C is a powerful general-purpose programming language, while C++ is an extension of C that incorporates object-oriented features, allowing for more complex and efficient code development.
Here’s a simple C++ code snippet demonstrating the basic syntax of a "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C: The Core Language
What is C?
C is a general-purpose programming language that has had a significant impact on the modern computing landscape. Developed in the early 1970s, C is renowned for its efficiency and control over system resources, which makes it an excellent choice for developing operating systems, embedded systems, and real-time applications.
Key features of C include:
- Low-level access to memory, enabling close interactions with hardware.
- Efficient performance, which is crucial in performance-critical applications.
- Portability, allowing C programs to run on various platforms with minimal modifications due to its standardization by ANSI.
C Syntax Basics
The structure of a C program is straightforward, often beginning with header files and a main function. Here's an example of a simple C program that prints "Hello, World!" to the console:
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
This example shows the fundamental aspects of C programming, including how to include libraries and define the entry point of a program.
Data Types in C
Understanding data types is crucial when programming in C.
Primitive Data Types:
- `int`: Represents integers (e.g., `int age = 30;`)
- `char`: Represents single characters (e.g., `char letter = 'A';`)
- `float`: For floating-point numbers (e.g., `float temperature = 23.5;`)
- `double`: A double-precision floating-point (e.g., `double price = 29.99;`)
Derived Data Types: C also allows the creation of derived types, including:
- Arrays: A collection of elements of the same type.
int numbers[5] = {1, 2, 3, 4, 5}; // Example of an array
- Pointers: Variables that store memory addresses, enabling dynamic memory allocation and manipulation.
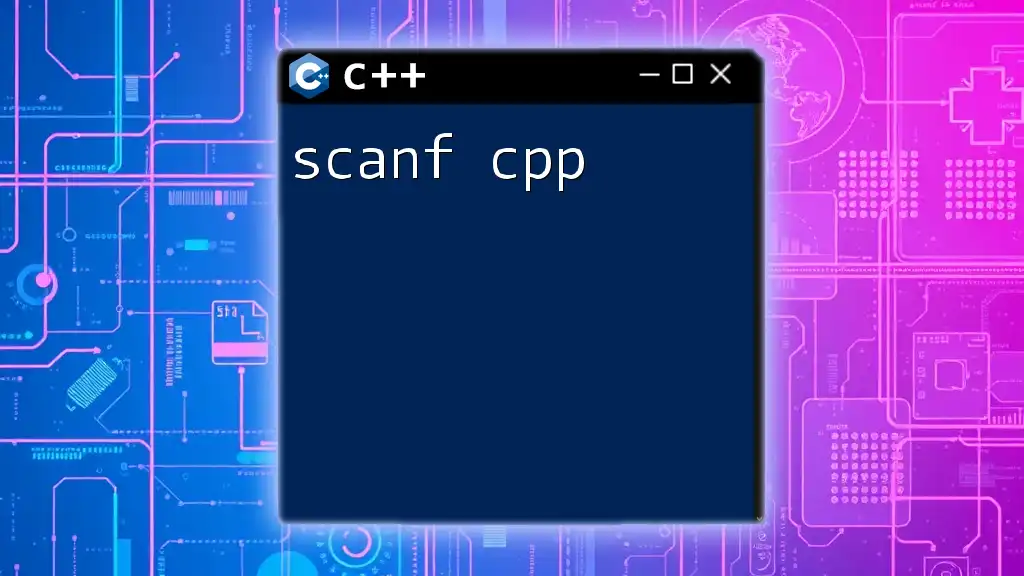
Control Flow in C
Conditional Statements
Conditional statements like `if`, `else if`, and `else` facilitate control flow in C programs. Here's an example demonstrating their use:
int x = 10;
int y = 20;
if (x > y) {
printf("x is greater");
} else {
printf("y is greater");
}
This code evaluates the conditions and executes the appropriate block based on whether `x` or `y` is greater.
Loops
Loops are fundamental in allowing repetition of code. The most common loops in C are `for`, `while`, and `do-while`. Here’s how a `for` loop works:
for (int i = 0; i < 5; i++) {
printf("%d ", i);
}
This loop will print the numbers 0 through 4, illustrating how loops can simplify repetitive tasks.
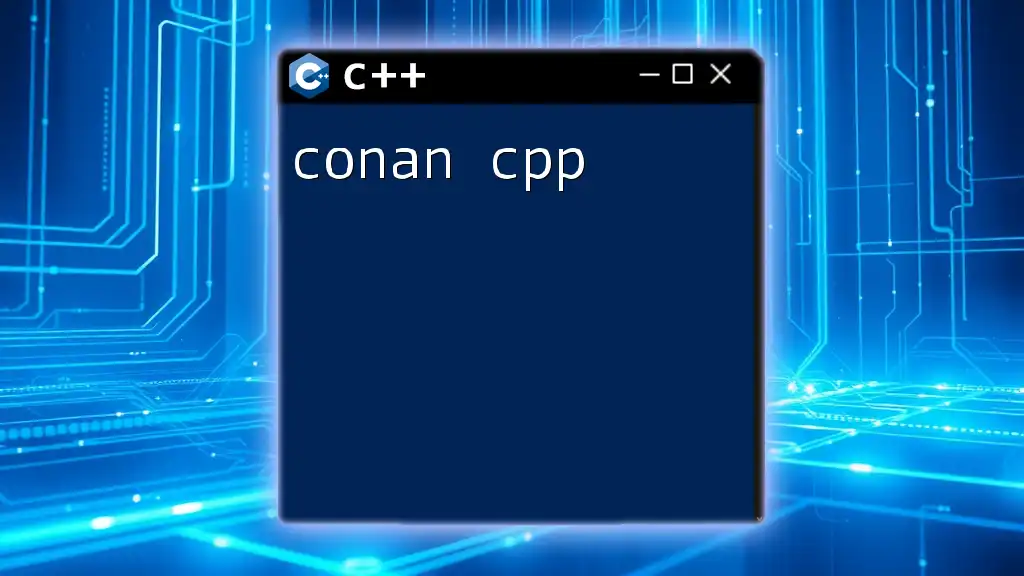
Functions in C
Defining and Calling Functions
Functions in C allow for code reuse and better organization. A simple function that prints a greeting might look like this:
void greet() {
printf("Hello!");
}
To use this function, you simply call `greet();` from your `main` function, enabling modular programming.
Scope and Lifetime
Understanding variable scope and lifetime is essential. Local variables are accessible only within the block they are defined, while global variables are available throughout the program. This distinction is crucial for managing data effectively and avoiding conflicts in larger programs.
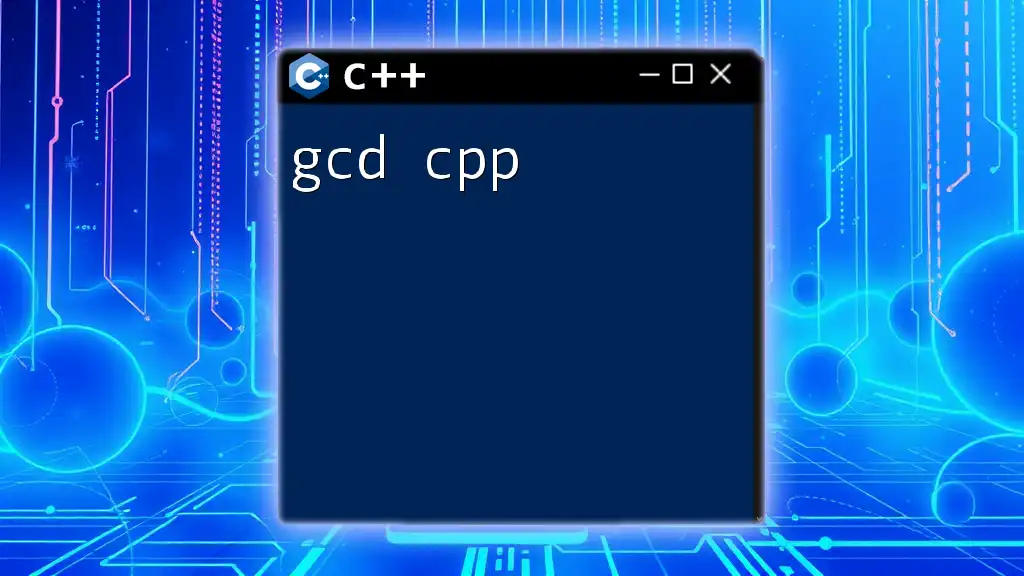
Introduction to C++
What is C++?
C++ is an extension of C that incorporates features of object-oriented programming (OOP), making it possible to create complex systems more intuitively. Developed in the early 1980s, C++ retains the efficiency of C while enabling greater abstraction.
Differences and Similarities Between C and C++: While C focuses on procedural programming, C++ supports multiple programming paradigms, allowing developers to leverage OOP principles such as encapsulation, inheritance, and polymorphism. Despite these differences, many syntax and structures are consistent between the two languages.
Key Features of C++
One of the standout features of C++ is its support for object-oriented programming. Classes and objects provide a way to model real-world phenomena. For instance, you can define a class called `Car` which encapsulates properties and behaviors related to cars.
Additionally, C++ features the Standard Template Library (STL), which provides a rich set of template classes for common data structures and algorithms.
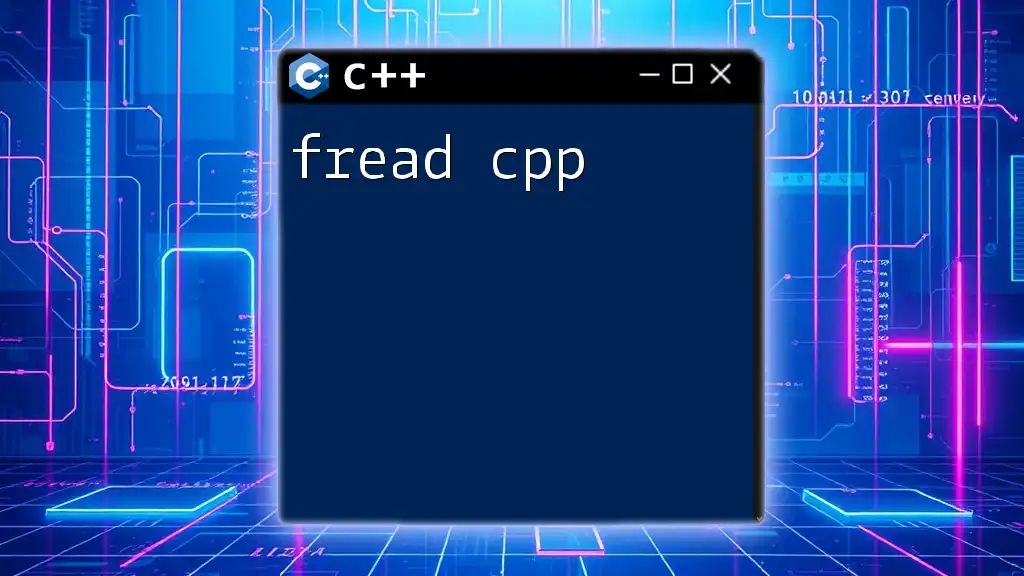
C++ Syntax Basics
Structure of a C++ Program
Like C, a C++ program begins with header files and contains a main function. Here’s a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
This example demonstrates how C++ uses `iostream` for input and output operations, showcasing a more modern approach to string handling compared to C.
Data Types in C++
C++ expands its data types to include higher-level constructs like `string` and `vector`, which facilitate more complex data handling:
#include <vector>
#include <string>
std::string name = "Alice";
std::vector<int> scores = {95, 88, 76};
This allows for easier manipulation of collections and strings, enhancing the language's versatility.
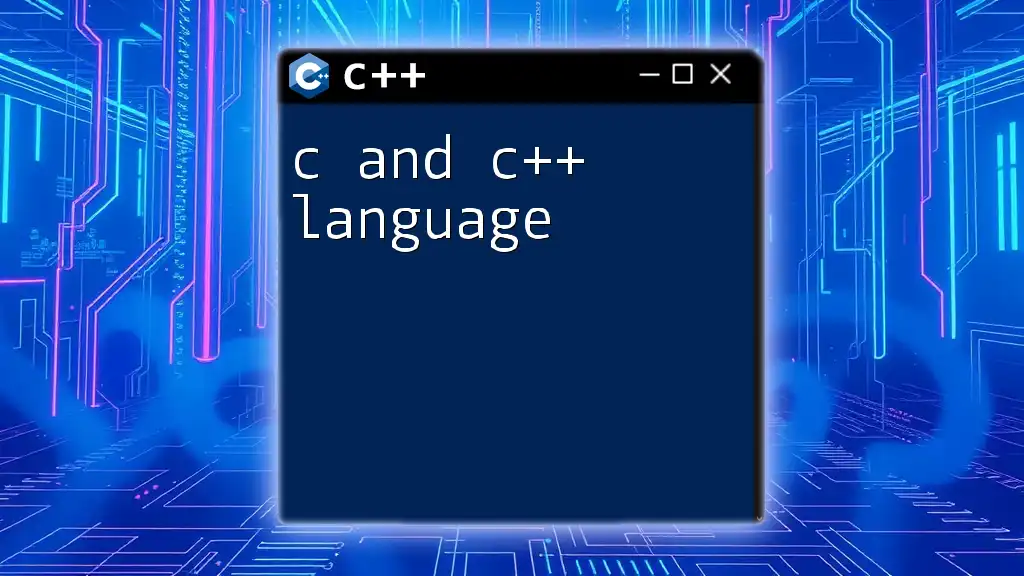
Control Flow in C++
Conditional Statements
Conditional programming flows in C++ mirror C's structures, providing intuitive syntax for decision-making. Consider this C++ version:
int x = 10;
int y = 20;
if (x > y) {
std::cout << "x is greater";
} else {
std::cout << "y is greater";
}
Loops
Just like in C, C++ supports `for`, `while`, and `do-while` loops. Here's an example of a `while` loop:
int i = 0;
while (i < 5) {
std::cout << i << " ";
i++;
}
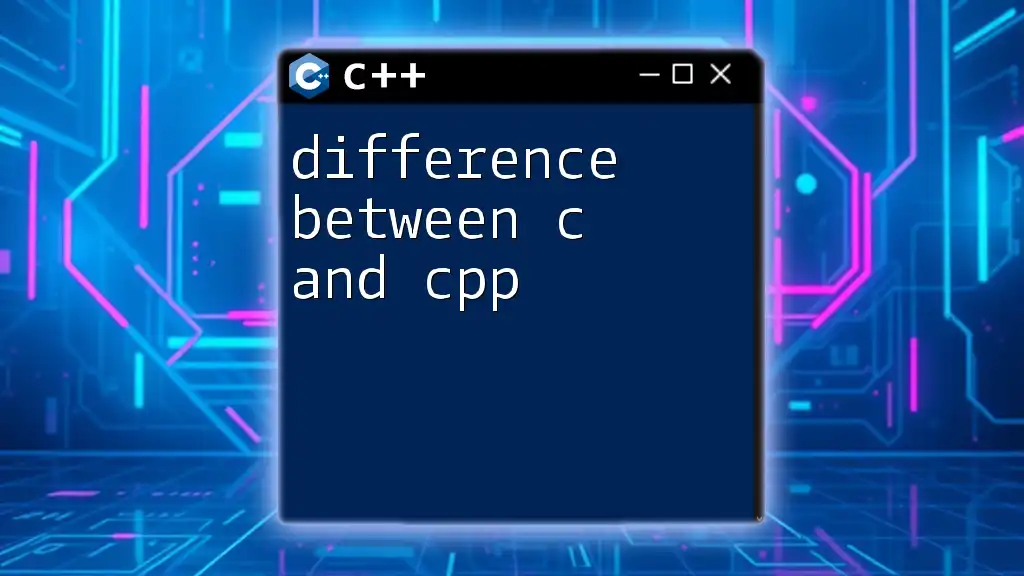
Functions in C++
Function Overloading
C++ allows multiple functions with the same name, known as function overloading, which differentiates them based on their parameter lists:
void print(int i);
void print(double f);
This feature enhances the flexibility and readability of your code.
Inline Functions
Inline functions in C++ provide a way to suggest to the compiler to embed the function's code directly into the calling code to optimize performance. This is useful for small, frequently called functions.
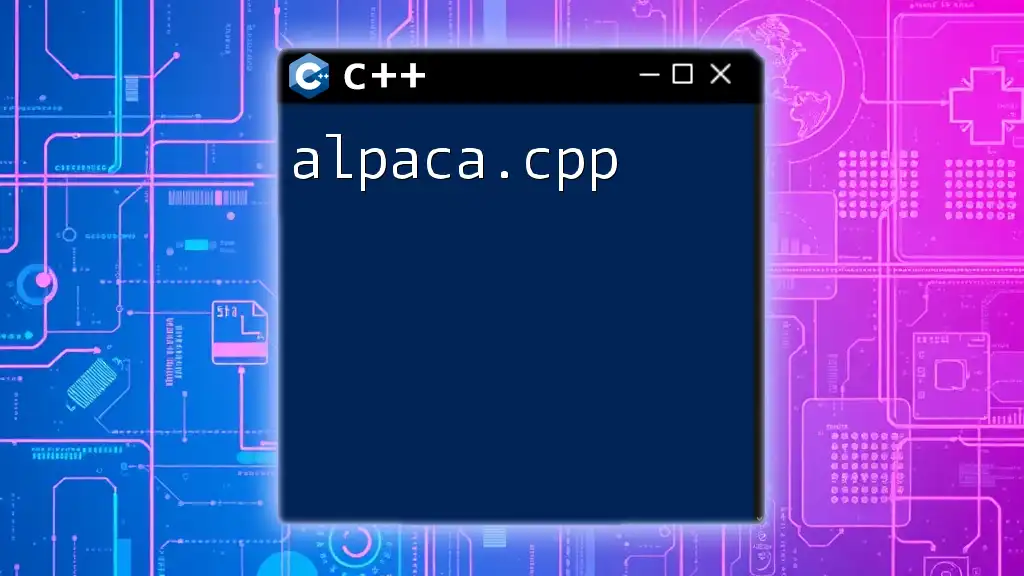
Object-Oriented Programming in C++
Understanding Classes and Objects
C++'s cornerstone is its ability to support classes and objects. Through classes, you can define the properties and methods that analogize real-world objects.
For example, consider this simple class definition:
class Car {
public:
void honk() {
std::cout << "Honk!";
}
};
This class encapsulates behavior associated with cars, allowing for easy instantiation and utilization.
Inheritance and Polymorphism
C++ facilitates inheritance, where a class can derive properties from another class. This promotes reuse of code and abstraction. Polymorphism allows methods to be used with objects of different types, making code extensible.
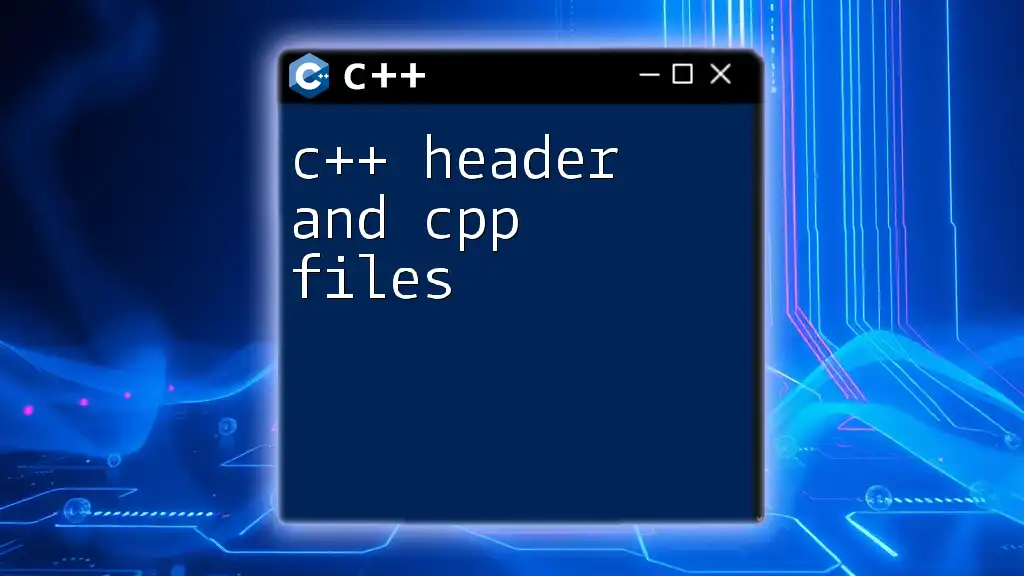
Best Practices in C and C++
Coding Standards and Style Guides
Maintaining a clean and readable codebase is paramount for any project. Consistent naming conventions, indentation, comments, and file organization significantly contribute to code maintainability, making it easier to understand and modify.
Debugging and Optimization
Debugging tools such as `gdb` (for C and C++) aid developers in finding and resolving issues within their code efficiently. Memory management and optimization techniques, such as minimizing the use of unnecessary variables and loops, can lead to significant performance improvements.
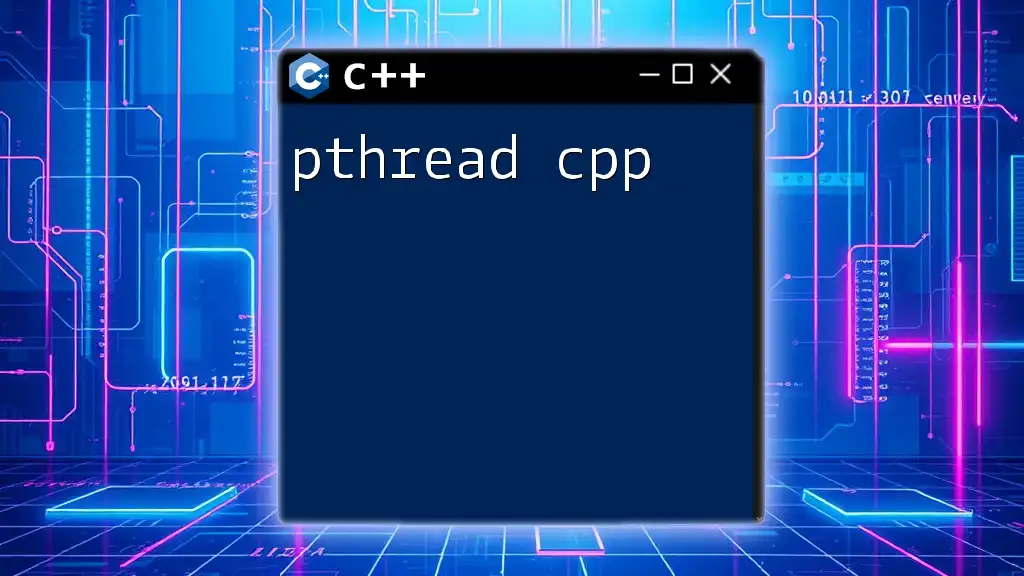
Conclusion
Mastering C and C++ unlocks a deep understanding of programming concepts that underpin many modern systems and applications. By learning these languages, you equip yourself with the skills necessary to tackle complex problems in various domains, from embedded systems to large-scale software development.
Additional Resources
To further deepen your knowledge, consider exploring resources such as foundational books on C and C++, engaging in online communities, and taking structured courses that reinforce your understanding of these vital programming languages.