In C++, the maximum value that an integer can hold is defined by the constant `INT_MAX`, which is part of the `<limits.h>` header file. Here's a code snippet to demonstrate this:
#include <iostream>
#include <limits>
int main() {
std::cout << "The maximum value of an int in C++ is: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
What is the Maximum Integer Value in C++?
In C++, the concept of max int cpp refers to the highest value an integer variable can hold. Understanding this maximum value is crucial for avoiding overflow errors that can lead to unexpected behavior in your programs.
An integer in C++ can be signed or unsigned. A signed integer can hold both negative and positive values, while an unsigned integer can only hold non-negative values, effectively doubling the maximum positive value it can represent.
Exploring the C++ Int Data Type
What is an int in C++?
The `int` data type in C++ is a fundamental type that is used to represent integral (whole) numbers. Integers are stored in memory as binary values, and the amount of memory allocated for an `int` depends on the system architecture (commonly 4 bytes in modern systems). This data type is used for a vast array of programming tasks—from counting iterations in loops to representing discrete values in various applications.
Signed vs Unsigned Integers
Signed integers can represent values from `-(2^(n-1))` to `(2^(n-1)-1)` where `n` is the number of bits used to store the number. Therefore, if `n=32`, the range is typically from `-2,147,483,648` to `2,147,483,647`.
On the other hand, unsigned integers extend the positive range, where they can hold values from `0` to `2^n - 1`. This is perfect for situations where negative values are not needed, such as counting items or indexing.
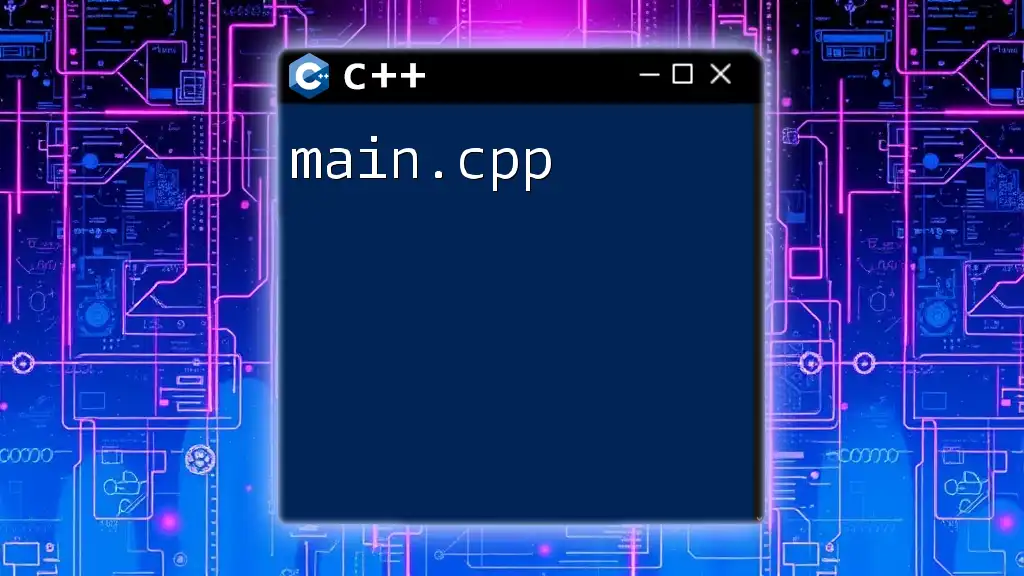
How to Determine the Maximum Integer Value
Using `limits` Header
In C++, the `<limits>` header provides a standardized way to query the properties of fundamental data types, including their maximum and minimum values. To access the maximum integer value, you can use the `std::numeric_limits` class.
Here’s how you can fetch the maximum integer value in C++:
#include <iostream>
#include <limits>
int main() {
std::cout << "Maximum value for int: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
This snippet outputs the maximum value that an `int` can hold, which is `2,147,483,647` in most systems. Understanding this limit helps prevent errors related to variable overflow.
Explanation of `std::numeric_limits`
The `std::numeric_limits` template class provides a wealth of information about data types. With it, you can easily access properties like the smallest and largest values a type can represent. It's a reliable way to ensure that your code adheres to the limits imposed by the C++ standard, making your code more portable and robust.
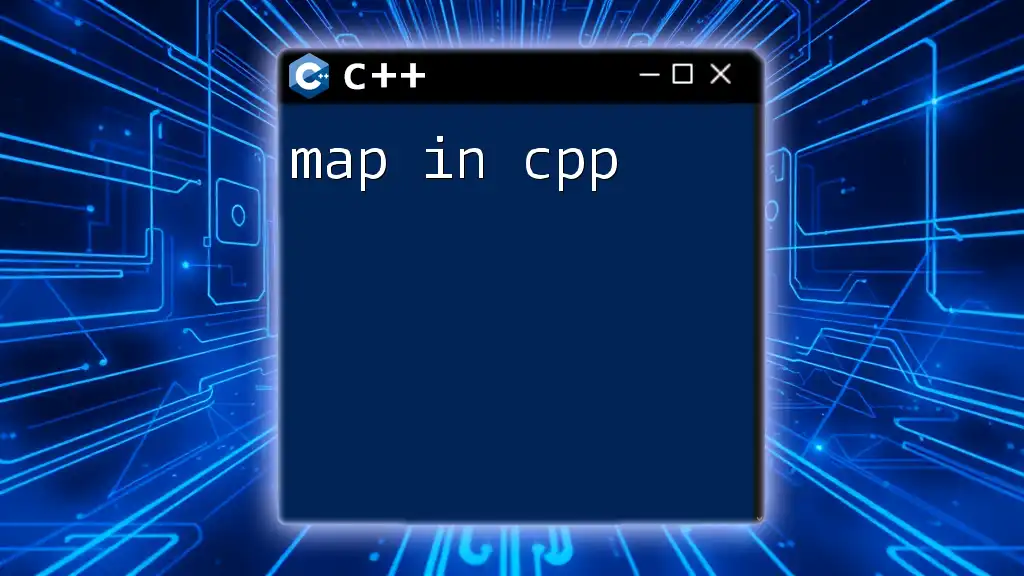
Visualizing Max Int Value
Memory Representation of Max Int
An `int` is stored in a computer’s memory as a series of binary digits (0s and 1s). For a 32-bit integer, the maximum value, `2,147,483,647`, is represented as `01111111 11111111 11111111 11111111`. Understanding this binary representation helps to visualize how numbers are stored and processed in memory.
Real-World Examples of Using Max Int
In practical software development, being aware of the maximum integer value is vital. For instance, if you are counting items or processing data in loops, you need to ensure that your variables do not exceed the maximum limit, thereby avoiding pitfalls like overflow errors.
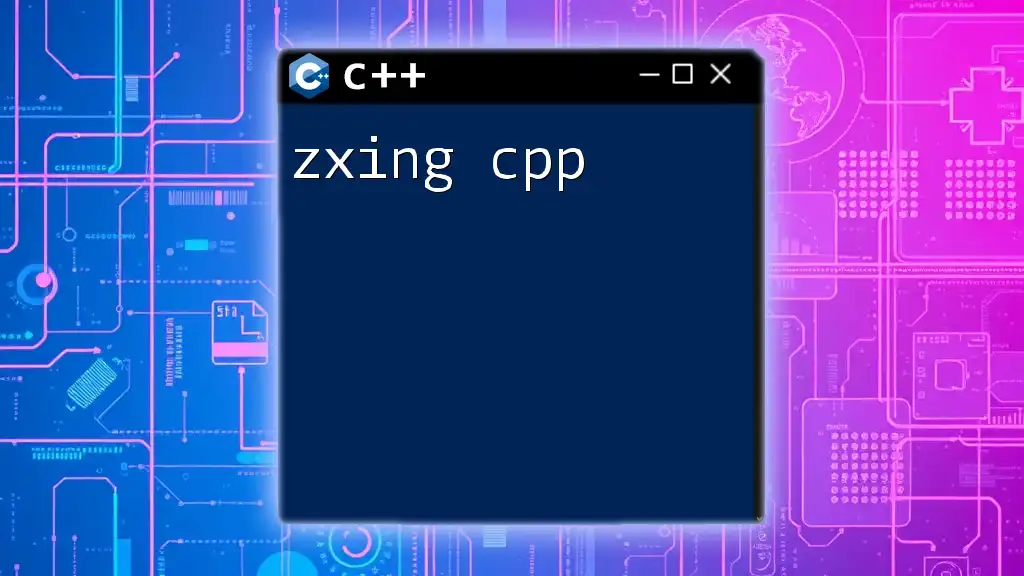
Common Use Cases for Max Int in C++
Loop Limitations
Using the maximum integer value as a boundary condition in loops is a common scenario. If your loop counter exceeds the maximum `int` value, it will wrap around to a negative number, causing premature termination or an infinite loop.
Here’s an example:
for (int i = 0; i < std::numeric_limits<int>::max(); ++i) {
// loop code
if (i == 10) break; // Breaking for demonstration
}
In this example, we set a loop limit using the maximum integer value to ensure we remain within the acceptable range of integers.
Graph Algorithms
In algorithms, such as Dijkstra's for the shortest path, using `max int cpp` can help signify the maximum weight or value when initializing distances. It is crucial for correctly implementing the algorithm.
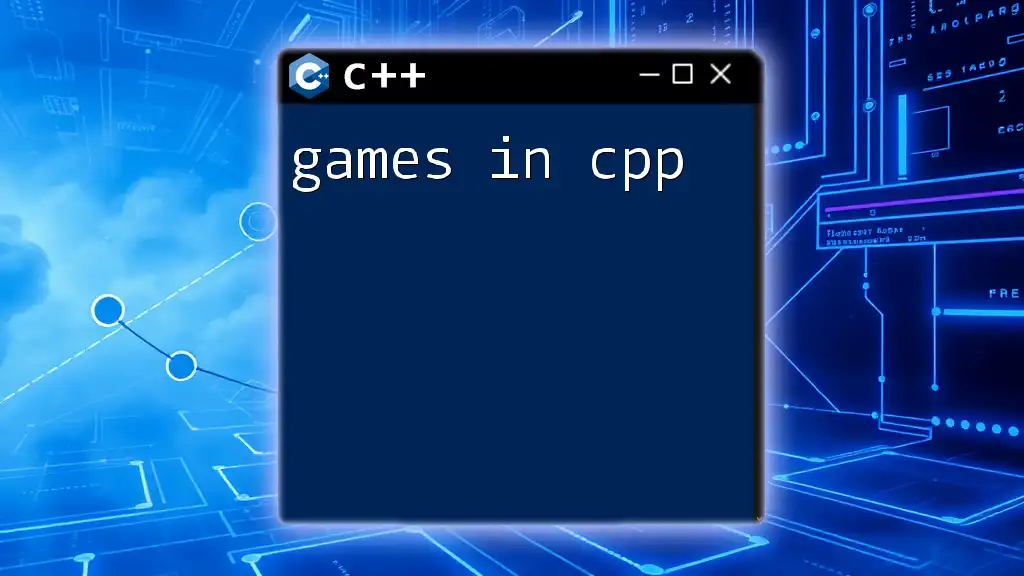
Handling Integer Overflows
Understanding Overflows
An integer overflow occurs when you try to store a value that exceeds the range that a data type can represent. This often leads to incorrect calculations and erratic program behavior, which can be detrimental in critical applications.
Techniques to Prevent Overflows
To prevent overflows, one effective strategy is to use larger data types. For example, switching to `long long` can significantly widen the range for integer values.
Here's a simple safety check for addition to avoid overflow:
#include <limits>
void safeAdd(int a, int b) {
if (a > 0 && b > std::numeric_limits<int>::max() - a) {
// Handle overflow condition
std::cout << "Overflow will occur!" << std::endl;
} else {
// safe to add
int result = a + b;
std::cout << "Result: " << result << std::endl;
}
}
In this code, we check whether adding `a` and `b` would exceed the maximum value representable by an `int`, allowing for proactive detection of potential overflow scenarios.
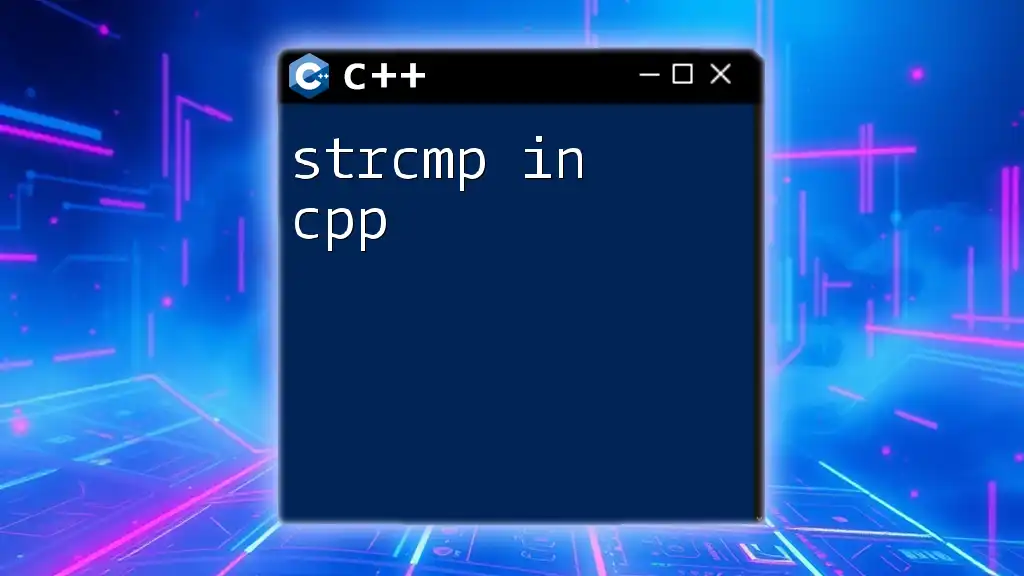
Conclusion
Understanding the max int cpp is essential for programmers, especially those working with performance-intensive applications or algorithms. By leveraging the capabilities of `std::numeric_limits`, being mindful of data types, and implementing strategies to handle potential overflow scenarios, developers can create more robust and reliable C++ programs.
FAQs about Max Int in C++
What is the maximum value of an int in C++?
The maximum value of an `int` in C++ is typically `2,147,483,647` for a signed 32-bit integer. This can be confirmed using the `<limits>` header.
Can I use max int in C++ for all data types?
The maximum value concept applies specifically to integer data types. Other data types, such as floating point or character types, have their respective maximum values.
How do I switch from int to long long in C++?
To switch from `int` to `long long`, simply declare your variable as `long long` instead of `int`. This enables the variable to handle much larger values (often up to `9,223,372,036,854,775,807` for `long long`).