A "double range" in C++ often refers to a range of double values created using standard libraries like `<algorithm>` or `<numeric>` for iteration or manipulation of floating-point numbers.
Here's an example of how you might use a double range with `std::vector`:
#include <vector>
#include <iostream>
#include <algorithm>
int main() {
std::vector<double> values = {1.5, 2.5, 3.5, 4.5};
std::for_each(values.begin(), values.end(), [](double val) {
std::cout << val << " ";
});
return 0;
}
Understanding the Basics of C++ Ranges
C++ ranges are a powerful feature introduced in C++20 that allow developers to handle sequences of elements more efficiently and intuitively. They provide a higher-level abstraction for iterating over a range of values and support various operations, such as transformation and filtering.
Key Features of Ranges
One of the primary advantages of ranges is their ability to work with iterable sequences directly. Ranges promote lazy evaluation, meaning computations are deferred until absolutely necessary. This can lead to performance improvements because not all values need to be computed at once. Additionally, using ranges can enhance code readability, making it easier to express complex data manipulations.
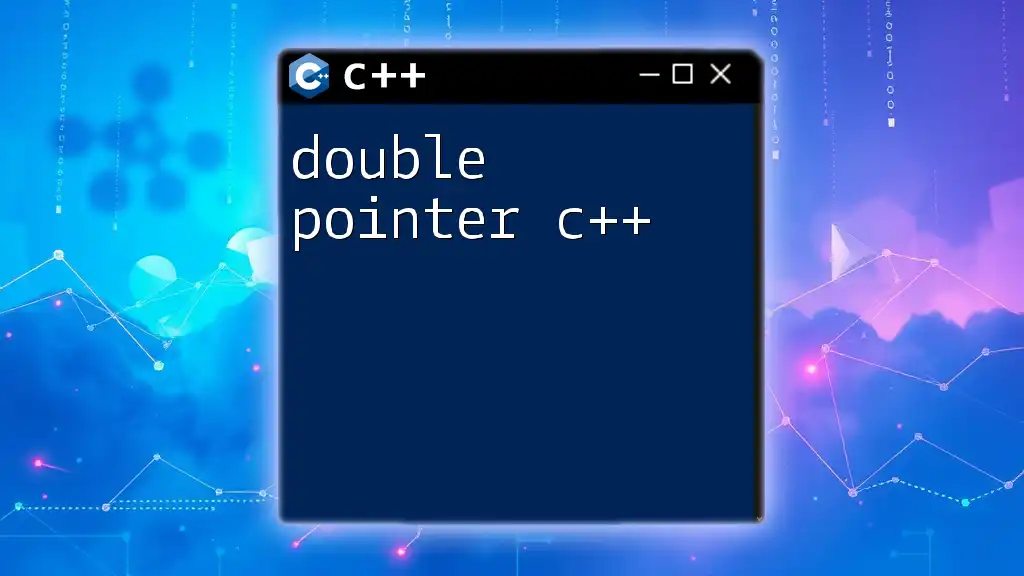
The Concept of Double Ranges
A double range refers to working with sequences of floating-point numbers (specifically `double` type) in the context of C++ ranges. Understanding how to effectively use double ranges can streamline your code and make it much clearer, particularly when dealing with numerical data.
Why Use Double Ranges?
Utilizing double ranges is particularly beneficial in scenarios involving mathematical computations or data analysis where precision is key. They allow you to leverage the full power of the C++ ranges library to perform operations such as transformations and aggregations seamlessly.
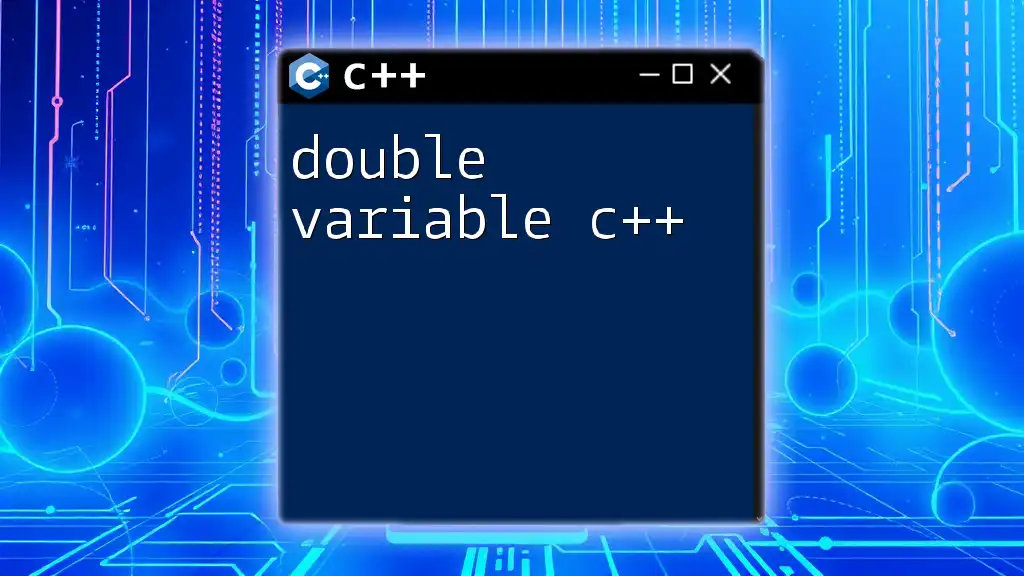
Implementing Double Ranges in C++
Syntax for Double Ranges
The syntax for double ranges follows the same principles as regular ranges. Here's a brief explanation of the syntax involved in creating and using double ranges:
- Use the `std::views` namespace to create views of your data, allowing you to express transformations.
- Employ features like `std::views::transform` and `std::views::filter` to manipulate and filter data.
Example of Double Range Usage
Let's look at a simple example of how to use double ranges in practice. Below is a code snippet demonstrating how to double the values in a vector of doubles.
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<double> values = {1.1, 2.2, 3.3, 4.4};
auto result = values | std::views::transform([](double val) { return val * 2; });
for (double val : result) {
std::cout << val << " "; // Output: 2.2 4.4 6.6 8.8
}
return 0;
}
In this example, we create a vector of doubles and use `std::views::transform` to generate a new range where each element in the original vector is doubled. The results are printed to the console.
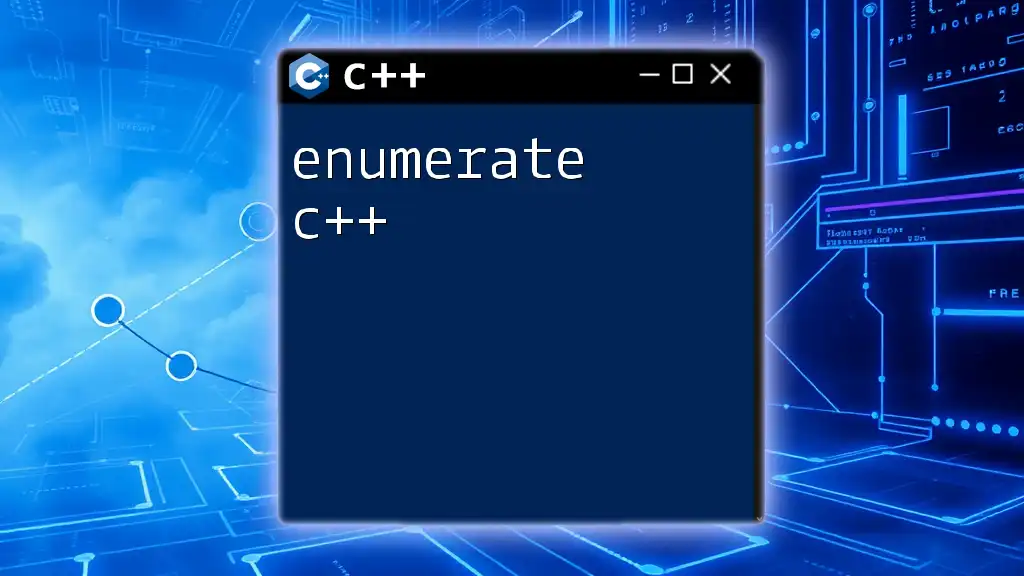
Advanced Techniques with Double Ranges
Combining Double Ranges with Other Algorithms
One of the key advantages of using double ranges lies in their compatibility with existing C++ algorithms. You can apply filters or transformations in a way that is composable and concise. Consider the following code snippet that filters values greater than 2.0.
auto filtered = values | std::views::filter([](double val) { return val > 2.0; });
Chaining Double Ranges for Complex Operations
Chaining allows you to execute multiple operations sequentially without intermediate variables for improved readability. Here's an example that combines filtering and transforming into one coherent pipeline.
auto complex_result = values | std::views::filter([](double val) { return val > 1.0; })
| std::views::transform([](double val) { return val + 1.0; });
In this instance, we first filter the values to include only those greater than 1.0 and then transform them by adding 1. This illustrates how double ranges can facilitate complex data manipulations in a clean and efficient manner.
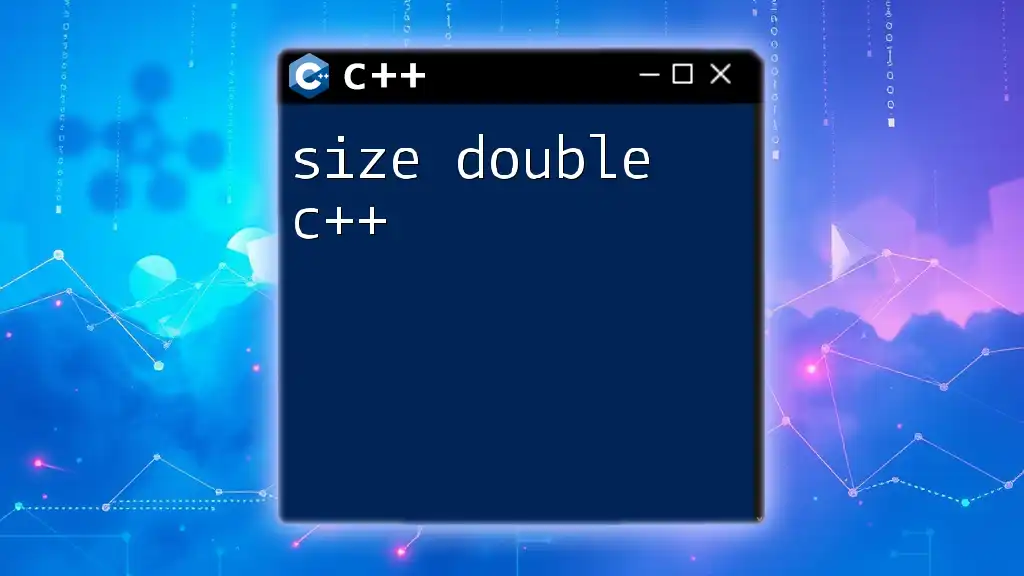
Common Pitfalls to Avoid with Double Ranges
Working with double ranges comes with its unique challenges. Understanding the lifetime and scope of your range objects is crucial. When ranges are created, they remain valid only as long as the underlying data exists. Failure to maintain appropriate lifetimes may lead to accessing invalid data.
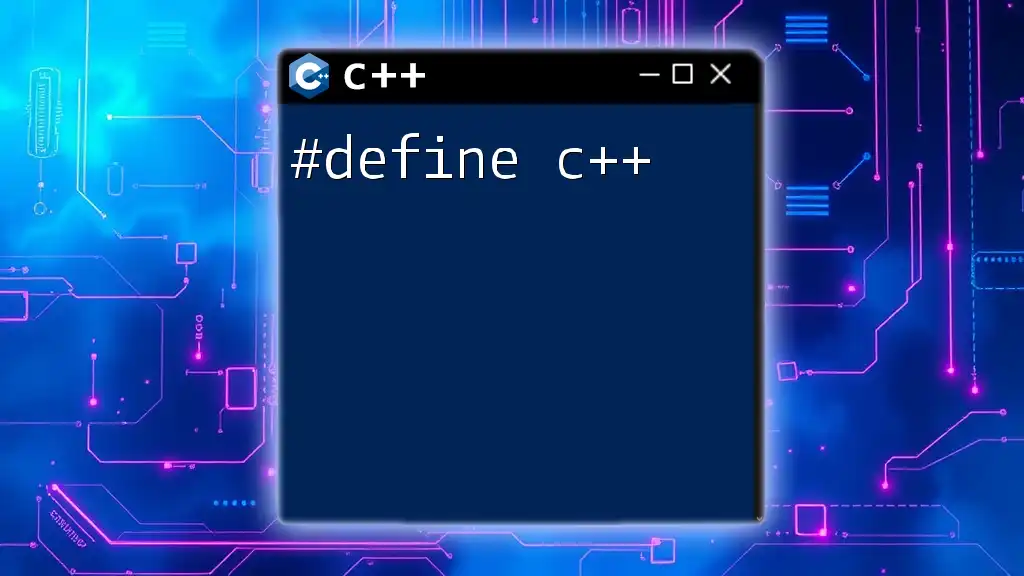
Performance Considerations with Double Ranges
Efficiency of Using Double Ranges
Double ranges provide significant performance advantages due to their lazy evaluation and optimized pipelines. Using ranges effectively minimizes memory overhead and speeds up computations, especially when working with large datasets.
Profiling Double Range Performance
To assess the efficiency of your double ranges, you may want to use profiling tools like `gprof` or `Valgrind`. These tools can help pinpoint bottlenecks in your code, allowing you to validate the performance improvements that double ranges offer.
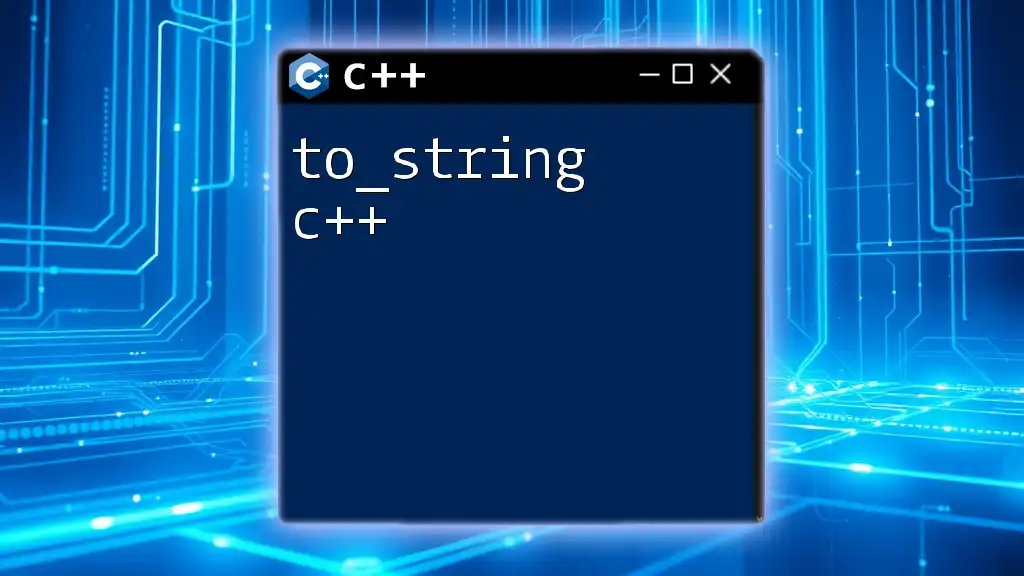
Practical Applications of Double Ranges in C++
Real-World Examples of Double Range Use
Double ranges shine in scenarios such as data processing, scientific simulations, and numerical analysis, where large datasets need to be manipulated quickly and accurately. By reducing boilerplate code and enhancing clarity, double ranges allow for more efficient algorithm implementation.
Case Study
Consider a case study where we analyze a dataset of temperature readings collected over time. By using double ranges, we can quickly compute averages, apply filters for specific criteria, and transform the data for visualization while keeping the code concise. This showcases how double ranges elevate programming efficiency.
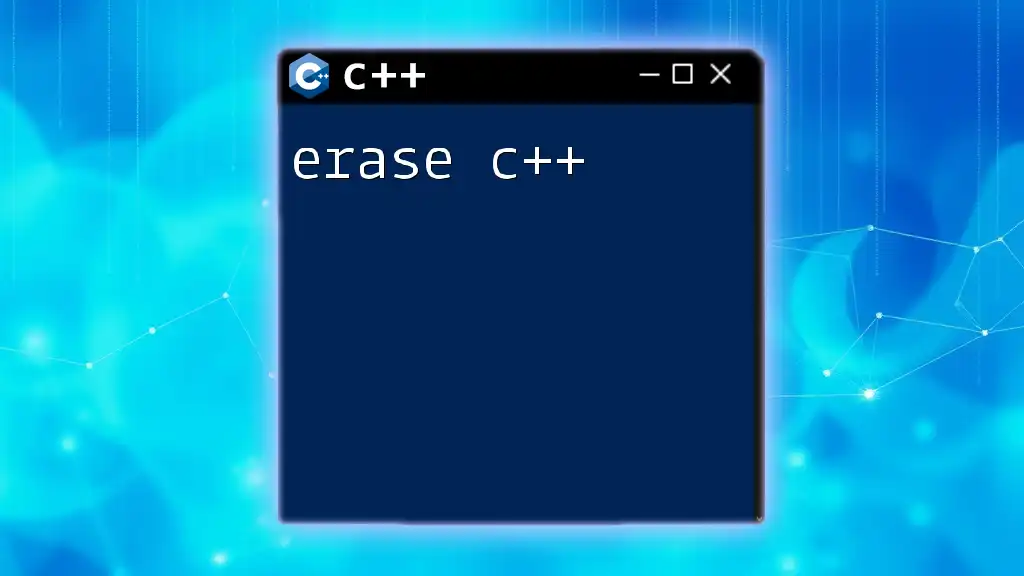
Conclusion
Mastering double ranges in C++ is essential for developers looking to enhance their coding practices in data-centric applications. With their clear syntax and powerful capabilities, double ranges allow for more intuitive and efficient manipulation of floating-point data.
As you explore this feature, don't hesitate to experiment with your own examples. The power of double ranges awaits to streamline your coding experience!
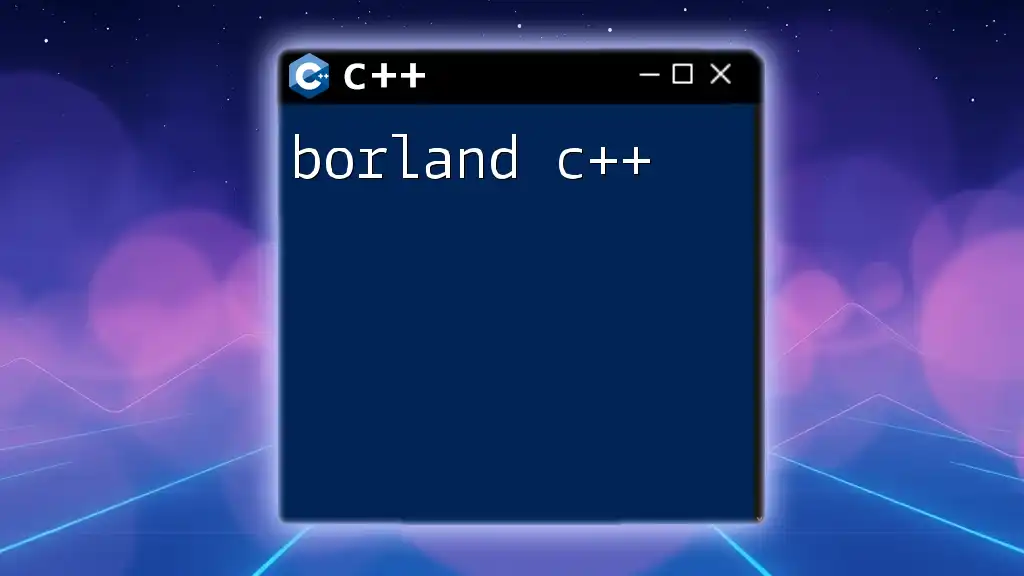
Additional Resources
To further your understanding of double ranges in C++, consider exploring recommended books and tutorials specifically on C++ ranges and algorithms. Engaging with online communities and forums dedicated to C++ can also provide valuable insights and ongoing learning opportunities.