Class type redefinition in C++ occurs when a class is defined more than once with the same name in the same scope, which typically results in a compilation error due to conflicting declarations.
Here is a simple code snippet demonstrating class type redefinition:
class MyClass {
public:
void display() {
cout << "Hello from MyClass!" << endl;
}
};
class MyClass { // This will cause a redefinition error
public:
void show() {
cout << "This is a redefined MyClass!" << endl;
}
};
Understanding Classes in C++
What is a Class?
A class in C++ is an essential building block of object-oriented programming (OOP). It serves as a blueprint for creating objects, encapsulating data and behavior in a single entity. Classes allow developers to model real-world entities, making it easier to manage and structure complex systems.
Syntax of Class Declaration
The syntax for declaring a class in C++ involves defining its members and methods. A basic structure looks like this:
class ClassName {
public:
// Members
Type memberName;
void memberFunction();
};
In this syntax:
- `ClassName` is the name of the class.
- The `public` access modifier allows class members to be accessible from outside the class.
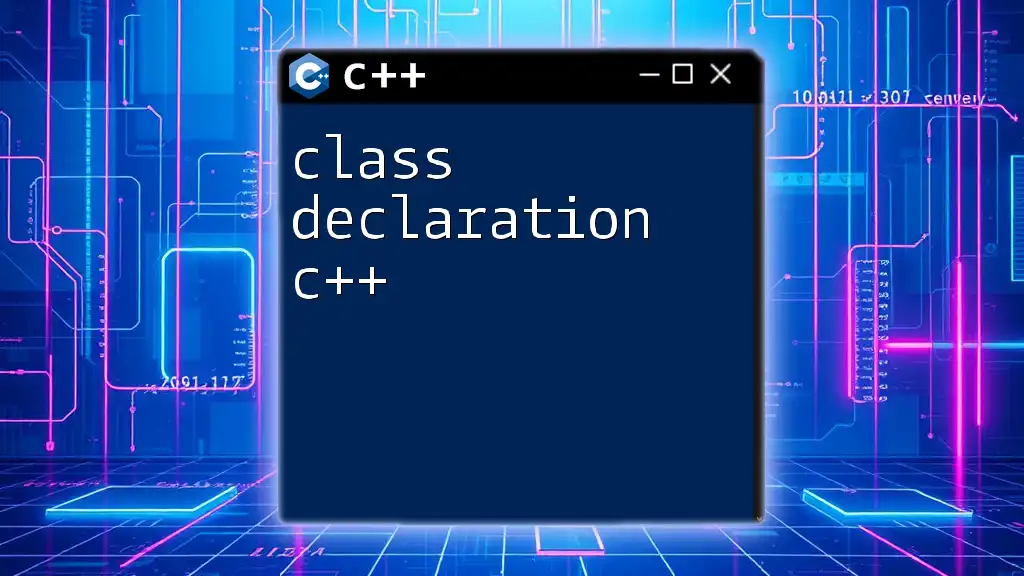
Class Type Redefinition: An Overview
What is Class Type Redefinition?
Class type redefinition in C++ occurs when you create an alias for an existing class type, providing a new name that can be used interchangeably with the original class. This approach can be invaluable for improving code readability and simplifying complex type definitions.
C++ Enhancements: The `using` Directive
A modern way to perform class type redefinition is using the `using` directive. This method allows you to create a new type name for an existing type, enhancing clarity and maintainability.
Syntax:
using NewTypeName = OriginalTypeName;
Example:
class Person {
public:
string name;
int age;
};
using Individual = Person;
With this definition, `Individual` can now be used wherever `Person` was originally referenced, enabling clearer and more concise code.
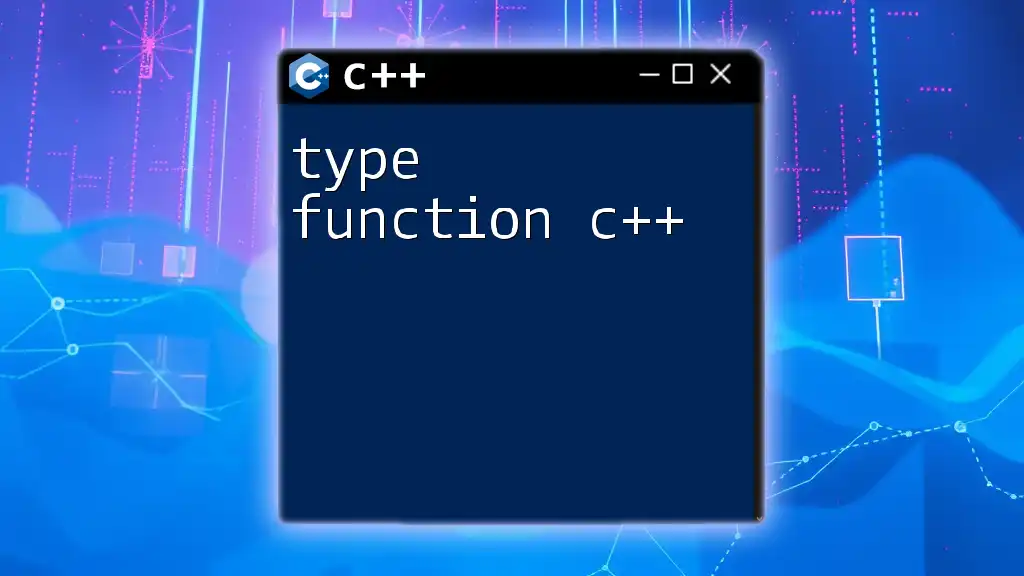
Common Scenarios of Class Type Redefinition
Redefining Types for Improved Clarity
Redefinition is often employed to enhance code readability. By using a more descriptive type name, the intention behind the type can be made clearer.
Example Scenario:
class Point {
public:
int x, y;
};
using Coordinate = Point;
In this case, the name `Coordinate` gives a better understanding of what the class represents, benefiting anyone reading or maintaining the code.
Type Aliases with Structs
Structs in C++ can also be redefined in a similar manner. A struct definition allows you to group variables, and sometimes it helps to offer a type alias that signifies its purpose better.
Example:
struct MyStruct {
int value;
};
using AliasStruct = MyStruct;
Now, `AliasStruct` can be used anywhere in the code to refer to `MyStruct`, allowing for clearer semantics when used in different contexts.
Combining Type Redefinition with Inheritance
Class type redefinition also plays a critical role when working with inheritance. It can be used to create more expressive and self-explanatory class hierarchies.
Example Framework for Clarity:
class Base {
public:
virtual void show() {}
};
using DerivedType = Base;
class Derived : public DerivedType {
public:
void show() override {}
};
In this example, `DerivedType` acts as an alias for `Base`, and any further modifications or implementations of inherited classes can utilize the alias for enhanced clarity about its purpose in the hierarchy.
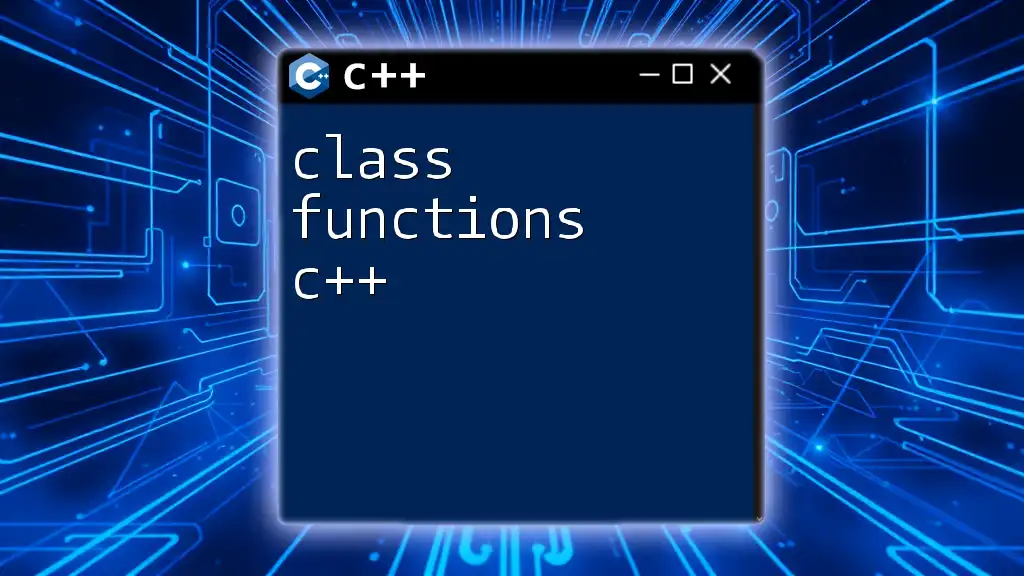
Practical Example of Class Type Redefinition
Step-by-Step Walkthrough
Consider a scenario where you have an abstract class designed for different animal behaviors. You want to redefine its type for better clarity and maintainability.
Code Snippet Illustrating the Redefinition Process:
class Animal {
public:
virtual void speak() = 0; // Pure virtual function
};
using Creature = Animal;
class Dog : public Creature {
public:
void speak() override { std::cout << "Woof!" << std::endl; }
};
In this example:
- The `Animal` class represents a base type for all animal behavior.
- The alias `Creature` is created to refer to `Animal`, making it clear that each derived class represents a creature.
- The `Dog` class, inheriting from `Creature`, implements the `speak` function.
Benefits of Using Class Type Redefinition
Using class type redefinition offers several benefits:
- Improved Code Management: Larger codebases become easier to manage when types are clearly defined and understood.
- Enhanced Compatibility: Type redefinitions help in merging different codebases more seamlessly, as the purpose of types becomes obvious.
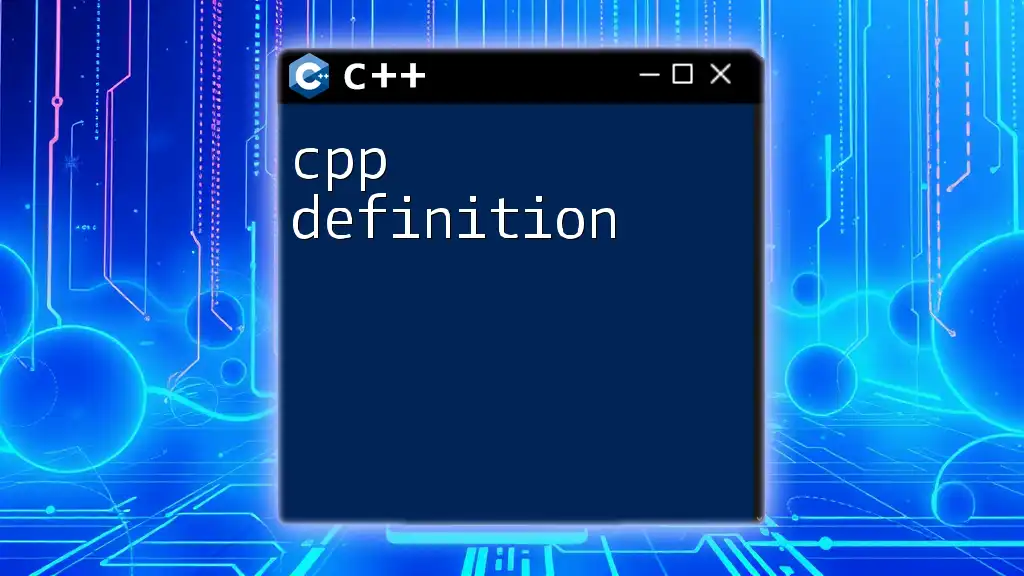
Best Practices
When to Use Class Type Redefinition
Defining type aliases is beneficial when:
- You have complex type names that would benefit from simplification.
- You want to convey a more descriptive purpose of a type without changing the original implementation.
Avoiding Common Pitfalls
Be mindful of potential pitfalls when using class type redefinition:
- Ambiguity: Overusing redefinitions can lead to confusion if multiple aliases may refer to similar underlying types.
- Naming Conflicts: Ensure that your newly defined names do not clash with existing class names or standard library types.
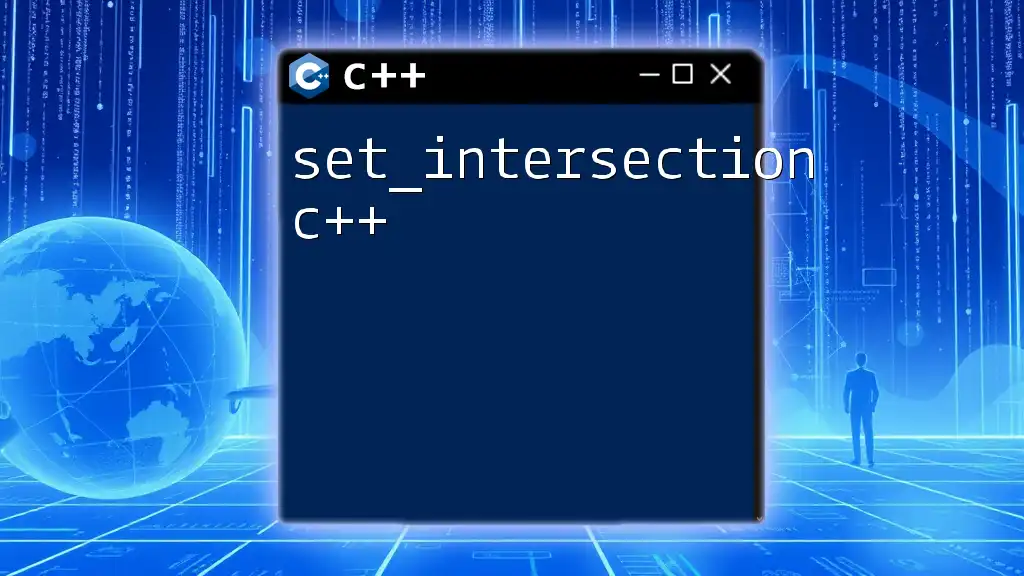
Conclusion
Understanding class type redefinition in C++ is crucial for developing clean, maintainable code. It allows developers to simplify complex type definitions and improve code clarity, ultimately leading to better collaboration and easier long-term maintenance of software projects. As with any technique in programming, it is imperative to apply it thoughtfully and adhere to best practices to realize its full potential.
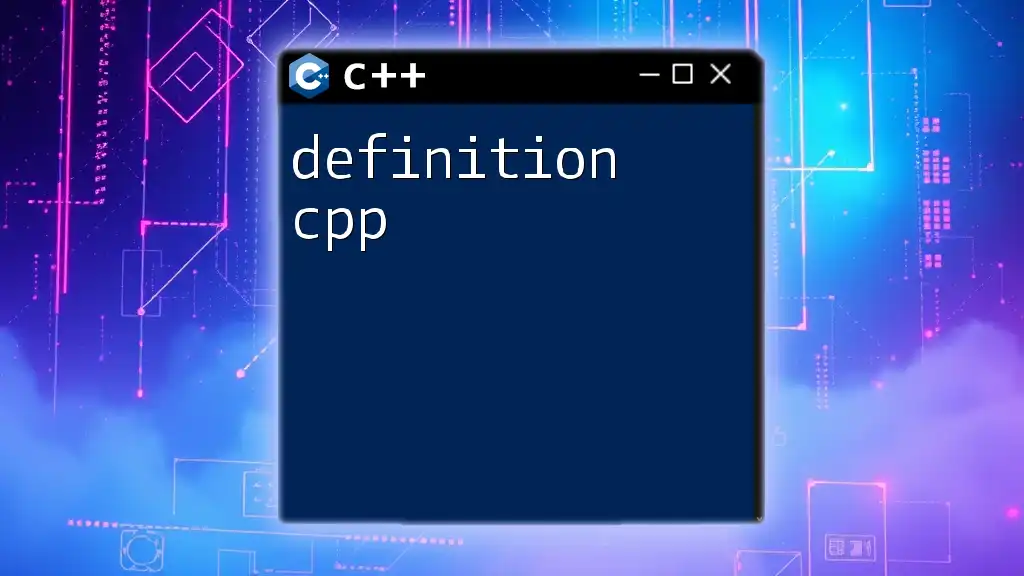
Additional Resources
To deepen your knowledge on class type redefinition and other C++ techniques, consider exploring recommended readings and participating in community forums for ongoing discussions.
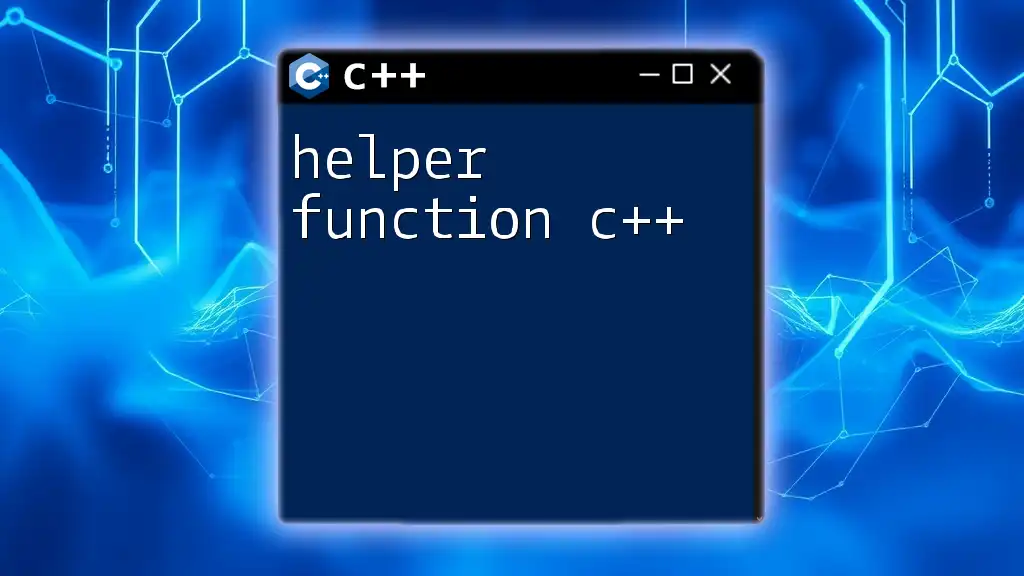
FAQs
What happens when you redefine a class type multiple times?
Redefining a class type multiple times can lead to compilation errors if the redefinitions conflict. It's essential to ensure consistency and avoid ambiguous definitions across your codebase.
Can class type redefinition be utilized across different files?
Yes, class type redefinition can be effectively utilized across different files by placing the appropriate `using` declarations in header files, allowing for a consistent understanding of types throughout your project.
Are there alternatives to class type redefinition?
Alternatives, such as templates or namespaces, can be utilized. Templates allow for type-safe programming when you need generic types and can provide similar benefits without needing to redefine types explicitly.