In C++, the `if` statement allows you to execute a block of code conditionally, based on whether a specified expression evaluates to true.
Here's a simple code snippet demonstrating the use of an `if` statement in C++:
#include <iostream>
int main() {
int number = 10;
if (number > 5) {
std::cout << "Number is greater than 5." << std::endl;
}
return 0;
}
What is an If Statement in C++?
An `if` statement is a fundamental control structure in C++ that allows for conditional execution of code. By evaluating a specified condition, the program can decide whether to execute the subsequent block of code or skip it. This ability is crucial for making decisions within your programs.
Example of a Simple If Statement:
int number = 10;
if (number > 5) {
cout << "Number is greater than 5";
}
In this example, since the condition `number > 5` evaluates to true, the output will confirm that the number meets the specified criteria.
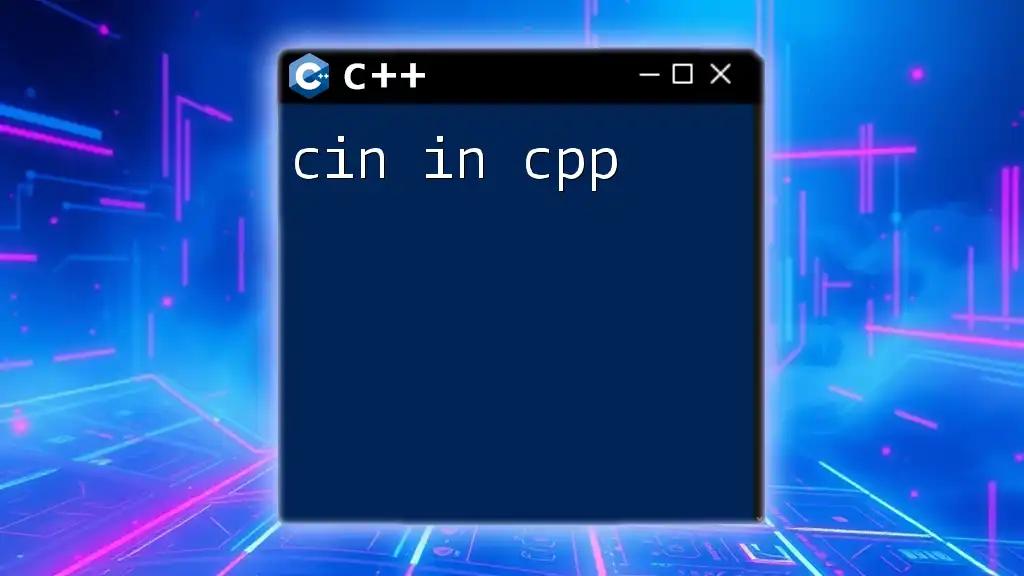
The Syntax of If Statements in C++
Understanding the structure is key when working with `if` statements in C++. The syntax for an if statement in C++ follows this basic form:
if (condition) {
// code to execute if condition is true
}
- The condition is placed within parentheses, and it's evaluated as either true or false.
- If true, the code block inside the curly braces `{}` is executed. If false, it is skipped.
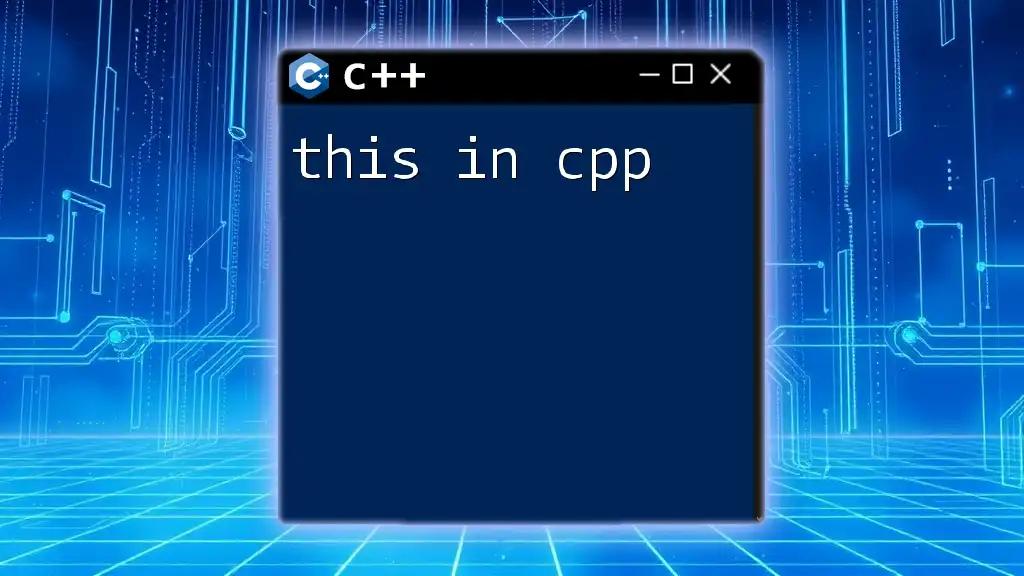
Types of If Statements in C++
Basic If Statement
The basic if statement is the simplest form of conditional logic. It evaluates a single condition and executes the code block if the condition is true.
Example of a Basic If Statement:
int num = 3;
if (num > 0) {
cout << "Positive number";
}
In this scenario, the condition checks if `num` is greater than zero. Since it is, the message "Positive number" will be printed.
If-Else Statement
The if-else statement expands your options by allowing an alternative path for execution when the condition is false. This is particularly useful when you need to perform two mutually exclusive actions.
Example of an If-Else Statement:
int num = 4;
if (num % 2 == 0) {
cout << "Even number";
} else {
cout << "Odd number";
}
Here, if `num` is even, it prints "Even number". If not, it outputs "Odd number".
If-Else If Ladder
When dealing with multiple conditions, the if-else if ladder is your go-to structure. This allows you to evaluate several conditions in a sequential manner.
Example of an If-Else If Ladder:
int score = 85;
if (score >= 90) {
cout << "Grade A";
} else if (score >= 80) {
cout << "Grade B";
} else {
cout << "Grade C";
}
In this grading system, the code checks the score against different thresholds and assigns a corresponding grade.
Nested If Statements
Nested if statements are used when you require a decision structure inside another decision. This can help manage complex logical flows.
Example of Nested If Statements:
int income = 50000;
int creditScore = 700;
if (income > 30000) {
if (creditScore > 650) {
cout << "Eligible for loan";
} else {
cout << "Not eligible due to credit score";
}
} else {
cout << "Not eligible due to income";
}
In this example, eligibility for a loan is determined by evaluating both income and credit score conditions.

Common Use Cases for If Statements
Control Flow: If statements play a pivotal role in controlling the execution flow of your program. They help in running different blocks of code based on user input or other dynamic factors.
Input Validation: If statements are frequently used for validating user inputs. By checking conditions, you can ensure that the input meets required criteria before processing it.
Gaming: In game development, if statements manage various game states based on player actions, allowing for responsive gameplay.
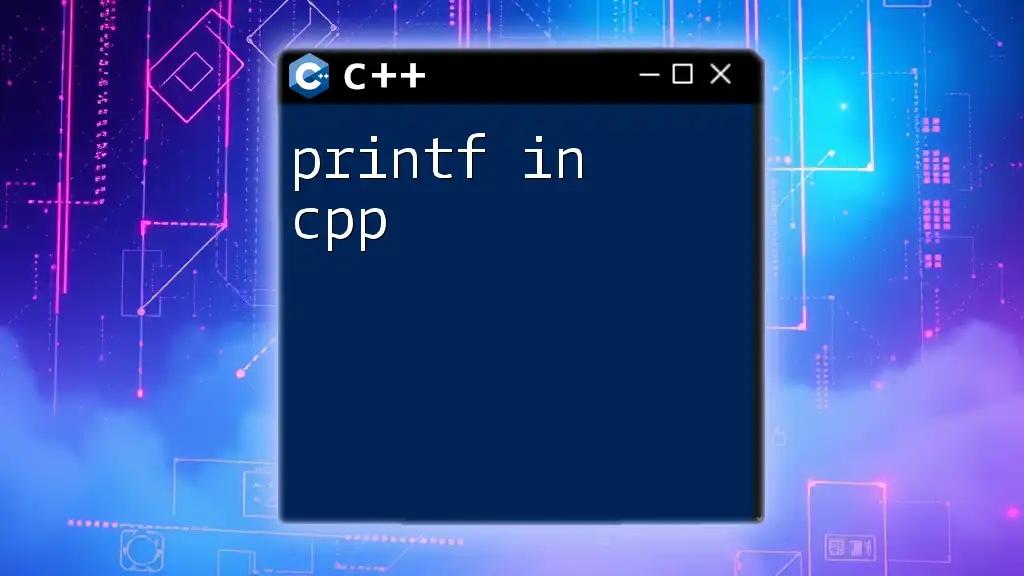
Best Practices for Using If Statements in C++
Clarity and Readability
When writing if statements, clarity and readability should always be a priority. Use descriptive variable names, and structure your conditions simply to enhance understanding.
Avoiding Complex Conditions
It's best to avoid overly complex conditions within a single if statement, as this can lead to confusion. Whenever possible, break complex logic into simpler checks or use additional variables to enhance clarity.

Performance Considerations
While if statements are a powerful feature, unnecessary checks can degrade performance, especially in large-scale applications. Optimize conditions where possible. Prioritize common or highly likely conditions first to improve efficiency and minimize execution time.

Conclusion
In summary, the `if` statement in C++ is an essential tool for controlling program flow. Recognizing its various forms—from basic to nested—and understanding how to apply them effectively is crucial for developing robust C++ applications. As you practice implementing conditional logic, you'll find that it enhances your programming skills significantly.
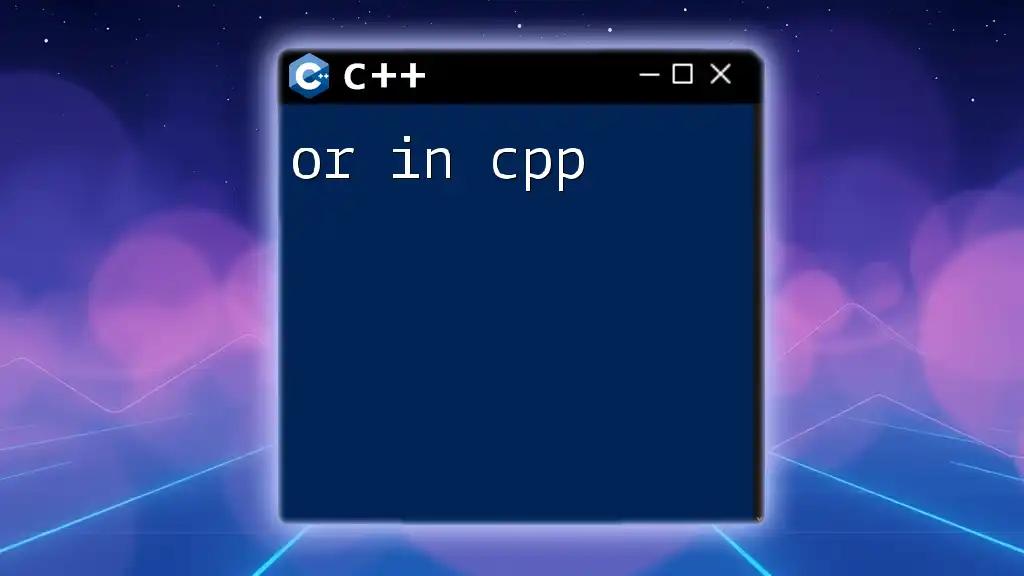
Call to Action
Now that you have a thorough understanding of if statements in C++, it's time to apply this knowledge! Try incorporating if conditions in your personal projects or coding exercises. The more you practice, the more comfortable you'll become with C++'s decision-making capabilities.

Additional Resources
To deepen your understanding, consider referring to the official C++ documentation for precise definitions and extensive examples. Additionally, exploring books on C++ programming and engaging in online courses can further bolster your skills. Participate in community forums or coding challenge platforms to pose questions and improve through real-world examples.