A double variable in C++ is a data type that can hold decimal values with double precision, allowing for more accurate representation of real numbers.
double pi = 3.14159; // Example of a double variable holding the value of pi
What is a Double Variable in C++?
A double variable in C++ is a data type that is designed to hold floating-point numbers, which are numbers that can have a fractional part. The `double` type stands for "double precision", and it offers more precision than its counterpart, `float`. This increased precision is crucial when you need more accuracy in your calculations, especially in scientific and financial applications.
Difference Between `float` and `double`
The fundamental difference between `float` and `double` lies in their precision and memory requirements. A `float` typically requires 4 bytes of memory, while a `double` requires 8 bytes. This means that a `double` can represent values with more digits of precision when compared to a `float`.
For example:
- A `float` can accurately represent values roughly up to 7 decimal digits.
- A `double`, on the other hand, can handle about 15 to 16 decimal digits.
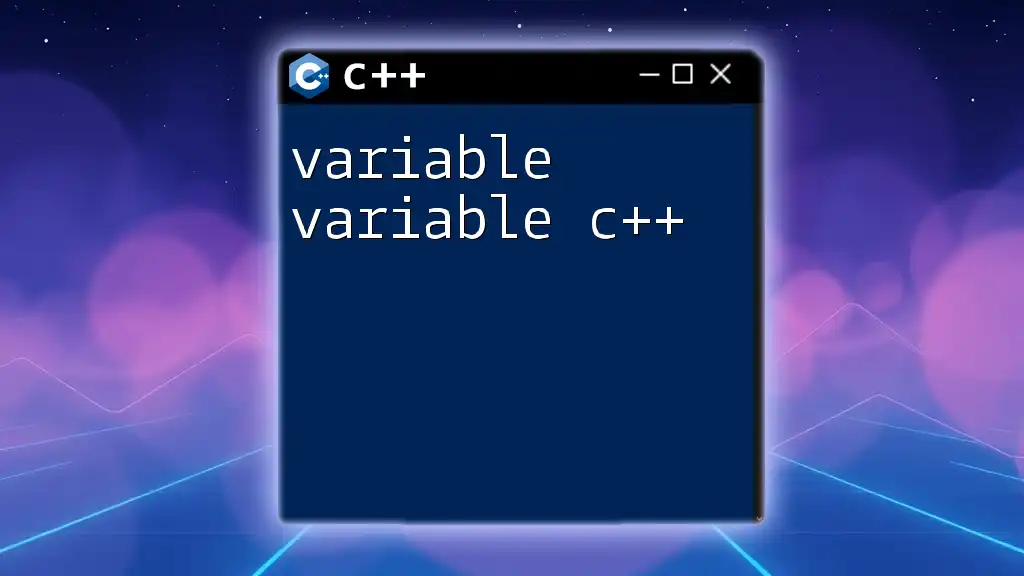
Declaring and Initializing Double Variables
Syntax for Declaration
Declaring a double variable is straightforward. The syntax involves specifying the `double` keyword followed by the variable name.
Example code snippet:
double myNumber;
Initialization Methods
You can initialize double variables in several ways.
Direct Initialization
The most common method is to initialize at the point of declaration. For instance:
double pi = 3.14159;
Assignment After Declaration
You can also declare a variable first and then assign a value to it later:
double myNumber;
myNumber = 2.5;
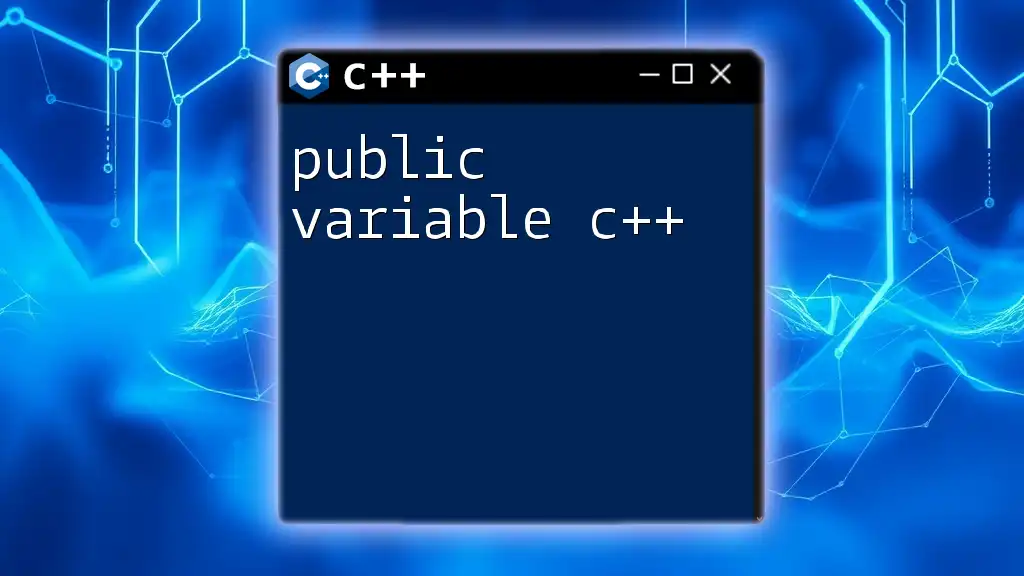
Working with Double Variables
Performing Arithmetic Operations
One of the primary uses of double variables is performing arithmetic operations. With `double`, you can easily carry out basic arithmetic such as addition, subtraction, multiplication, and division.
Here are some example code snippets demonstrating these operations:
double a = 5.5, b = 2.2;
double sum = a + b; // Addition
double difference = a - b; // Subtraction
double product = a * b; // Multiplication
double quotient = a / b; // Division
Using Functions with Double
C++ provides a number of library functions that support double variables, enhancing your ability to perform mathematical operations. Common functions include `sqrt`, `pow`, and `sin`.
For example, to compute the square root using `sqrt`:
#include <cmath>
double result = sqrt(25.0); // Square root
This feature showcases the versatility of `.cpp` and allows for complex mathematical calculations with ease.
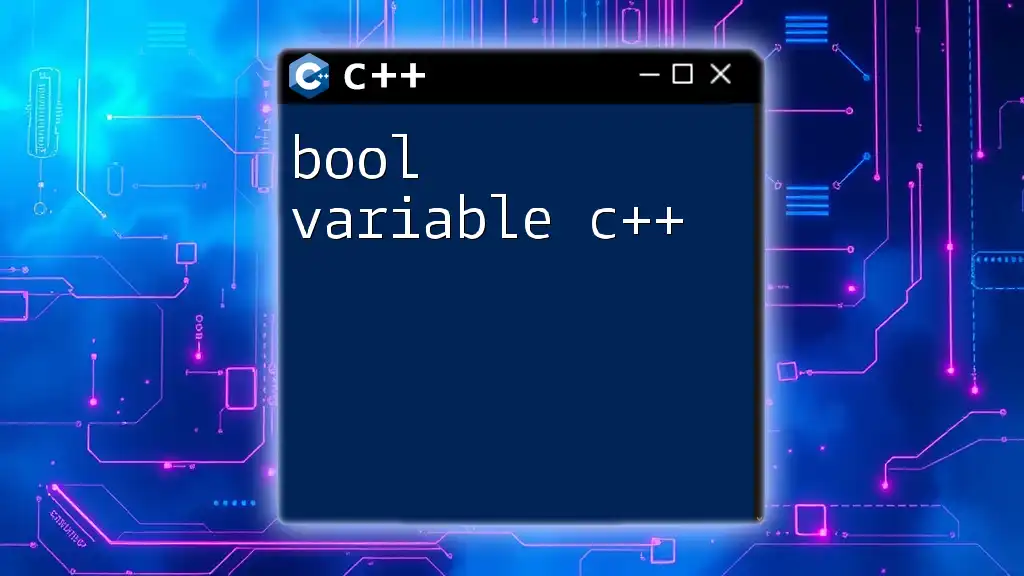
Type Casting and Double Variables
Implicit vs Explicit Casting
In C++, type conversion can happen automatically (implicit cast) or through explicit casting.
An example of implicit casting would be:
int wholeNumber = 10;
double convertedNumber = wholeNumber; // Implicit casting
In this case, the integer `wholeNumber` is automatically converted to a double.
Using `static_cast`
Sometimes, explicit casting is necessary for clarity and control over your code. The `static_cast` keyword allows for safe casting between types.
Example:
double doubleNumber = static_cast<double>(5); // Explicit casting
This method ensures that the conversion is intentional and understood.
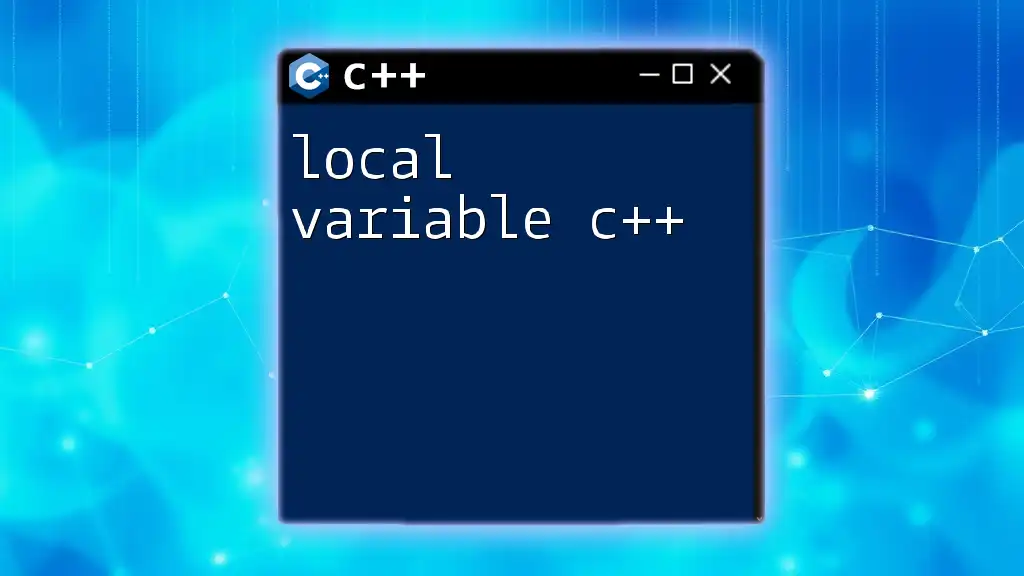
Precision and Rounding Issues with Double Variables
Understanding Precision
Although `double` variables can express a broad range of numbers with high precision, they are not immune to rounding errors. Due to how floating-point arithmetic works, very small differences can appear after calculations, leading to unexpected results.
Using `<iomanip>` for Formatting
To handle precision effectively, you can use the `<iomanip>` library, which provides manipulators like `std::setprecision` to format how your output appears.
Here’s a code example illustrating how to set the precision of output:
#include <iostream>
#include <iomanip>
double value = 0.1 + 0.2;
std::cout << std::setprecision(2) << value; // Set precision to 2
This feature not only makes your output clearer but also reinforces the significance of precision when dealing with `double` types.
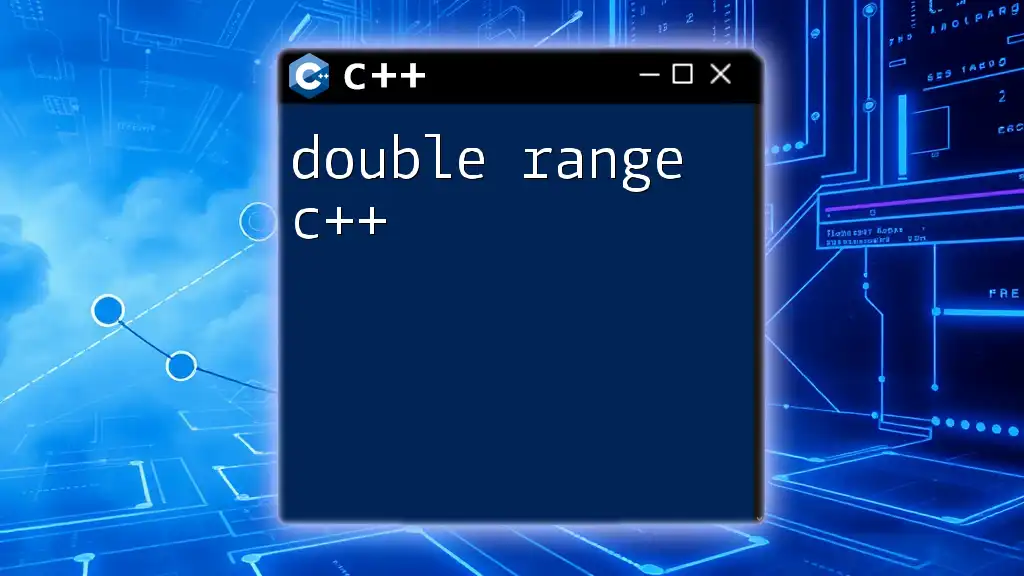
Common Mistakes When Using Double Variables
Comparing Double Values
One frequent pitfall is comparing double values directly. Due to precision limitations and rounding errors, two double values that seem equal may not be precisely the same in binary representation.
To avoid this, you should implement a tolerance threshold:
if (fabs(a - b) < 0.0001) {
// Treat a and b as equal
}
This method helps mitigate issues arising from floating-point comparisons.
Initializing Without Assigning
Always initializing double variables is good practice to prevent undefined behavior. Failing to do so can lead to unexpected results in your application.
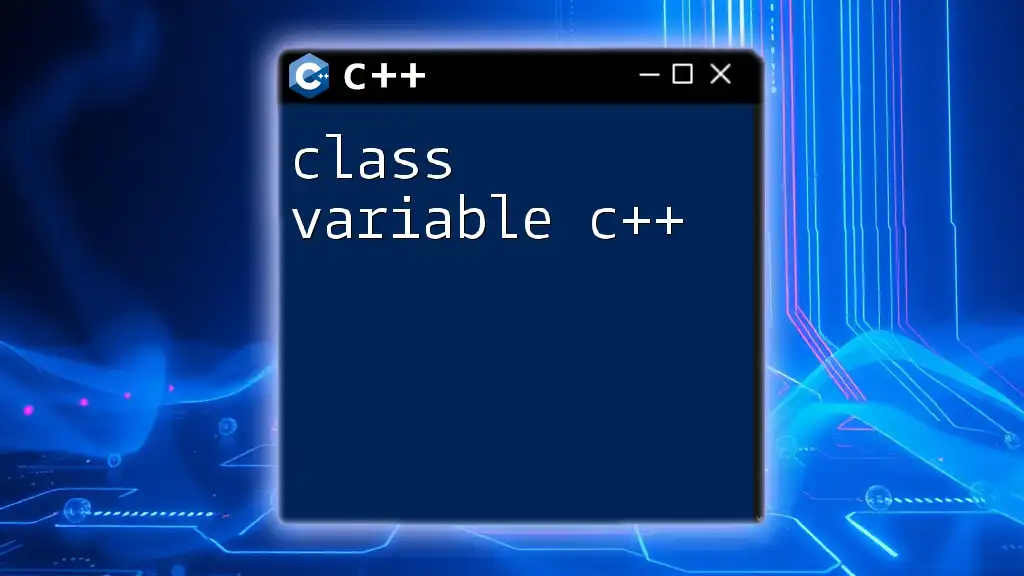
Practical Applications of Double Variables
Scientific Calculations
Double variables are extensively used in scientific computations where precision is paramount. These applications might include physics simulations, data modeling, or any task requiring accurate calculations of real-world phenomena.
Financial Applications
Similarly, in the financial realm, ensuring calculation precision is vital, especially during monetary transactions. As the stakes are high, even a small rounding error might result in significant financial discrepancies.
Here’s a simple example demonstrating simple interest calculation:
double principal = 1000.0;
double rate = 5.0; // 5%
double time = 2.0; // 2 years
double interest = (principal * rate * time) / 100; // Simple Interest
This example illustrates how `double` types can be directly applied to real-world financial calculations.
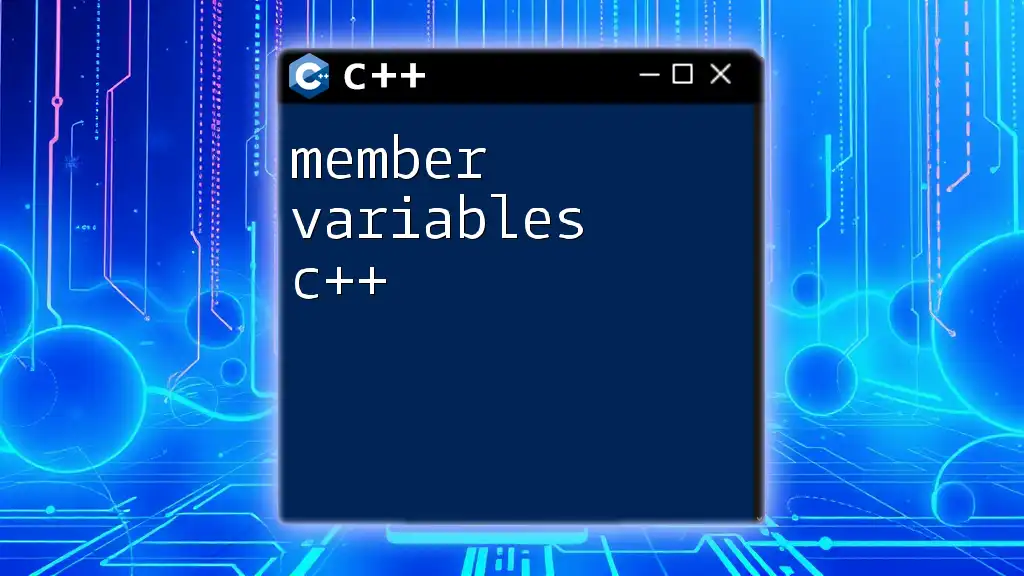
Conclusion
In summary, understanding double variables in C++ is essential for anyone looking to perform numeric computations with high precision. By grasping how to declare, initialize, and manipulate double variables effectively, you can harness the full power of C++ for your programming projects. Always practice and experiment with double variables to solidify your knowledge and application skills.
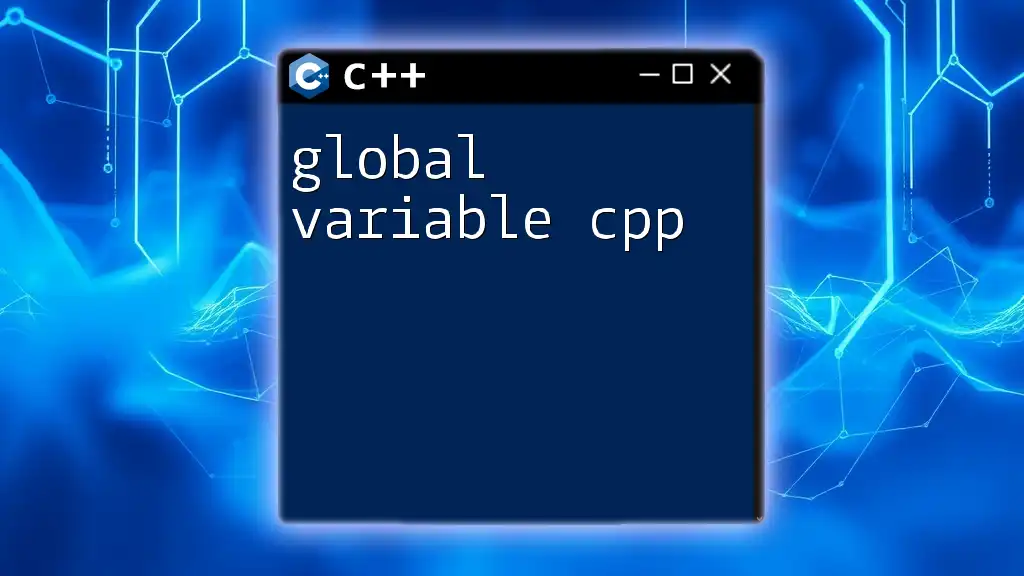
Additional Resources
For those eager to delve deeper into C++ and the intricacies of double variables, consider exploring online tutorials, documentation, and community forums dedicated to programming languages and numeric computation techniques.