An online C++ IDE (Integrated Development Environment) allows users to write, compile, and execute C++ code directly in their web browser without needing to install software locally.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is an Online C++ IDE?
An online C++ IDE is a web-based environment that enables developers to write, compile, and run C++ code directly from their browsers. This flexibility eliminates the need to install any software on a local machine, significantly enhancing accessibility and convenience for programmers at all levels, from beginners to experts.
Benefits of Using Online C++ IDEs
Accessibility and Convenience
With an online IDE, users can code from anywhere, whether on a personal computer, tablet, or even a smartphone. This eliminates the constraints of needing a particular device or operating system.
No Installation Required
One of the most significant advantages is that users can jump straight into coding without the hassle of downloading and installing complex software. This is particularly valuable for beginners who may be overwhelmed by setup processes.
Collaboration Features
Many online C++ IDEs offer collaboration tools that allow multiple users to work on the same code simultaneously. This is ideal for team projects or educational settings, where peer feedback is essential for growth.
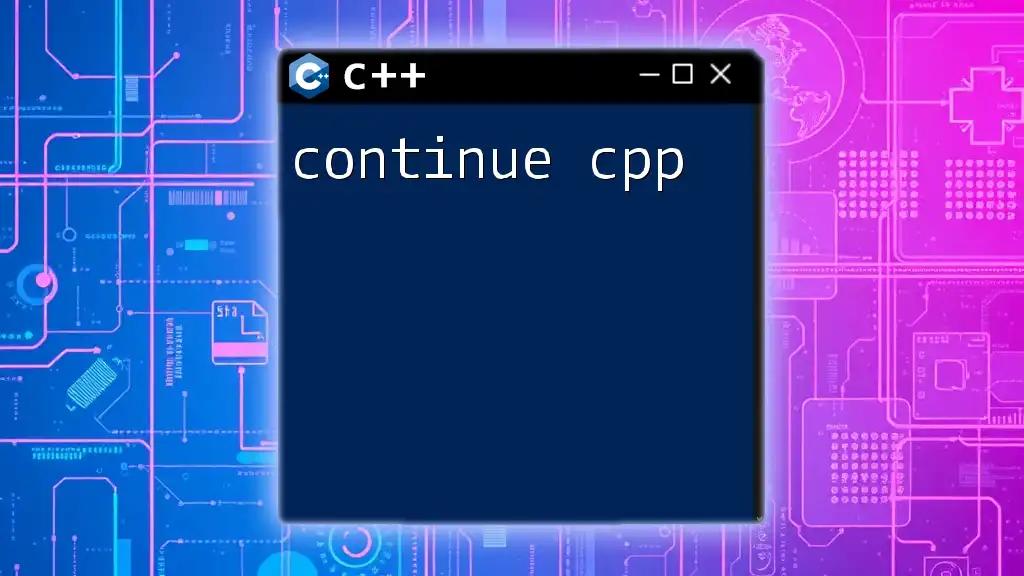
Features of an Online C++ IDE
User Interface
Clean and Intuitive Layout
Most online C++ IDEs feature user-friendly designs that make navigation straightforward for new users. These interfaces often include code editors with syntax highlighting, making it easier to read and understand code.
Code Highlighting and Formatting
Syntax highlighting is a crucial feature that helps programmers quickly identify their code structure, making it easier to spot errors or understand the flow of the program.
Code Compilation
How Does Code Compilation Work?
Online C++ IDEs compile code in the cloud. When you click the "Run" button, your code is sent to the server, where it’s compiled and executed. The output and any compile-time errors are then sent back to your browser.
Real-Time Feedback and Error Detection
Most platforms provide real-time feedback, allowing programmers to identify and fix errors as they code. This feature is instrumental for beginners who are learning C++ syntax and concepts.
Integrated Debugging Tools
Importance of Debugging in C++
Debugging is an essential part of programming that ensures code runs as expected. Effective debugging tools can save considerable time and reduce frustration during the development process.
Popular Debugging Features in Online IDEs
Many online C++ IDEs come with built-in debugging features, such as breakpoints, variable watches, and step-through execution. These capabilities make it easier to troubleshoot issues and understand code execution behavior.
Collaboration and Sharing
Overview of Collaboration Tools
Several online C++ IDEs allow users to share their code in real time. Users can invite others to edit or view their code through a simple link.
Sharing Code Snippets with Others
Most platforms enable easy sharing of code snippets. This is especially useful for educational purposes, where instructors might want to share examples or corrections with students quickly.

Best Online C++ IDEs
Overview of Popular Platforms
CPP.sh: Ideal for Quick Tests
CPP.sh is perfect for quick testing of C++ code. Its interface is minimalistic, which helps beginners focus on learning concepts rather than getting caught up in features.
Example Usage: Here’s a simple program demonstrating its capabilities:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, CPP.sh!" << endl;
return 0;
}
Repl.it: Collaboration at Its Best
Repl.it brings collaboration to the forefront, allowing multiple programmers to work on projects simultaneously. Unlike some other platforms, it supports a wide range of programming languages, making it a versatile choice.
Example Usage: To build a basic console app, you can write:
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
cout << "Sum: " << a + b << endl;
return 0;
}
JDoodle: User-Friendly Interface
JDoodle offers a clean interface with various themes, making it visually appealing. It also provides a vast library of online resources, enhancing the learning experience.
Example Usage:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to JDoodle!" << endl;
return 0;
}
OnlineGDB: Debugging Made Easy
OnlineGDB not only compiles code but also comes with advanced debugging tools, making it suitable for more complex applications. This platform is particularly beneficial for intermediate users looking to polish their skills.
Example Usage:
#include <iostream>
using namespace std;
int main() {
// Simple factorial program
int n, fact = 1;
cout << "Enter a number: ";
cin >> n;
for (int i = 1; i <= n; ++i) {
fact *= i;
}
cout << "Factorial: " << fact << endl;
return 0;
}
Ideone: Code Sharing Simplified
Ideone allows users to not only compile code but also share it easily with friends or colleagues. This platform offers community-driven features like code reviews and suggestions for improvement.
Example Usage:
#include <iostream>
using namespace std;
int main() {
cout << "Check this out on Ideone!" << endl;
return 0;
}
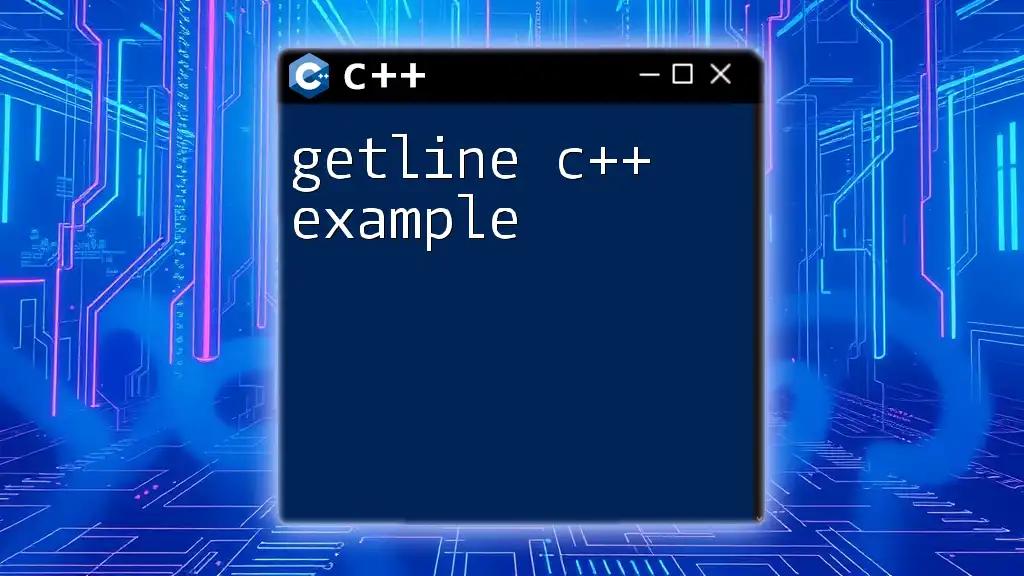
How to Choose the Right Online Compiler for C++
Consider Your Needs
When selecting an online C++ IDE, consider your specific needs. Are you coding casually, or are you engaged in serious development? Understanding your requirements will help you find the best platform suited to your workflow.
Collaboration Needs and Team Size
If you are part of a team, look for IDEs that offer strong collaboration features. If you’re working alone, a simpler tool may suffice.
Evaluating Features
Choosing Based on Real-Time Compilation Speed
Some online C++ IDEs compile faster than others. If speed is critical for your projects, you may need to test a few platforms to find the most efficient option.
Importance of Integrated Libraries and Frameworks
Consider whether the online IDE supports the libraries and frameworks you frequently use, as this will impact your development process.
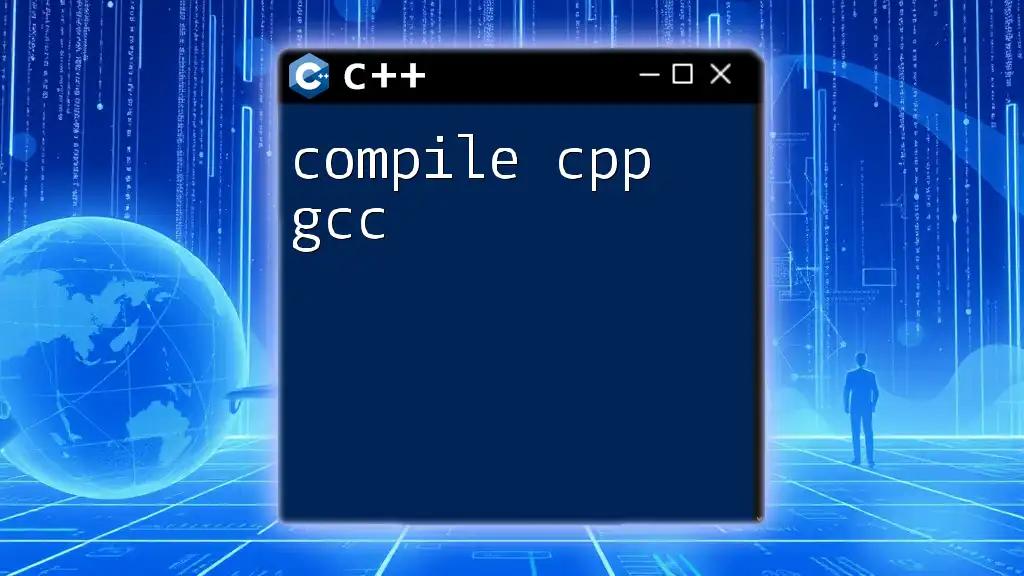
Working with a C++ Online Compiler
Getting Started
To begin using an online C++ IDE, simply navigate to the site of your choice, create an account (if needed), and select the option to create a new C++ project. Most interfaces are similar, allowing you to write and edit your code directly in the browser.
Writing Your First C++ Program
Writing your first C++ program in an online IDE is straightforward. Below is a classic “Hello, World!” example that you can type directly into your IDE:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Compiling and Running Your Code
Once your code is complete, you’ll typically find a “Run” or “Compile” button. Clicking this will compile your code on the server. You should see output or any errors in a separate console window.
Troubleshooting Common Errors
If you encounter issues, review your code for common mistakes such as missing semicolons, unmatched braces, and incorrect variable types. Most IDEs will highlight errors visually, helping you to resolve issues quickly.

Advantages of Using C++ Online Compilers
Cost-Effectiveness
Most online C++ IDEs are free, allowing you to experiment with coding without incurring costs. This contrasts with purchasing professional IDEs or local compilers.
Learning Opportunities
Real-Time Feedback and Its Importance
Online IDEs provide instant feedback, making them invaluable for learning. As you code, you can immediately see what works and what doesn’t, fostering a more effective learning cycle.
Experimenting Without Fear of Damage
Since your projects are stored in the cloud, you can tinker with code freely without the risk of damaging your local environment.
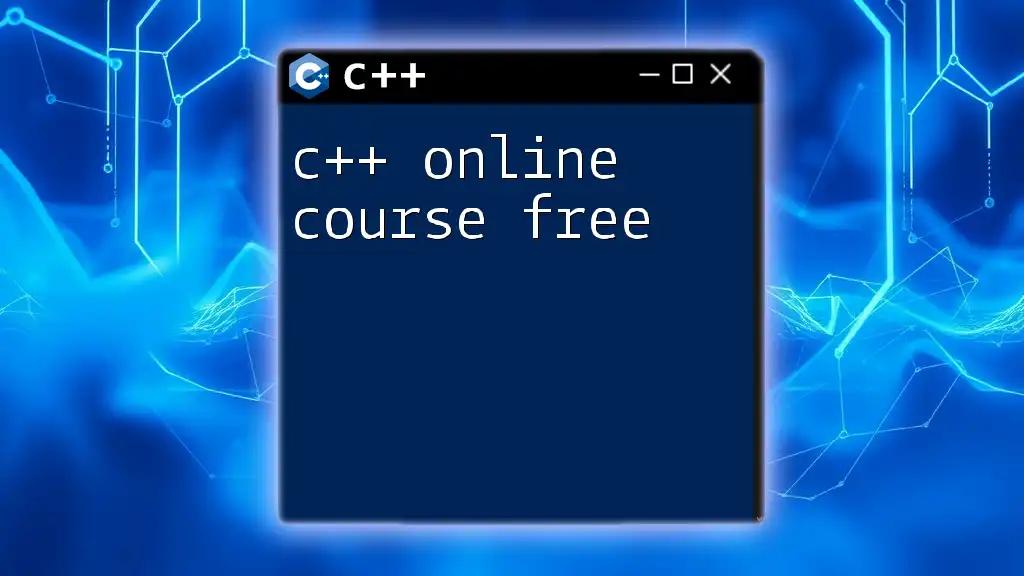
Limitations of Online C++ IDEs
Internet Dependency
Many online IDEs require a consistent internet connection, making them less reliable in areas with poor connectivity.
Performance Constraints Compared to Local IDEs
Although online IDEs are convenient, they may lack the performance and advanced features of installed applications, especially for larger projects.
Limited by Browser Capability
Some complex C++ applications may not run optimally in a browser environment due to limitations on resources compared to native compilers.
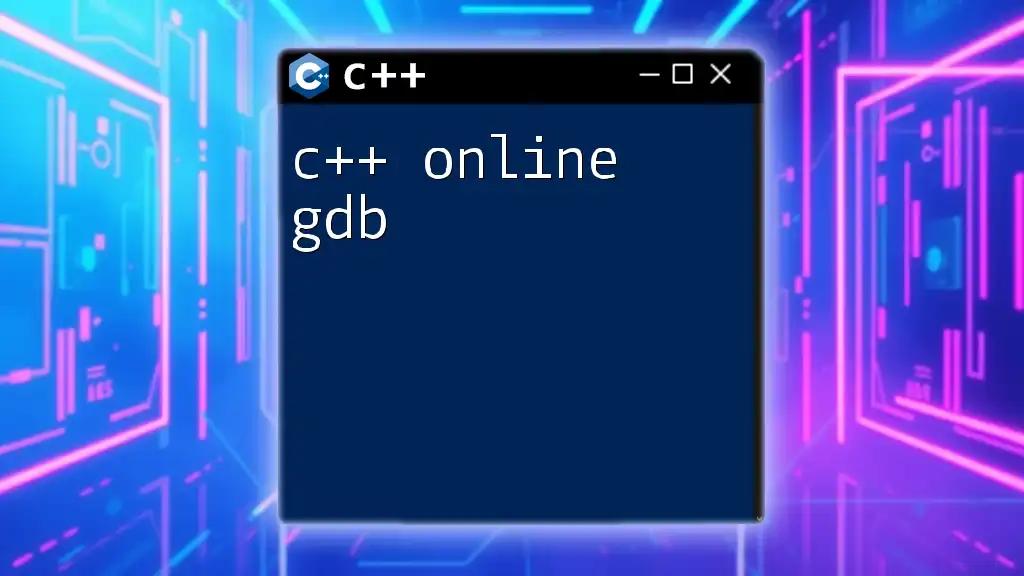
Conclusion
The landscape of online C++ IDEs continues to evolve, with unique features emerging to support various programming tasks. As you navigate this world, keep in mind the benefits and limitations. Selecting the right tool can significantly enhance your coding experience and skills.
The future of online C++ IDEs holds promise, particularly with advancements in cloud computing and collaboration tools.
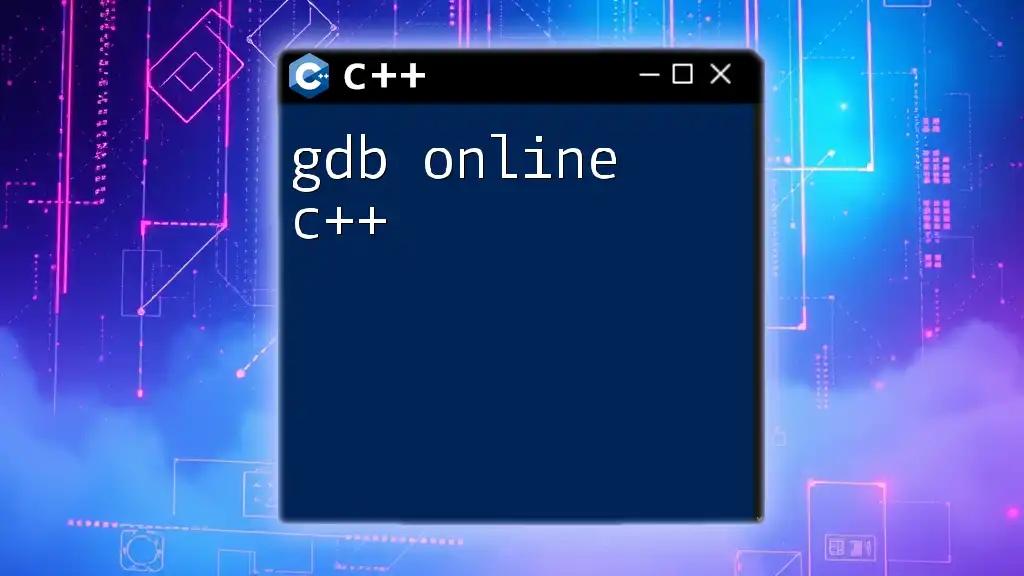
Additional Resources
To delve deeper into C++, consider exploring various online learning platforms and community forums dedicated to sharing knowledge and troubleshooting common programming challenges.
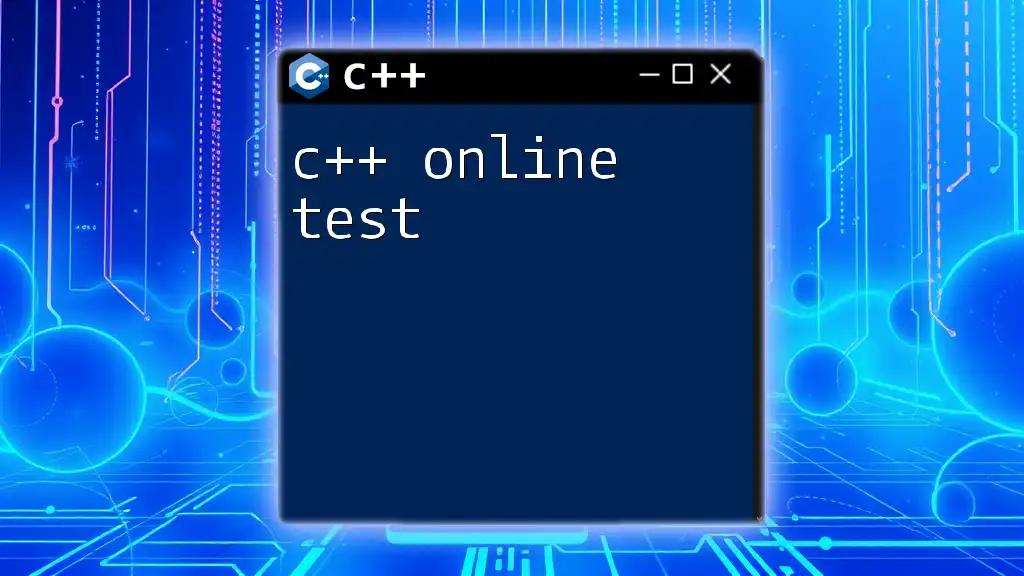
FAQs About C++ Online Compilers
What is the best free online C++ compiler?
While multiple options exist, Repl.it and JDoodle are frequently praised for their user-friendly interfaces and collaborative features.
Are online C++ IDEs suitable for full-scale projects?
For smaller projects and educational purposes, they are excellent. However, for larger projects, a local IDE may provide more robust tools.
Can I use online C++ IDEs for competitive programming?
Yes, many platforms cater to competitive programming and offer timer features and instant compilation, which are crucial in contests.
Are there any security concerns with online IDEs?
While most reputable platforms take security seriously, sharing sensitive code online can pose risks. It’s best to avoid using them for proprietary or sensitive work.