Conan is a powerful C++ package manager that simplifies the process of managing dependencies, allowing developers to easily install, update, and configure libraries in their C++ projects.
Here's a snippet demonstrating how to create a basic Conan package:
# conanfile.py
from conans import ConanFile, CMake
class MyLibraryConan(ConanFile):
name = "MyLibrary"
version = "0.1"
settings = "os", "compiler", "build_type", "arch"
generators = "cmake"
def source(self):
self.run("git clone https://github.com/user/mylibrary.git")
def build(self):
cmake = CMake(self)
cmake.configure()
cmake.build()
def package(self):
self.copy("*.h", dst="include", src="src")
self.copy("*MyLibrary.lib", dst="lib", keep_path=False)
def package_info(self):
self.cpp_info.libs = ["MyLibrary"]
What is Conan?
Conan is an open-source C++ package manager designed to simplify the process of managing C++ dependencies. It allows developers to easily configure, distribute, and manage libraries, thereby improving productivity and collaboration within teams.
Key Features of Conan
- Cross-platform Capabilities: Conan works seamlessly across different platforms, making it easy for developers to ensure that their applications can be built on Windows, Linux, and macOS without compatibility issues.
- Dependency Management: Conan automates the handling of dependencies. This means that developers can focus on their application rather than the tedious task of downloading, building, and linking libraries manually.
- Simple Configuration: With Conan, configuring libraries is often as simple as writing a few lines of code in a configuration file.

Getting Started with Conan
Installing Conan
Before you can begin using Conan, you must install it on your system. The installation process is straightforward.
-
System Requirements: Ensure that you have Python installed, as Conan is distributed as a Python package.
-
Installation Methods: The simplest way to install Conan is through pip. You can do this by running the following command in your terminal:
pip install conan
-
Verifying Installation: To confirm that Conan has been successfully installed, you can type the following command:
conan --version
This should display the current version of Conan, indicating that the installation process was successful.
Basic Conan Commands
As you start using Conan, you'll encounter several foundational commands that streamline package management:
-
`conan new`: This command creates a new package template. It's the starting point for your libraries.
conan new libname/0.1@user/channel
-
`conan create`: This command builds and uploads your package.
conan create . user/channel
-
`conan install`: This will install the required packages and their dependencies into your project.
conan install . --build=missing
-
`conan search`: This command lets you find existing packages.
conan search package_name
Conan Configuration
Properly configuring your Conan environment is crucial for effective package management.
Setting Up the Conan Profile
The Conan profile allows you to define your build settings like compiler, version, architecture, and build type. This ensures that your packages are built consistently across different environments.
To create a custom profile, you can run:
conan profile new myprofile --detect
and then modify it in the profile directory.
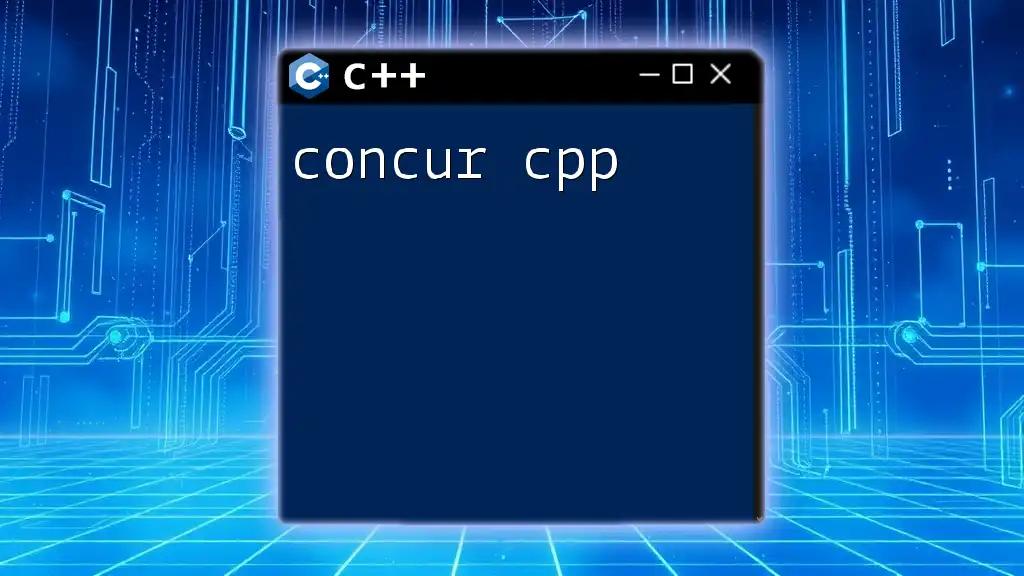
Working with Packages in Conan
Creating a New Package
Creating a new package in Conan involves several steps:
-
Writing the `conanfile.py`: This is the core file that defines your package. It includes essential details like name, version, settings, and requirements. Here’s an example of a simple `conanfile.py`:
from conans import ConanFile, CMake class MyLibraryConan(ConanFile): name = "MyLibrary" version = "0.1" settings = "os", "compiler", "build_type", "arch" generators = "cmake" def build(self): cmake = CMake(self) cmake.configure() cmake.build()
-
Building the Package: After writing the conanfile, you can build your package with the command:
conan create . user/channel
-
Testing the Package: It’s essential to test your package after creating it to ensure everything works as expected.
Using Existing Packages
Using existing packages in Conan is just as simple. You can find and install them easily through the following steps:
-
Finding Packages: Use the `conan search` command to look for packages in the Conan Center, the default repository.
conan search boost
-
Installing from the Conan Center: To install a package, run:
conan install boost/1.75.0@
This command will automatically download and configure the Boost library for your project.
- Integrating Installed Packages: To use the installed packages in your C++ project, ensure that you include them in your build files, such as `CMakeLists.txt`, using appropriate commands to link them.
Managing Dependencies
One of Conan's powerful features is its ability to manage dependencies. Understanding dependency graphs is essential for C++ development.
-
Transitive Dependencies: Conan automatically handles transitive dependencies for you. If your library depends on another library, Conan will retrieve it, ensuring all required libraries are accounted for.
-
Example: If your `conanfile.py` needs a library like `Boost`, simply add it under the requirements section:
requirements = "boost/1.75.0"
When you run the `conan create` or `conan install` command, Conan fetches Boost and any of its dependencies automatically.

Advanced Features of Conan
Versioning and Package Updates
Managing package versions is crucial for long-term project maintenance. When you want to update a package, you should always adhere to semantic versioning rules.
-
Updating Packages: Use the following command to update a specific package:
conan install . --update
This command checks for updates to the dependencies listed in your `conanfile.py` and fetches the latest versions.
Creating and Using Hooks
Hooks in Conan allow you to customize the package creation process and enforce certain policies for your team.
-
Creating a Simple Hook: Hooks can be placed in the `~/.conan/hooks` directory. An example of a hook might log each package creation:
import os def pre_create(conanfile): with open("creation_log.txt", "a") as log: log.write(f"Creating package: {conanfile.name}/{conanfile.version}\n")
Custom Remote Servers
For teams that need to share packages privately, setting up a custom Conan remote server is invaluable.
-
Setting Up a Custom Remote: You can add a remote server with the command:
conan remote add myremote https://my.custom.remote
-
Managing Multiple Remotes: To list and manage your remotes, use:
conan remote list conan remote remove myremote
These commands help maintain and access multiple repositories efficiently.
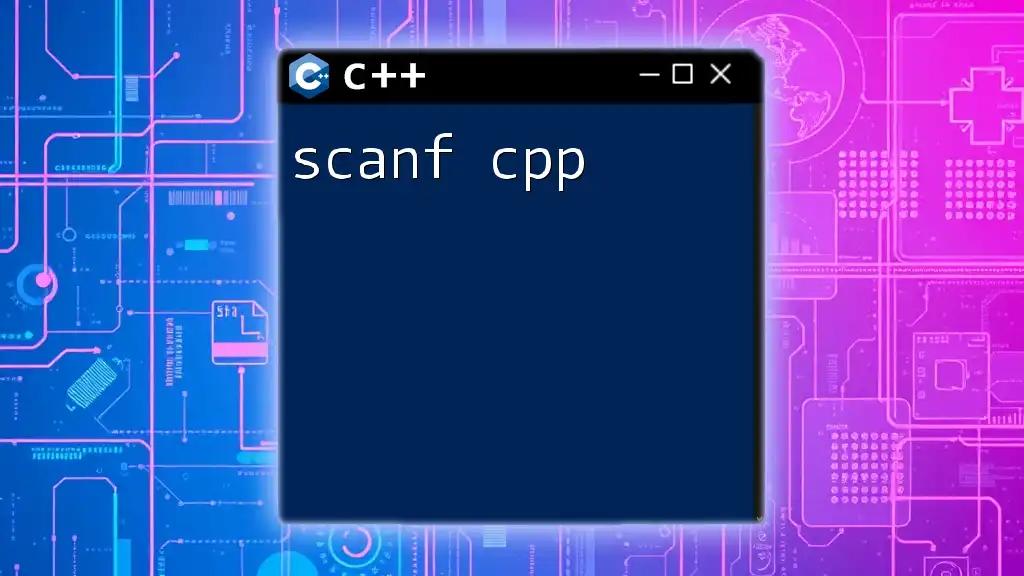
Integrating Conan with Build Systems
CMake Integration
CMake is a popular build system and integrates seamlessly with Conan.
-
Basic Configuration: To configure CMake with Conan, you need to include the generated `conanbuildinfo.cmake` file. Here's how to do it in your `CMakeLists.txt`:
include(${CMAKE_BINARY_DIR}/conanbuildinfo.cmake) conan_basic_setup()
-
Sample CMakeLists.txt: Below is a simple example showcasing how to integrate Conan into a `CMakeLists.txt`:
cmake_minimum_required(VERSION 3.1) project(MyApp) include(${CMAKE_BINARY_DIR}/conanbuildinfo.cmake) conan_basic_setup() add_executable(myapp main.cpp) target_link_libraries(myapp ${CONAN_LIBS})
Other Build Systems
In addition to CMake, Conan supports various build systems like Makefile and Visual Studio.
-
Makefile Integration: To integrate Conan with Makefiles, you can invoke Conan commands directly from your Makefile, placing dependencies in the build targets.
-
Visual Studio: For Visual Studio user, installation and usage will be automatic once Conan is configured, making it a smooth experience.
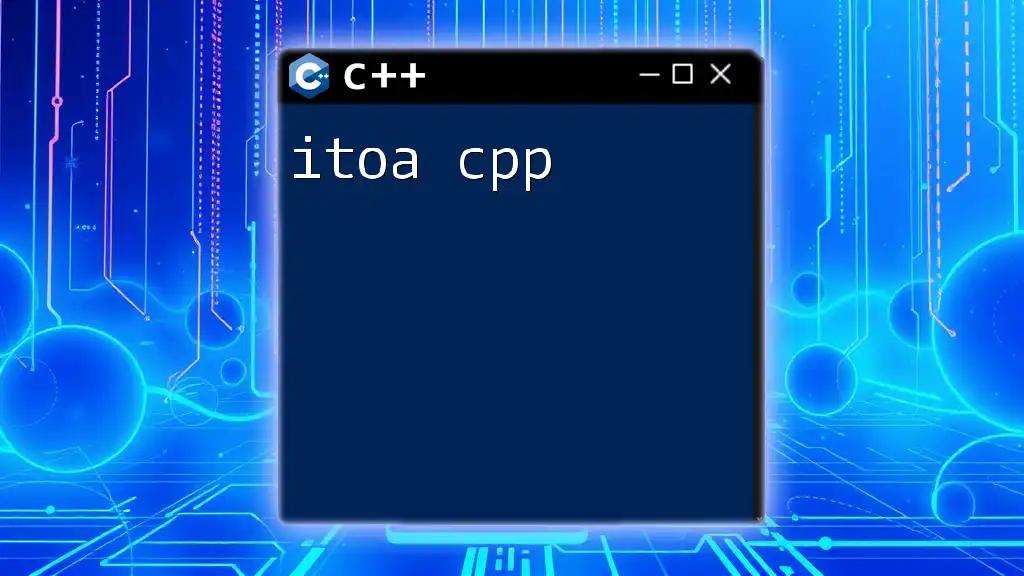
Troubleshooting Common Issues
Common Errors
As with any tool, you may encounter issues when using Conan. Here are some common errors:
- Resolving Dependencies: If Conan reports that it cannot find a package, ensure that the package name and version are correctly specified and that you have access to the relevant remotes.
FAQ
-
How does Conan compare to other package managers? Conan is specialized for C++, focusing on the unique challenges faced in C++ development, such as complex dependency management and build configuration.
-
Can I use Conan with non-C++ projects? While Conan is primarily designed for C++, some users have successfully employed it for mixed-language projects that involve C++ libraries.
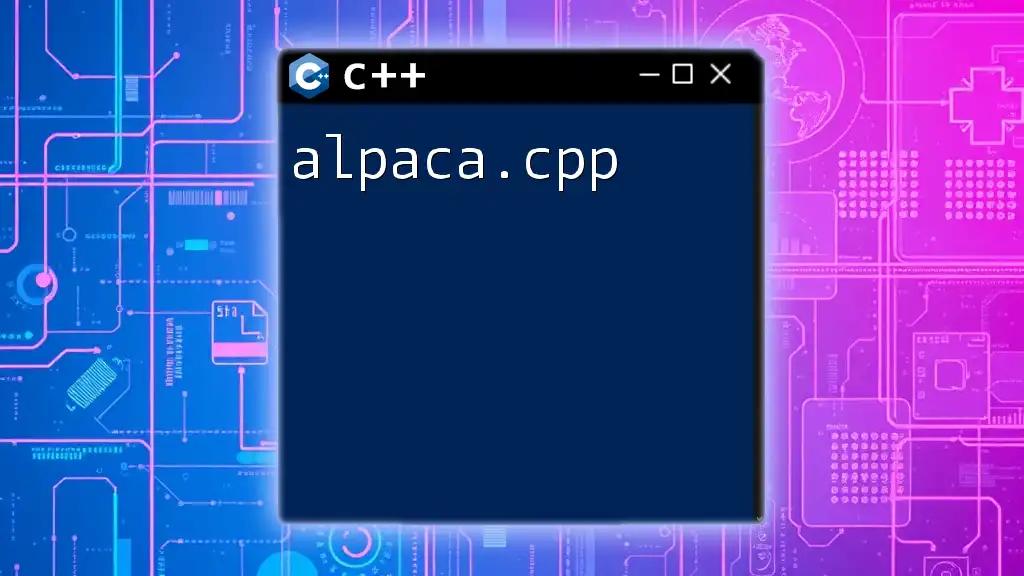
Conclusion
Conan serves as a powerful tool in the C++ ecosystem, helping developers manage their packages effortlessly. By using Conan, you not only save time but also streamline your workflow, allowing you to focus more on writing robust, high-quality code. We encourage developers to dive in and explore the vast capabilities of Conan for a more efficient C++ development experience.
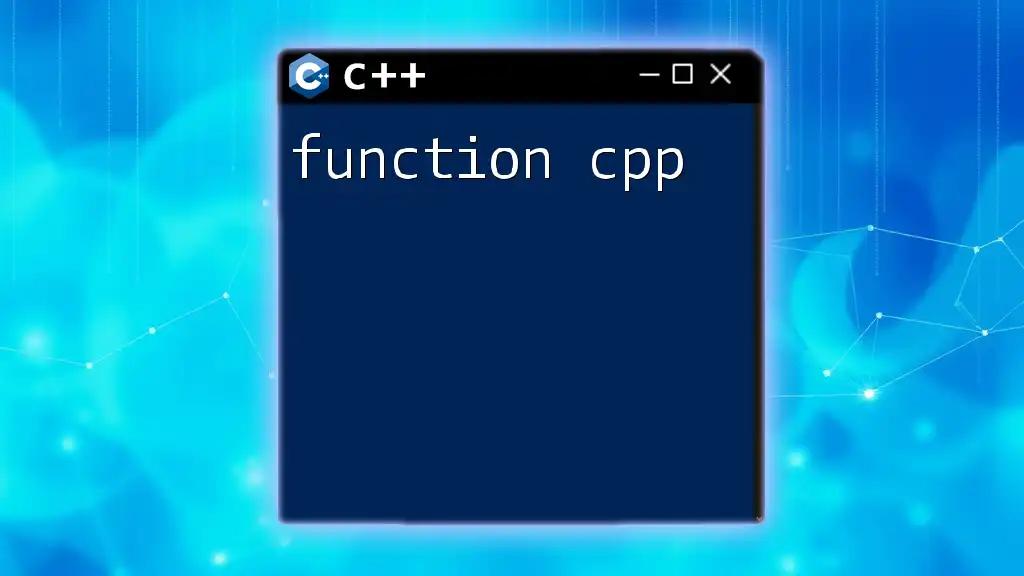
References and Resources
For further learning, consider exploring the following resources:
- [Official Conan Documentation](https://docs.conan.io/)
- [Conan Tutorials and Guides](https://docs.conan.io/en/latest/tutorials.html)
- [Conan Community Forums](https://community.conan.io)
By leveraging the capabilities of Conan, you can enhance your C++ development processes significantly, making your projects more manageable and collaborative.