In C++, access specifiers `public`, `private`, and `protected` control the accessibility of class members, where `public` members can be accessed from anywhere, `private` members can only be accessed within the class itself, and `protected` members can be accessed within the class and by derived classes.
class Example {
public:
int publicVar; // Accessible from anywhere
private:
int privateVar; // Accessible only within Example
protected:
int protectedVar; // Accessible within Example and derived classes
};
Understanding Object-Oriented Programming in C++
Object-Oriented Programming (OOP) is a programming paradigm that allows developers to model software based on real-world entities. The core principles of OOP include Encapsulation, Inheritance, and Polymorphism. Understanding these principles is essential for effective software design.
In OOP, encapsulation refers to the bundling of data (attributes) and methods (functions) that operate on the data into a single unit known as a class. This brings us to the topic of access modifiers, which are integral to achieving encapsulation.
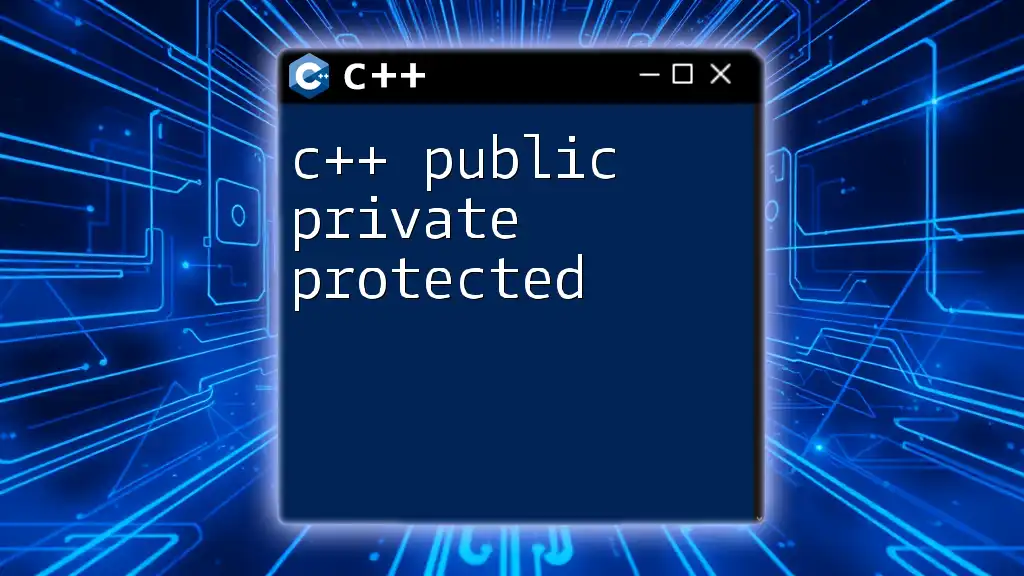
Access Modifiers in C++
Access modifiers are keywords used in class definitions to specify the visibility of class members (attributes and methods). C++ provides three primary access modifiers: public, private, and protected. Each plays a unique role in controlling access to class members, thus supporting the core principles of OOP. Let’s explore each modifier in detail.
Public Access Modifier
What is Public?
The `public` access modifier allows class members to be accessible from any part of the program. It is the least restrictive access level, making it suitable for data that should be freely available.
Example:
class Example {
public:
int publicVar;
};
In the above example, `publicVar` can be accessed from anywhere in the program.
Characteristics of Public Members
- Public members can be accessed by code outside the class definition.
- They are often used for methods that are intended to be part of the class's interface.
When to Use Public Members Use public access when you want certain members to be available for interaction, such as getters and setters or utility functions that need to be called outside the class.
Private Access Modifier
What is Private?
The `private` access modifier restricts access to class members, making them accessible only within the class itself. This provides a level of protection for the data, ensuring that it can't be modified or accessed directly from outside the class.
Example:
class Example {
private:
int privateVar;
};
Here, `privateVar` cannot be accessed from outside the `Example` class, reinforcing encapsulation.
Characteristics of Private Members
- Private members are hidden from outside access, which helps protect the internal state of the object.
- They can only be accessed through member functions or friends.
When to Use Private Members Private members should be employed when you want to protect sensitive or critical data, ensuring that it is only modified through well-defined methods within the class. This is a vital part of maintaining the integrity of the object.
Protected Access Modifier
What is Protected?
The `protected` access modifier provides a middle ground; it allows access to class members from within the class and by derived classes, but not from outside the class hierarchy.
Example:
class Base {
protected:
int protectedVar;
};
class Derived : public Base {
public:
void display() {
// Accessing protected member
std::cout << protectedVar;
}
};
In this illustration, `protectedVar` can be accessed by any derived classes but not by external code.
Characteristics of Protected Members
- Protected members enable inheritance, as they are accessible to derived classes but remain hidden from the global scope.
When to Use Protected Members Utilize protected access when you have a base class with members that should be accessible to derived classes but remain hidden from the outside world. This is particularly useful in OOP design when creating a hierarchy.
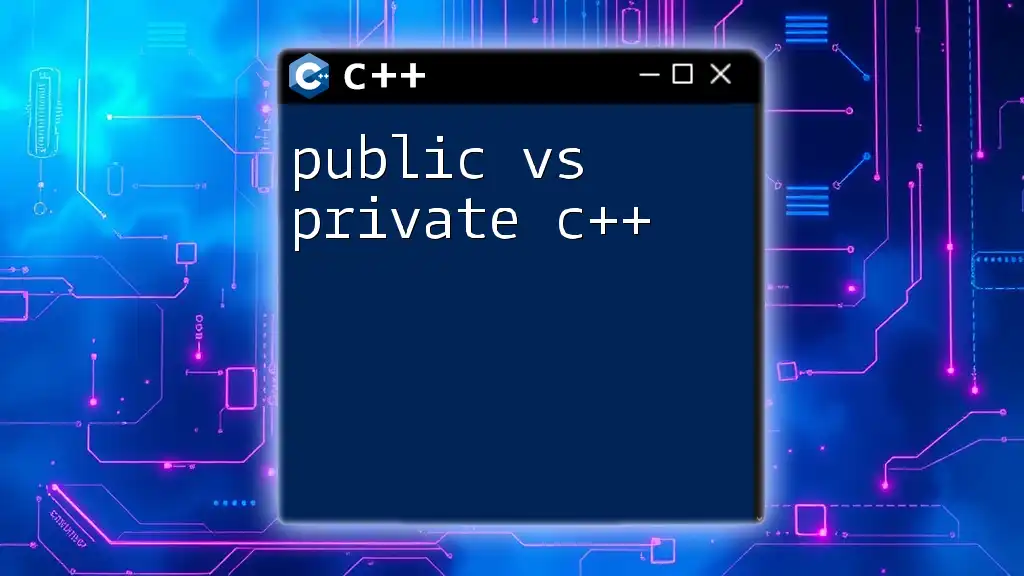
Comparing Public, Private, and Protected
To effectively understand the differences among public, private, and protected access modifiers, let’s examine a straightforward comparison:
- Public:
- Accessible from anywhere.
- Best for interface methods.
- Private:
- Accessible only within the class.
- Ideal for internal data protection.
- Protected:
- Accessible within the class and derived classes.
- Useful for inherited attributes.
Use Cases for Each Modifier Choosing an access modifier depends on the desired visibility and encapsulation needs:
- Use public for properties and methods that need to be readily accessible.
- Use private for internal data that should not be directly modified.
- Use protected for attributes that derived classes may need to use and access.
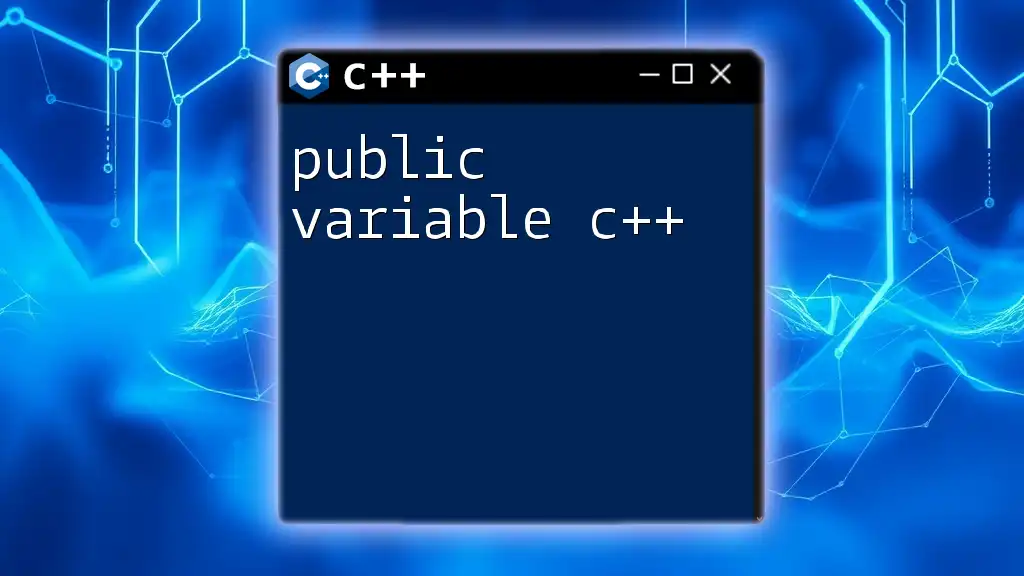
Best Practices for Using Access Modifiers
Principle of Least Privilege
A critical concept in programming is the principle of least privilege, which states that a module (class, function, etc.) should only have access to the data necessary for its operation. This principle helps you minimize vulnerabilities and potential bugs.
Encapsulation Techniques
By strategically using access modifiers, you encapsulate your objects effectively. Make sure to limit access to data when it's not necessary, thereby preserving the integrity of the object.
Code Example of Proper Encapsulation
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
double getBalance() const {
return balance;
}
};
In the `BankAccount` example, `balance` is private and can only be modified through the `deposit` method, which checks for a valid amount, thus enforcing rules for modification.
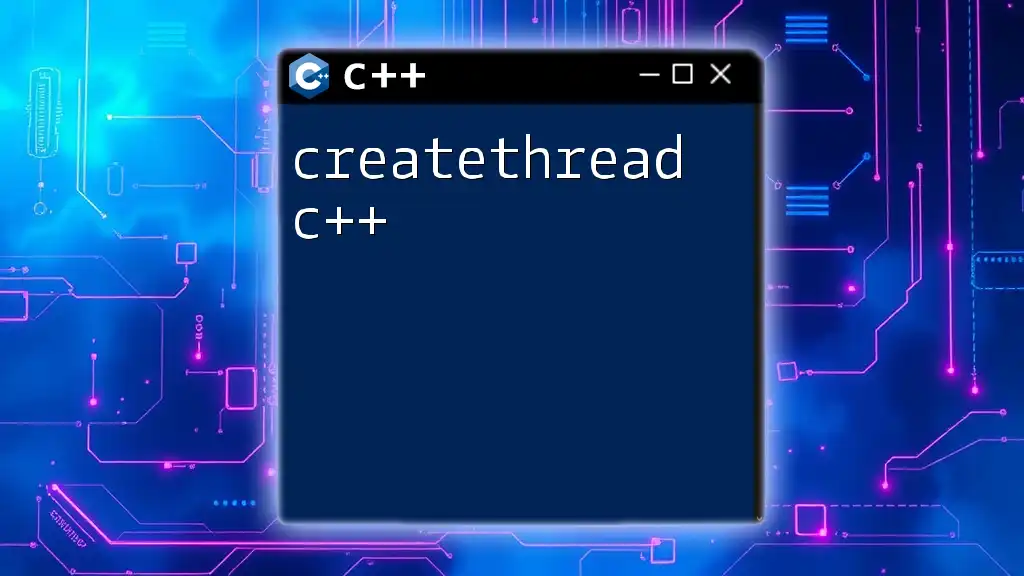
Conclusion
Understanding the distinctions between public, private, and protected access modifiers in C++ is crucial for creating robust and maintainable code. Each modifier serves a specific purpose in controlling access to class members, which is essential for good encapsulation practices.
As you continue to develop your programming skills, applying these principles will not only enhance your understanding of C++ but also improve your overall software design. Embrace the practice of encapsulation and strive for clean, efficient code by utilizing these access modifiers wisely.
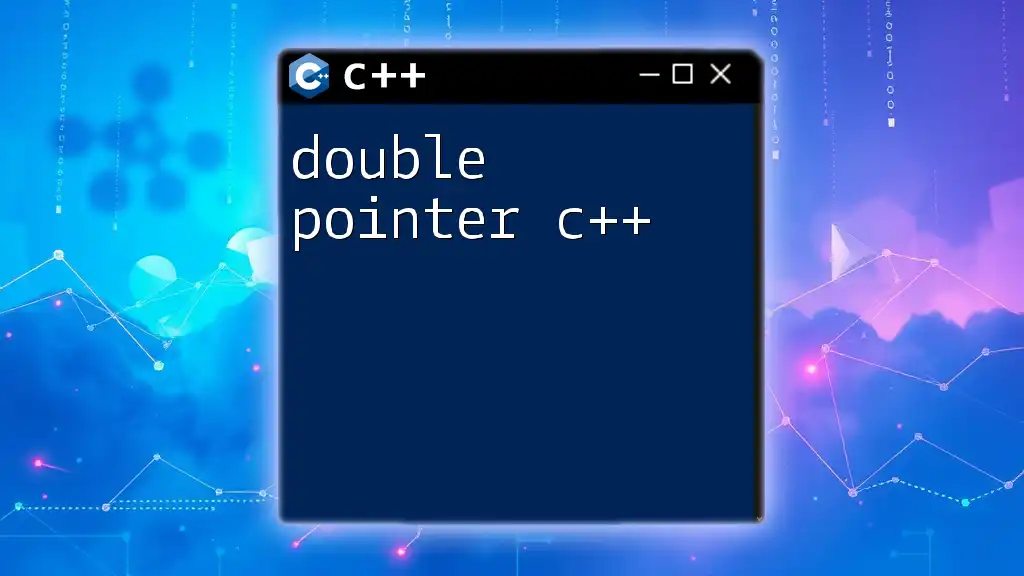
Additional Resources
To deepen your understanding of C++ and access modifiers, consider exploring further readings or online courses on C++ programming paradigms and best practices. Embrace your learning journey, and don't hesitate to experiment with your own class structures to see how access modifiers can influence your design!
By learning from mistakes and continually refining your approach to accessing class members, you'll be on your way to mastering C++ and its encapsulation capabilities.