C++ features include object-oriented programming, strong type checking, low-level memory manipulation, and the ability to use templates for generic programming.
#include <iostream>
using namespace std;
class Animal {
public:
void speak() {
cout << "Animal speaks!" << endl;
}
};
int main() {
Animal myAnimal;
myAnimal.speak(); // Calls the speak function
return 0;
}
General Features of C++
Overview of C++ Language Characteristics
C++ as a Multi-Paradigm Language
C++ is celebrated for its flexibility, allowing developers to use various programming paradigms. This includes:
- Procedural Programming: This paradigm emphasizes a sequence of actions. It is foundational in programming where functions are defined and called to perform tasks.
- Object-Oriented Programming (OOP): This is a central feature of C++, focusing on encapsulating data and functionality within objects. It includes concepts like classes, inheritance, and polymorphism.
- Generic Programming: C++ supports the use of templates that allow developers to write algorithms and data structures that work with any data type.
Code Example: Paradigms in Action
#include <iostream>
using namespace std;
// Procedural approach
void greet() {
cout << "Hello, World!" << endl;
}
// OOP approach
class Greeter {
public:
void greet() {
cout << "Hello from OOP!" << endl;
}
};
// Generic programming with templates
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
greet(); // Procedural
Greeter g;
g.greet(); // OOP
cout << add<int>(3, 4) << endl; // Generic
return 0;
}
High Performance and Efficiency
Memory Management
C++ offers powerful memory management capabilities, allowing developers to allocate and deallocate memory manually, leading to efficient resource management. However, this requires caution as it can lead to memory leaks if not handled properly.
Code Example: Dynamic Memory Allocation
#include <iostream>
using namespace std;
int main() {
int* arr = new int[5]; // allocating memory for an array of 5 integers
for (int i = 0; i < 5; i++) {
arr[i] = i * 10;
cout << arr[i] << " ";
}
delete[] arr; // deallocate memory
return 0;
}
In the above example, memory for an integer array is allocated dynamically. It’s crucial to `delete` this memory to avoid leaks.

Object-Oriented Programming Features
Encapsulation
Definition and Benefits
Encapsulation is a fundamental principle of OOP allowing the bundling of data (attributes) and methods (functions) that operate on that data within a single unit, or class. It promotes data hiding and the protection of object integrity.
Code Example: Demonstrating Encapsulation
#include <iostream>
using namespace std;
class Account {
private:
double balance;
public:
Account() : balance(0) {}
void deposit(double amount) {
balance += amount;
}
double getBalance() const {
return balance;
}
};
int main() {
Account myAccount;
myAccount.deposit(100);
cout << "Account balance: " << myAccount.getBalance() << endl; // Accessing through public method
return 0;
}
Inheritance
Types of Inheritance
Inheritance allows a class (derived class) to inherit characteristics and behaviors (methods) from another class (base class). C++ supports multiple types, including:
- Single Inheritance: One derived class inherits from one base class.
- Multiple Inheritance: A derived class inherits from multiple base classes.
- Hierarchical Inheritance: Multiple derived classes inherit from one base class.
Code Snippet: Illustrating Inheritance
#include <iostream>
using namespace std;
class Animal {
public:
void sound() {
cout << "Animal sound!" << endl;
}
};
class Dog : public Animal { // Inherits from Animal
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.sound(); // Accessing base class method
myDog.bark();
return 0;
}
Polymorphism
Compile-time and Runtime Polymorphism
Polymorphism allows methods to perform differently based on the object that it is acting upon. In C++, this can be achieved via function overloading (compile-time) and virtual functions (runtime).
Code Example: Demonstrating Polymorphism
#include <iostream>
using namespace std;
class Shape {
public:
virtual void draw() {
cout << "Drawing a shape." << endl;
}
};
class Circle : public Shape {
public:
void draw() override { // Overriding base class method
cout << "Drawing a circle." << endl;
}
};
void render(Shape* shape) {
shape->draw(); // Dynamic dispatch
}
int main() {
Shape s;
Circle c;
render(&s);
render(&c);
return 0;
}
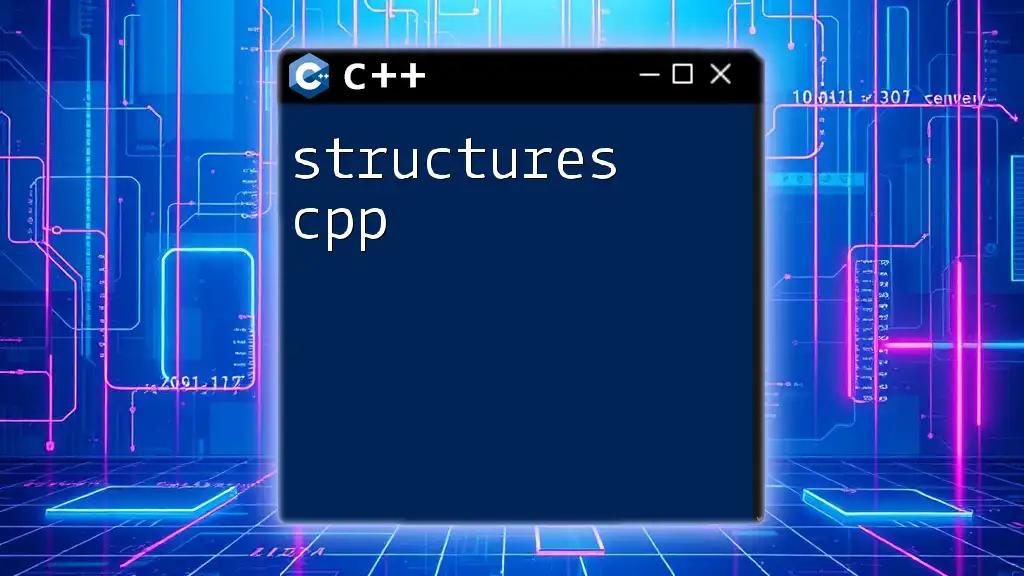
Advanced Features of C++
Standard Template Library (STL)
Overview of STL
The Standard Template Library (STL) in C++ is an essential component that provides a set of C++ template classes to use data structures and algorithms efficiently. Key components include:
- Containers (e.g., vectors, lists, sets, maps): These store data.
- Algorithms: These perform operations on containers.
Code Example: Using STL Containers
#include <iostream>
#include <vector>
#include <map>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
map<string, int> ageMap = {{"Alice", 30}, {"Bob", 25}};
// Accessing vector
for (int num : nums) {
cout << num << " ";
}
cout << endl;
// Accessing map
for (const auto& entry : ageMap) {
cout << entry.first << " is " << entry.second << " years old." << endl;
}
return 0;
}
Function Templates and Class Templates
Generic Programming Explained
Templates in C++ facilitate the creation of generic functions and classes. This means code can operate with any data type, enhancing code reusability.
Code Snippet: Function Template Example
#include <iostream>
using namespace std;
template <typename T>
T multiply(T a, T b) {
return a * b;
}
int main() {
cout << "Multiply int: " << multiply(5, 6) << endl;
cout << "Multiply double: " << multiply(5.5, 6.6) << endl;
return 0;
}

Exception Handling
Importance of Robust Programs
Handling Errors Gracefully
C++ allows for the implementation of strong error handling through exceptions. This encapsulates error handling logic, keeping code organized and robust.
Code Example: Exception Handling
#include <iostream>
#include <stdexcept>
using namespace std;
double divide(int a, int b) {
if (b == 0) {
throw runtime_error("Division by zero error");
}
return static_cast<double>(a) / b;
}
int main() {
try {
cout << divide(10, 2) << endl; // Normal case
cout << divide(10, 0) << endl; // Will throw exception
} catch (const runtime_error& e) {
cerr << "Error: " << e.what() << endl; // Handling exception
}
return 0;
}
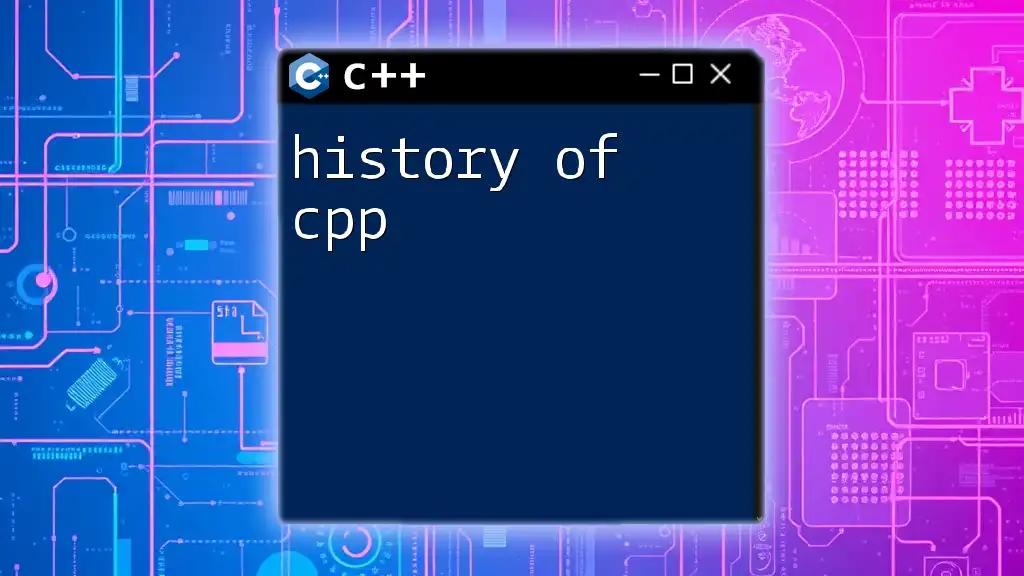
Operator Overloading
Redefining Operators for Custom Types
Concept and Utility
C++ allows developers to redefine the functionality of existing operators to work with user-defined types (classes). This enhances code readability and usability.
Code Example: Operator Overloading
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r, double i) : real(r), imag(i) {}
Complex operator + (const Complex& other) {
return Complex(real + other.real, imag + other.imag);
}
void display() {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex c1(3.0, 4.0);
Complex c2(1.0, 2.0);
Complex c3 = c1 + c2; // Using overloaded '+' operator
c3.display();
return 0;
}

Namespaces and Scope
Managing Code Complexity
Understanding Namespaces
Namespaces in C++ help mitigate naming conflicts by differentiating identifiers. By encapsulating logic, they improve code organization and readability.
Code Snippet: Showing Namespace Usage
#include <iostream>
using namespace std;
namespace Math {
double add(double a, double b) {
return a + b;
}
}
namespace Science {
double add(double a, double b) {
return a + b + 10; // Different behavior
}
}
int main() {
cout << "Math add: " << Math::add(5.0, 4.0) << endl; // Calls Math namespace
cout << "Science add: " << Science::add(5.0, 4.0) << endl; // Calls Science namespace
return 0;
}
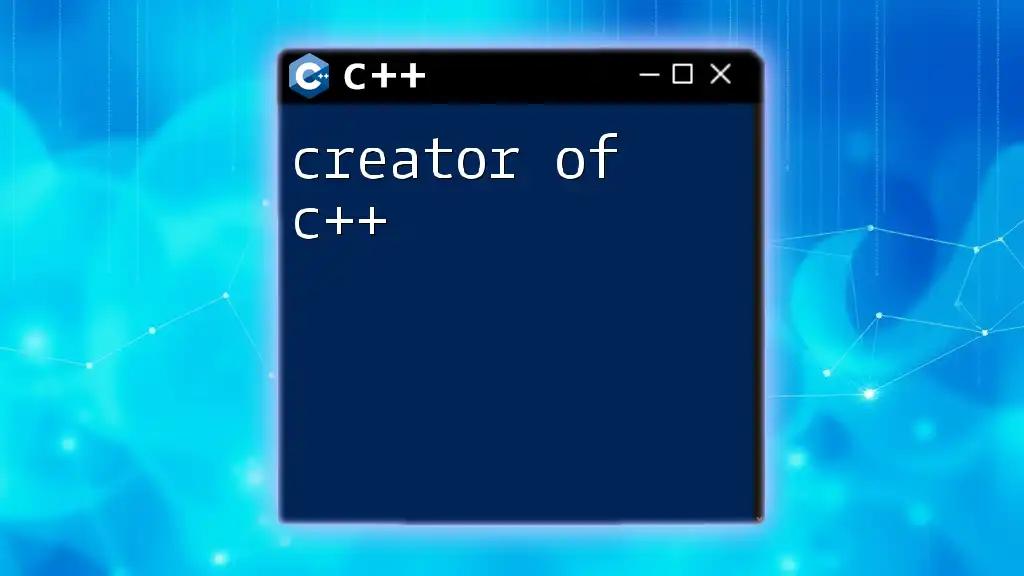
Conclusion
The features of C++ make it a versatile and powerful programming language. This article has explored several aspects, from its multi-paradigm nature to its advanced capabilities such as the STL and exception handling. Understanding these features allows programmers to utilize C++ effectively in various applications. As you practice and implement these concepts, you’ll find C++ a robust tool for tackling complex programming problems. Dive deeper, experiment with examples, and you'll continue to develop your skills in this rich language!
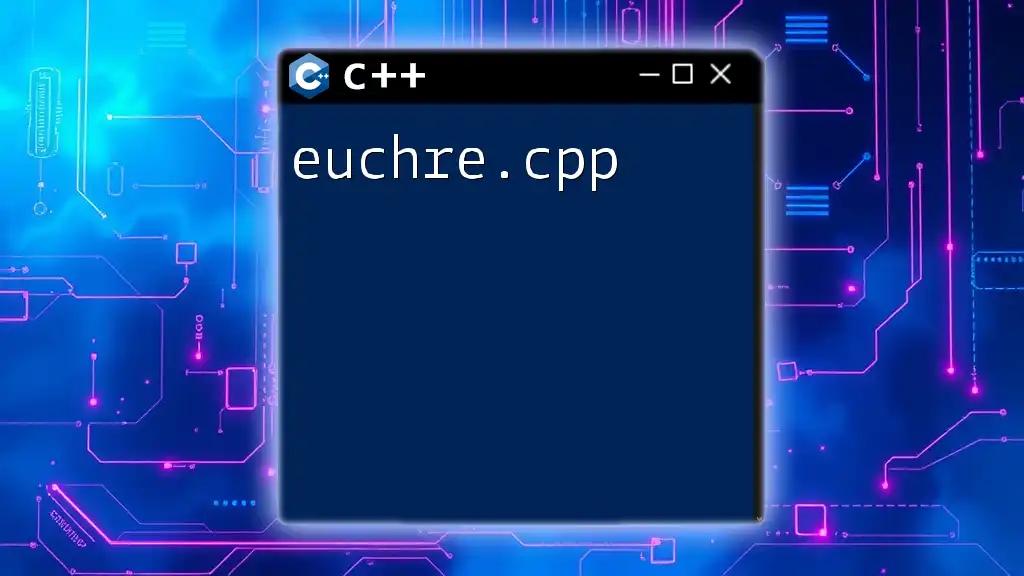
Additional Resources
To further enhance your C++ knowledge, consider exploring online tutorials, forums, and community discussions. Engaging with the C++ community can provide insights and help you solve programming challenges as you grow your expertise.