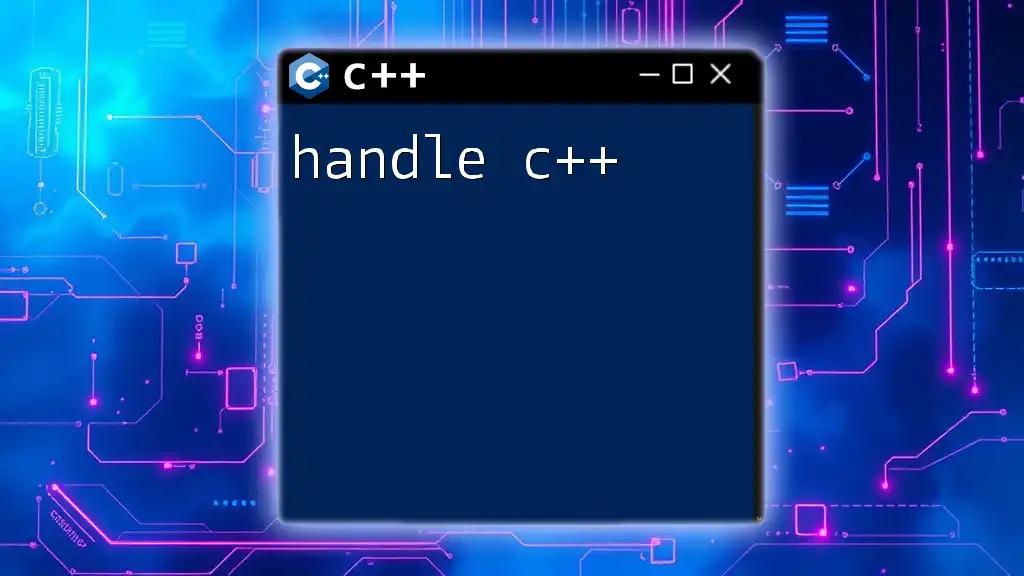
Setting Up Your C++ Environment
Before using `random_shuffle`, you need to ensure your C++ environment is properly set up.
Installing a C++ Compiler
You can use various compilers such as GCC, Clang, or MSVC depending on your operating system. Here are some quick steps to get started:
- For Windows users, consider downloading MinGW or Visual Studio.
- For macOS users, Xcode provides a straightforward solution.
- Linux users typically have access to GCC through package managers like `apt` or `yum`.
Including Necessary Headers
To utilize `random_shuffle`, you will need to include specific libraries in your C++ code. The most essential include statements are:
#include <iostream>
#include <vector>
#include <algorithm>
#include <ctime>
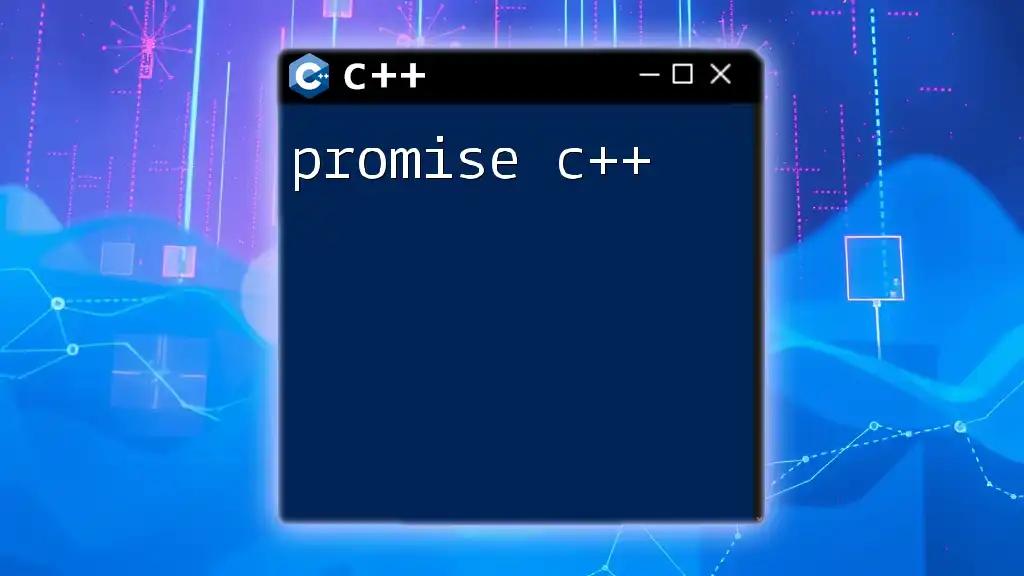
The Syntax of `random_shuffle`
Function Prototype
The syntax for `random_shuffle` is as follows:
template<class RandomIt>
void random_shuffle(RandomIt first, RandomIt last);
This prototype highlights that `random_shuffle` is a template function, allowing it to work with various data types as long as they support iterators.
Parameters Explained
- first: This is the starting iterator of the range you want to shuffle.
- last: This is the ending iterator, which is one-past the last element in the range.

Using `random_shuffle` in Practice
Basic Example
Let’s dive into a simple demonstration to see how `random_shuffle` works in practice.
#include <iostream>
#include <vector>
#include <algorithm>
#include <ctime>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::random_shuffle(numbers.begin(), numbers.end());
std::cout << "Shuffled Numbers: ";
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this example, after including the necessary headers and using `random_shuffle`, you will see the output displaying the numbers in a random order. Each time you run the program, you may get different arrangements, showcasing the randomization effect.
Advanced Example with Custom Random Function
While the basic `random_shuffle` implementation is robust, you can enhance the shuffling process by using a custom random number generator. Here’s how you can do this using the `<random>` library:
#include <iostream>
#include <vector>
#include <algorithm>
#include <random>
#include <ctime>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::default_random_engine engine(std::time(0)); // Seed with current time
std::shuffle(numbers.begin(), numbers.end(), engine);
std::cout << "Shuffled Numbers with custom engine: ";
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this example, we leverage `std::default_random_engine`, seeded with the current time, to randomly shuffle the numbers. This increases the randomness and provides better distribution among the permutations.

Performance Considerations
Time Complexity of `random_shuffle`
The time complexity of `random_shuffle` is typically linear, O(n), where n is the number of elements in the range being shuffled. While efficient in most use cases, understanding how it compares to other shuffling techniques is crucial for performance-critical applications.
Potential Drawbacks of Using `random_shuffle`
It is worth noting that `random_shuffle` has been deprecated in C++14 and removed in C++17. As an alternative, you should use `std::shuffle`, which offers better randomization properties and is generally preferred in modern C++ programming.

Best Practices
When to Use `random_shuffle`
While `random_shuffle` is simple, it is vital to know when shuffling is required. Typical scenarios include:
- Games and simulations where randomness affects outcomes
- Random sampling techniques in statistical applications
- Any situation where you want to avoid predictable patterns
Ensuring Fairness in Shuffling
To ensure a fair and unbiased shuffle, always consider using a good random number generator. Avoid seeding with constant values as this will result in predictable outputs. Instead, utilize seeding methods such as `std::time(0)` or a high-resolution clock for better randomness.
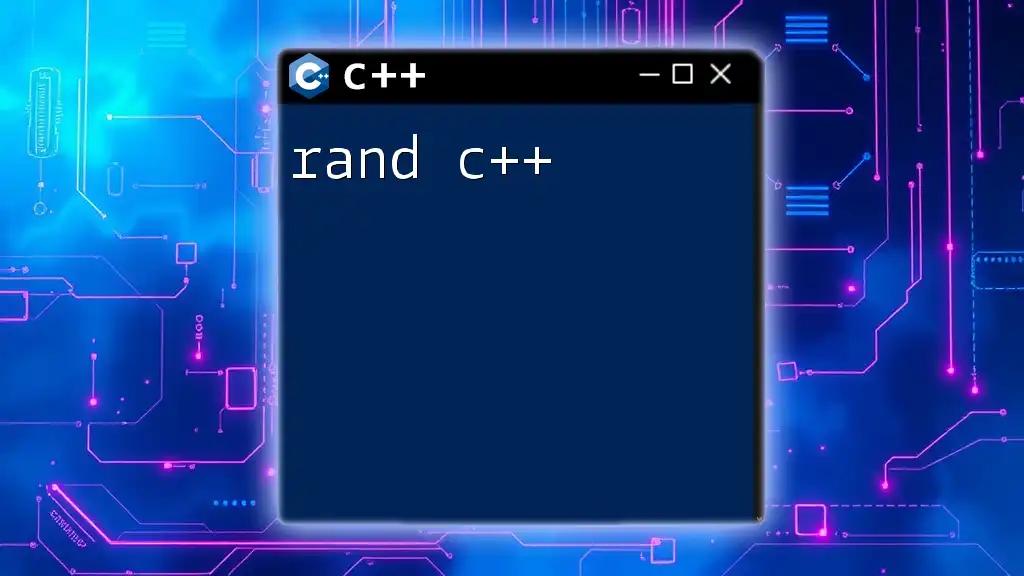
Conclusion
In summary, `random_shuffle c++` serves as a powerful tool for developers looking to randomize data sequences. While it has historically been a go-to function, developers should transition to `std::shuffle` for better randomization and support in modern C++ standards. As you practice using these functions, you will develop a richer understanding of randomness and its applications in programming.
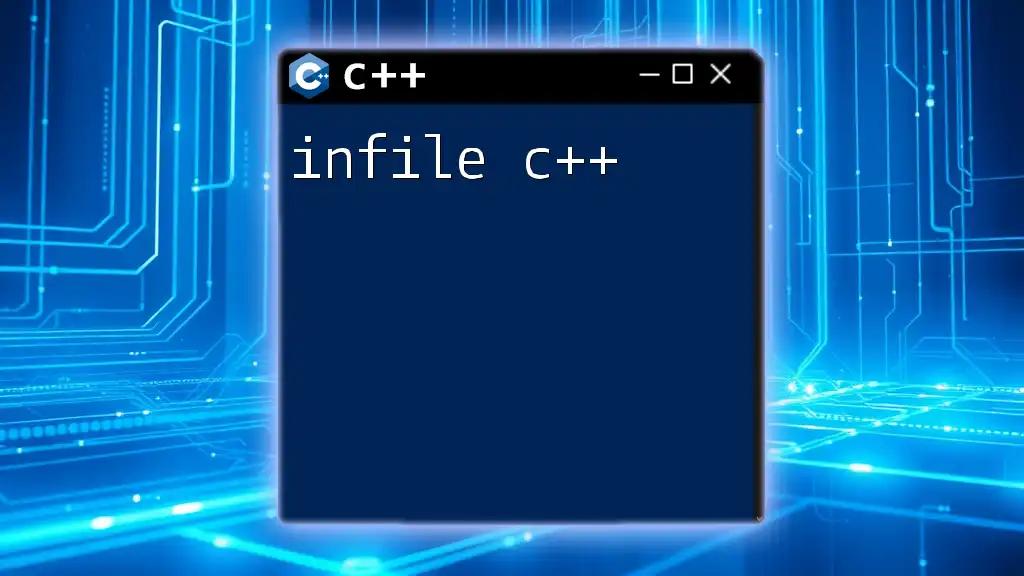
Additional Resources
Further Reading
For those eager to expand their knowledge, check out the official [C++ documentation](https://en.cppreference.com/w/cpp/algorithm/random_shuffle) and explore comprehensive resources such as relevant books and online courses centered around C++ programming. Engaging with communities and forums dedicated to C++ can also enhance your learning experience and offer valuable insights from fellow developers.