In C++, `enum` is a user-defined type that consists of a set of named integral constants, which enhances code readability and maintainability.
Here's a simple code snippet demonstrating the use of `enum`:
enum Color { Red, Green, Blue };
int main() {
Color myColor = Green;
return 0;
}
What is an Enumeration?
In C++, an enumeration (often referred to as an enum) represents a user-defined type that allows developers to work with a set of named integral constants. Enumerations provide a way to define collections of related constants in a clean and readable manner. By using enums, programmers can improve code readability and maintainability, allowing for better logic representation compared to plain integers.
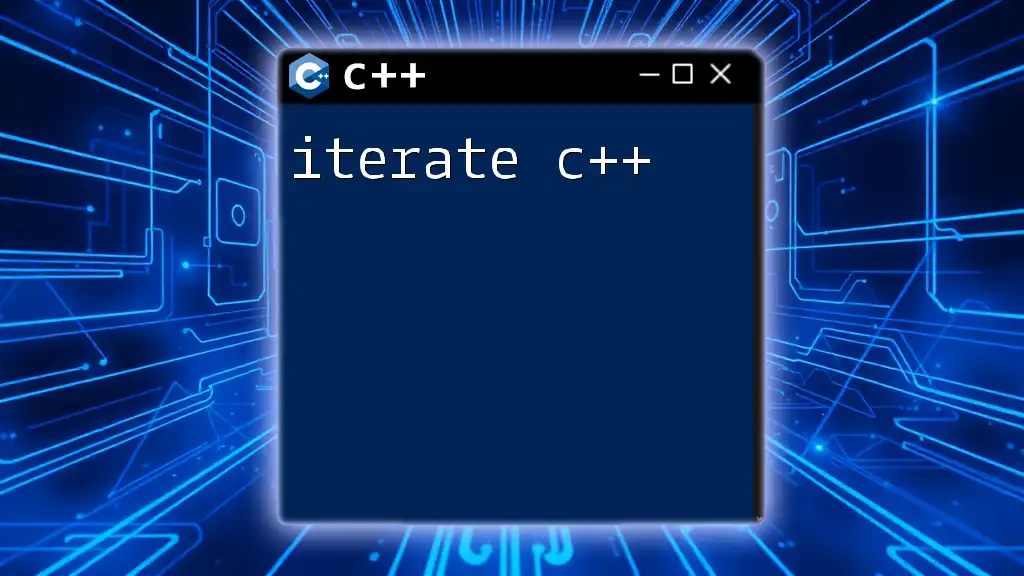
Declaring an Enumeration
To declare an enumeration in C++, you can use the following syntax:
enum EnumName { Constant1, Constant2, Constant3, ... };
Here’s an example of a simple enumeration declaration that represents colors:
enum Color { Red, Green, Blue };
In this case, `Red`, `Green`, and `Blue` are named constants. C++ automatically assigns the integral values starting from 0 to the first constant, 1 to the second, and so on. Thus, `Red` is 0, `Green` is 1, and `Blue` is 2. This can be particularly useful for improving code clarity by replacing cryptic numbers with meaningful names.
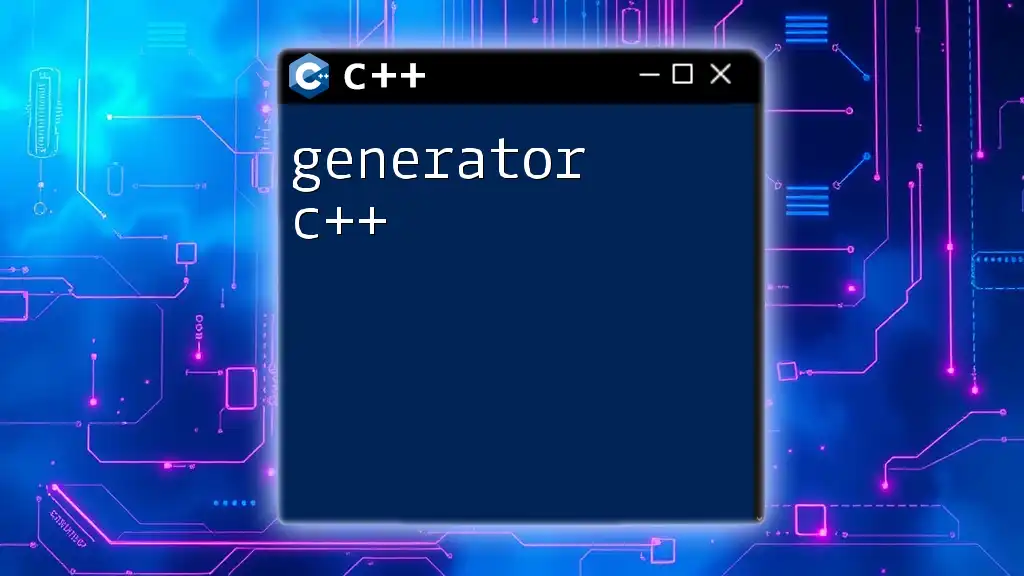
Working with Enumerations
Accessing Enumeration Values
Once an enumeration is declared, you can use its values in your code just like any other integral type. Here’s how you can utilize an enumeration in a switch statement:
Color myColor = Red;
switch (myColor) {
case Red:
std::cout << "Selected Color: Red\n";
break;
case Green:
std::cout << "Selected Color: Green\n";
break;
case Blue:
std::cout << "Selected Color: Blue\n";
break;
}
This code snippet demonstrates how to check the value of `myColor` and execute different actions based on that value. Using enums in switch statements not only improves clarity, but it also reduces the chances of bugs due to incorrect value comparisons.
Assigning Custom Values
Enums also allow you to assign specific values to each constant, which can be beneficial for a variety of reasons, such as mapping constants to existing codes. You can specify values like this:
enum ErrorCode {
SUCCESS = 0,
WARNING = 1,
ERROR = 2
};
In this example, you are explicitly defining the values associated with the enum. This is useful when you need a particular representation or integration with other systems, as you can map these values directly to corresponding statuses.
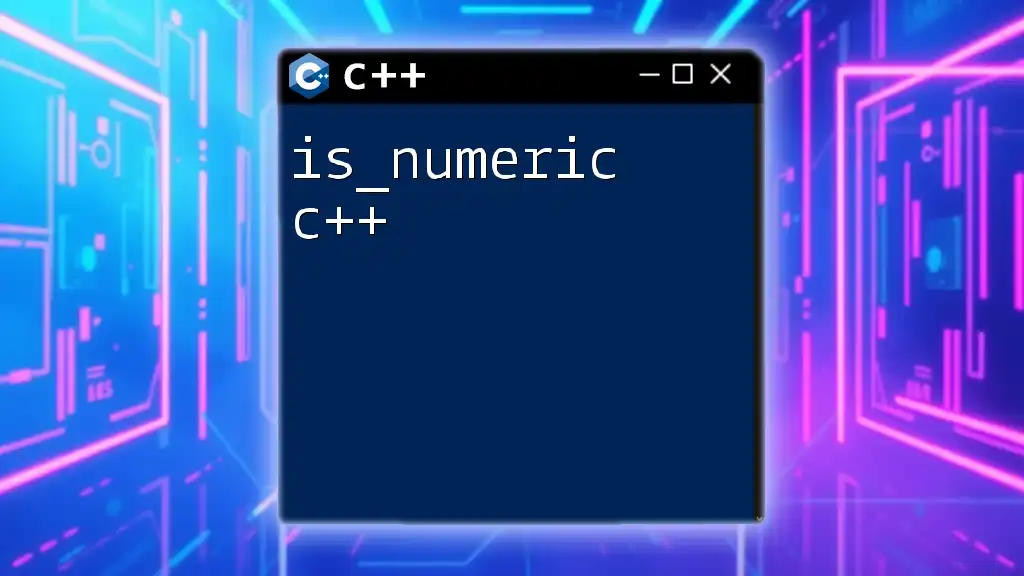
Enumerated Types and Scope
Structuring Enumerations to Avoid Conflicts
In larger projects, naming conflicts can become an issue as different parts of the code may define enumerations (or other identifiers) with the same name. To resolve this, you can use namespacing.
For instance:
namespace VehicleType {
enum Type { Sedan, SUV, Truck };
}
namespace AnimalType {
enum Type { Dog, Cat, Bird };
}
By encapsulating the enums within different namespaces, you can avoid any potential conflicts between the `Type` enums in `VehicleType` and `AnimalType`. This practice is especially crucial in complex systems where multiple modules might have similar enumerations.
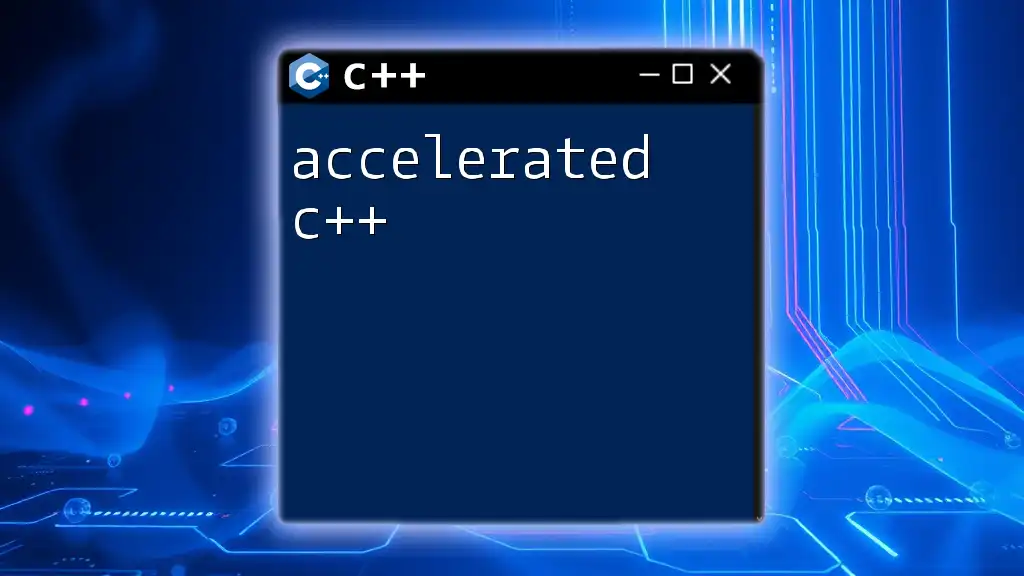
Using `enum class`
Moving Beyond Traditional Enumerations
C++11 introduced a new feature called `enum class`, which enhances the traditional enumeration by providing strong typing and scope resolution. With `enum class`, you avoid type conversion issues and improve clarity in your code.
Here's how to declare an `enum class`:
enum class Direction { North, South, East, West };
In this case, `North`, `South`, `East`, and `West` are contained within the `Direction` scope, which means you will need to prefix the enumeration values with `Direction::` when accessing them.
Example with `enum class`
Using `enum class` in a switch statement might look like this:
Direction dir = Direction::North;
switch (dir) {
case Direction::North:
std::cout << "Facing North\n";
break;
case Direction::South:
std::cout << "Facing South\n";
break;
case Direction::East:
std::cout << "Facing East\n";
break;
case Direction::West:
std::cout << "Facing West\n";
break;
}
With `enum class`, you significantly reduce the chance of ambiguous or incorrect value assignments, as only the values defined within that particular enumeration can be used.
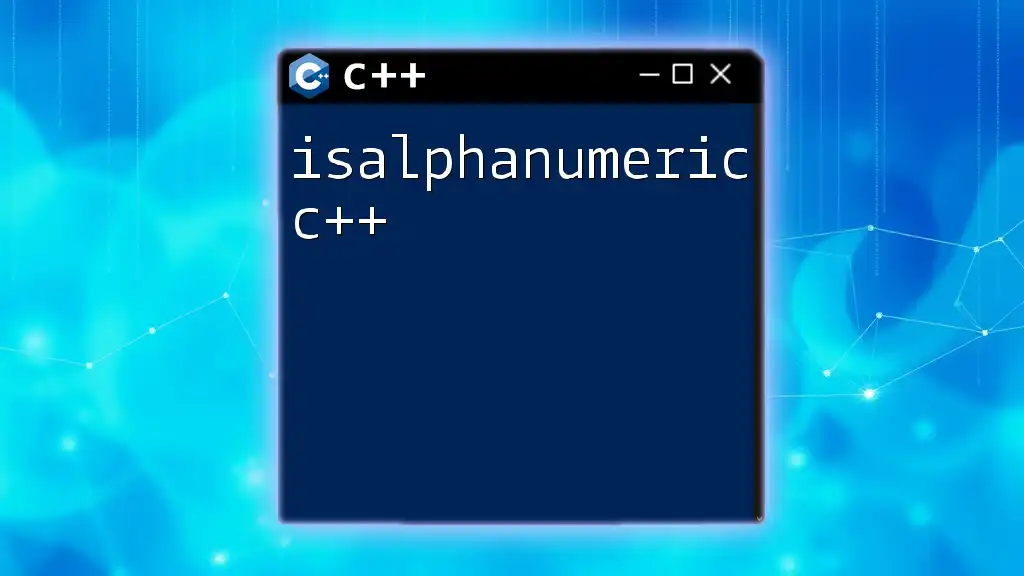
Best Practices for Using Enumerations
When working with enumerations in C++, consider the following guidelines:
- Use enums to improve code clarity: Replacing numbers with meaningful names makes your code self-documenting, which aids in future maintenance.
- Avoid overlap: Ensure that enumeration names and values do not conflict with other identifiers in your code to prevent confusion and errors.
- Use `enum class` where applicable: Leverage the benefits of strong typing and scoping offered by `enum class` features to mitigate bugs and maintain clarity.
Properly documenting enums and their purpose will further help other developers and even your future self understand your code’s logic.
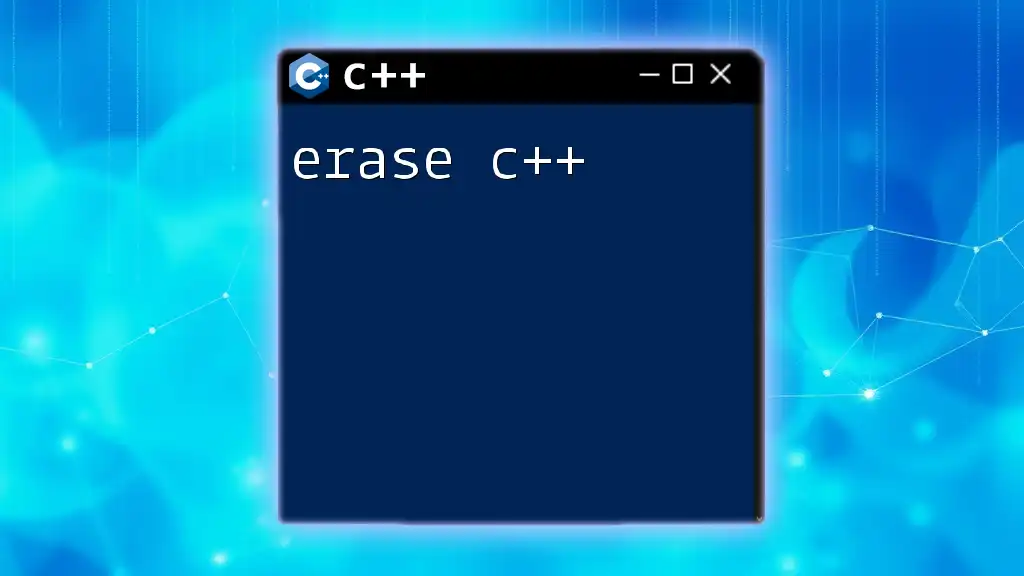
Conclusion
Enumerated types in C++ provide a robust, clear, and intuitive way to work with sets of related constants. By understanding how to declare, utilize, and best practices surrounding enumerations, you can write more readable and maintainable code. Embrace enumerations in your coding practices, and watch your code clarity soar to new heights.
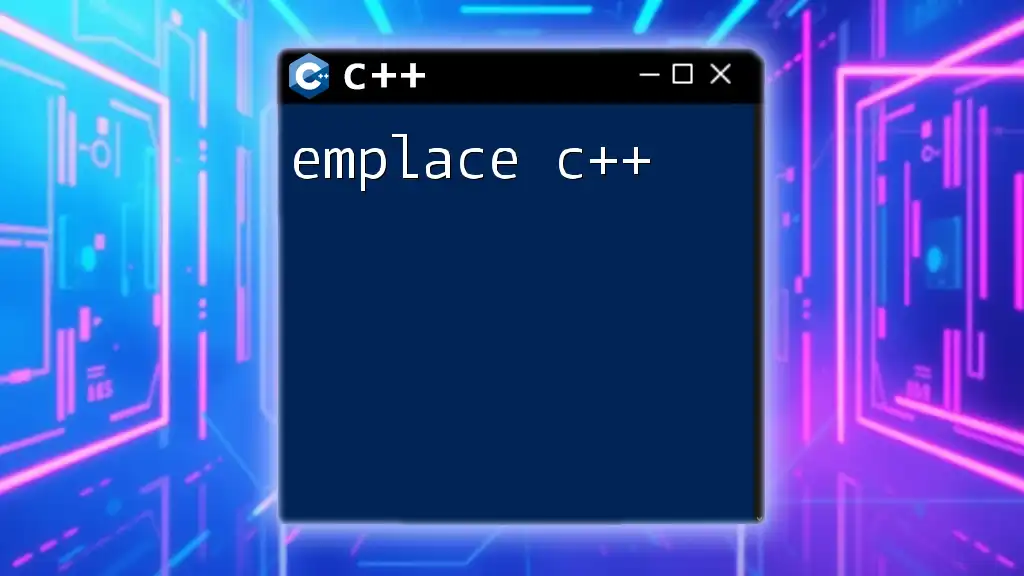
Additional Resources
To deepen your understanding of C++ enumerations beyond this guide, consider exploring recommended books, engaging in online forums, or experimenting with various coding exercises on reliable platforms that focus on C++.