File I/O in C++ allows you to read from and write to files using streams, enabling data persistence and manipulation beyond the runtime of a program.
Here’s a simple example of reading from and writing to a file in C++:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
outFile << "Hello, world!" << std::endl;
outFile.close();
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
What is File I/O?
File I/O, or file input/output, refers to the processes of reading from and writing to files in a programming environment. It allows programs to store data persistently, enabling data retrieval and manipulation over time. Effective file handling is crucial for applications that require data retention, such as databases, logging systems, and configuration management.
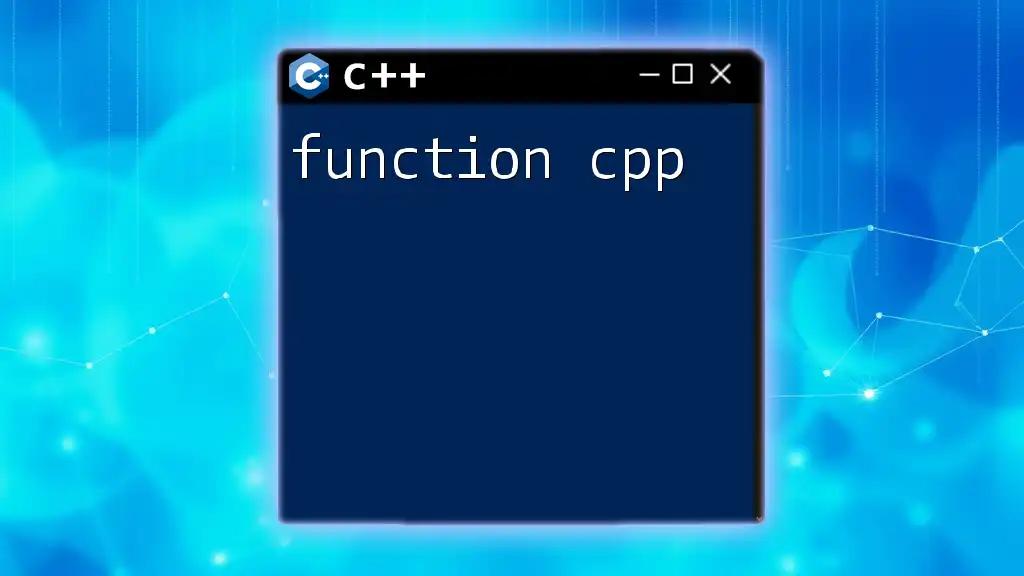
Overview of C++ File I/O
C++ provides a robust framework for file handling through its standard library. The language's versatility allows developers to manage files with ease, making it a popular choice for applications involving data storage. Common use cases include:
- Saving user settings.
- Logging application events.
- Reading configuration files.
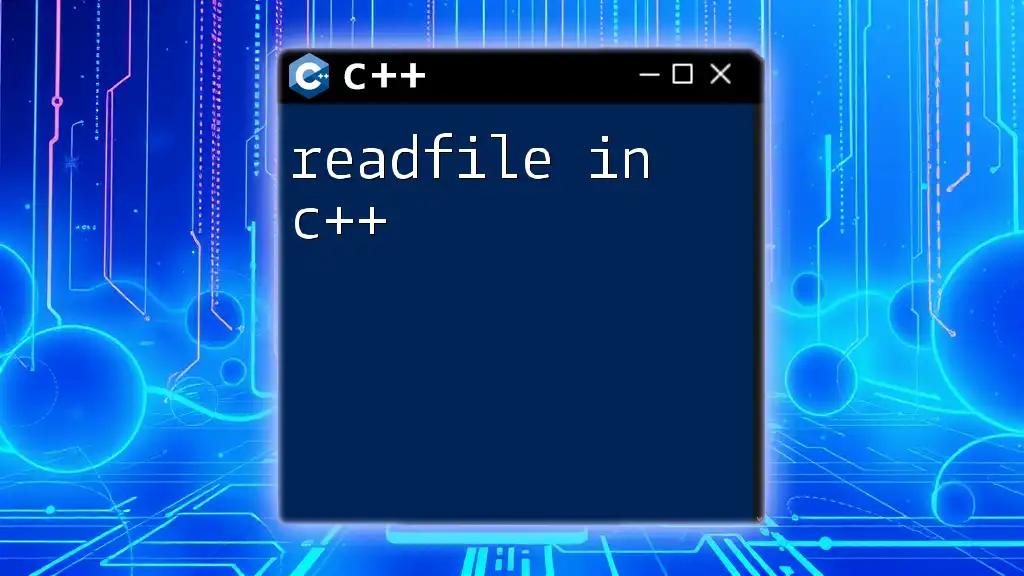
Understanding C++ File Streams
What are File Streams?
In C++, file streams are the means through which data is read from or written to files. The `<fstream>` library, part of the C++ standard library, includes three main components:
- `ifstream`: Stands for "input file stream" and is used for reading data from files.
- `ofstream`: Stands for "output file stream" and is used for writing data to files.
- `fstream`: Combines both functionalities, allowing input and output operations on the same file.

Opening and Closing Files
How to Open a File
To perform file I/O, the first step is to open a file. This can be achieved using constructors or member functions of the file stream classes. Here is a basic syntax for opening a file:
std::ifstream inputFile("example.txt");
std::ofstream outputFile("output.txt");
Error Handling while Opening Files
It’s essential to check if a file opens successfully to avoid unexpected behavior. You can do so using the `is_open()` method:
if (!inputFile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
}
Closing a File
After completing file operations, closing the file is crucial. This action frees up system resources and ensures data is properly written and flushed. Use the `close()` method as shown below:
outputFile.close();

Reading from a File
Methods to Read Data
In C++, there are several methods for reading data from a file. The most common ones include:
Using `getline()` to Read Lines
The `getline()` function is effective for reading an entire line from a file and is especially useful for text files. Here’s an example:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
Reading Formatted Data using the `>>` Operator
The extraction operator (`>>`) can read formatted data types directly from files, such as integers and strings. Here is a quick example:
int age;
inputFile >> age;
Error Handling while Reading
When reading from files, always be prepared to encounter potential errors. Use the `fail()` method to check the status of the input stream. An example to handle this gracefully is:
if (inputFile.fail()) {
std::cerr << "Error reading from file!" << std::endl;
}

Writing to a File
Writing Data to a File
Writing data to files in C++ involves using the output stream. The output stream can write text or binary data. Here’s how to write text:
outputFile << "Hello, World!" << std::endl;
Keep in mind that formatting can also be achieved using manipulators, which control output appearance, such as setting precision for floating-point numbers.
Appending Data to a File
When you want to add data without overwriting existing content, it's vital to open the file in append mode. You can do this by using the `std::ios::app` flag while opening the file:
std::ofstream outputFile("output.txt", std::ios::app);
This ensures that new content is appended to the end of the file.

Advanced File I/O Techniques
Binary File I/O
Reading and writing binary files differ from text files primarily in how data is represented. Binary files store data in a format that is more compact and faster to read/write but is less human-readable.
Here’s an example of writing and reading binary data:
std::ofstream binaryFile("data.bin", std::ios::binary);
int number = 42;
binaryFile.write(reinterpret_cast<char*>(&number), sizeof(number));
Serializing and Deserializing Data
Serialization involves transforming a data structure into a format suitable for storage. In C++, this often entails converting class or struct objects into a byte format for storage in files.
For example, consider the following struct:
struct Data {
int id;
std::string name;
};
To store instances of this struct, you’d have to handle the `std::string` carefully, writing its size followed by the characters themselves to ensure proper reading back into a program.

Best Practices for File I/O in C++
Resource Management
Always ensure adequate management of file resources to prevent memory leaks. Keep track of all files that are opened and make sure to close them appropriately.
Performance Considerations
To optimize performance, read and write data in large blocks when possible, rather than doing it byte by byte. This practice can drastically reduce the number of I/O operations and enhance overall efficiency.
Cross-Platform Compatibility
Understand the differences in file handling across operating systems, especially concerning file paths and permissions. Always use the appropriate methods to handle these differences to ensure your code works seamlessly across different platforms.

Conclusion
In summary, mastering file I/O in C++ opens up a vast array of possibilities for data management in applications. By utilizing file streams, understanding how to read and write data effectively, and following best practices, programmers can develop robust solutions that meet user needs. Your best approach now is to practice these concepts, implement your own examples, and explore the various functionalities that C++ offers for file manipulation.

Additional Resources
Your learning journey can be further enriched by exploring recommended books, online tutorials, or engaging with communities that share insights and experiences in C++ programming. Don’t hesitate to participate in forums or discussion groups where you can seek guidance and share your progress with fellow learners.