The "handshake" in C++ typically refers to a synchronized communication process between two systems or threads, often used to establish a connection or confirm readiness.
Here’s a simple example of a handshake mechanism using condition variables in C++:
#include <iostream>
#include <thread>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool ready = false;
void worker() {
std::this_thread::sleep_for(std::chrono::seconds(1)); // Simulate work
std::lock_guard<std::mutex> lock(mtx);
ready = true;
cv.notify_one(); // Notify the main thread
}
int main() {
std::thread t(worker);
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return ready; }); // Wait for the worker to signal readiness
std::cout << "Handshake complete!" << std::endl;
t.join();
return 0;
}
What is Handshake C++?
Handshake C++ refers to the protocols and methods in C++ used to establish secure connections over a network. It plays a crucial role in ensuring that both the client and the server are synchronized and agree on the parameters of communication before actual data transfer takes place. This is particularly important in scenarios where security and data integrity are essential.
Features of Handshake C++
The primary features of Handshake C++ include:
-
Lightweight Efficiency: Handshake C++ is designed to be resource-efficient, which is important for applications needing to handle a high volume of requests without overloading the system.
-
Asynchronous Communication Capabilities: It supports asynchronous operations, allowing applications to handle multiple connections simultaneously without blocking, which is ideal for real-time systems.
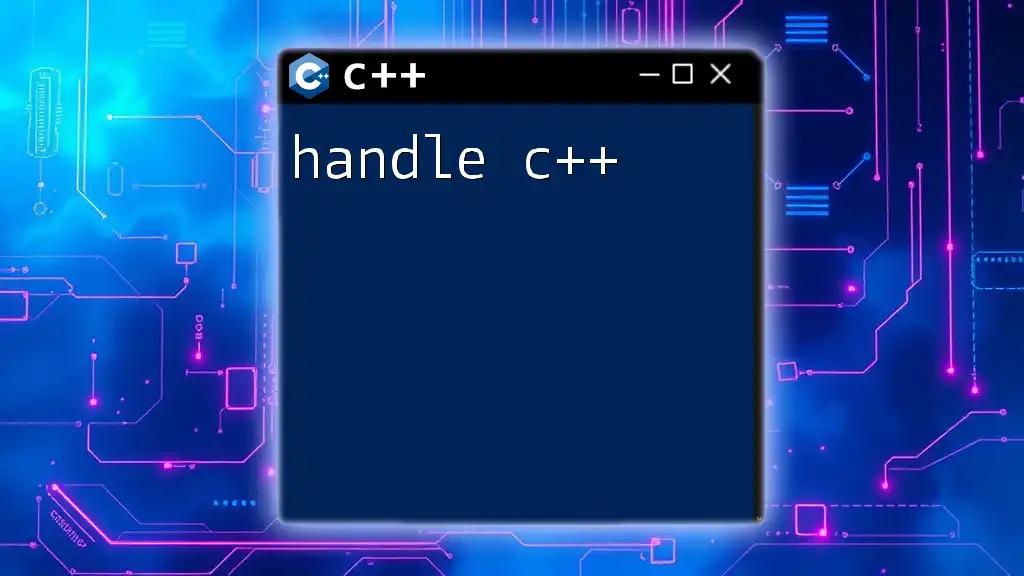
Setting Up Your Development Environment
To start using Handshake C++, you need to ensure that your development environment is properly configured.
Requirements for Handshake C++
Before diving into Handshake C++, you need a compatible C++ compiler such as GCC, Clang, or MSVC. Additionally, there may be specific library dependencies required to utilize Handshake functionalities.
Installing Handshake C++
The installation process can vary based on your operating system and preferred package manager. Here’s a basic step-by-step guide using a popular package manager like `vcpkg`:
- Install vcpkg: Follow the official documentation to get `vcpkg` set up on your system.
- Install Handshake Library: Use the following command to install the library:
vcpkg install handshake-cpp
- Include in Your Project: Ensure your project configuration links to the installed library.
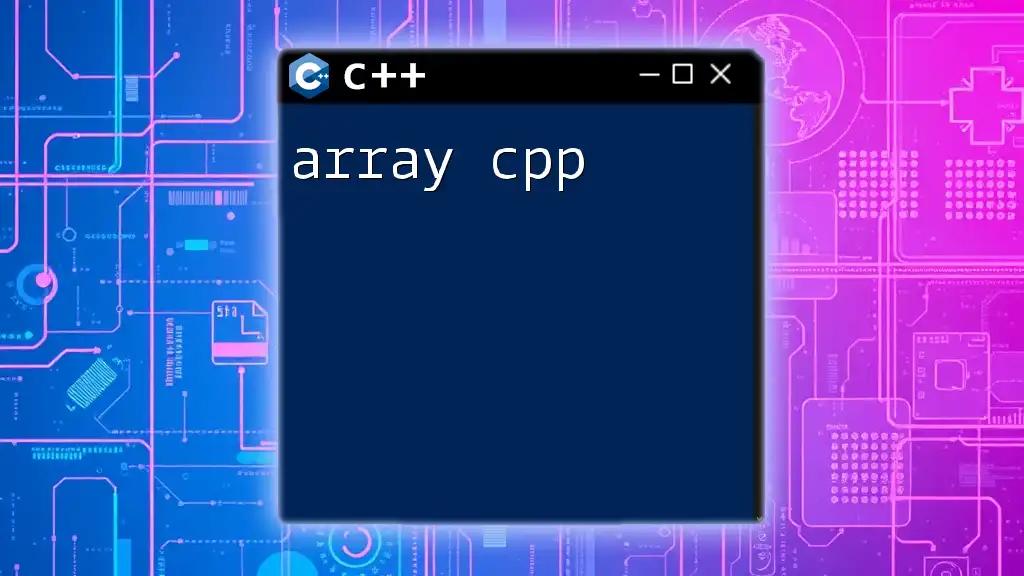
Core Concepts of Handshake C++
Understanding the core concepts behind Handshake C++ is essential to implementing it effectively.
Understanding Handshake Process
A typical handshake process involves a series of steps that must occur for a secure connection to be established. When a client wishes to connect to a server, they follow a sequence that may include initiating a request, the server responding, and both parties agreeing on communication parameters, including encryption methods and session keys.
Key Terminology
Familiarizing yourself with key terms is crucial:
- Client: The entity that initiates a connection request.
- Server: The entity that listens for connection requests and responds.
- Session: The established communication link between the client and server.
- Cipher Suites: A set of algorithms that help secure network connections.
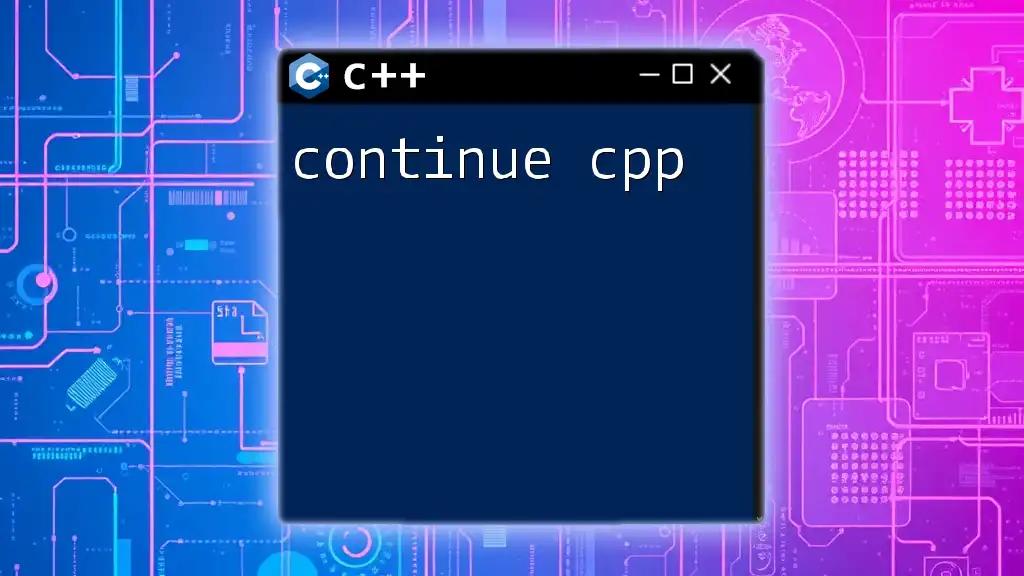
Implementing Handshake C++
Now that you have an understanding of the basic concepts, let’s dive into the implementation of Handshake C++.
Basic Structure of Handshake Code
An effective implementation starts with organizing your code clearly. The structure should reflect the lifecycle of a handshake process.
Creating a Basic Handshake Example
Here’s a simple example of implementing a basic handshake mechanism:
Client-Side Handshake Code Snippet:
// Example: Client Side Handshake
void initiateHandshake() {
std::cout << "Initiating Handshake..." << std::endl;
// Additional code to send handshake request to server
}
Server-Side Handshake Code Snippet:
// Example: Server Side Handshake
void respondToHandshake() {
std::cout << "Responding to Handshake..." << std::endl;
// Additional code to respond with handshake confirmation
}
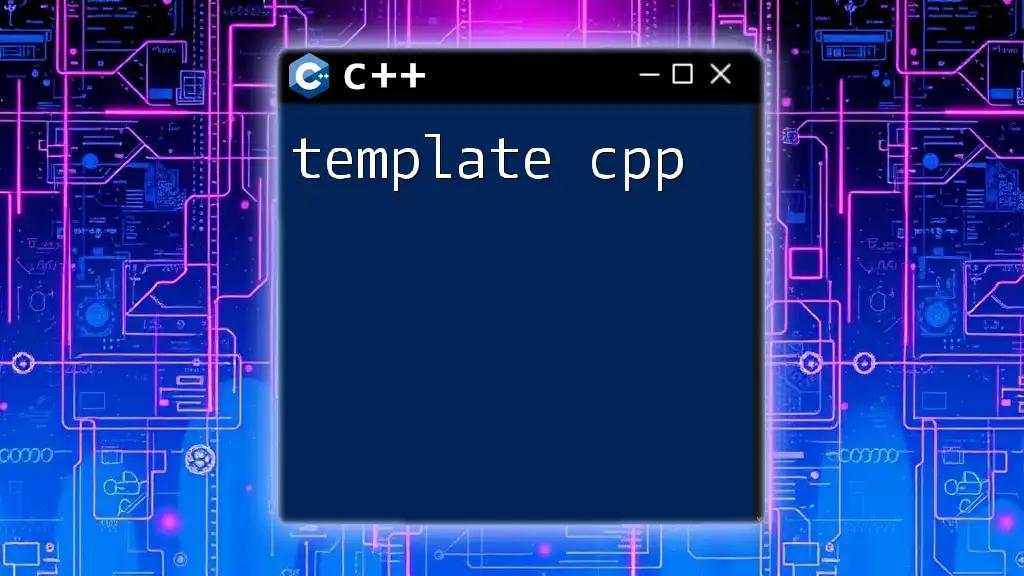
Handling Connections
Creating a robust Handshake C++ experience requires managing connections effectively.
Creating a Handshake Class
A well-defined Handshake class can encapsulate the functionality and state management needed for a handshake. Here’s a simple class implementation:
class Handshake {
public:
void initiate();
void respond();
private:
// Private member variables can hold session details
};
Establishing Connection
After defining the class, it’s vital to implement methods that establish and verify connections between the client and the server. Using member functions like `initiate()` and `respond()`, you can encompass the handshake logic comprehensively.
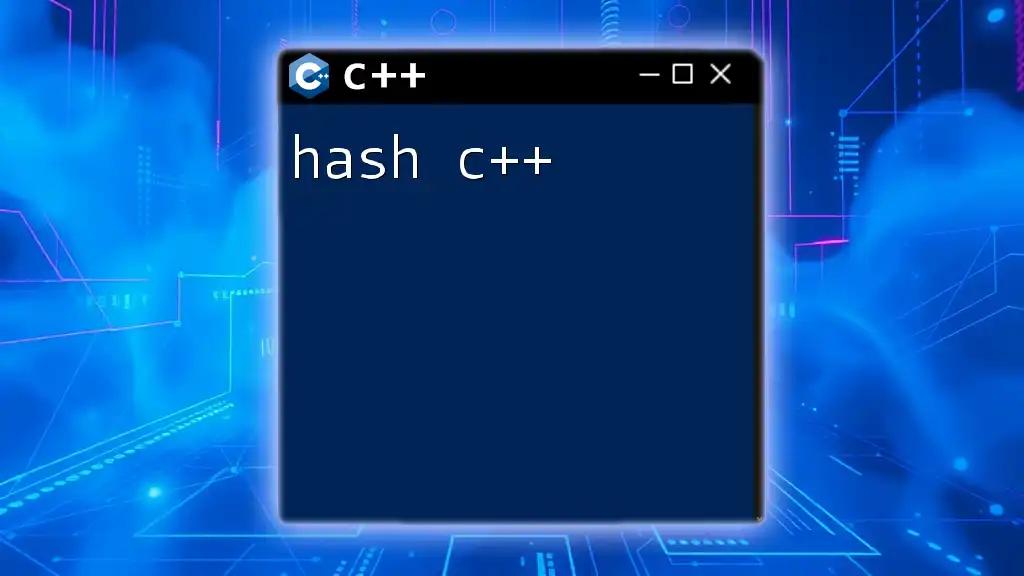
Error Handling in Handshake C++
Errors are inevitable in any network protocol implementation, and it's crucial to handle them gracefully.
Common Errors and Solutions
Some common errors you might encounter include:
- Timeout Issues: If either the client or server takes too long to execute a handshake, implement a retry mechanism with exponential backoff.
- Invalid Handshake Response: Ensure proper validation of responses to handle unexpected behavior.
Implementing Error Handling Strategies
Utilizing try-catch blocks can help manage errors during the handshake process. For example:
try {
initiateHandshake();
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}

Best Practices for Using Handshake C++
Implementing Handshake C++ efficiently requires adherence to certain best practices.
Security Considerations
Ensure your handshake procedures are secure. Use robust encryption methods and regularly update Cipher Suites to defend against vulnerabilities.
Optimizing Performance
Reducing latency is crucial for efficient communication. Leveraging asynchronous programming can enhance performance, allowing simultaneous connection handling without blocking. Implementing non-blocking sockets can facilitate responsive applications.
Here’s an example of asynchronous communication:
async void startAsyncHandshake() {
// Code to initiate handshake without blocking main thread
}
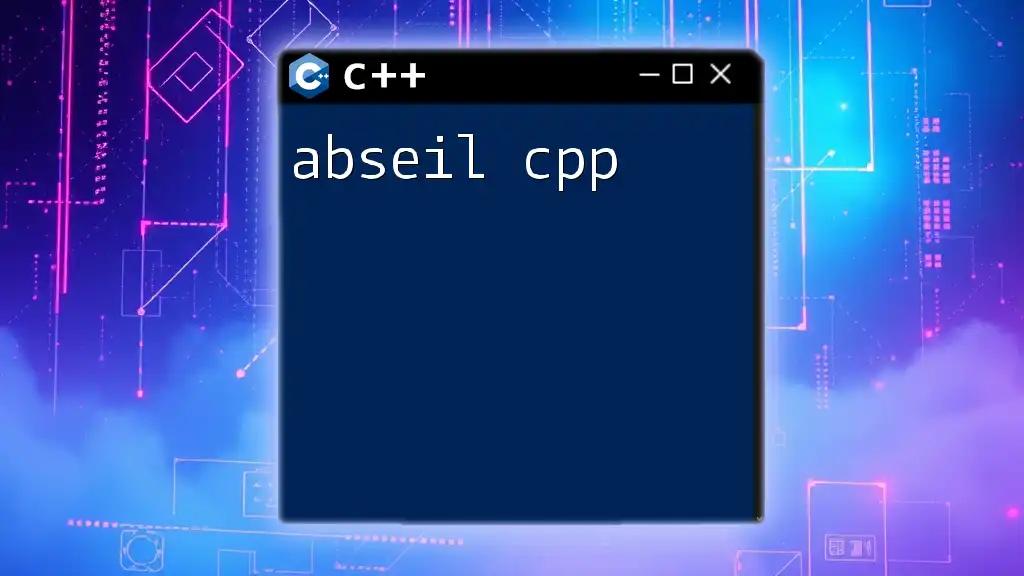
Real-World Applications
Understanding real-world applications can help solidify your knowledge.
Use Cases of Handshake C++
Handshake C++ finds its applications in various fields, including:
- IoT devices: Where numerous small devices need to connect securely.
- Online gaming: For ensuring seamless player interactions.
- Real-time communication apps: Like video conferencing tools that require low latency and secure connections.
Case Study Example
Consider a project where a gaming server employs Handshake C++ to manage user connections and ensure data integrity through established secure channels. This leads to smoother gameplay and enhanced security.
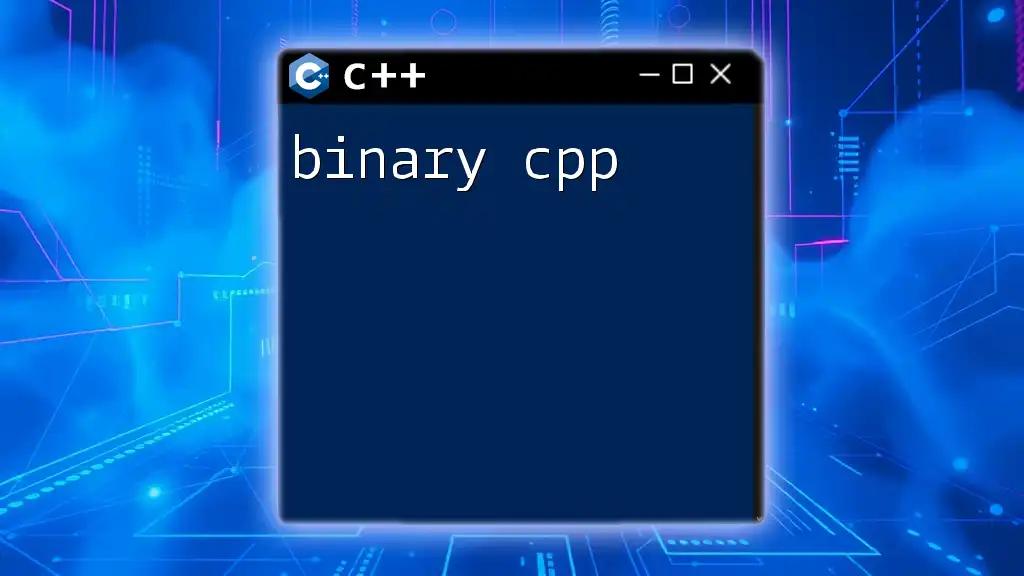
Testing Your Handshake Implementation
Testing is a vital phase in any development process to ensure reliability.
Setting Up a Test Environment
Utilizing frameworks like Google Test can streamline the testing of your Handshake C++ code by providing an infrastructure for writing and running tests.
Sample Test Cases
Implementing sample test cases for handshake functionality can validate that both initiation and response methods work as intended. Investigate expected and edge cases to improve robustness.
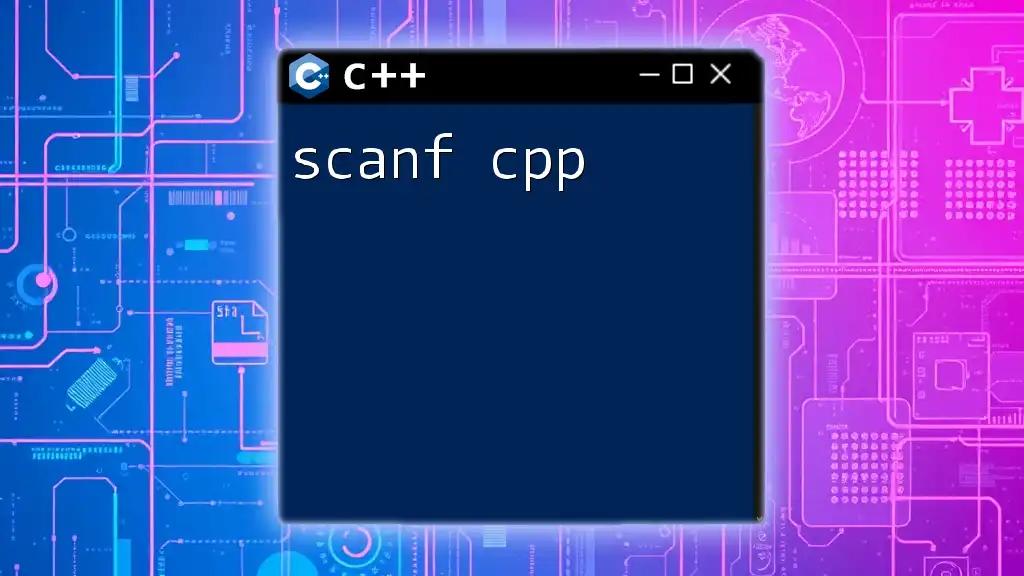
Conclusion
In summary, Handshake C++ is fundamental for establishing secure and efficient network connections. Understanding its core concepts, implementation strategies, error handling, and best practices allows developers to harness this powerful protocol effectively. As you advance, consider exploring more about asynchronous programming and security enhancements to elevate your projects even further. Remember, the journey of mastering C++ and its networking capabilities is one of continuous learning and application.