The greatest common divisor (GCD) can be calculated in C++ using the `std::gcd` function from the `<numeric>` header to efficiently find the largest integer that divides two numbers without leaving a remainder.
#include <iostream>
#include <numeric>
int main() {
int a = 36, b = 60;
std::cout << "GCD of " << a << " and " << b << " is " << std::gcd(a, b) << std::endl;
return 0;
}
Understanding the Concept of GCD
What is GCD?
The Greatest Common Divisor (GCD) of two integers is defined as the largest integer that divides both numbers without leaving any remainder. For example, the GCD of 48 and 18 is 6, as both numbers can be divided by 6 cleanly.
Visualizing GCD helps in better understanding its computation. For example, if we take the numbers 48 and 18, their divisors are:
- Divisors of 48: 1, 2, 3, 4, 6, 8, 12, 16, 24, 48
- Divisors of 18: 1, 2, 3, 6, 9, 18
The biggest number that appears in both lists is 6, making it the GCD.
Properties of GCD
Understanding the properties of GCD can simplify calculations and problem-solving:
- Commutative Property: GCD does not depend on the order of numbers, meaning `gcd(a, b) = gcd(b, a)`.
- Associative Property: For three integers, `gcd(a, gcd(b, c)) = gcd(gcd(a, b), c)`.
- Behavior with Zero and Negatives:
- The GCD of zero and any integer `b` is `|b|` (the absolute value of b).
- The GCD of zero with zero is undefined, while the GCD of two negative integers is the same as the GCD of their absolute values.
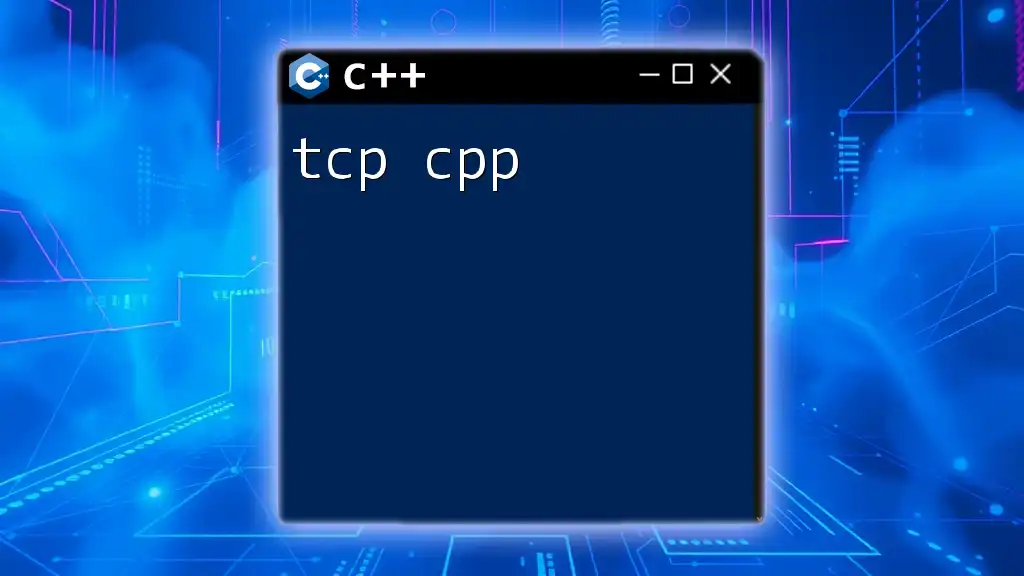
Methods to Calculate GCD in C++
Introduction to GCD Algorithms
There are several algorithms to compute GCD, each with its efficiency and use cases. Below are the most commonly utilized methods in C++.
Euclidean Algorithm
The Euclidean algorithm is one of the oldest and most effective methods for determining GCD. The principle behind this algorithm is that the GCD of two numbers also divides their difference.
To visualize the process, consider the example of finding the GCD of 48 and 18:
- Divide 48 by 18 to get a quotient of 2 and a remainder of 12 (48 = 18*2 + 12).
- Now, find the GCD of 18 and 12.
- Divide 18 by 12 to get a quotient of 1 and a remainder of 6 (18 = 12*1 + 6).
- Next, find the GCD of 12 and 6.
- Since 12 mod 6 is 0, the GCD is 6.
Here’s how you can implement the Euclidean algorithm in C++:
int gcd(int a, int b) {
return (b == 0) ? a : gcd(b, a % b);
}
Iterative Approach
The iterative method is another way to calculate GCD, avoiding recursion. This method utilizes a loop to achieve the same result as the recursive Euclidean algorithm.
Here's an example demonstrating the iterative method:
int gcdIterative(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
In this implementation, we continue to compute `b` until it becomes zero, at which point `a` holds the GCD.
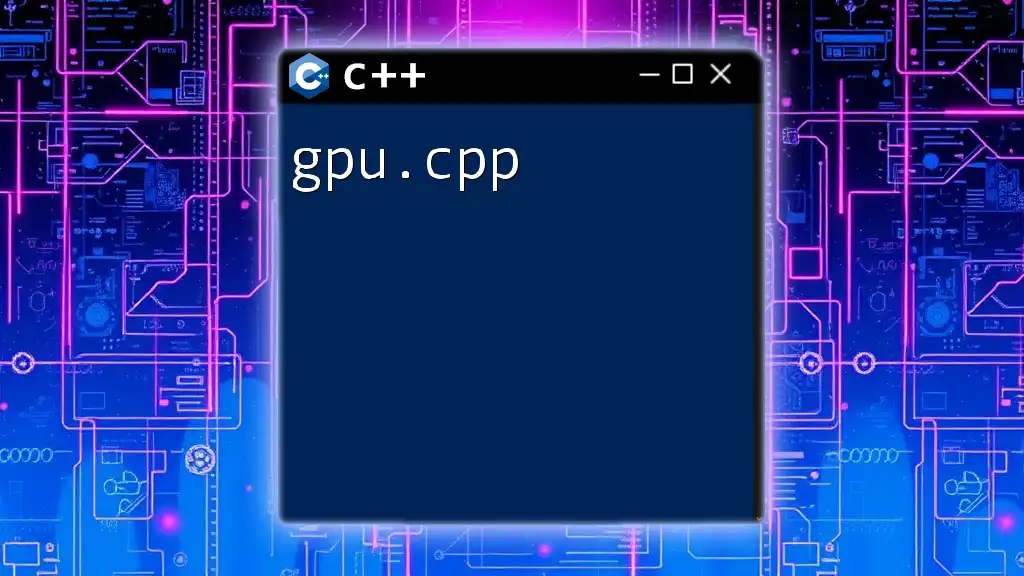
Using C++ STL for GCD
With the introduction of C++17, the Standard Template Library (STL) includes a built-in `std::gcd` function, providing a straightforward way to calculate GCD without implementing algorithms manually. This facilitates ease of use and reliability.
Here's how to use `std::gcd`:
#include <numeric> // for std::gcd
#include <iostream>
int main() {
int a = 48, b = 18;
std::cout << "GCD: " << std::gcd(a, b) << std::endl;
return 0;
}
Utilizing STL has the advantage of relying on well-tested implementations optimized for performance.
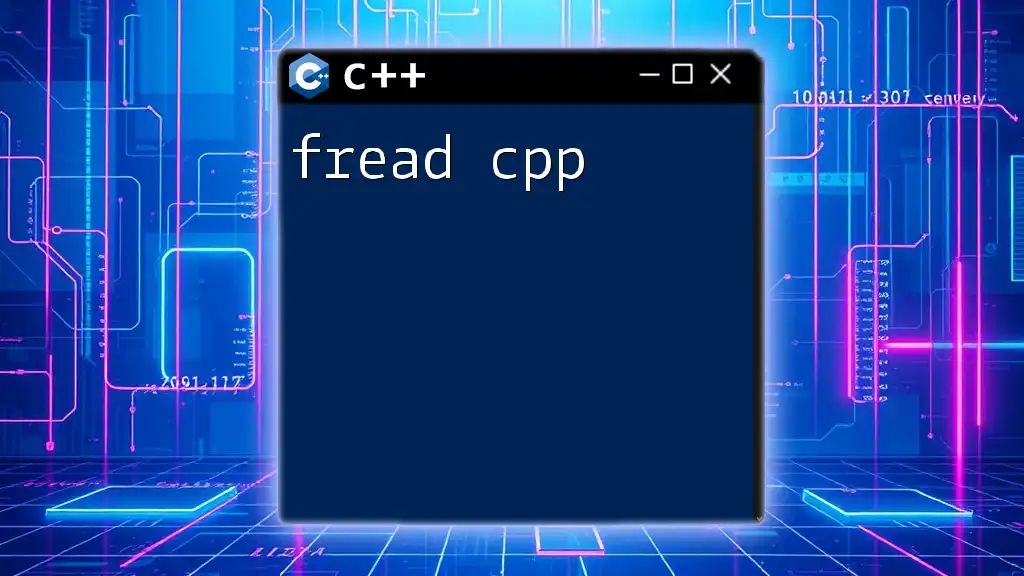
Practical Applications of GCD in C++
Reducing Fractions
One of the most common applications of GCD is reducing fractions to their simplest form. By finding the GCD of the numerator and denominator, you can divide both by the GCD to achieve the simplest representation.
For example, to reduce the fraction 48/18:
- Compute GCD(48, 18) = 6.
- Divide both the numerator and denominator by 6, yielding 8/3.
Cryptography
In cryptography, GCD plays a crucial role in algorithms such as RSA. The public and private keys are generated based on the properties of coprime numbers, and GCD helps ensure these numbers share no factors.
Problem Solving
GCD can also be instrumental in various algorithm challenges. For instance, determining common factors among multiple integers or solving problems in combinatorial mathematics often utilizes GCD.
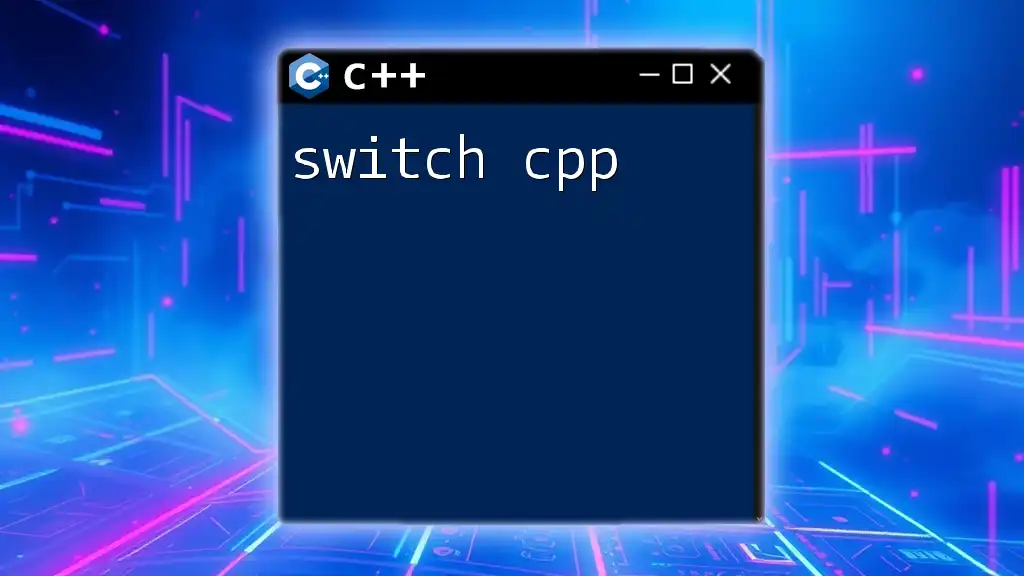
Optimizing GCD Calculations
In performance-sensitive applications, optimizing GCD calculations is necessary, especially with large numbers. The Euclidean algorithm has a logarithmic time complexity, making it efficient for most cases. Using the iterative approach can further enhance performance by avoiding the overhead of recursive function calls.
Best practices include:
- Pre-computing GCD for small ranges of numbers when possible.
- Leveraging efficient algorithms and built-in functions provided by C++ STL.
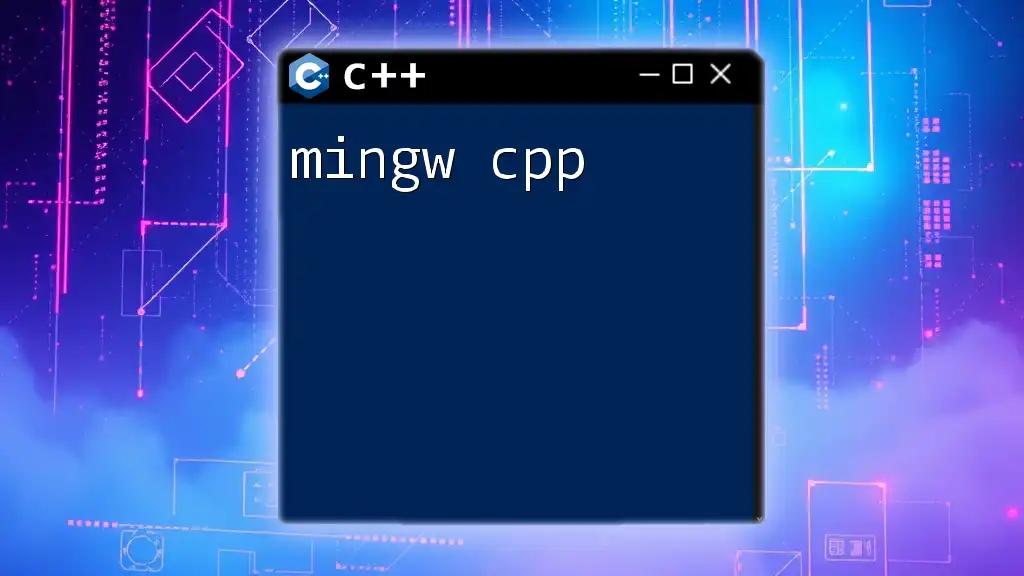
Common Mistakes and FAQs
Common Mistakes
One common mistake is neglecting to consider the case when one of the numbers is zero. Ensuring that GCD is well-defined leads to clearer outcomes.
FAQs
-
What happens if one or both numbers are negative? The GCD is defined in terms of absolute values, thus `gcd(-a, b)` is the same as `gcd(a, b)`.
-
What is the GCD of (0, b) or (a, 0)? The answer will be the absolute value of the non-zero number.
-
How does the algorithm handle large numbers? While the algorithms are efficient, using the built-in `std::gcd` is preferred for handling large integers, as they are optimized internally.
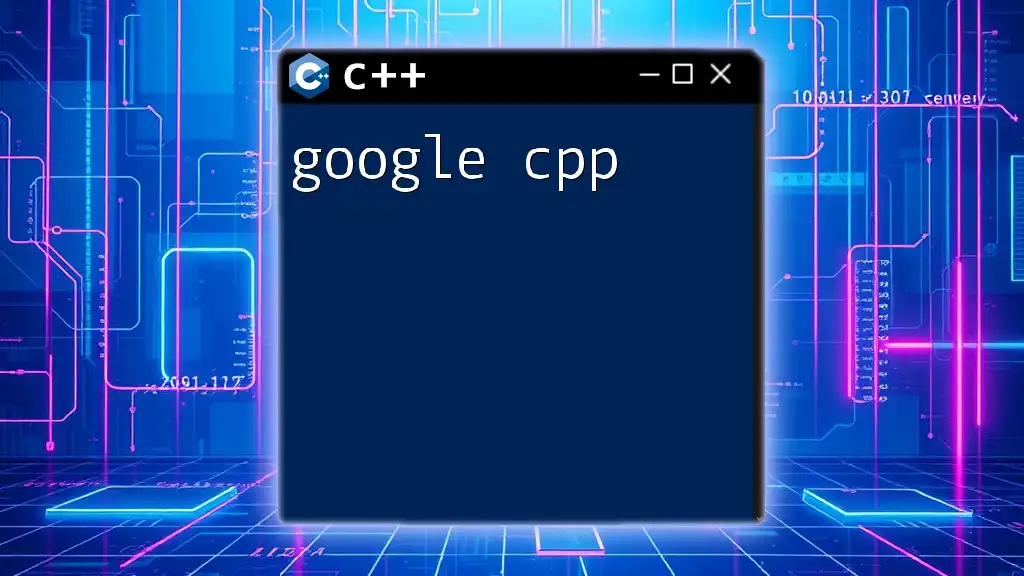
Conclusion
Mastering the concept of GCD in C++ is essential for effective problem-solving in mathematical computations and software development. From foundational algorithms to C++ STL implementations, understanding GCD opens doors to numerous applications across various fields, including mathematics, cryptography, and programming. Practicing with different examples will cement your understanding and enhance your coding skills.