In C++, a class can be redefined by creating a new definition with the same name, which is generally not recommended as it can lead to ambiguity and errors, unless it occurs within a different scope or conditionally via preprocessor directives.
Here’s a code snippet to illustrate a simple redefinition of a class:
class MyClass {
public:
void display() {
std::cout << "Original definition." << std::endl;
}
};
// Redefining the same class, potentially causing confusion
class MyClass {
public:
void display() {
std::cout << "Redefined definition." << std::endl;
}
};
The Basics of C++ Classes
What is a Class?
A class in C++ is a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data, providing a convenient way to organize and manage related functionalities. Classes combine both attributes (data members) and methods (functions) into a single unit, making it easier to manage the complexity of your code.
Constructors and Destructors
Constructors are special member functions that are automatically called when an object of a class is instantiated. Their primary purpose is to initialize the object’s data members efficiently. On the contrary, destructors are called when an object goes out of scope or is explicitly deleted. They clean up any resources allocated by the object, such as memory or file handles.
C++ Class Redefinition Explained
Definition of Class Redefinition in C++
The C++ redefinition of class occurs when a class that has already been defined is defined again in the same scope. This can be useful in scenarios where a class requires updates, but it is crucial to understand that it can lead to compilation errors if not handled correctly. Redefining a class allows you to change its structure, add new methods, or alter existing ones, thereby extending its functionality.
Syntax for Redefining a Class
The syntax for class redefinition is straightforward; however, it may vary depending on what changes are being made. Below is a simple example of how a class can be redefined:
class MyClass {
public:
void show() {
cout << "Hello from MyClass!" << endl;
}
};
// Redefining MyClass with an updated method
class MyClass {
public:
void show() {
cout << "Hello from the redefined MyClass!" << endl;
}
};
By redefining `MyClass`, you can modify the behavior of the `show` method, allowing for flexibility in how classes are utilized.
Guidelines for Redefining Classes Safely
Preventing Redefinition Errors
One of the most common pitfalls in C++ programming is encountering redefinition errors. To prevent these errors, it is essential to utilize include guards or `#pragma once` directives, which ensure that classes are only defined once within a single compilation unit.
#ifndef MYCLASS_H
#define MYCLASS_H
class MyClass {
// Class definition here
};
#endif // MYCLASS_H
This usage prevents multiple definitions of the same class and helps maintain clean and error-free code.
Scope of Redefined Classes
Redefining classes can lead to potential issues with variable scope. When redefined, it's essential to understand how the new class definition interacts with previously defined versions. It’s best practice to clearly delineate class scopes using namespaces or selectively using classes to avoid naming conflicts.
Real-world Scenarios for Class Redefinition
Extending Class Functionality
C++ class redefinition serves a powerful purpose when extending the functionality of existing classes. You can redefine classes to add new methods that enhance their capabilities. Here is an example:
class MathOperations {
public:
int add(int a, int b) { return a + b; }
};
// Redefining MathOperations to include subtract method
class MathOperations {
public:
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
};
In this case, the `MathOperations` class is redefined to include a new `subtract` method, thus enriching the functionality of the class without the need for creating a new class entirely.
Version Control in Class Redefinitions
In more complex environments, managing different versions of classes becomes critical. Using careful comments and versioning strategies can help maintain clarity when working with redefined classes. Always ensure to include documentation on changes made to assist future developers (or yourself) in understanding the decision-making process behind redefinitions.
Challenges and Considerations
Understanding Compiler Behavior
A fundamental understanding of how compilers treat class redefinitions is essential to avoid unintended behaviors. When a class is redefined, the compiler might choose to discard the old definition unless properly managed. Key factors include ensuring correct access permissions (private, public, protected) to prevent access violations.
Class Redefinition in Inheritance
Inheritance can complicate the redefinition process. When redefining a base class, ensure that all derived classes are updated accordingly to prevent inconsistencies. For example:
class Base {
public:
virtual void info() {
cout << "Base class" << endl;
}
};
// Redefining in derived class
class Derived : public Base {
public:
void info() override {
cout << "Derived class" << endl;
}
};
In the code above, the `Derived` class overrides a method from the `Base` class, demonstrating how class redefinitions in an inheritance hierarchy can introduce additional complexities.
Best Practices for Class Redefinition
Maintain Clear Documentation
Documentation is critical when redefining classes. Use comments to mark changes clearly and consider maintaining a CHANGELOG file to track modifications over time. Clear documentation removes ambiguity and helps clarify the evolution of your software architecture.
Unit Testing Redefined Classes
Integrating unit tests for your redefined classes is crucial. Testing helps ensure that redefinitions do not introduce bugs or break existing functionality. Automated tests can help maintain stability as you continue to evolve the class structure.

Conclusion
The C++ redefinition of class is a powerful tool in the arsenal of any C++ programmer, enabling the extension and modification of class functionalities while managing code complexity. By understanding its implications and following best practices, you can leverage class redefinitions to enhance your codebase effectively and efficiently.
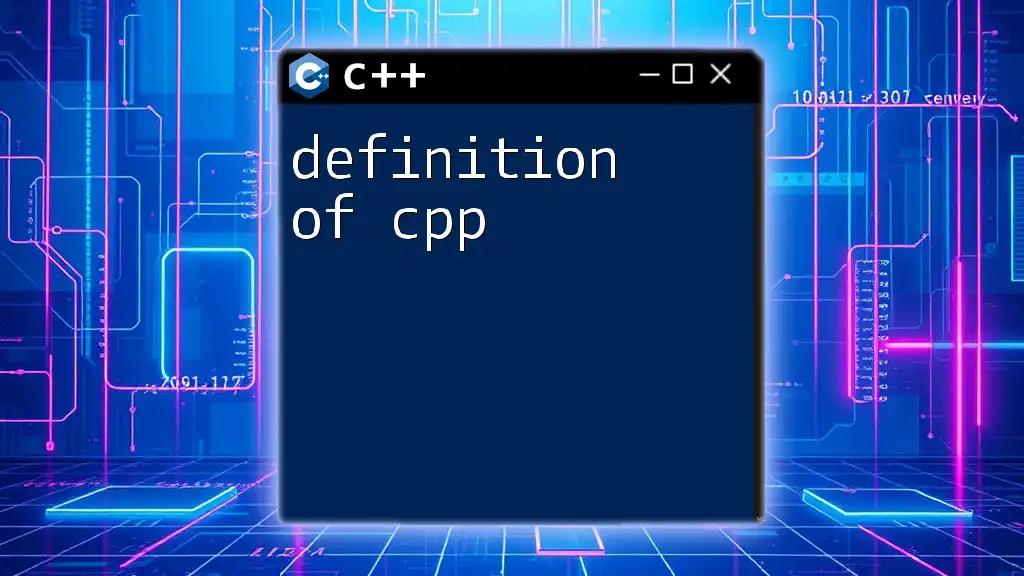
Additional Resources
For further exploration of C++ programming and class design principles, consider diving into recommended books, online courses, and developer communities that can support your learning journey.