In C++, the `protected` access specifier allows members of a class to be accessible within the class itself and by derived classes, but not through instances of the class.
Here’s a simple code snippet demonstrating the use of `protected`:
#include <iostream>
class Base {
protected:
int protectedVar;
public:
Base() : protectedVar(42) {}
};
class Derived : public Base {
public:
void show() {
std::cout << "Protected variable: " << protectedVar << std::endl; // Accessible here
}
};
int main() {
Derived obj;
obj.show();
return 0;
}
What is a Protected Member in C++?
The protected access specifier is a fundamental concept in C++ that allows a class to define members that are accessible to derived classes but not to the general public. This means that a protected member can be accessed directly within its own class and by any subclasses that inherit from it, making it an essential tool for controlling access while allowing flexibility in derived classes.
Differences Between Access Specifiers
- Public Members: Accessible from anywhere in the code.
- Private Members: Accessible only within the defining class, completely hidden from subclasses and outside access.
- Protected Members: Accessible within the defining class and by subclasses, but not available outside these classes, enhancing encapsulation while providing flexibility for inheritance.
The main goal of using protected in C++ is to facilitate inheritance and provide controlled access to class members, which is crucial in object-oriented programming.
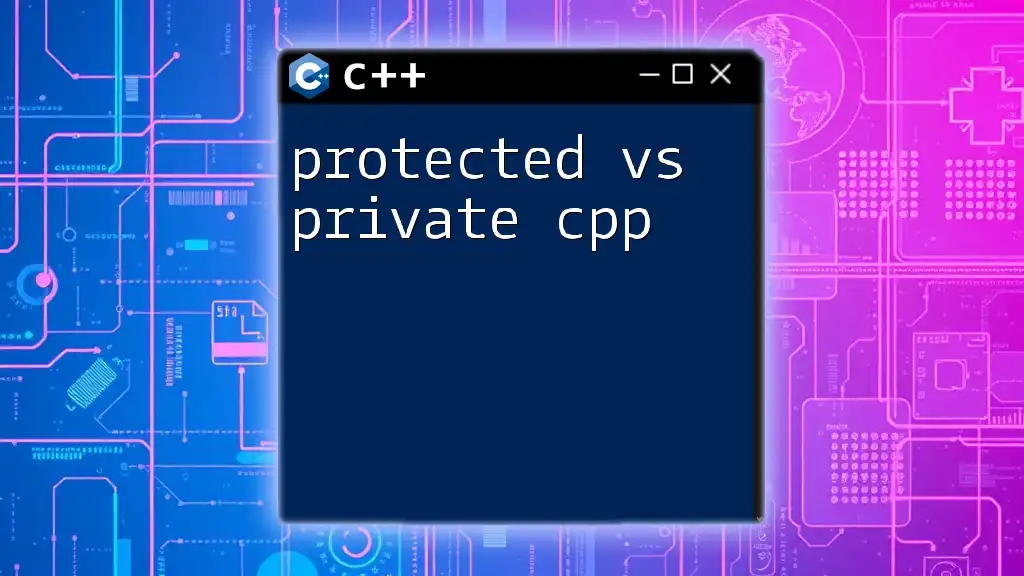
Advantages of Using Protected Members in C++
Using protected members comes with several advantages:
-
Facilitating Inheritance: When you want derived classes to inherit properties or functionality from a base class while keeping certain members hidden from the outside world, protected members serve this purpose well.
-
Maintaining Data Encapsulation: Protected members allow subclasses to access important data, ensuring that core functionalities remain intact while still adhering to the principles of encapsulation.
-
Use Cases: In many frameworks and libraries, you might find that you need a base class with certain configuration values that subclasses must specify or modify. Using protected members allows this while preventing external classes from manipulating them directly.
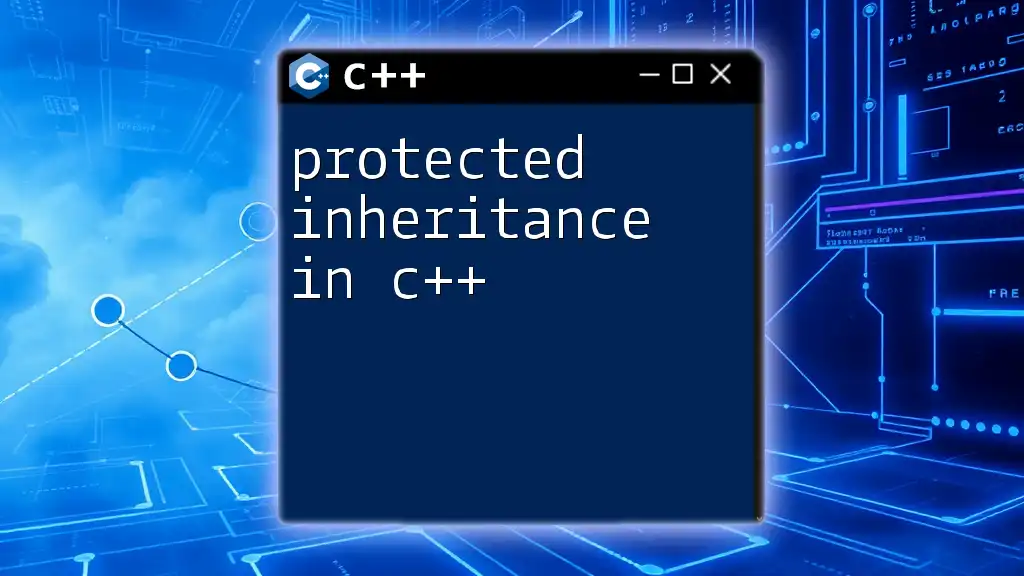
Creating a Protected Class in C++
To create a protected class in C++, you'll define a class and specify which member variables or functions are protected.
Syntax of a Protected Class
Here's a simple example to illustrate how you might define a class with a protected member:
class Base {
protected:
int protectedValue;
public:
Base() : protectedValue(10) {}
};
In this example, `protectedValue` is accessible in any class that inherits from `Base`, but it cannot be accessed from outside the class hierarchy.
Accessing Protected Members
Protected members can be accessed within derived classes. The following code demonstrates this concept clearly:
class Derived : public Base {
public:
void showValue() {
std::cout << "Protected Value: " << protectedValue << std::endl;
}
};
In the `Derived` class above, we can see that by inheriting from `Base`, it can access `protectedValue` directly.
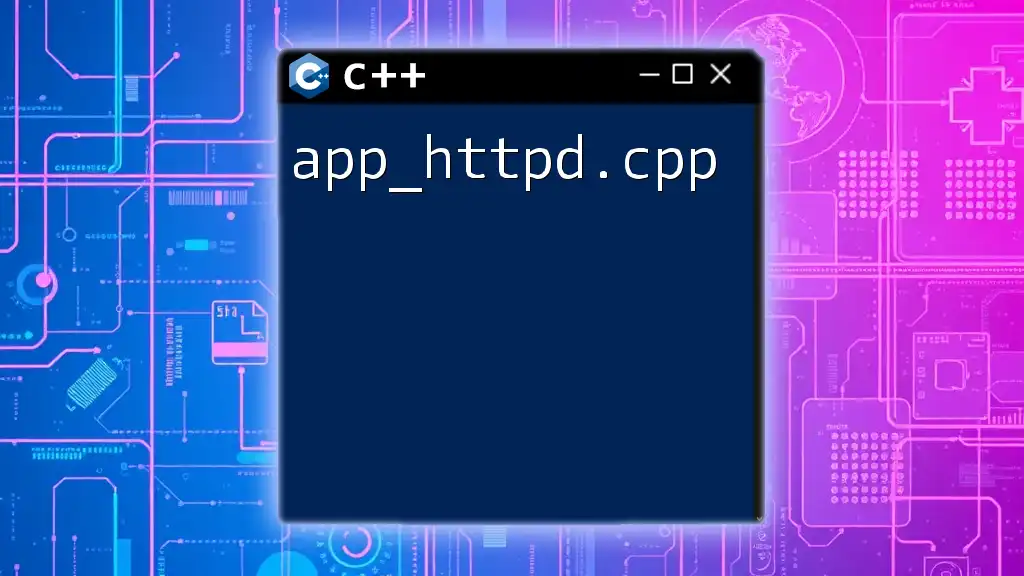
Implementing Protected Members in C++ Classes
Implementing protected members is straightforward. The key is to define your class architecture thoughtfully, so you understand when and how to use protected access.
Code Example: Implementing a Protected Member
Below is an example of implementing a protected member in a class that represents an animal:
class Animal {
protected:
std::string name;
public:
Animal(std::string n) : name(n) {}
void display() {
std::cout << "Animal: " << name << std::endl;
}
};
class Dog : public Animal {
public:
Dog(std::string n) : Animal(n) {}
void bark() {
std::cout << name << " says Woof!" << std::endl;
}
};
In this example, `name` is a protected member. The `Dog` class can access `name` and utilize it methodically, while external code cannot directly manipulate it.
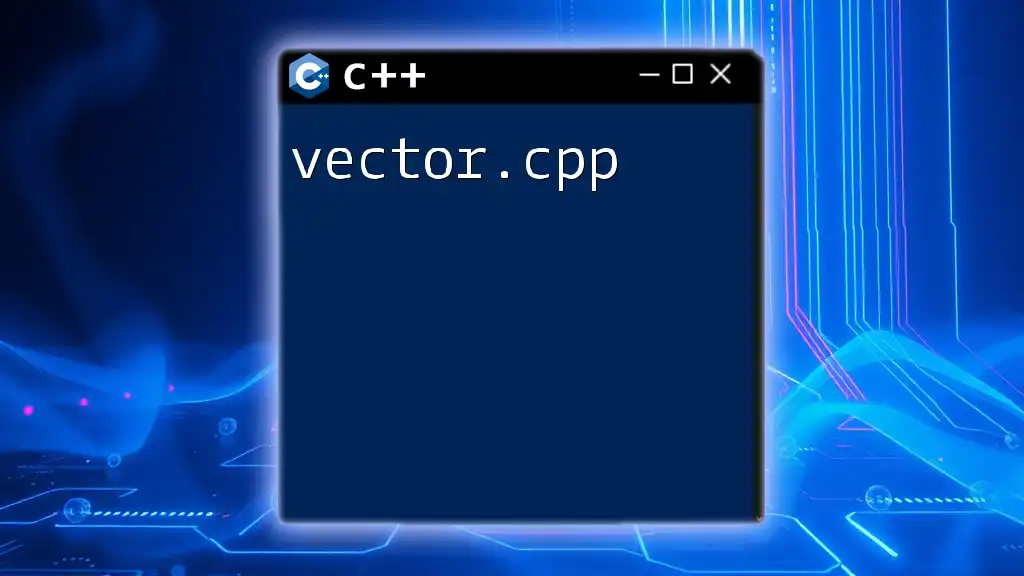
Protected vs Private: What's the Difference?
Understanding the distinction between protected and private members is essential for effective class design.
-
Protected Access: Members declared as protected can be accessed by derived classes. This promotes code reuse and facilitates the concept of inheritance, allowing subclasses to inherit essential properties without exposing those properties to all other classes.
-
Private Access: Members declared private cannot be accessed outside the defining class, including by derived classes. This restricts access entirely, which could lead to duplication of code if subclasses require similar functionalities.
Example to Illustrate Differences
class MyClass {
private:
int privateValue;
protected:
int protectedValue;
public:
MyClass() : privateValue(5), protectedValue(10) {}
void showValues() {
std::cout << "Private Value: " << privateValue << ", Protected Value: " << protectedValue << std::endl;
}
};
In this code, `privateValue` is completely hidden from derived classes and outside code, while `protectedValue` is accessible within any subclass extending `MyClass`.
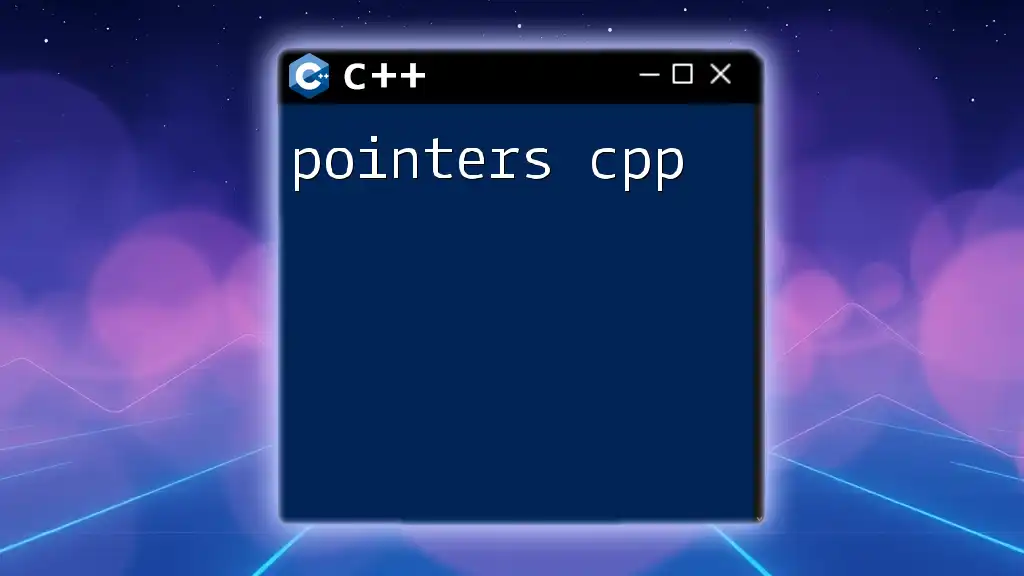
Best Practices for Using Protected Members
There are several best practices to keep in mind when using protected members:
-
Use When Necessary: Protect members should only be used when you have a clear need for subclasses to access certain variables directly. Otherwise, consider using public getter and setter methods to maintain better control.
-
Limit Use: Too many protected members can lead to tightly coupled classes, which makes your code harder to maintain. Strive for a balance between accessibility and encapsulation.
-
Document Access: When using protected members, ensure you document why they are protected and how they should be used by subclasses.
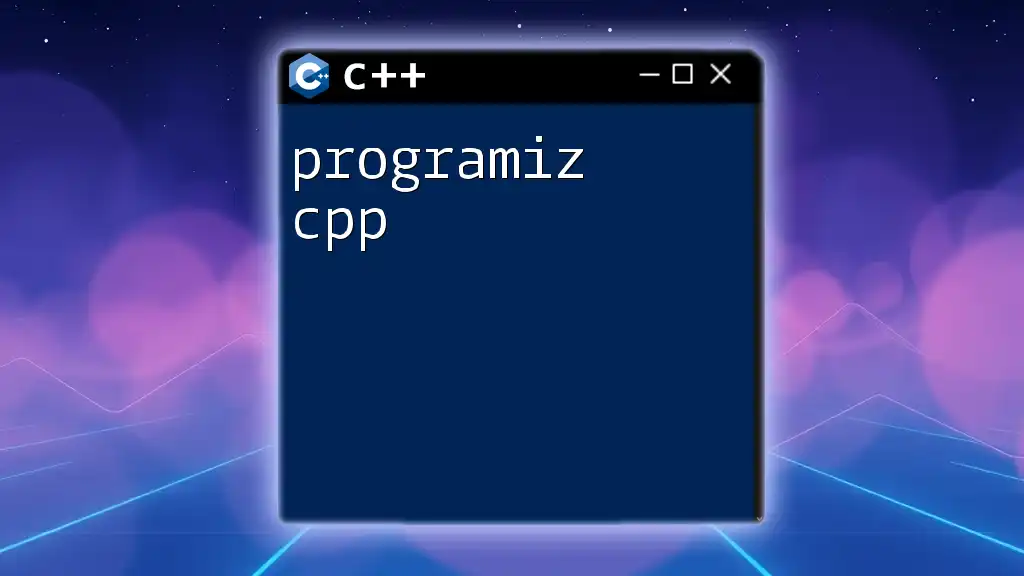
Common Mistakes with Protected Members in C++
Despite their usefulness, developers commonly make mistakes with protected members. These include:
-
Overusing Protected Members: Creating too many protected members can lead to complex class hierarchies that are challenging to manage.
-
Assuming Protected Members Are Always Safe: Just because a member is protected doesn't mean it can't be misused. It’s essential to establish clear guidelines for how subclasses should interact with protected members.
-
Neglecting Encapsulation: Relying heavily on protected members may break encapsulation principles. Ensure that your design maintains clear boundaries around class responsibilities.
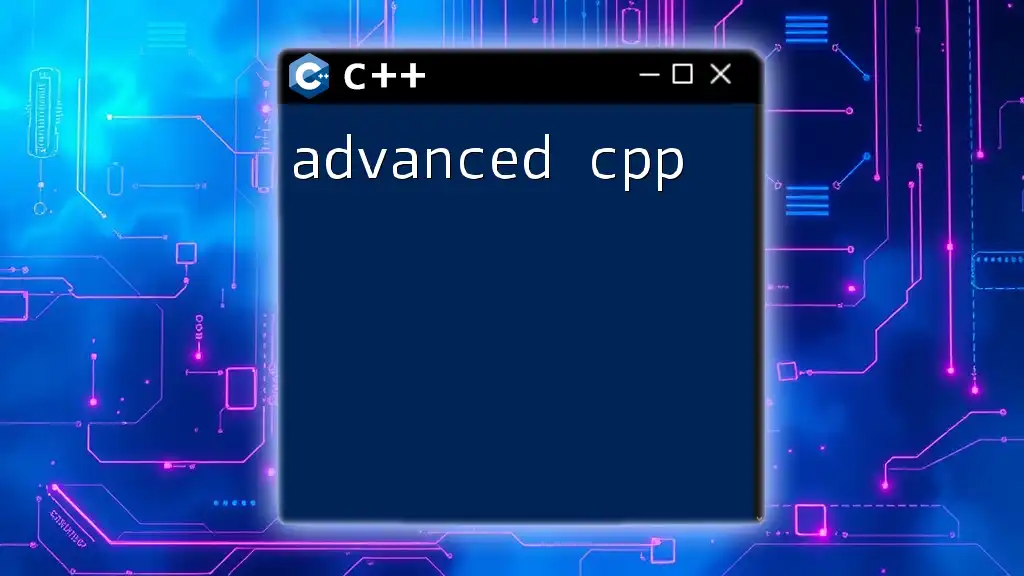
Conclusion
In summary, understanding how to use protected members in C++ is crucial for effective object-oriented programming. They provide a powerful way to control access to class members while allowing flexibility for derived classes. By leveraging protected members wisely, you can create a robust, maintainable code structure that adheres to best practices.
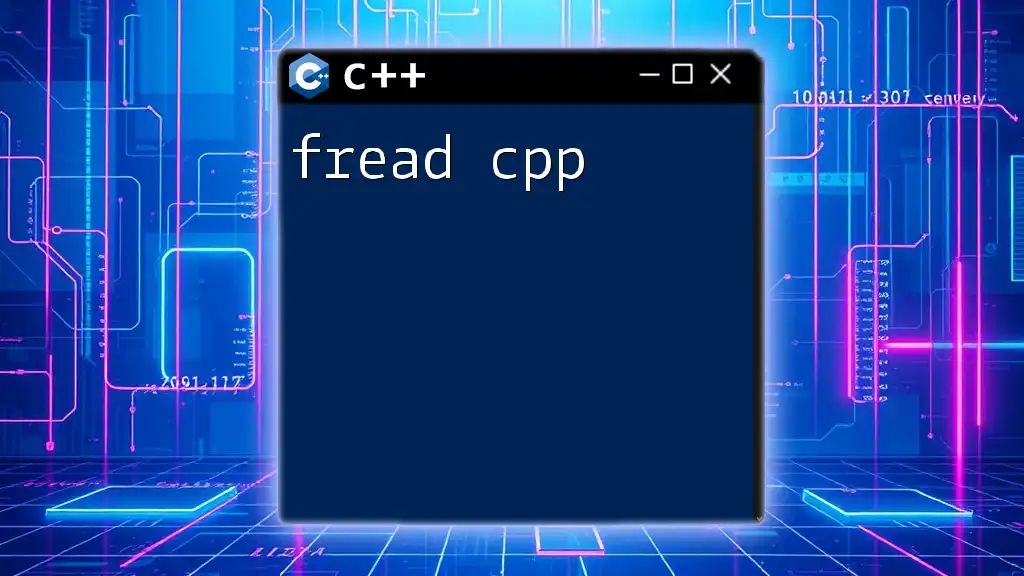
Additional Resources
For further exploration of protected in C++, consult the following resources:
- Recommended readings on access specifiers in C++.
- Online tutorials and documentation specific to C++ programming.
- C++ communities and forums to enhance your understanding and share experiences with other developers.
This understanding of the protected cpp keyword and its implications will enhance your ability to design effective class hierarchies and write cleaner, more maintainable code.