ZXing (Zebra Crossing) is an open-source library for barcode image processing that provides a simple way to encode and decode barcodes in C++. Here's a code snippet showing how to decode a barcode using ZXing in C++:
#include <zxing/qrcode/QRCodeReader.h>
#include <zxing/DecodeHints.h>
#include <zxing/Reader.h>
#include <zxing/LuminanceSource.h>
#include <zxing/MatSource.h>
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("barcode.png", cv::IMREAD_GRAYSCALE);
zxing::MatSource source(image);
zxing::qrcode::QRCodeReader reader;
zxing::Ref<zxing::Result> result = reader.decode(source, zxing::DecodeHints::QR_CODE_HINT);
std::cout << "Decoded text: " << result->getText()->getText() << std::endl;
return 0;
}
Setting Up Your Environment
Installing zxing C++
To use zxing cpp, you first need to set up your development environment correctly. Here are the prerequisites and steps to get started.
Prerequisites
Make sure you have a working C++ development environment. You will need:
- A compatible C++ compiler (such as GCC or Clang).
- Essential libraries like libjpeg and libpng if you plan to process JPEG and PNG images.
Step-by-step Installation Instructions
-
Clone the Repository: Start by cloning the zxing repository using Git.
git clone https://github.com/zxing/zxing.git cd zxing
-
Build the Project: Use CMake to configure and build the project.
mkdir build cd build cmake .. make
-
Verify the Installation: After the build process, ensure zxing is correctly installed by running any of the provided sample codes.
Project Structure
Understanding the structure of your zxing project can significantly ease the development process. The main components include:
- Core files: These contain the core logic for barcode processing.
- Image handling files: Code for input image processing and conversion.
- Examples: Sample files showing how to use zxing.
Organize your project effectively to separate the core functionality from your custom implementations.
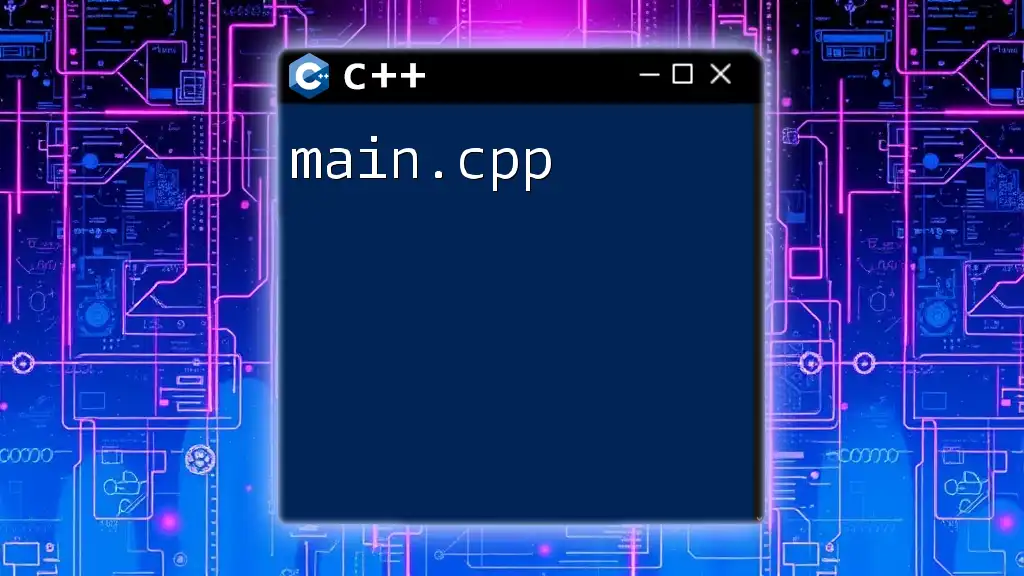
Understanding Barcode Types
Common Barcode Formats Supported by zxing
QR Codes
QR codes are two-dimensional barcodes widely used due to their efficient data storage. They can encode URLs, text, and other data types.
Data Matrix
This barcode type is popular in industrial applications, providing a compact way to store large amounts of data.
UPC and EAN
These are linear barcodes commonly found on retail products. They are simple to scan but limited in data capacity compared to QR codes.
PDF417
A multi-dimensional barcode capable of storing extensive data. It's often used in identification cards.
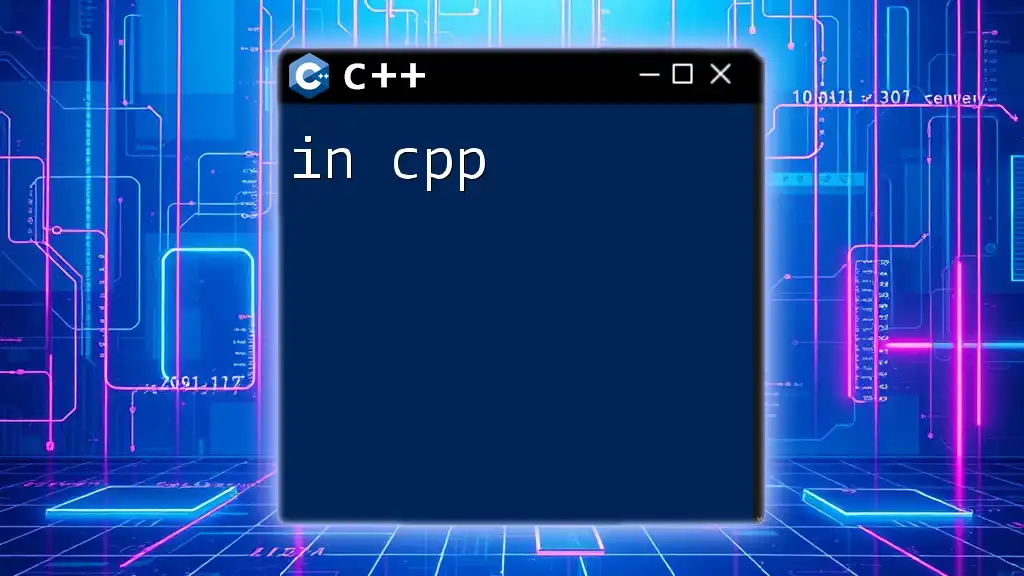
Basic Usage of zxing C++
Creating a Simple Barcode Reader
To read barcodes using zxing cpp, you only need a few lines of code. Imagine you have an image file with a QR code; here's how to read it:
#include <zxing/zxing.h>
#include <zxing/qrcode/QRCodeReader.h>
#include <zxing/DecodeHints.h>
#include <zxing/MatSource.h>
// Create a function to read QR codes from a file
std::string readQRCode(const std::string& filePath) {
// Load the image
zxing::Ref<zxing::LuminanceSource> source = zxing::MatSource::createMatSource(filePath);
zxing::Ref<zxing::BinaryBitmap> bitmap(new zxing::BinaryBitmap(zxing::Ref<zxing::GlobalHistogramBinarizer>(source)));
// Use the QR code reader
zxing::qrcode::QRCodeReader reader;
zxing::Ref<zxing::Result> result;
// Attempt to decode the barcode
try {
result = reader.decode(bitmap, zxing::DecodeHints::QR_CODE_HINT);
} catch (const std::exception& e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
return "";
}
return result->getText()->getText();
}
This code loads an image and uses zxing's QR code reader to decode it, demonstrating how straightforward it is to integrate zxing into your application.
Encoding Barcodes with zxing
Encoding a barcode is equally simple. Here's how to create a QR code from a string:
#include <zxing/qrcode/QRCodeWriter.h>
#include <zxing/EncodeHintType.h>
// Function to encode a string into a QR code
void createQRCode(const std::string& content, const std::string& filePath) {
zxing::qrcode::QRCodeWriter writer;
zxing::Ref<zxing::BitMatrix> matrix = writer.encode(content, zxing::BarcodeFormat::QR_CODE, 200, 200);
// Save the BitMatrix as an image using your preferred image library
saveBitMatrixAsImage(matrix, filePath);
}
In this example, we encode a simple string as a QR code. This illustrates how easy it is to generate barcodes using zxing.
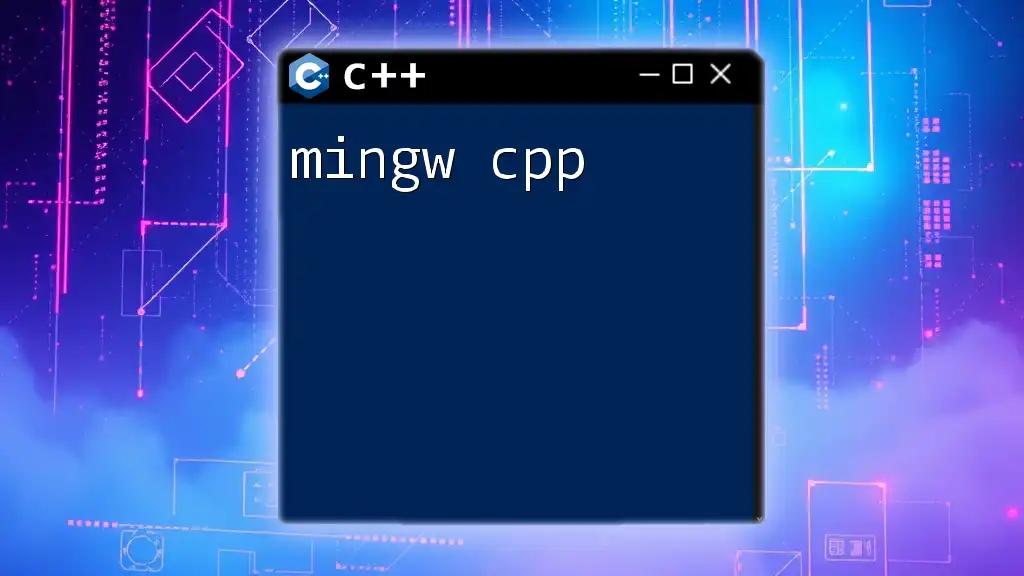
Advanced Features of zxing C++
Error Correction Capabilities
One of the standout features of zxing is its robustness in handling errors. Error correction allows barcodes to remain readable despite physical damage. zxing employs Reed-Solomon error correction, which helps recover lost information.
To utilize error correction, simply adjust the parameters in the reader as shown below:
zxing::DecodeHints hints(zxing::DecodeHints::QR_CODE_HINT);
hints.setErrorCorrectionLevel(zxing::ErrorCorrectionLevel::H); // High level of error correction
This setup can dramatically improve reading rates in adverse conditions.
Handling Different Image Formats
zxing cpp is versatile and supports several image formats. You can handle images in PNG, JPEG, and BMP formats. To convert images, consider using libraries like OpenCV or libpng. Here's a code snippet that demonstrates how to read a PNG image:
#include <opencv2/opencv.hpp>
// Function to load an image using OpenCV
zxing::Ref<zxing::LuminanceSource> loadImage(const std::string& filePath) {
cv::Mat image = cv::imread(filePath);
return zxing::MatSource::createMatSource(image);
}
This function uses OpenCV to read an image, which can then be processed by zxing methods.
Multi-code Detection
Detecting multiple codes in a single image can be achieved with slight modifications. This is particularly useful in inventory management where several products may be scanned in one go.
void detectMultiCodes(const cv::Mat& image) {
zxing::Ref<zxing::LuminanceSource> source = zxing::MatSource::createMatSource(image);
// Other necessary preparations...
// Now process to detect all codes
// Iterate over detected codes
}
While the actual implementation will require more details, this snippet gives you an idea of how to extend your application.
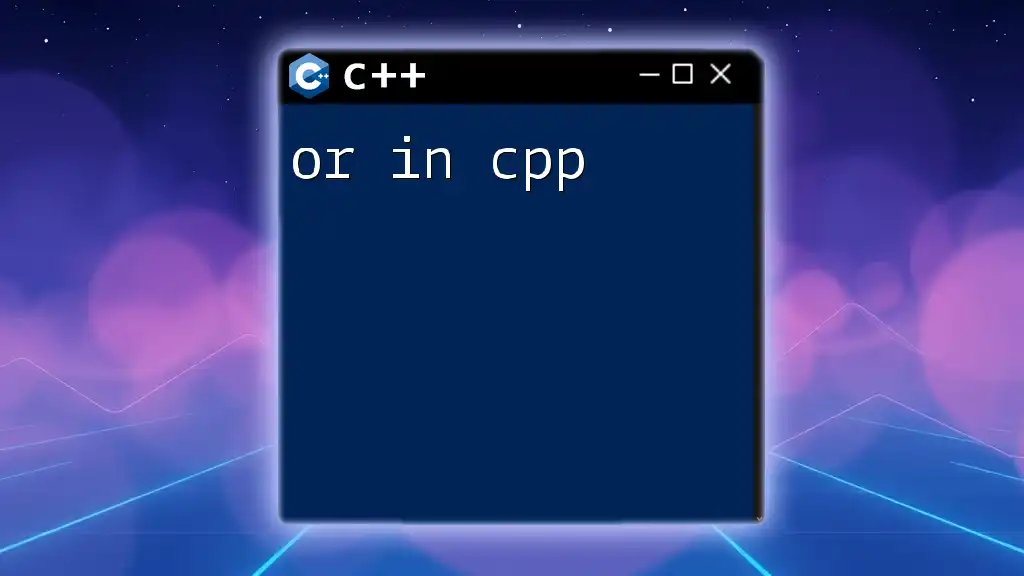
Integrating zxing C++ into Your Projects
Best Practices
When integrating zxing cpp into your projects, consider these best practices:
- Organize Your Code: Keep core functionality separate from application logic.
- Error Handling: Implement robust error handling to manage exceptions that may arise during barcode reading/writing.
- Performance Optimization: Profile your application for performance issues, especially when processing large images or multiple barcodes.
Real-world Application Examples
Implementing zxing in Mobile Applications
Using zxing in mobile apps is becoming common due to its lightweight nature. Libraries such as zxing-android can wrap around zxing cpp to provide seamless integration.
Using zxing in Web Applications
With advancements in WebAssembly, it's possible to incorporate zxing cpp into web applications, allowing users to scan barcodes directly from their browsers.
A notable case study is a retail app that increased customer interaction by enabling QR code scanning for product info, utilizing zxing cpp effectively.
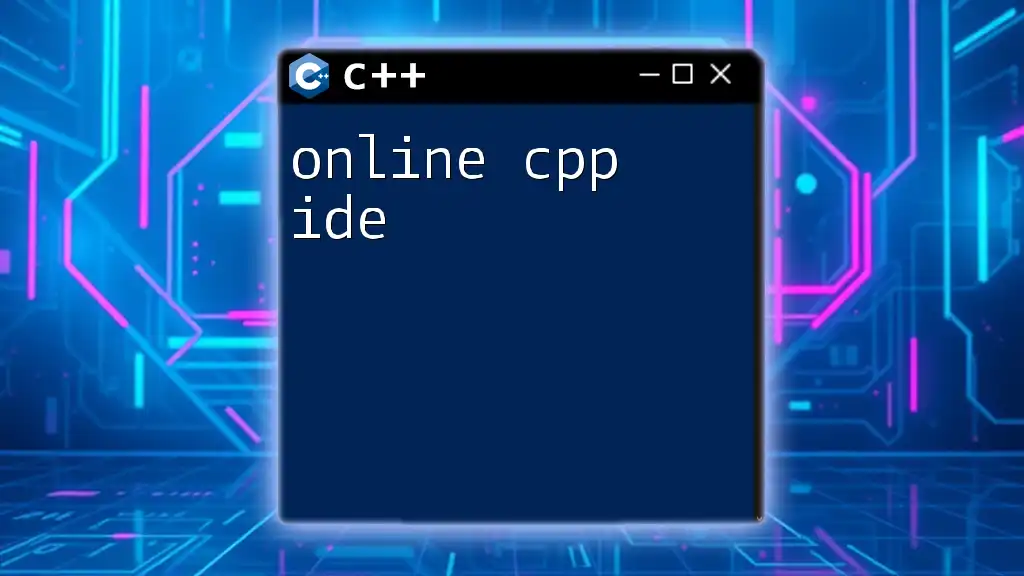
Troubleshooting Common Issues
Error Messages and Solutions
While working with zxing cpp, you may encounter various errors such as `Format Exception` or `No Result`. Common solutions include:
- Ensuring the source image is clear and without distortions.
- Checking if you have included the necessary image processing libraries.
Community Resources
The zxing community is active and provides numerous resources:
- Forums and discussion boards are good places to ask questions and share experiences.
- Contributing to zxing can enhance your understanding and provide real-time feedback on your questions. It’s an excellent way to learn from experts.
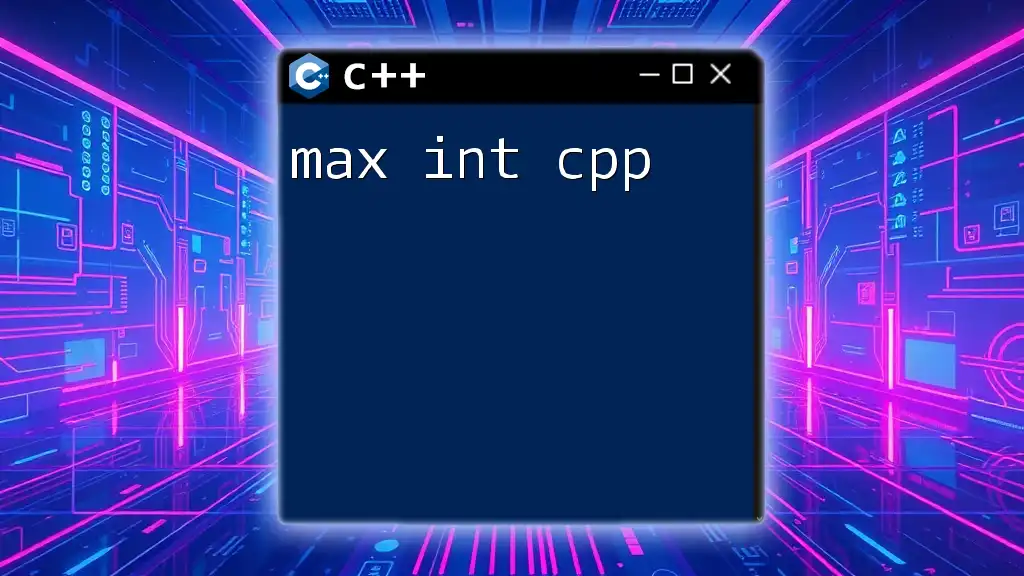
Conclusion
In conclusion, zxing cpp offers powerful capabilities for barcode processing. From simple QR code reading to advanced features like error correction and multi-code detection, zxing is a robust library suited for various applications. Experimenting with the library will enhance your understanding and strengthen your application development skills. Explore further resources and dive deep into the world of zxing to unlock its full potential.