The `#define` directive in C++ is used to create macros, allowing you to define constants or create shorthand for expressions in your code.
Here's an example in markdown format:
#define PI 3.14159
Definition of C++
What is C++?
C++ is a powerful general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs in the late 1970s. It is an extension of the C programming language and introduces features such as classes and objects, making it a multi-paradigm language. C++ allows programmers to efficiently manage software resources and is widely used in various applications, including system/software development, game development, and high-performance applications.
Characteristics of C++
Compiled Language: C++ is a compiled language, which means that code written in C++ is translated into machine code that the computer’s hardware can understand, through a process known as compilation. This results in highly optimized and efficient code execution.
Object-Oriented: One of the key features of C++ is its support for Object-Oriented Programming (OOP). OOP allows developers to create classes (blueprints for objects) that encapsulate data and behaviors, enhancing code reusability and organization.
Multi-Paradigm: C++ supports multiple programming paradigms, including procedural programming, object-oriented programming, and even functional programming techniques. This versatility makes it suitable for a broad spectrum of programming tasks.
Why is C++ Popular?
C++ enjoys tremendous popularity due to its:
- Speed and Efficiency: Programs written in C++ generally run faster and use resources more efficiently compared to those written in many other languages.
- Flexibility and Versatility: C++ can be used for systems software, application software, drivers, client-server applications, and more.
- Community and Resources Available: Being one of the oldest programming languages still in active use, C++ has a vast community and plenty of resources for learners.
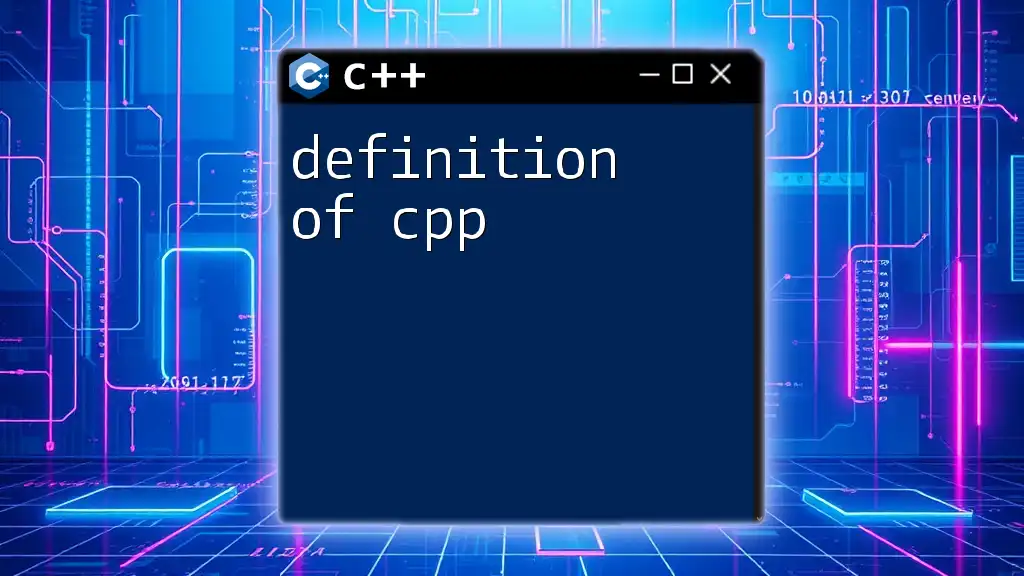
Key Features of C++
Object-Oriented Programming
Encapsulation: This principle involves bundling the data (attributes) and methods (functions) that operate on that data into a single unit, or class. It restricts direct access to some of the object’s components and is a way of preventing unintended interference.
For example:
class Encapsulated {
private:
int data;
public:
void setData(int value) { data = value; }
int getData() { return data; }
};
Inheritance: Inheritance allows a new class (derived class) to inherit properties and methods from an existing class (base class), promoting code reuse.
class Base {
public:
void display() { std::cout << "Base class display" << std::endl; }
};
class Derived : public Base {
public:
void show() { std::cout << "Derived class show" << std::endl; }
};
Polymorphism: Polymorphism enables methods to do different things based on the object it is working with, essentially allowing one function to be used for different data types.
An example of runtime polymorphism can be illustrated through virtual functions:
class Base {
public:
virtual void greet() { std::cout << "Hello from Base!" << std::endl; }
};
class Derived : public Base {
public:
void greet() override { std::cout << "Hello from Derived!" << std::endl; }
};
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful library that provides a set of common classes and functions. It includes various data structures (like vectors and lists), algorithms (like sort and search), and iterators that streamline the process of developing applications.
The benefits of using STL include:
- Code Efficiency: It allows developers to implement complex functionalities without writing extensive code.
- Reusability: Templates can be reused for different data types.
An example of using STL can be demonstrated with vectors:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Memory Management
Dynamic Memory Allocation: In C++, we manage memory with pointers. Dynamic memory allocation allows the program to request memory during runtime. This is achieved using the `new` and `delete` keywords.
Example:
int* p = new int; // dynamically allocating memory
*p = 10;
// use the pointer...
delete p; // freeing the memory
Smart Pointers: To enhance memory management, C++11 introduced smart pointers like `std::unique_ptr` and `std::shared_ptr`, which automate memory management and reduce memory leaks.
Example of a smart pointer:
#include <memory>
int main() {
std::unique_ptr<int> p(new int); // unique ownership
*p = 20;
// no need to delete, memory is managed automatically
return 0;
}
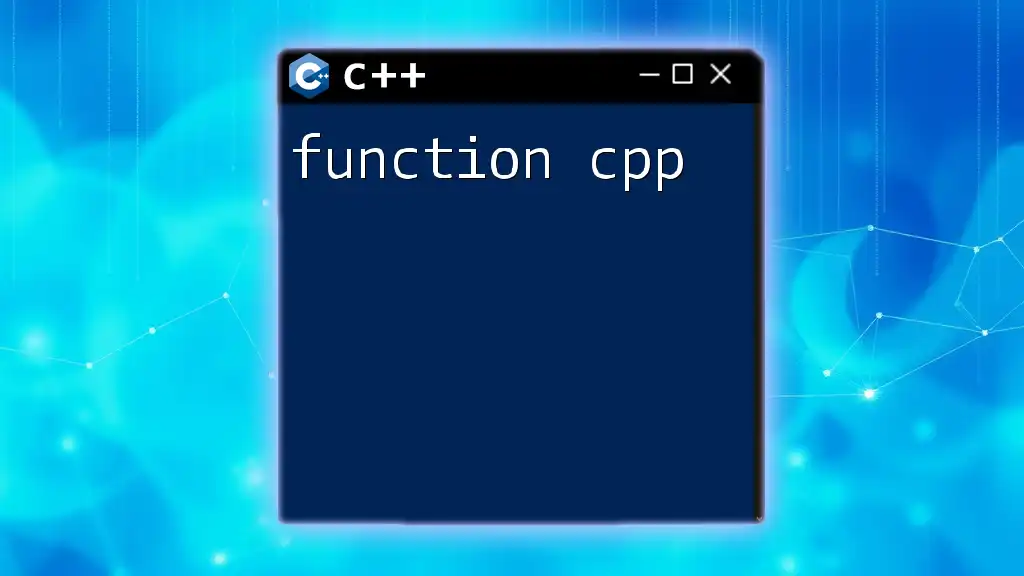
Basic Syntax and Structure of C++
C++ Syntax Overview
C++ has a syntax that is both powerful and complex, but a basic C++ program structure typically includes the following components:
#include <iostream> // include header files
int main() {
std::cout << "Hello, World!" << std::endl; // output message
return 0; // return statement
}
Data Types and Variables
C++ provides several built-in data types including:
- int: for integer values.
- float: for floating-point numbers.
- char: for single characters.
- bool: for boolean values (true/false).
Variable declaration involves specifying the type followed by the variable name:
int age = 25;
float salary = 50000.5;
char grade = 'A';
bool isEmployed = true;
Control Structures
Conditional Statements: C++ supports conditional statements like `if`, `else`, and `switch` to control the flow of the program based on certain conditions.
int number = 10;
if (number > 0) {
std::cout << "Positive number" << std::endl;
} else {
std::cout << "Negative number" << std::endl;
}
Loops: C++ offers loops such as `for`, `while`, and `do-while` for repeated execution of code blocks. For instance, a `for` loop looks like this:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl; // prints numbers 0 to 4
}
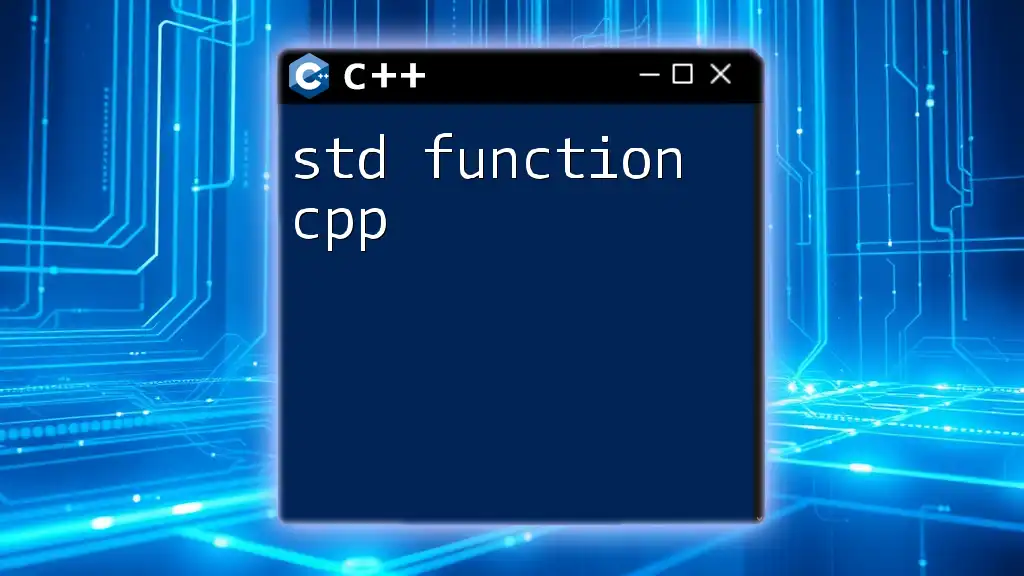
Frequently Used C++ Commands
Input and Output
C++ simplifies input and output handling using the `std::cin` for input and `std::cout` for output. This is one of the most fundamental operations in C++.
Example:
std::cout << "Enter a number: ";
int num;
std::cin >> num; // reading user input
std::cout << "You entered: " << num << std::endl;
Functions in C++
Functions are blocks of code that perform specific tasks. They can be defined and called as needed, promoting code reusability.
Example of defining and calling a function:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet(); // function call
return 0;
}
Function Overloading allows us to define multiple functions with the same name but different parameter lists.
void display(int a) {
std::cout << "Integer: " << a << std::endl;
}
void display(float b) {
std::cout << "Float: " << b << std::endl;
}
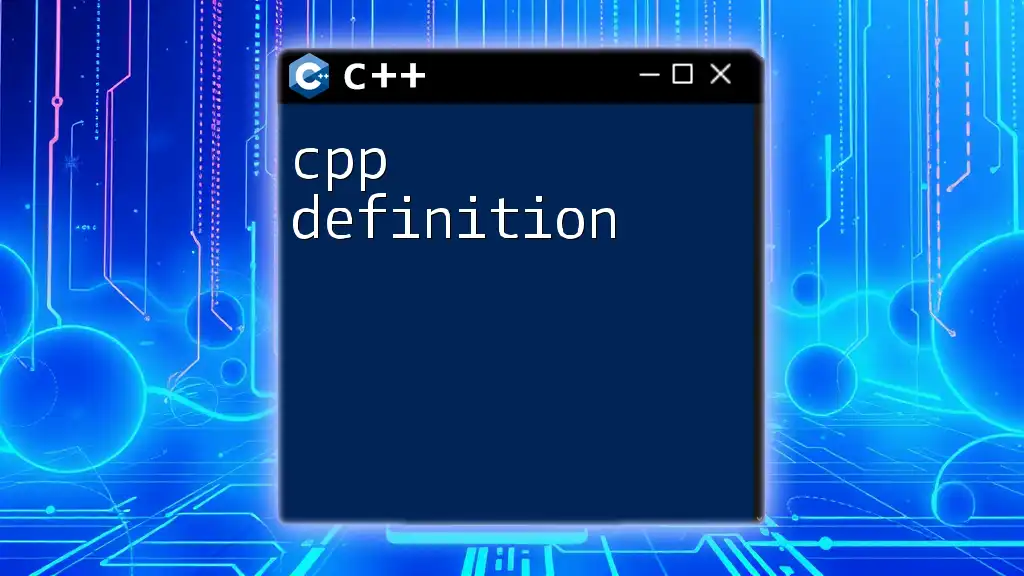
Best Practices in C++
When coding in C++, adhering to certain best practices can improve the quality and maintainability of the code:
- Code Organization and Structure: Keep the code clean and organized. Use meaningful names and consistent formatting.
- Commenting and Documentation: Add comments to explain complex sections of code and maintain documentation for future references.
- Error Handling and Debugging Techniques: Implement error checking and use debugging tools to identify and solve problems effectively.
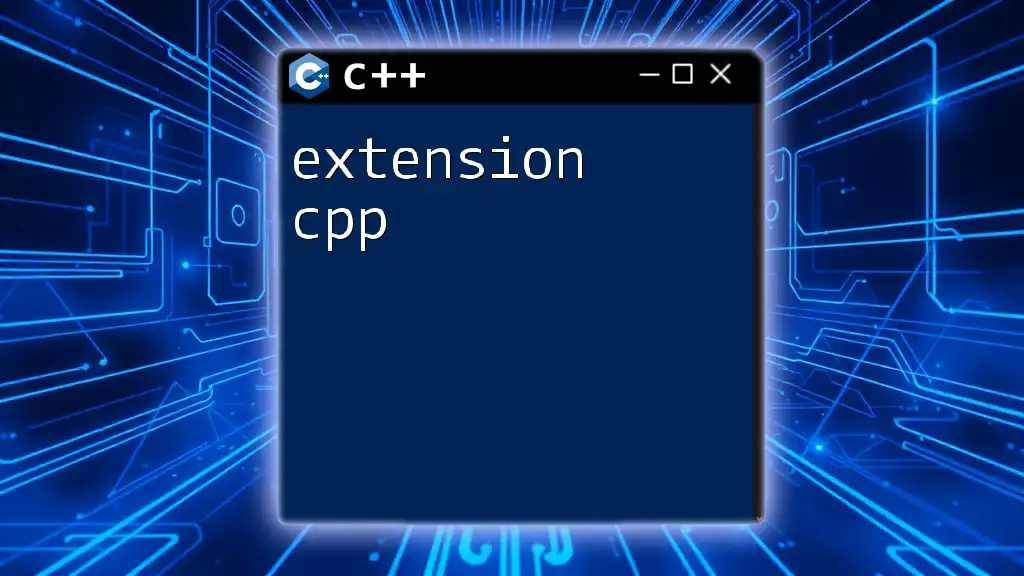
Conclusion
In summary, understanding the definition of C++ involves grasping its core principles, unique features, and the syntax that distinguishes it from other programming languages. C++ holds a vital place in the programming community due to its efficiency, versatility, and robust framework. As you delve deeper into C++, remember that practice and continuous learning are keys to becoming proficient in this powerful language.
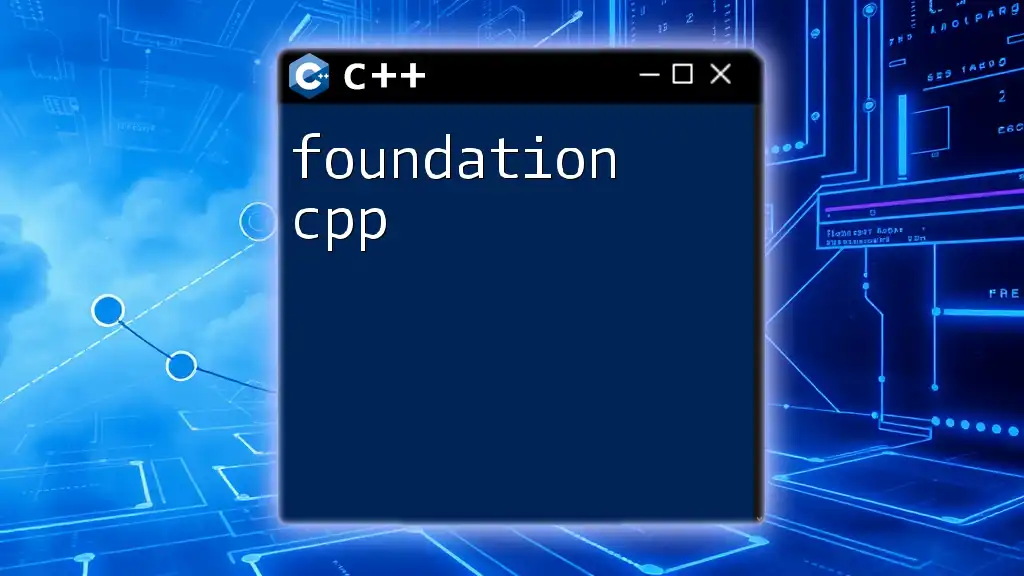
Additional Resources
To further enhance your knowledge of C++, consider exploring recommended books, online courses, and engaging with community forums. Don’t forget to experiment with various tools and IDEs available for C++ development, thereby boosting your programming skills efficiently.