The `continue` statement in C++ is used inside loops to skip the current iteration and proceed to the next iteration, allowing for control over the loop's execution flow.
#include <iostream>
int main() {
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) continue; // Skip even numbers
std::cout << i << " "; // Print odd numbers
}
return 0;
}
Understanding the `continue` Statement
What is the `continue` Statement?
The `continue` statement in C++ is a control flow statement that is used within loops to skip the current iteration and move directly to the next iteration. When `continue` is encountered, the remaining code inside the loop for that iteration is bypassed, and the flow jumps back to the beginning of the loop to evaluate the loop condition again. This is particularly useful when you want to avoid executing certain parts of code under specific conditions.
Where Can `continue` Be Used?
The `continue` statement is applicable in the following types of loops:
- For Loop: Allows iteration with a specific count.
- While Loop: Continues running as long as a condition remains true.
- Do-While Loop: Similar to the while loop, but performs the code block at least once.
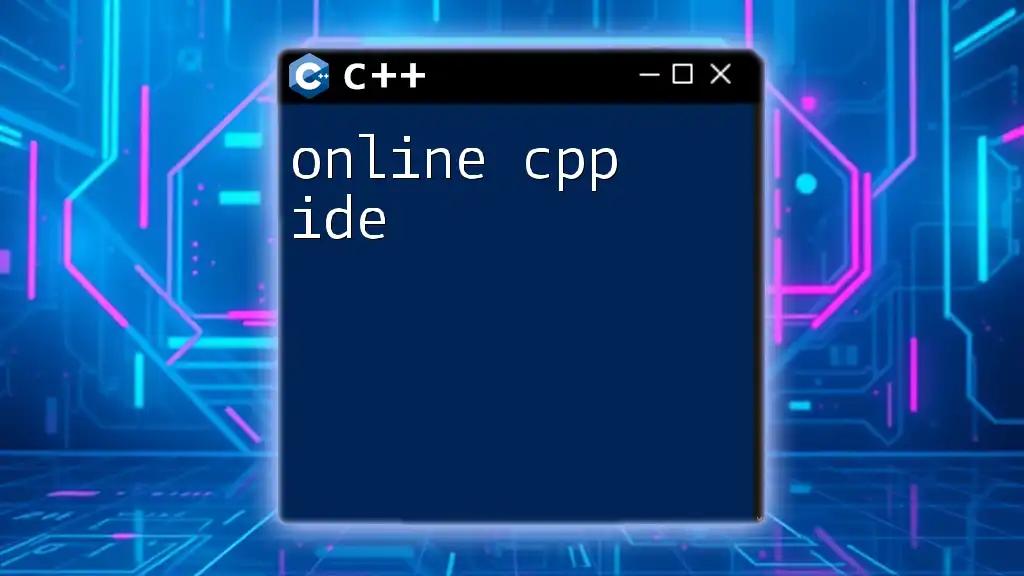
How to Use the `continue` Statement
Syntax of the `continue` Statement
The basic syntax of the `continue` statement is straightforward:
continue;
This can be used within a loop structure, and it has no arguments.
For Loop Example
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue; // Skip even numbers
}
std::cout << i << " "; // Print odd numbers
}
In this example, the `for` loop iterates from 0 to 9. The `if` condition checks if the number is even; if it is, the `continue` statement is executed, which skips the rest of the loop and goes to the next iteration. The output will be the odd numbers from 1 to 9.
While Loop Example
int i = 0;
while (i < 10) {
i++;
if (i % 2 == 0) {
continue; // Skip even numbers
}
std::cout << i << " "; // Print odd numbers
}
In this `while` loop, the loop counter `i` is incremented with each iteration. If `i` is even, the `continue` statement is called, causing the loop to skip printing. The result will be the same: odd numbers between 1 and 9.
Do-While Loop Example
int i = 0;
do {
i++;
if (i % 2 == 0) {
continue; // Skip even numbers
}
std::cout << i << " "; // Print odd numbers
} while (i < 10);
Here, the `do-while` loop ensures that the code block is executed at least once. Although the loop structure is different, the logic remains the same. The output will still print the odd numbers.
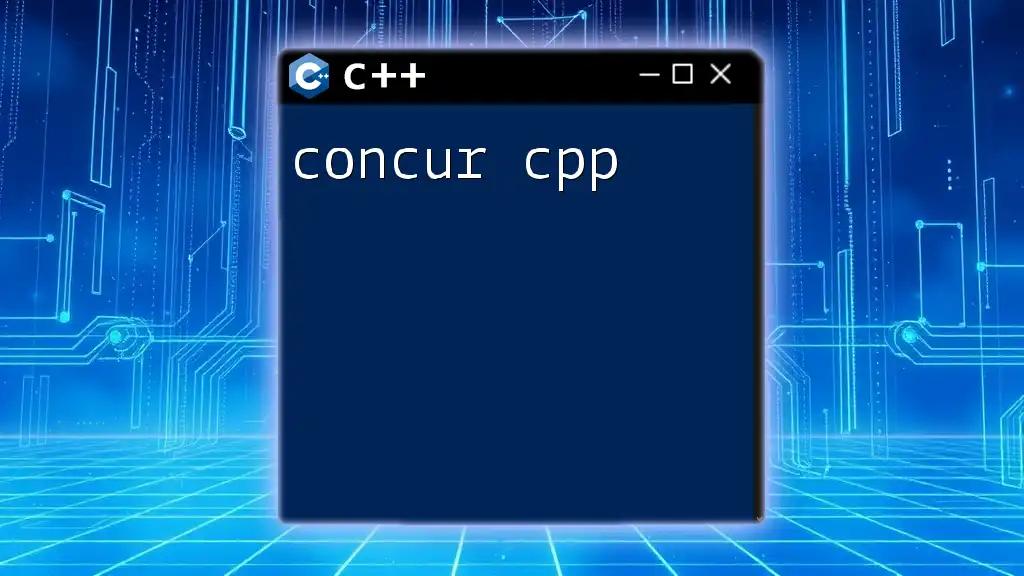
Best Practices When Using `continue`
When to Use `continue`
Using `continue` can be beneficial in many scenarios:
- When you want to skip specific iterations based on a condition without deep nesting of `if` statements.
- When simplifying logic that involves skipping over iterations, resulting in cleaner and more readable code.
When to Avoid `continue`
Although `continue` can make certain tasks easier, there are scenarios where its use might be less desirable:
- Overusing `continue` can lead to confusion about the flow of the program, especially for someone unfamiliar with the code.
- In situations where nested logic becomes complicated, it might be clearer to structure conditions without `continue`.
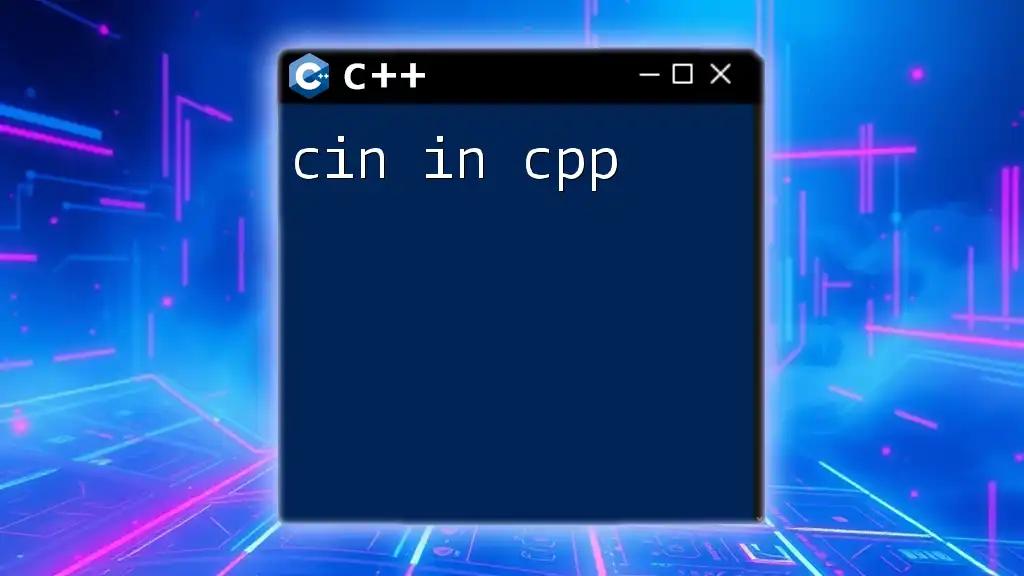
Common Mistakes with the `continue` Statement
Overusing `continue`
Excessive use of `continue` can obscure the logic of the loop. Instead of providing clarity, it can lead to a situation where the reader has to jump around the code to understand what happens in each iteration.
Misplaced `continue`
A common mistake is placing `continue` at the wrong point in the loop, leading to unintended logic errors:
for (int i = 0; i < 10; i++) {
if (i < 5) {
continue; // Incorrect logic if you want to do something for all 'i'
}
std::cout << i << " "; // This won't print numbers less than 5
}
In the example above, numbers from 0 to 4 are entirely skipped, and only numbers from 5 to 9 are printed. This clearly demonstrates how misplaced `continue` can disrupt expected output.
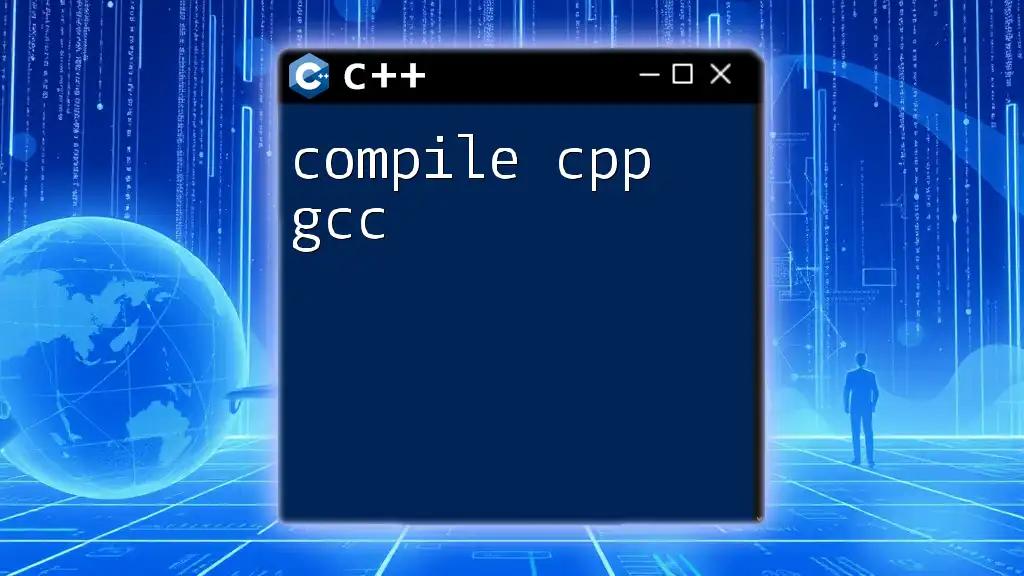
Conclusion
The `continue` statement in C++ is a powerful tool for controlling loop execution. Understanding how to employ it effectively can greatly simplify your coding logic while helping maintain clarity. By practicing its use through real-life coding scenarios, you can ensure a strong grasp of when and where to incorporate the `continue` statement into your code.
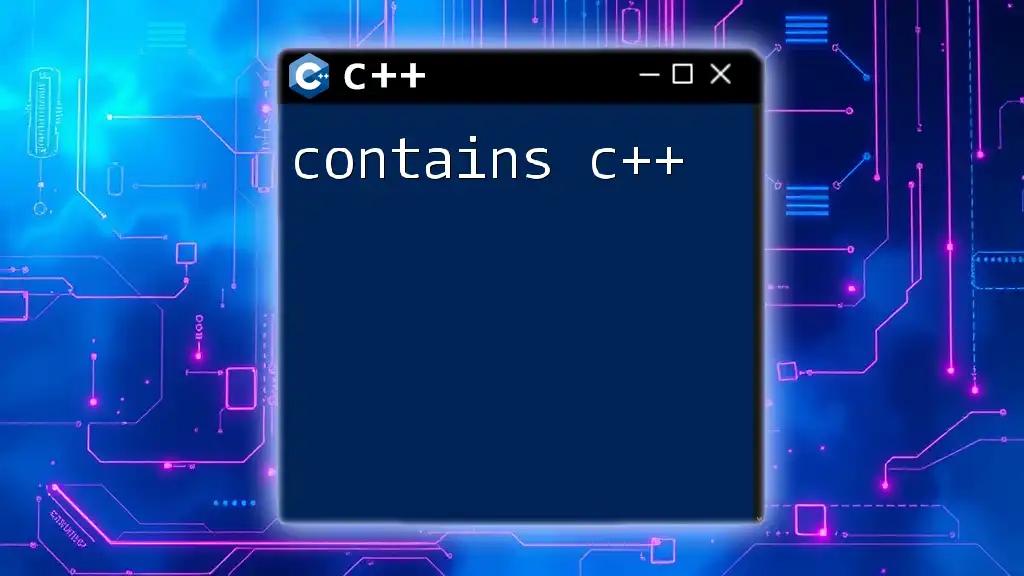
Additional Resources
To deepen your knowledge of C++ control flow statements, consider exploring the following resources:
- Comprehensive C++ reference manuals
- Advanced textbooks on C++ programming focusing on control structures

FAQs about the `continue` Statement
What happens if `continue` is used in a nested loop?
In nested loops, the `continue` statement will only affect the nearest enclosing loop. For instance:
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
if (j == 2) continue; // Only skips the current iteration of the inner loop
std::cout << "i: " << i << ", j: " << j << std::endl;
}
}
This will skip printing when `j` equals 2, but `i` will still increment.
Can `continue` be used with `switch` statements?
The `continue` statement does not directly apply within a `switch` statement. Instead, it's used as part of loop control. If you want similar behavior, consider using a combination of `break` for exiting cases and controlled flow with loops outside the `switch`.
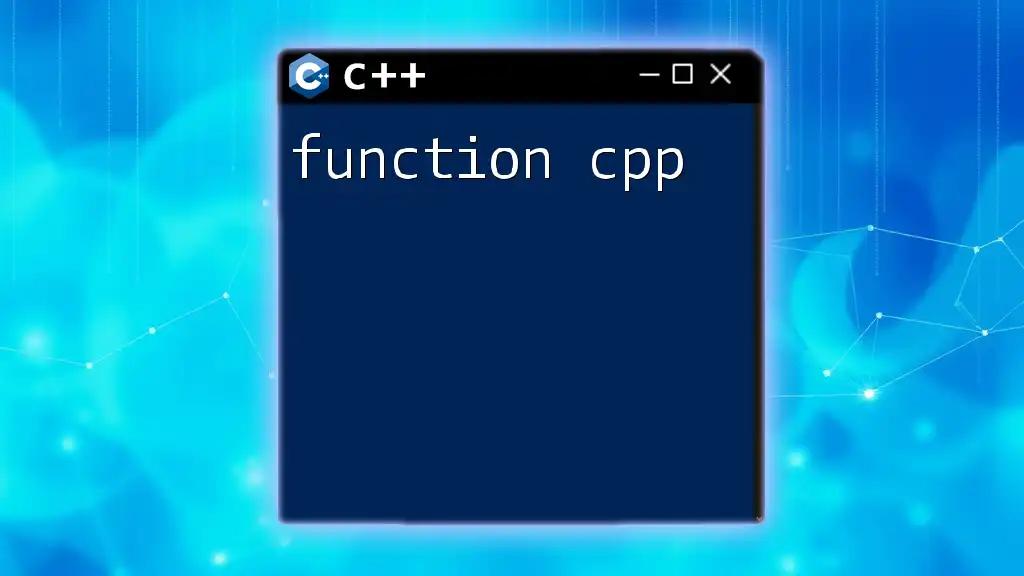
Call to Action
Now that you have a comprehensive understanding of the `continue` statement in C++, put your knowledge to the test! Try implementing `continue` in various coding exercises or existing projects. Feel free to share your experiences or questions in the comments below, fostering an environment of collaborative learning.