The `fread` function in C++ is used to read a specified number of bytes from a file into a buffer, allowing efficient data retrieval for binary files.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cstdio>
int main() {
FILE *file = fopen("data.bin", "rb");
if (file) {
char buffer[256];
size_t bytesRead = fread(buffer, sizeof(char), sizeof(buffer), file);
std::cout << "Bytes read: " << bytesRead << std::endl;
fclose(file);
} else {
std::cerr << "Failed to open file." << std::endl;
}
return 0;
}
What is `fread`?
The `fread` function in C++ is a standard library function that facilitates the reading of binary data from a file. It is part of the C standard I/O library and is widely used in C++ for efficient data reading. Understanding how to use `fread` effectively can enhance performance when dealing with large amounts of data.
Using `fread`, developers can directly read binary files into memory buffers, allowing for the swift processing of data. This direct access can be particularly advantageous when compared to other functions such as `fgets` or `fscanf`, which are primarily used for text data.
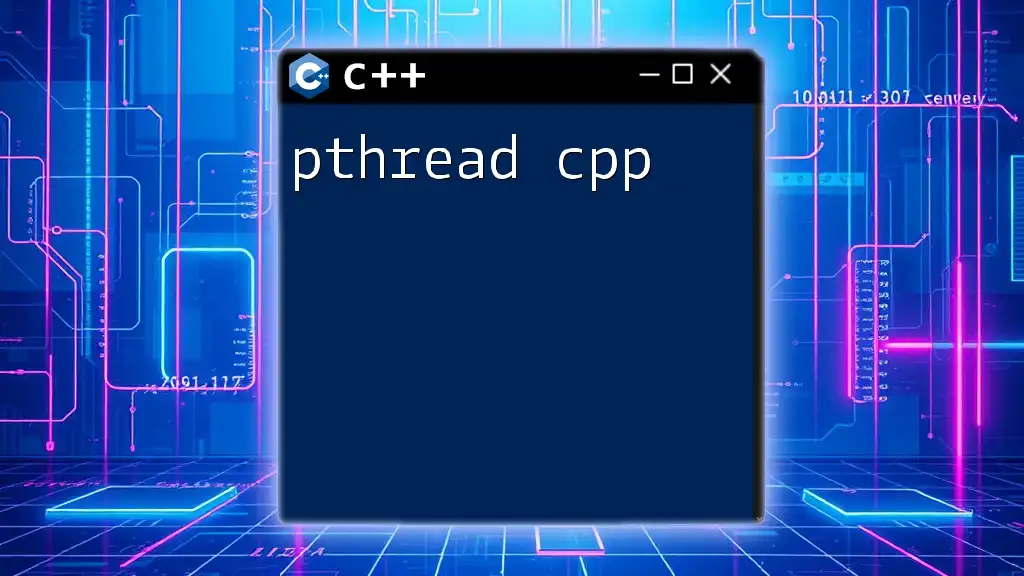
Use Cases for `fread`
`fread` is particularly useful in scenarios such as reading binary file formats, handling multimedia data, and working with custom file structures. It excels in situations where performance is critical, and precise control over input is required. The function allows for bulk reading, which can significantly reduce the overhead typically associated with slower read functions.
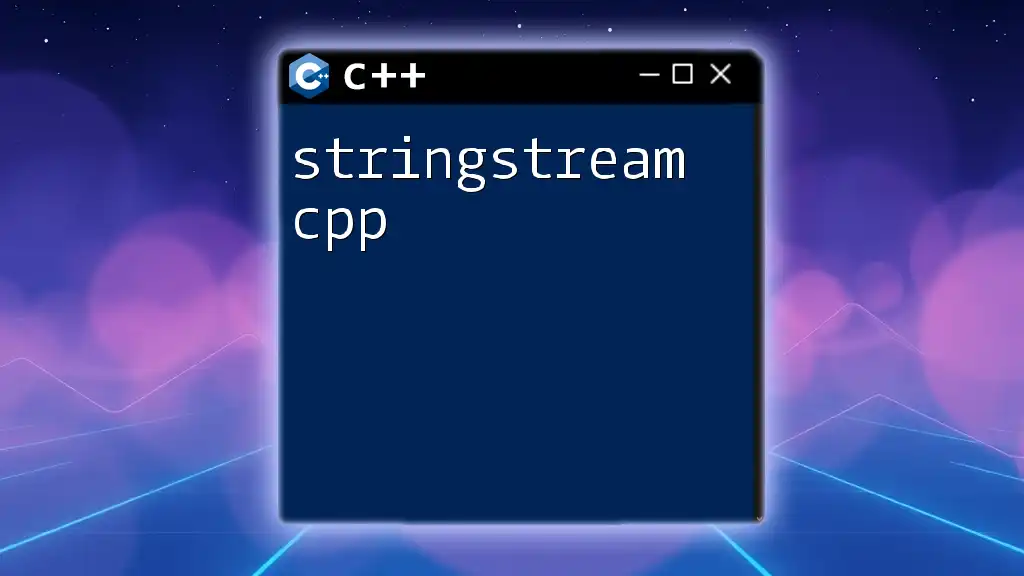
Understanding the `fread` Function
Syntax of `fread`
The syntax of the `fread` function is as follows:
size_t fread(void *ptr, size_t size, size_t count, FILE *stream);
Breaking down the parameters:
- ptr: A pointer to the memory block where the read data will be stored.
- size: The size in bytes of each element to be read.
- count: The number of elements to read.
- stream: Pointer to the file object that specifies the input stream.
Example of Using `fread`
Let's say you want to read binary data into a buffer. Here is a basic example demonstrating `fread` in action:
FILE *file = fopen("data.bin", "rb");
if (file) {
char buffer[256];
size_t bytesRead = fread(buffer, sizeof(char), sizeof(buffer), file);
fclose(file);
}
In this example, we open a binary file named `data.bin`, read up to 256 bytes into `buffer`, and then close the file. The `fread` function reads raw binary data directly into the allocated memory.
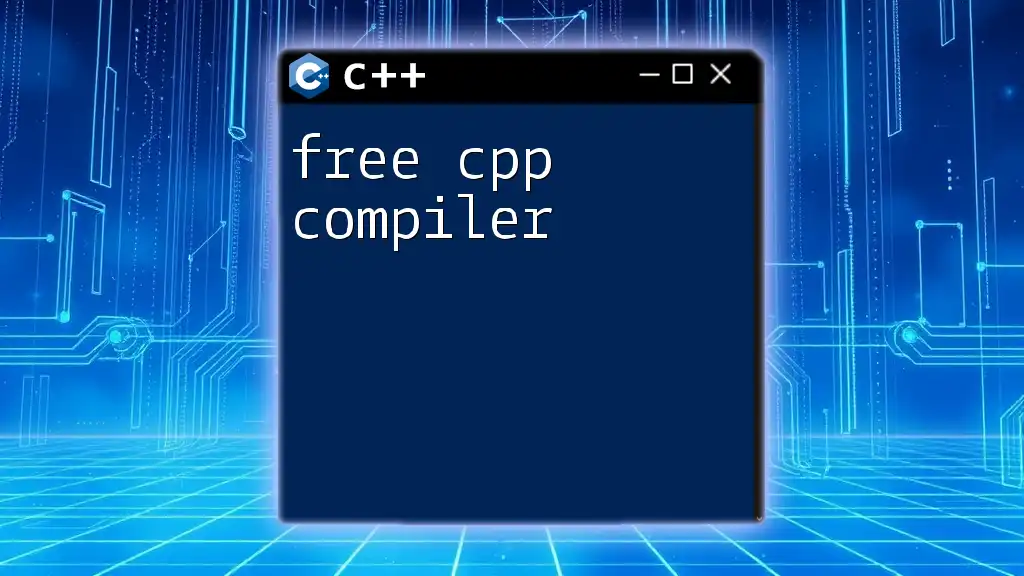
How `fread` Works
Memory Management
Using `fread` effectively requires understanding memory allocation. You must allocate sufficient memory to hold the data being read. If the allocated space is insufficient, this could lead to undefined behavior or memory corruption. Here's an example using dynamic memory allocation:
FILE *file = fopen("data.bin", "rb");
if (file) {
char *buffer = (char *)malloc(1024); // Dynamically allocate memory
if (buffer) {
fread(buffer, sizeof(char), 1024, file);
// Process buffer
free(buffer); // Always free allocated memory
}
fclose(file);
}
In this example, we allocate memory for 1024 bytes and read data into the buffer. Remember to always free your allocated memory after use.
Return Value of `fread`
The value returned by `fread` indicates how many items were successfully read. If it returns a value less than count, it might indicate an error or that the end of the file was reached. You can check for conditions like this:
size_t result = fread(buffer, sizeof(char), 1024, file);
if (result < 1024) {
if (feof(file)) {
// Handle end-of-file condition
} else if (ferror(file)) {
perror("Error reading the file");
}
}
It’s crucial to handle these return values properly to ensure smooth execution.
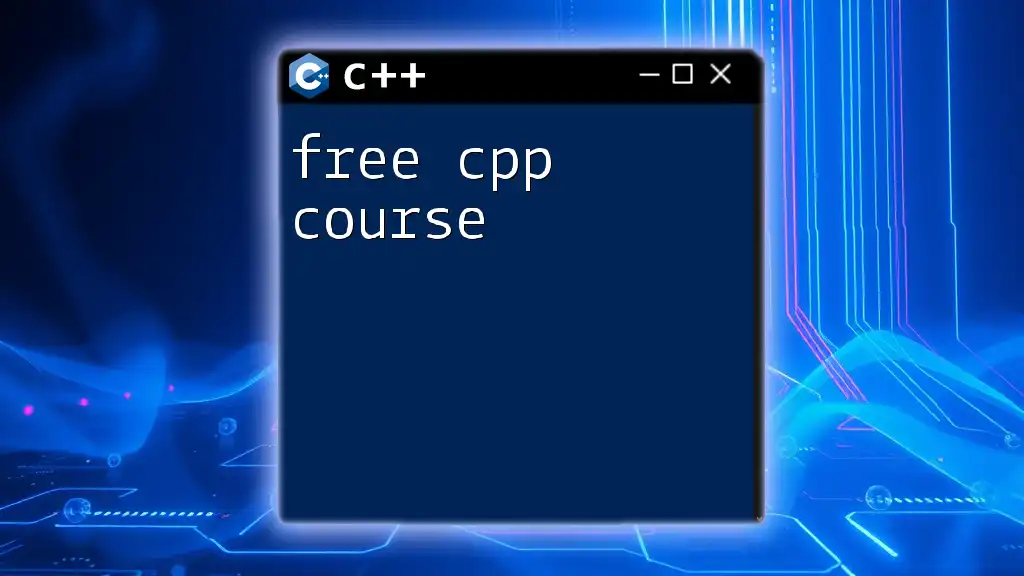
Example Usage of `fread`
Reading Structure Data
`fread` excels in scenarios where structured data is involved. Consider a data file containing a binary representation of a custom structure, such as:
struct Person {
char name[50];
int age;
};
FILE *file = fopen("people.dat", "rb");
if (file) {
Person person;
fread(&person, sizeof(Person), 1, file);
printf("Name: %s, Age: %d\n", person.name, person.age);
fclose(file);
}
In this example, we define a structure `Person` with a name and age. The `fread` function reads the data directly into an instance of `Person`, demonstrating the simplicity with which structured data can be processed.
Handling Errors with `fread`
Effectively managing errors is crucial when dealing with file operations. After reading data with `fread`, always check for potential read errors. Utilize the `feof` and `ferror` functions to ascertain the conditions that occurred:
size_t result = fread(buffer, sizeof(char), 1024, file);
if (result != 1024) {
if (feof(file)) {
printf("End of file reached.\n");
} else if (ferror(file)) {
perror("Error reading the file");
}
}
This approach ensures robust error handling and facilitates debugging.
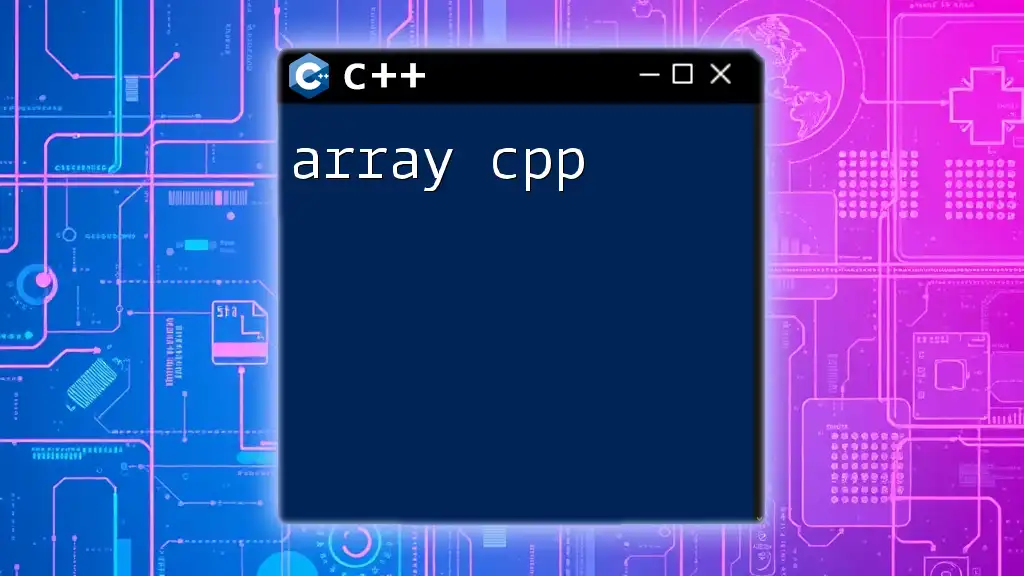
Best Practices for Using `fread`
Choosing the Right Data Types
Choosing the right data types when utilizing `fread` is key to ensuring effective and efficient data reading. Consider what data types match the structure of your binary files. Mismatched data types can lead to misreads and corrupted values.
Buffer Size Considerations
Selecting an appropriate buffer size is vital for performance. A larger buffer size allows for more data to be read in a single call to `fread`, reducing the number of I/O operations and enhancing performance. Here’s an example of increasing buffer size:
const size_t bufferSize = 4096; // Larger buffer for efficiency
char *buffer = (char *)malloc(bufferSize);
if (buffer) {
// Perform fread with a larger buffer
fread(buffer, sizeof(char), bufferSize, file);
free(buffer); // Don't forget to free
}
Closing Files and Memory Management
Upon completion of file operations, always ensure that files are closed using `fclose`, and any dynamically allocated memory is freed with `free()`. Neglecting these practices can lead to memory leaks and resource exhaustion.
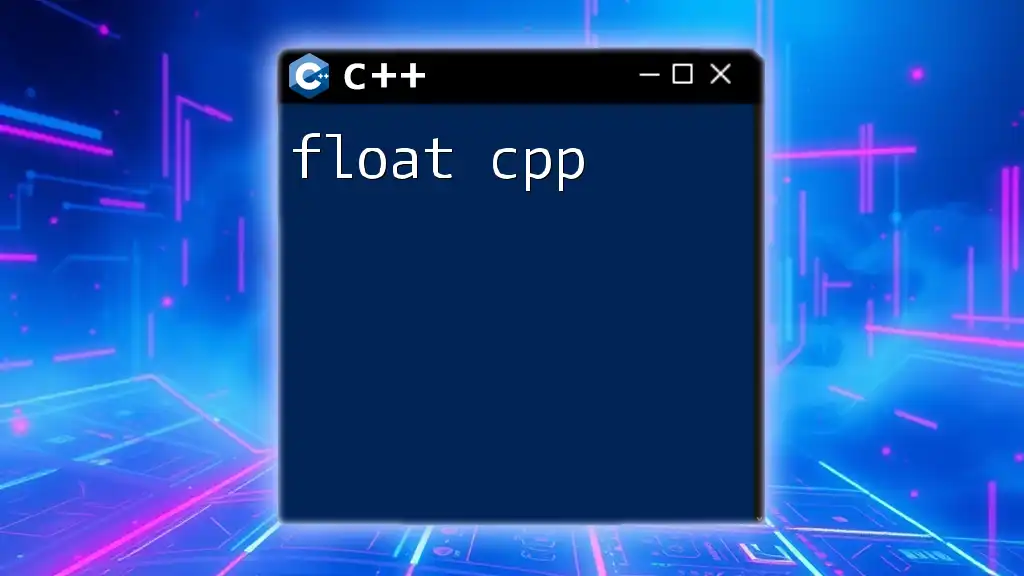
Common Mistakes to Avoid
Not Checking File Pointers
One common mistake is failing to check whether the file pointer was opened successfully. Always validate the file pointer before proceeding with any read operations:
FILE *file = fopen("data.bin", "rb");
if (!file) {
perror("Failed to open file");
}
Ignoring Return Values
Another frequent error is neglecting to check the return values of `fread`. Not verifying how many elements were read can lead to unexpected behavior, especially in critical operations.
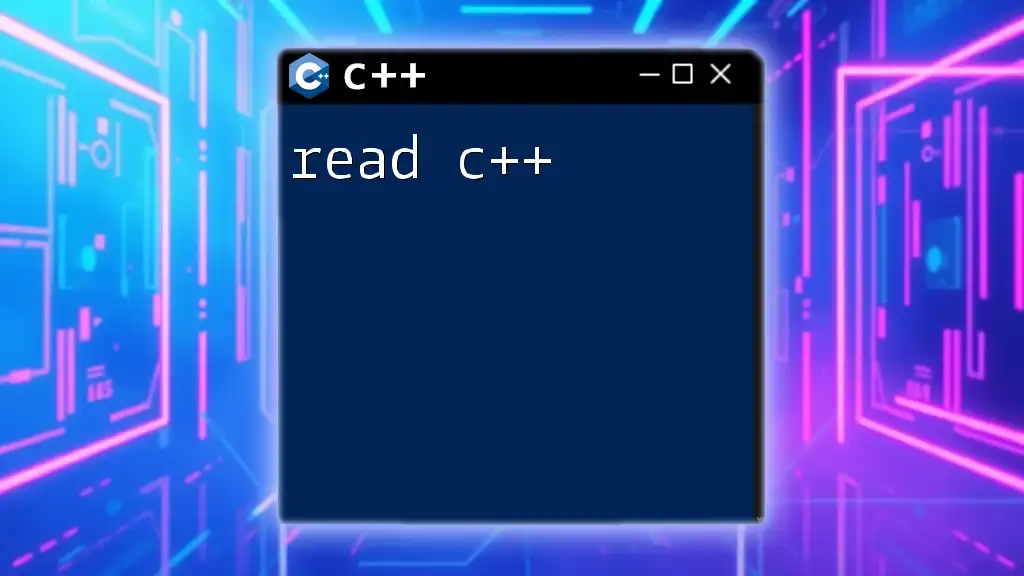
Conclusion
In summary, the fread cpp function is a powerful tool for reading binary data efficiently. By understanding its syntax, working principles, and best practices, developers can leverage `fread` for high-performance data processing. Always remember to handle memory responsibly and incorporate error checking to produce robust applications.
For those looking to deepen their knowledge, consider exploring additional resources on file handling, memory management, and advanced data processing techniques in C++. Following these guidelines will facilitate a smoother experience when utilizing `fread` in your C++ projects.