"Advanced C++ involves mastering complex features such as templates and smart pointers to enhance code efficiency and safety."
Here's a code snippet demonstrating a simple template function for swapping two values:
#include <iostream>
template <typename T>
void swap(T &a, T &b) {
T temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
swap(x, y);
std::cout << "x: " << x << ", y: " << y << std::endl; // Output: x: 10, y: 5
return 0;
}
Understanding Advanced C++ Concepts
The Nature of C++: A Multifaceted Language
C++ is renowned for its hybrid approach, effectively merging procedural programming with object-oriented programming. This versatility allows it to be applied in various domains such as systems programming, game development, and high-performance applications. Understanding its multifaceted nature is essential for modern software development.
Memory Management in C++
Memory management is a fundamental aspect of advanced cpp. Properly handling memory not only ensures efficiency but also prevents resource leaks and crashes.
Dynamic Memory Allocation enables programmers to allocate memory at runtime using the `new` keyword. It’s crucial to release this memory using `delete` to avoid memory leaks. Here’s an example of allocating and deallocating memory dynamically:
int* arr = new int[10]; // allocate array of 10 integers
// Use the array...
delete[] arr; // deallocate memory
Contrarily, managing memory through the stack is straightforward but limited in size. For larger data structures, the heap proves necessary.
Smart Pointers provide a more robust solution. They automate memory management and help avoid memory leaks.
-
`unique_ptr`: Owns a dynamically allocated object and ensures it is deleted when it goes out of scope.
std::unique_ptr<int> p(new int(10));
-
`shared_ptr`: Allows multiple pointers to own the same dynamically allocated object, maintaining a reference count.
std::shared_ptr<int> sp1(new int(20)); auto sp2 = sp1; // sp2 shares ownership with sp1
-
`weak_ptr`: Works in conjunction with `shared_ptr` to prevent circular references by allowing temporary, non-owning access.
Advanced Object-Oriented Programming
C++ shines in its object-oriented features, such as inheritance and polymorphism.
Inheritance and Polymorphism
- Complex Inheritance Structures enable you to create rich class hierarchies. Single, multiple, and multi-level inheritance each have their use cases. Consider:
class Base {}; // Base class
class Derived : public Base {}; // Single inheritance
class MultiDerived : public Base {}; // Multiple inheritance
- Polymorphism permits functions to operate in different ways based on the object type. This includes function overloading, operator overloading, and virtual functions. For example, using virtual functions makes it possible to call derived class methods through base class pointers:
class Base {
public:
virtual void show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class" << std::endl;
}
};
Templates and Generic Programming
Templates in C++ allow you to create functions and classes that can operate with any data type, promoting code reuse.
What are Templates?
Templates can be classified into function templates and class templates. Here’s how you would define and use a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
Template Specialization
You can specialize templates to handle specific data types differently. For example, if you want to handle the `char*` type distinctively:
template <>
const char* add<const char*>(const char* a, const char* b) {
return strcat(a, b); // assuming a is large enough to hold the result
}
Mastering the Standard Template Library (STL)
The Standard Template Library (STL) enhances productivity by providing ready-to-use classes and functions.
Introduction to STL
STL comprises three main components: containers, algorithms, and iterators. Using STL can drastically reduce coding time and improve quality.
Containers and Their Applications
-
Sequential Containers:
- `vector`: Dynamic arrays with fast random access.
- `list`: Doubly-linked lists suitable for frequent insertions/deletions.
- `deque`: Double-ended queues that allow insertion/removal at both ends.
-
Associative Containers:
- `set`: Stores unique elements.
- `map`: Key-value pairs with unique keys; allows quick lookups.
Example of using a `map`:
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
Algorithms and Functional Programming Paradigms
STL offers several algorithms (e.g., `sort`, `search`, `transform`). These can be effortlessly applied to containers to process data efficiently.
For example, sorting a vector:
std::vector<int> numbers = {5, 2, 8, 1, 3};
std::sort(numbers.begin(), numbers.end());
Concurrency in C++
With the increasing demand for high-performance applications, concurrency has become vital.
Understanding Threads and Thread Management
In C++, you can create and manage threads using the `<thread>` library. Here is how you can create a simple thread:
#include <thread>
void function() {
// Code to execute in the thread
}
std::thread t(function);
t.join(); // Wait for thread to finish
Mutexes and Locks
Mutexes prevent data races. Using `std::mutex`, you can synchronize threads to protect shared resources:
std::mutex mtx;
mtx.lock();
// Access shared resource
mtx.unlock();
Asynchronous Programming
Future and Promise mechanisms facilitate asynchronous programming. They allow tasks to run in the background, providing results upon completion:
std::promise<int> prom;
std::future<int> fut = prom.get_future();
std::thread t([&prom]() {
prom.set_value(10); // Set the value in the promise
});
t.join();
std::cout << "The answer is: " << fut.get() << std::endl; // Get the value
Advanced Features of C++
Move Semantics and Rvalue References
Move semantics enhance performance by transferring resources instead of copying them, especially in temporary objects.
Rvalue references let functions accept and modify temporary values without copying:
class Buffer {
public:
Buffer(size_t size) : data(new int[size]), size(size) {}
Buffer(Buffer&& other) noexcept : data(other.data), size(other.size) {
other.data = nullptr; // Transfer ownership
}
~Buffer() { delete[] data; }
private:
int* data;
size_t size;
};
Lambda Expressions
Lambda expressions allow you to write inline anonymous functions. For instance:
auto add = [](int a, int b) { return a + b; };
std::cout << add(5, 3); // Outputs 8
Error Handling in Advanced C++
Exception Handling
Using exception handling effectively can save your program from crashing. The basic structure involves:
try {
// Code that may throw exceptions
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
Custom Exception Classes can be defined to provide more informative error messages:
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred!";
}
};
Modern C++ Best Practices
Adhering to coding standards and conventions is crucial in advanced C++.
- Write code that is clean and maintainable.
- Avoid using raw pointers; prefer smart pointers instead.
- Regularly profile and optimize your code for performance.
Additionally, be aware of common pitfalls such as memory leaks, race conditions, and unhandled exceptions.
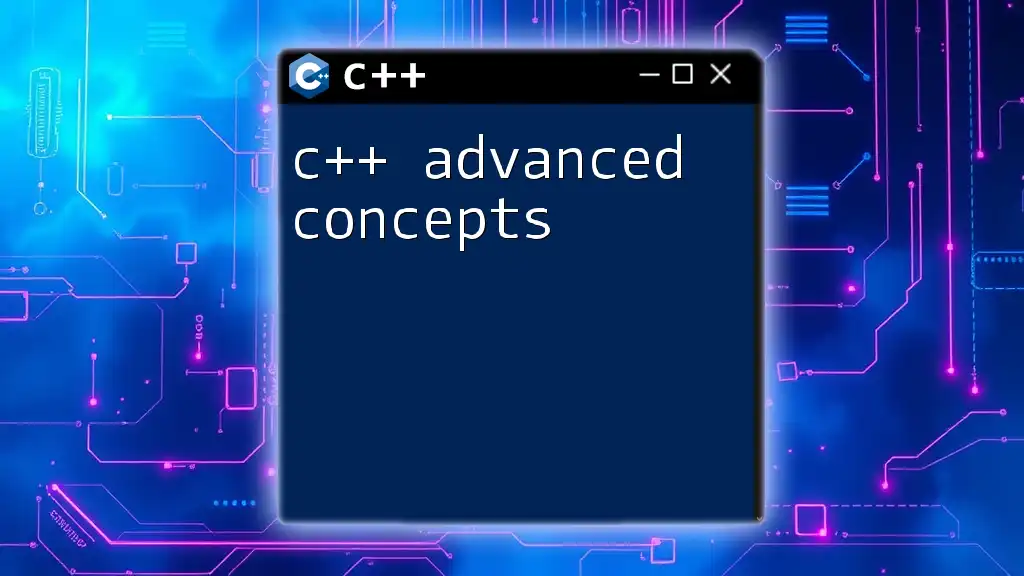
Conclusion
In this guide, we've explored various advanced cpp concepts, from memory management and object-oriented programming to the nuances of STL and concurrency. Mastering these areas is essential for developing high-performance applications in C++. Continue practicing these concepts, engage with coding communities, and never hesitate to seek help and share knowledge on your journey to becoming an advanced cpp developer.
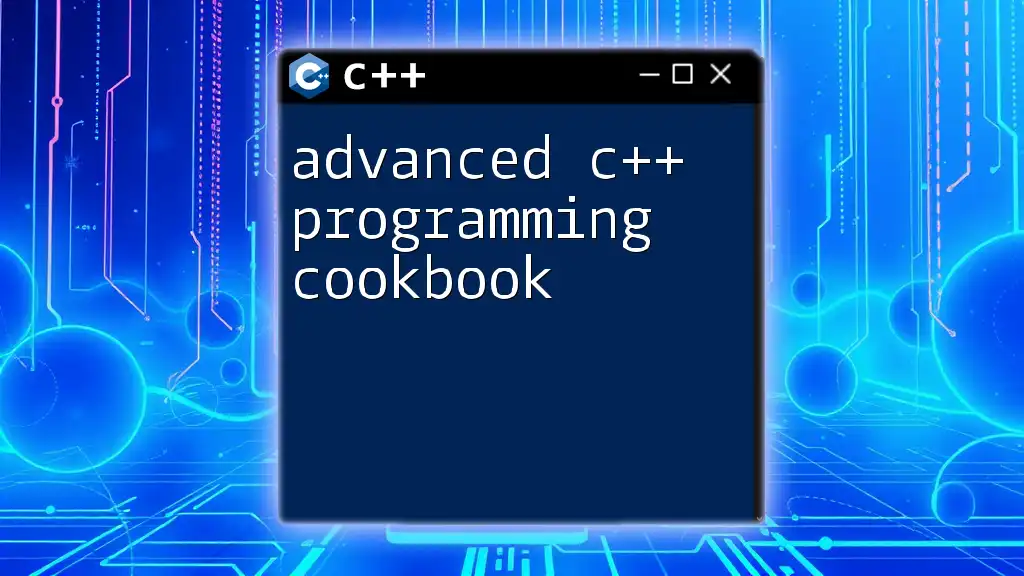
Additional Resources
For further learning on advanced C++, consider exploring recommended books, online courses, and programming communities. These resources can deepen your understanding and keep you updated with the latest practices and technologies in the world of C++.