In C++, the `sizeof` operator is used to determine the size, in bytes, of a data type or object, which can be particularly useful for memory allocation and optimization.
Here's an example of how to use the `sizeof` operator:
#include <iostream>
int main() {
int myInt;
std::cout << "Size of int: " << sizeof(myInt) << " bytes" << std::endl;
return 0;
}
What is the `sizeof` Operator in C++?
The `sizeof` operator in C++ is a key tool for developers to determine the size, in bytes, of data types, variables, or even user-defined types like structures and classes. It's essential for understanding how much memory is allocated for variables and managing memory effectively in your applications.
Definition of `sizeof`
The `sizeof` operator returns the size, in bytes, of a variable or data type at compile time. It evaluates the data type or object and gives you the exact number of bytes consumed in memory. This can be particularly useful when dealing with low-level programming where memory resources need to be carefully managed.
Syntax of `sizeof` in C++
The usage of `sizeof` is straightforward, and it follows this basic syntax:
sizeof(type)
sizeof(variable)
This syntax allows you to query the size of built-in data types, user-defined types, and even arrays.

The `sizeof` Operator in Action
Using `sizeof` with Fundamental Types
Understanding how much space fundamental data types occupy is crucial. For instance:
int a;
std::cout << "Size of int: " << sizeof(a) << " bytes" << std::endl;
When you run this code, it typically outputs `Size of int: 4 bytes` on most systems, reflecting that an `int` usually occupies 4 bytes of memory. However, this can vary based on the system architecture and compiler used.
Using `sizeof` with User-Defined Types
User-defined types, such as structures and classes, often require special attention due to potential alignment and padding. Consider the following example with a structure:
struct MyStruct {
int a;
double b;
};
std::cout << "Size of MyStruct: " << sizeof(MyStruct) << " bytes" << std::endl;
Running the above code may yield a size that is greater than the sum of its members due to padding introduced to align the data appropriately in memory. It’s vital to understand these nuances as they can affect performance and memory usage in your applications.

The `sizeof` Function vs. `sizeof` Operator
What is the `sizeof` Function in C++?
While commonly referred to as an operator, `sizeof` can also be viewed as a function due to its syntax. However, it doesn’t behave exactly like traditional functions in C++. The `sizeof` operator calculates the size of the object or type at compile time rather than at runtime.
Examples of Using `sizeof` as a Function
Here's how you can use `sizeof` effectively with arrays:
int arr[10];
std::cout << "Size of array: " << sizeof(arr) << " bytes" << std::endl;
The output here would be `Size of array: 40 bytes` if an `int` is 4 bytes, emphasizing that it calculates the total size allocated to the entire array.
When to Use `sizeof` Function and Operator
When deciding to use `sizeof`, keep in mind that you are generally working with the operator's capabilities. It simplifies memory calculations and helps prevent common errors related to manual size determinations.

Practical Applications of `sizeof` in C++
Memory Management
Understanding the size that different types consume in memory is crucial for efficient memory management, especially when dynamically allocating memory. For example:
int* pArray = new int[10];
std::cout << "Size allocated: " << sizeof(int) * 10 << " bytes" << std::endl;
This snippet clarifies how much memory you plan to allocate. Properly managing this allocation can help prevent memory leaks and optimize program efficiency.
Arrays vs. Pointers
A crucial distinction in using `sizeof` is between arrays and pointers. Arrays have a fixed size, while pointers allow for dynamic memory assignment. Consider this example:
int arr[5];
int* ptr = arr;
std::cout << "Size of array: " << sizeof(arr) << std::endl; // Size in bytes
std::cout << "Size of pointer: " << sizeof(ptr) << std::endl; // Size of pointer variable
The output will show that `sizeof(arr)` would give you the total size of the array, whereas `sizeof(ptr)` would yield the size of the pointer to the first element, typically 4 or 8 bytes depending on your system architecture. This distinction is vital for effective memory management.
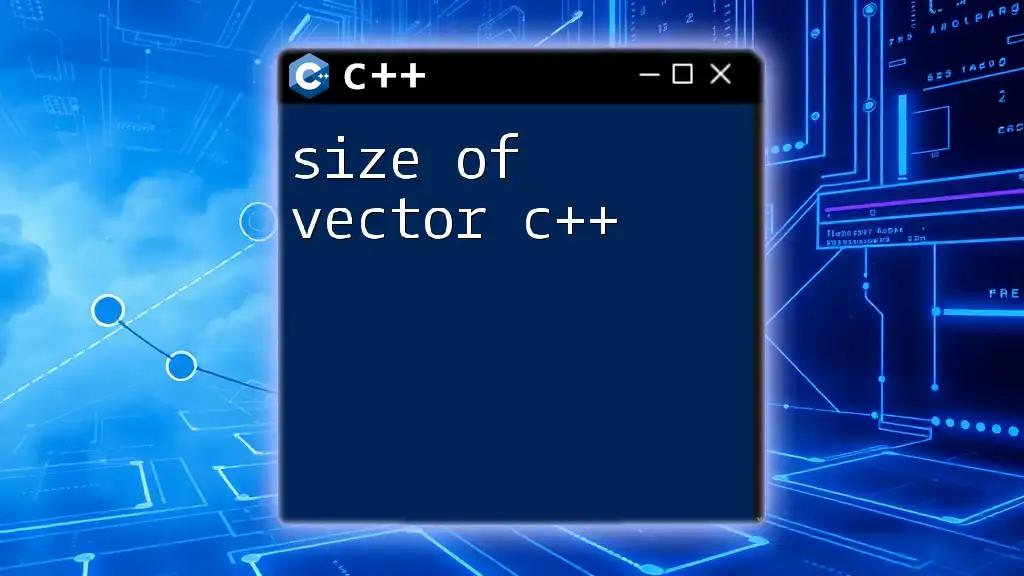
Common Misconceptions about `sizeof`
Array Decay
A common misconception is that using `sizeof` on an array will always yield the expected results. When you pass an array to a function, it decays into a pointer, leading to potentially misleading output:
void function(int arr[]) {
std::cout << "Size of array in function: " << sizeof(arr) << " bytes" << std::endl; // Size of pointer
}
Here, `sizeof(arr)` will only give the size of the pointer rather than the actual size of the array.
Analyzing Struct Padding
Structs may also behave unexpectedly due to padding within the structure to maintain alignment. For example:
struct A {
char c; // 1 byte
int i; // 4 bytes
};
std::cout << "Size of struct A: " << sizeof(A) << std::endl; // Output may show 8 bytes
The 8 bytes result includes padding after `char` to align the `int` correctly, which is essential for optimal CPU access patterns.

Conclusion
Understanding the size of data types and structures in C++ through the `sizeof` operator is crucial for efficient memory management and optimization in programming. Mastering this operator can significantly aid in your ability to write efficient and robust C++ applications. Don’t hesitate to experiment with `sizeof` in various scenarios to deepen your understanding and enhance your programming skills.
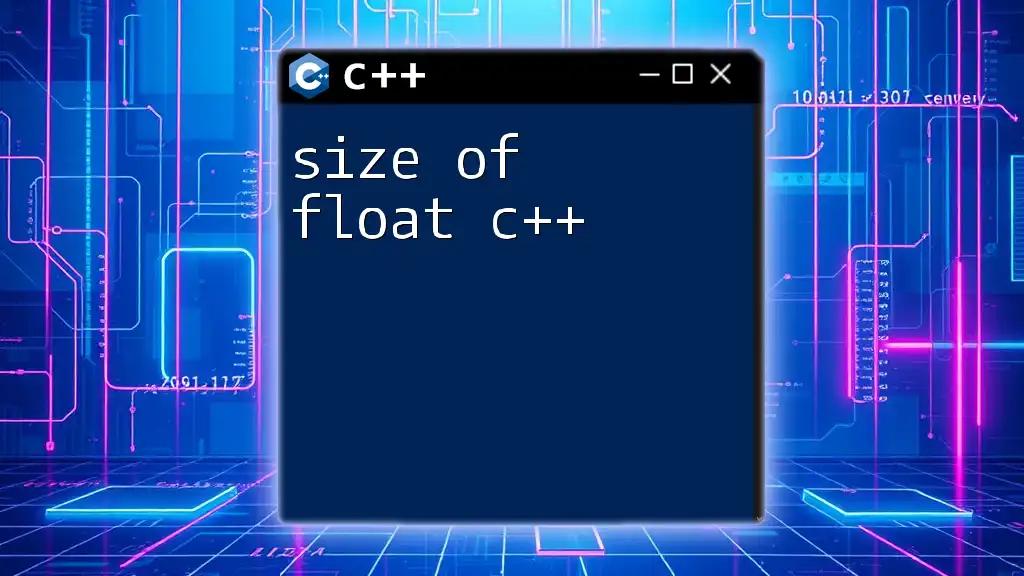
Further Reading and Resources
For those interested in diving deeper into C++ memory management, consider exploring additional articles and books on the subject. They can provide insights and practical exercises that reinforce what you have learned here.
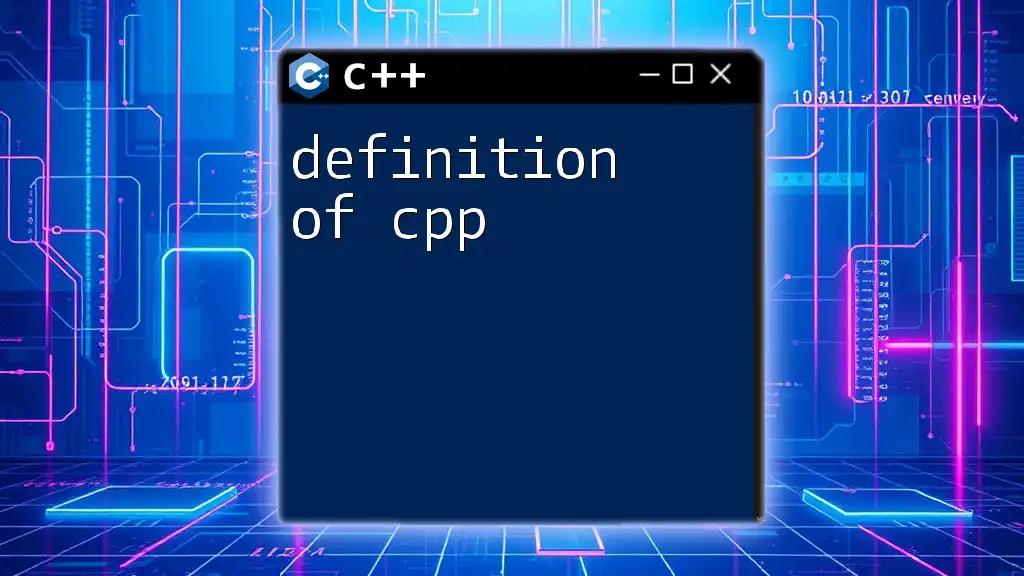
Call to Action
Continue your journey of mastering C++ by subscribing to our resources for even more concise tips and tutorials. Share your experiences or questions about using `sizeof` in your coding projects to engage with the community and enhance your learning process!