C++ (CPP) is a high-level programming language that supports object-oriented, procedural, and generic programming, enabling developers to create efficient and complex software applications.
Here’s a simple example of defining a class and creating an object in C++:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
What is CPP?
Definition of CPP
C++ (pronounced "C plus plus") is a general-purpose programming language created as an extension of the C programming language. The acronym "CPP" is often used as shorthand to refer to C++ and its various commands. Understanding the definition of cpp is crucial for anyone diving into the realms of software development, as it allows for writing efficient and performant code with object-oriented paradigms.
Brief History of C++
C++ was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It was designed to give C capabilities alongside the programming paradigms of Simula, particularly object-oriented programming. Key milestones in its evolution include:
- 1985: Release of the first edition of "The C++ Programming Language".
- 1998: The first standardized version of C++ (C++98) was published.
- 2011: Introduction of the C++11 standard, which brought significant improvements and features like auto keyword, range-based for loops, and smart pointers.
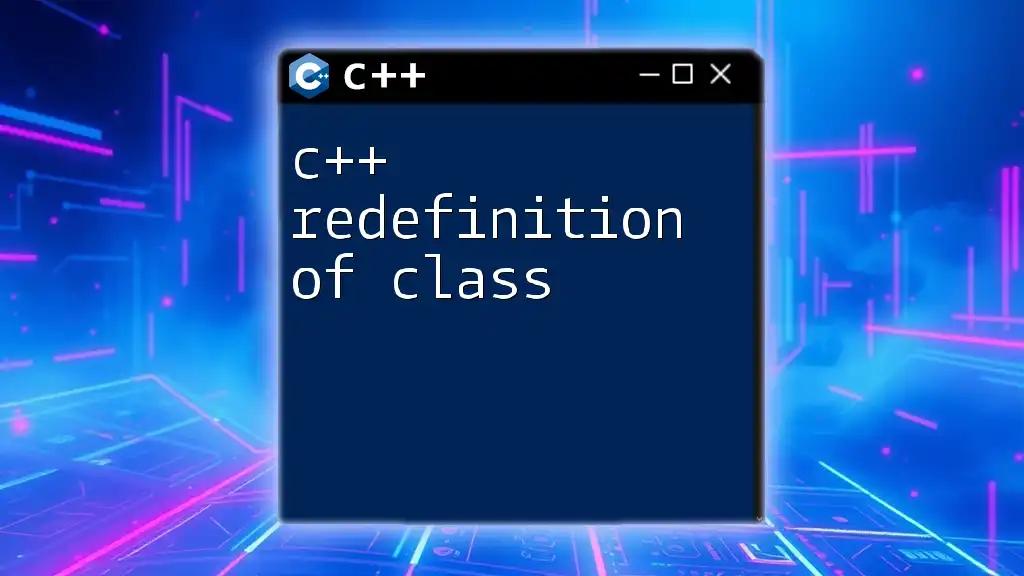
Features of C++
Object-Oriented Programming
C++ adopts object-oriented programming (OOP) principles, which encourage a modular design. Fundamental OOP concepts include:
- Classes: Templates for creating objects.
- Objects: Instances of classes representing real-world entities.
- Inheritance: Enables classes to derive properties and behaviors from existing classes.
Here’s an example that demonstrates OOP principles with a simple `Animal` class:
class Animal {
public:
void speak() {
cout << "Animal speaks!" << endl;
}
};
class Dog : public Animal {
public:
void speak() {
cout << "Bark!" << endl;
}
};
int main() {
Dog dog;
dog.speak(); // Output: Bark!
return 0;
}
Strongly Typed Language
C++ is a strongly typed language, which means that every variable must be declared with a data type. This helps in catching errors at compile-time rather than runtime, thus promoting safer and more predictable code. In contrast, languages like Python and JavaScript are dynamically typed, allowing more flexibility but resulting in potential runtime errors.
Standard Template Library (STL)
The Standard Template Library is a powerful C++ feature consisting of a set of C++ template classes to provide general-purpose classes and functions. STL includes the following components:
- Algorithms: Functions that operate on data structures (e.g., sorting, searching).
- Containers: Data structures that store collections of objects (e.g., vectors, lists, maps).
- Iterators: Abstractions used to traverse container elements.
Here’s an example of using the `std::vector` from the STL:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6);
for (int number : numbers) {
std::cout << number << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
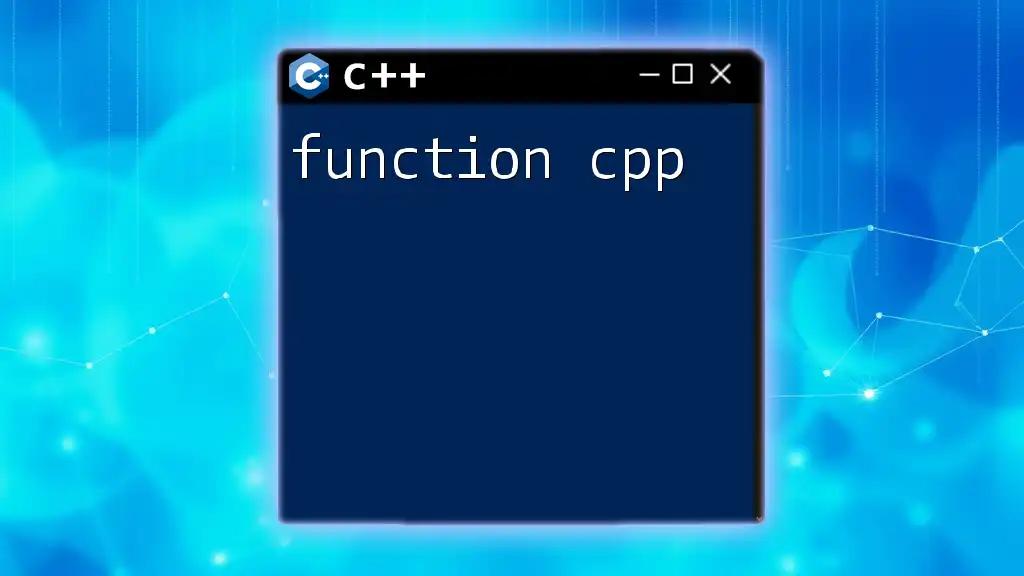
Understanding C++ Commands
What Are C++ Commands?
C++ commands refer to the syntax and instructions used to perform operations and define logic in C++. The structure of these commands is crucial, as even small syntax errors can lead to compilation issues or unexpected behavior in code.
Basic C++ Commands
Input and Output
C++ utilizes iostream for input and output operations. The primary commands used are `cin` (for input) and `cout` (for output). This can be illustrated with the following snippet:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "Your age is " << age << " years." << endl;
return 0;
}
Control Structures
Control structures manage the flow of execution in a program. Important structures include:
- If statements – For conditional execution.
- Switch statements – For multi-way branching.
- Loops (`for`, `while`, `do-while`) – For repeated execution.
Here’s a snippet using an `if` statement:
#include <iostream>
using namespace std;
int main() {
int number = 10;
if (number > 0) {
cout << "Number is positive." << endl;
} else {
cout << "Number is not positive." << endl;
}
return 0;
}
Functions in C++
Definition and Purpose
Functions in C++ are reusable blocks of code that execute a specific task. They can take parameters and return values, promoting modular programming and code reusability.
Example of Defining and Calling Functions
Here’s how you can define and invoke a simple function in C++:
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 3);
cout << "Sum: " << result << endl; // Output: Sum: 8
return 0;
}
Advanced C++ Commands
Memory Management Commands
C++ requires manual memory management, which is handled using keywords `new` and `delete`. Proper management is essential to prevent memory leaks.
Example:
#include <iostream>
using namespace std;
int main() {
int* p = new int(5); // dynamically allocate memory
cout << *p << endl; // Output: 5
delete p; // free allocated memory
return 0;
}
Exception Handling
C++ provides mechanisms for error handling through exception handling using the `try`, `catch`, and `throw` keywords. This is integral for maintaining the robustness of applications.
Here's a simple example:
#include <iostream>
using namespace std;
int main() {
try {
throw 42; // throw an integer exception
} catch (int e) {
cout << "Caught exception: " << e << endl; // Output: Caught exception: 42
}
return 0;
}

Best Practices for Using C++ Commands
Writing Clean Code
Writing clean, maintainable code is crucial in C++. This includes:
- Consistent naming conventions: Use meaningful names for variables and functions.
- Proper comments: Explain why certain decisions were made in the code.
- Code organization: Structure your code logically and conceptually.
Debugging Common C++ Errors
Even experienced programmers encounter common pitfalls in C++. These may include:
- Syntax errors: Misspelled keywords or missing punctuation.
- Type-related issues: Mismatched types in operations.
- Memory leaks: Failing to deallocate dynamically allocated memory.
An understanding of error messages and debugging tools, such as gdb, can greatly assist in identifying and resolving these issues.
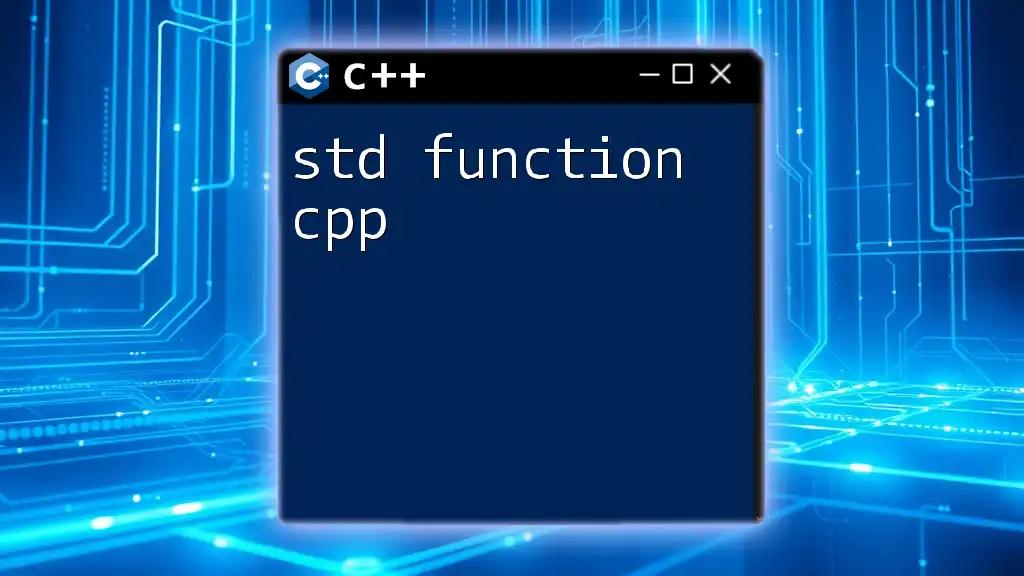
Resources for Learning C++
Recommended Books
Some noteworthy books for both beginners and advanced learners include:
- "The C++ Programming Language" by Bjarne Stroustrup
- "Effective C++" by Scott Meyers
- "C++ Primer" by Stanley B. Lippman
Online Courses and Tutorials
Several platforms offer comprehensive C++ tutorials that suit different learning needs:
- Codecademy
- Udacity C++ Nanodegree
- Coursera C++ courses
Community and Forums
Engaging with communities provides a wealth of knowledge and support. Popular forums include:
- Stack Overflow
- Reddit (r/cpp)
- Cplusplus.com forums
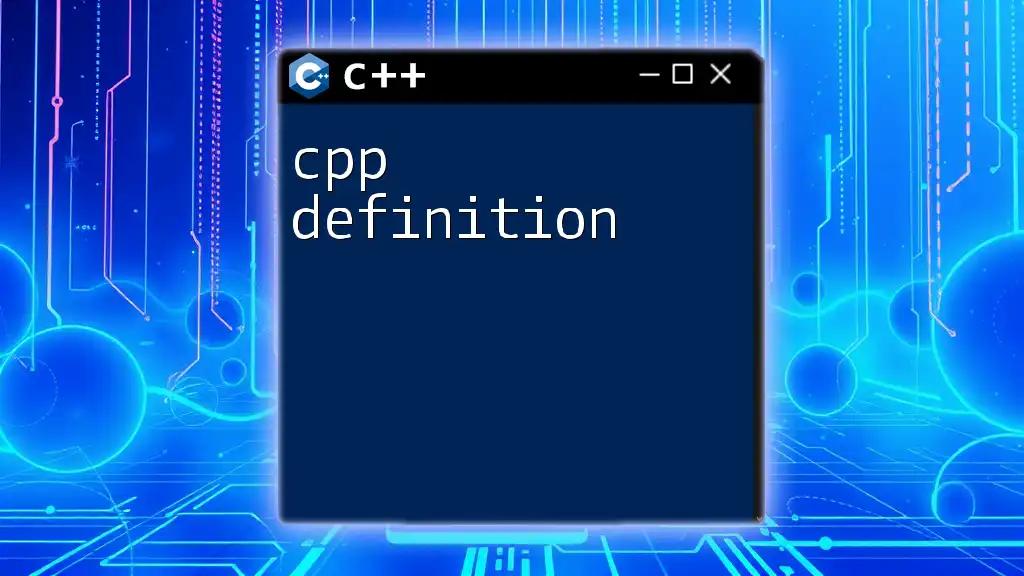
Conclusion
Understanding the definition of cpp is essential for anyone interested in programming with C++. The combination of object-oriented features, robust commands, and an extensive standard library makes it both powerful and versatile. As you explore C++, keep practicing and experimenting, and consider signing up for relevant courses to further deepen your understanding.