`protobuf_generate_cpp` is a CMake macro that automatically generates C++ source files from Protocol Buffer (.proto) definitions, enabling developers to incorporate Protocol Buffers into their C++ projects seamlessly.
Here’s a simple example of how to use it in your `CMakeLists.txt` file:
proto_generate_cpp(PROTO_SRCS PROTO_HDRS your_proto_file.proto)
Understanding `protobuf_generate_cpp`
Overview of `protobuf_generate_cpp`
`protobuf_generate_cpp` is an essential command used in CMake for generating C++ source files from Protocol Buffer (`.proto`) definitions. This command simplifies the integration of data serialization in C++ applications by automatically creating the necessary C++ classes and methods to handle your defined messages. This tool is especially effective in large applications where data interchange between services is vital.
How `protobuf_generate_cpp` Works
To use `protobuf_generate_cpp`, you typically include it in your CMake configuration. This command leverages the Protocol Buffers compiler to produce C++ classes that correspond to the Protocol Buffer messages defined in your `.proto` files. This means you won't need to manually write parsing and serialization code, thereby reducing errors and saving time.
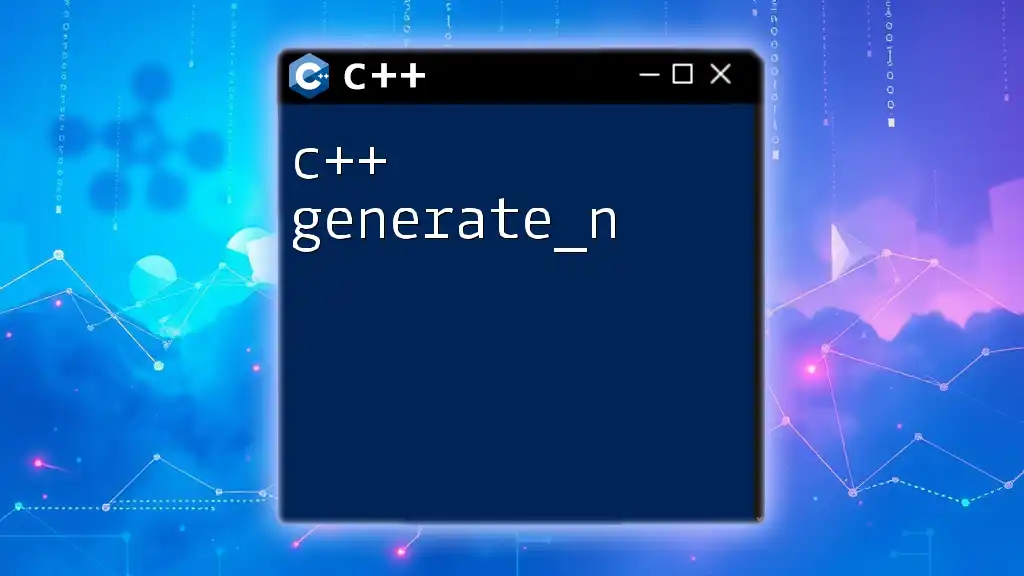
Setting Up Your Environment for `protobuf_generate_cpp`
Required Tools and Libraries
Before diving into `protobuf_generate_cpp`, ensure your development environment is equipped with the following:
- Protocol Buffers library: The core library necessary for using Protocol Buffers.
- CMake: A powerful build system generator that simplifies the compilation and linking process for your applications.
- C++ Compiler: Ensure that you are using a compatible C++ compiler that works well with CMake and Protocol Buffers.
Installing Protocol Buffers
Installation steps may vary depending on your operating system. Here’s a quick guide:
- Windows: Use vcpkg or download binaries from the official Protocol Buffers GitHub repository.
- macOS: Install via Homebrew with the command:
brew install protobuf
- Linux: Most distributions have Protocol Buffers in their repositories. For example, on Ubuntu, use:
sudo apt-get install protobuf-compiler libprotobuf-dev
After installation, verify that Protocol Buffers is correctly set up by running:
protoc --version
The command should display the installed version of Protocol Buffers.
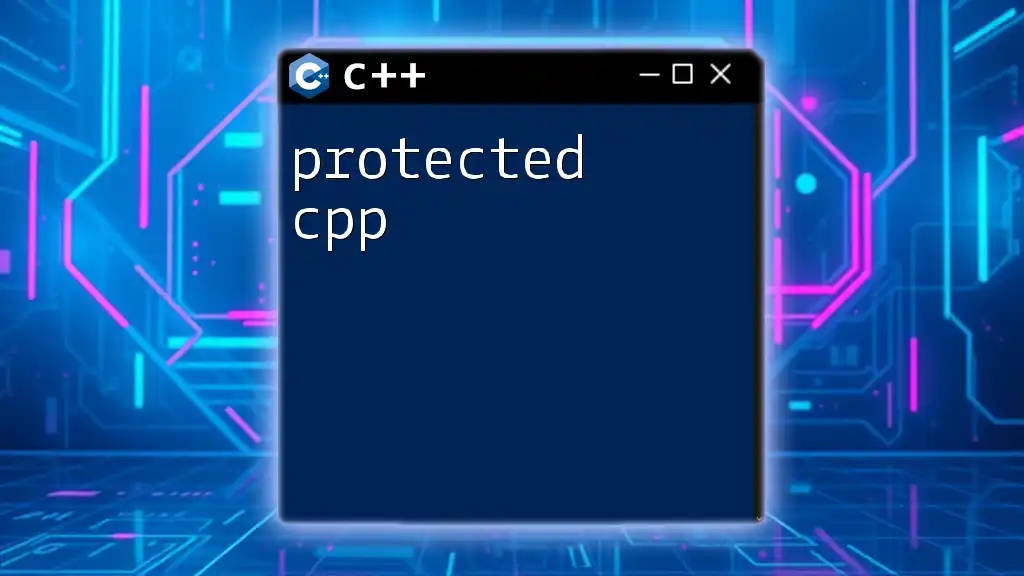
Using `protobuf_generate_cpp` with CMake
Creating a Basic CMake Project
The first step in using `protobuf_generate_cpp` is creating a CMake project. Here’s an example project structure:
MyProject/
│
├── CMakeLists.txt
├── main.cpp
└── myprotofile.proto
Integrating `protobuf_generate_cpp`
Syntax and Parameters
In your `CMakeLists.txt`, include the following lines to use `protobuf_generate_cpp`:
find_package(Protobuf REQUIRED)
include_directories(${Protobuf_INCLUDE_DIRS})
protobuf_generate_cpp(PROTO_SRCS PROTO_HDRS myprotofile.proto)
- PROTO_SRCS: This variable will hold the C++ source files generated from your `.proto` file.
- PROTO_HDRS: This variable will contain the corresponding header files.
Example Implementation
Here's a complete example of a simple `CMakeLists.txt` file:
cmake_minimum_required(VERSION 3.0)
project(MyProject)
find_package(Protobuf REQUIRED)
include_directories(${Protobuf_INCLUDE_DIRS})
protobuf_generate_cpp(PROTO_SRCS PROTO_HDRS myprotofile.proto)
add_executable(my_executable ${PROTO_SRCS} ${PROTO_HDRS} main.cpp)
target_link_libraries(my_executable ${Protobuf_LIBRARIES})
Compiling Your Code
Once you have your `CMakeLists.txt` set up, create a build directory and compile your project:
mkdir build
cd build
cmake ..
make
During this step, CMake will generate C++ source files from `myprotofile.proto`, compile the code, and link against the Protocol Buffers library. If everything is set up correctly, you should end up with an executable called `my_executable`.
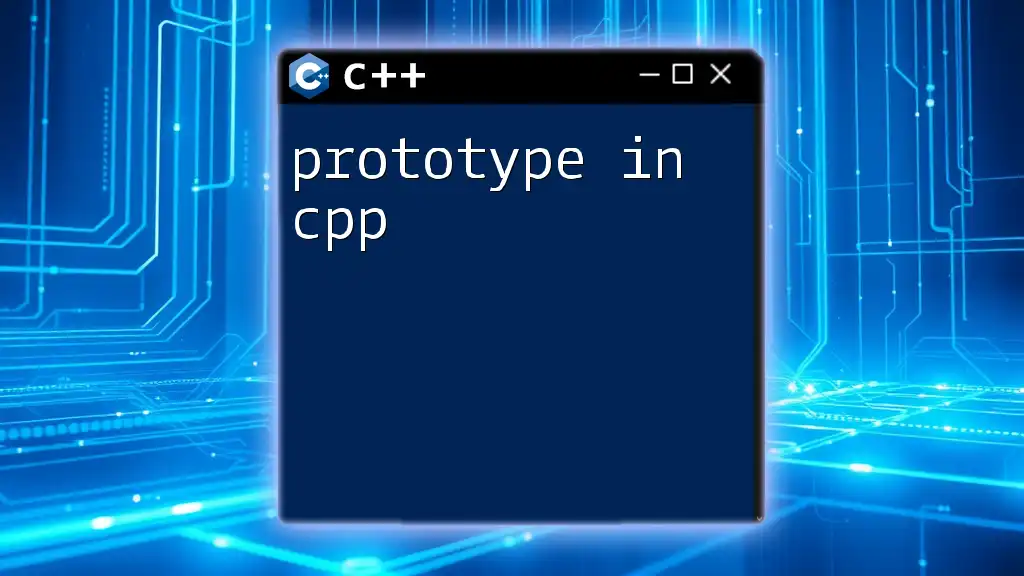
Designing Your `.proto` Files
Syntax of `.proto` Files
A `.proto` file describes the data structure used in your application. The syntax includes defining message types, enumerations, and other data structures following specific rules. Important elements include:
- Message: Defines user-defined types.
- Field: Represents the properties of the message with types and tags.
Best Practices in Designing Protobuf Schemas
When designing `.proto` files, adhering to best practices can save you significant headaches later:
- Naming Conventions: Use clear, consistent naming to clearly indicate the purpose of each message and field, which aids understanding and collaboration.
- Field Types: Choose appropriate types based on the expected data. For example, use `string` for text, `int32` or `float` for numbers, and `bool` for boolean values.
Example of a `.proto` File
Here is a simple example of a `.proto` file:
syntax = "proto3";
message Person {
string name = 1;
int32 id = 2;
string email = 3;
}
In this example, the `Person` message has three fields: `name`, `id`, and `email`. Each field is assigned a unique tag, which is essential for serialization.
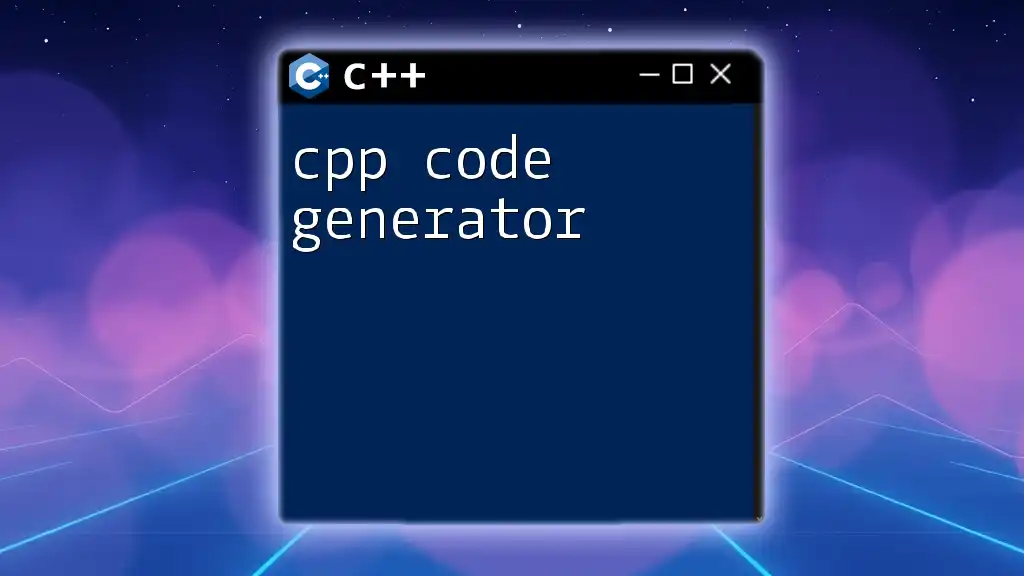
Generating C++ Code from `.proto` Files
Running CMake to Generate Code
Every time you run CMake, it will utilize the `protobuf_generate_cpp` command to automatically generate the C++ source files for your `.proto` definitions. Once generated, you can find them alongside your CMake project files.
Understanding Generated Code
Breakdown of Key Components
The generated `.pb.h` and `.pb.cc` files contain classes corresponding to the messages defined in your `.proto` files. These classes include methods for:
- Serialization: Converting messages to a binary format.
- Deserialization: Reconstructing a message from its binary representation.
Example of Using Generated Code
To demonstrate, here's how you might use the generated C++ code in conjunction with your message:
#include "myprotofile.pb.h"
void createPerson() {
Person person;
person.set_name("John Doe");
person.set_id(12345);
person.set_email("john.doe@example.com");
// Serialize
std::string output;
person.SerializeToString(&output);
// Deserialize
Person new_person;
new_person.ParseFromString(output);
}
In this example, we create a `Person` object, set its fields, serialize it to a string, and then parse a new `Person` object from that string.
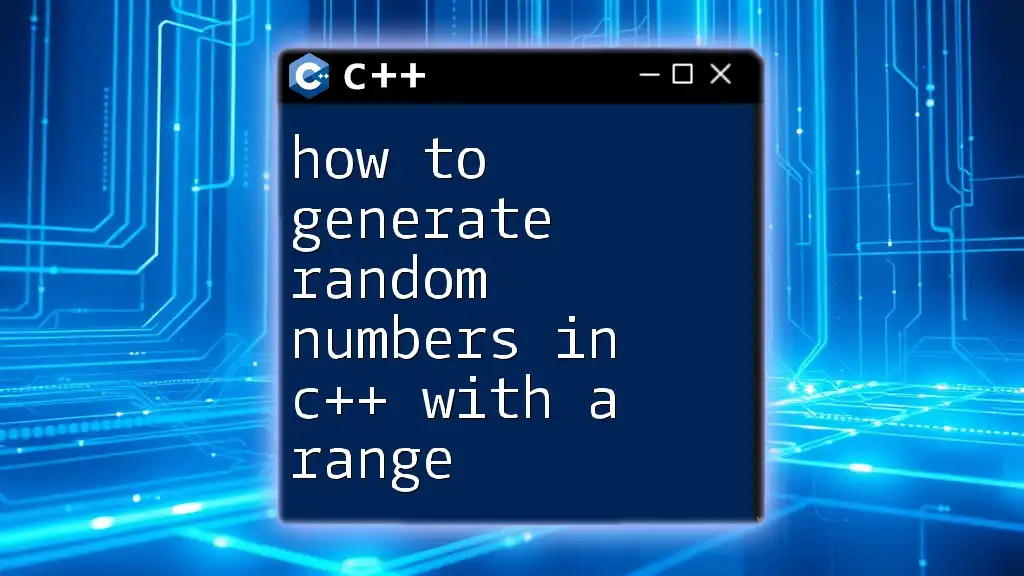
Common Issues with `protobuf_generate_cpp`
Compilation Errors
Users often encounter errors related to missing dependencies or improperly configured CMake files. Common issues include:
- Cannot find Protobuf: Ensure that Protobuf is installed and correctly pointed to in your CMake configuration.
- Invalid `.proto` syntax: Check for syntax issues in your `.proto` files.
Serialization/Deserialization Pitfalls
Serialization and deserialization can present challenges. Common pitfalls involve:
- Mismatched field types: Ensure that the types used in your code match those defined in the `.proto` file.
- Field omissions: Messages must contain all required fields during deserialization; otherwise, you may encounter runtime errors.
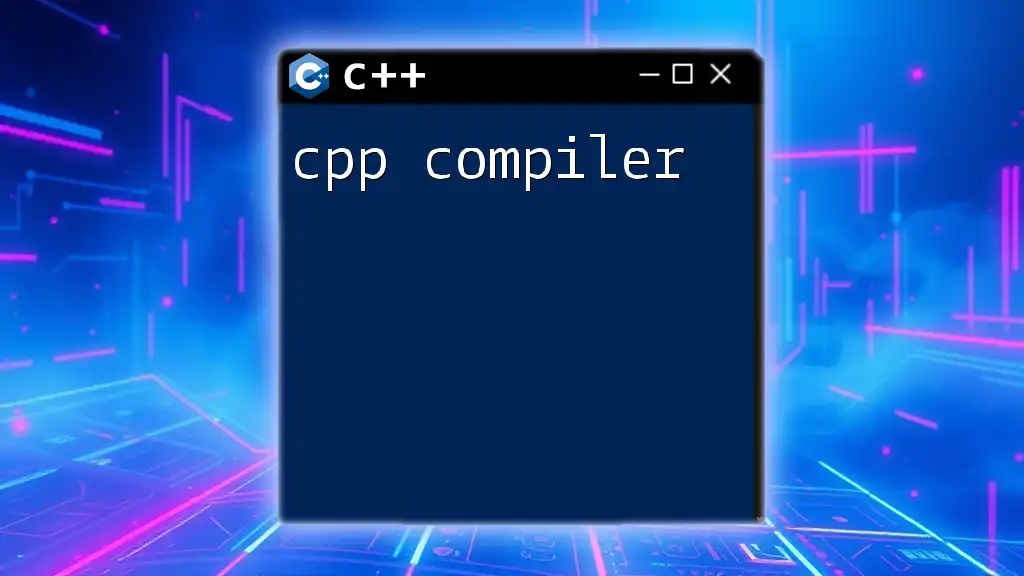
Advanced Usage of `protobuf_generate_cpp`
Custom Options in `.proto` Files
You can extend the capabilities of Protocol Buffers by defining custom options within your `.proto` files. This may be particularly useful for serialization options or integrating with other protocols.
Integrating with Other Languages
Protocol Buffers support multiple programming languages, ensuring that your data structures can be shared across different services. For instance, you can easily use the same `.proto` definition to generate code for Python or Java, facilitating seamless multi-language implementations.
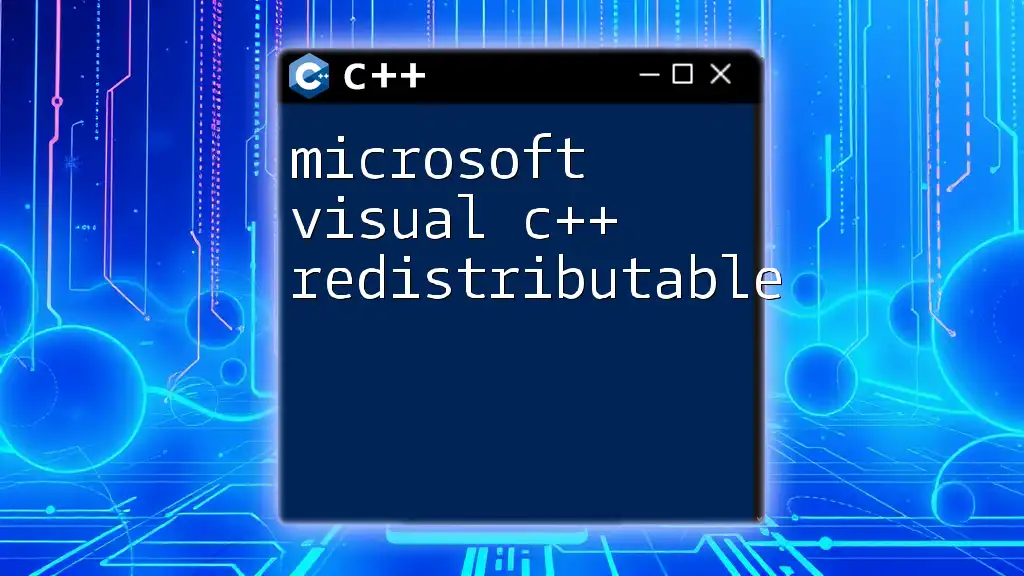
Conclusion
Recap of Key Takeaways
`protobuf_generate_cpp` offers a powerful way to automate the management of Protocol Buffers in C++. By eliminating boilerplate code and potential serialization errors, it allows developers to focus on building features rather than managing data formats.
Next Steps
Explore more advanced features and capabilities of Protocol Buffers. Familiarize yourself with the official Protocol Buffers documentation and further tutorials to enhance your understanding and skills.
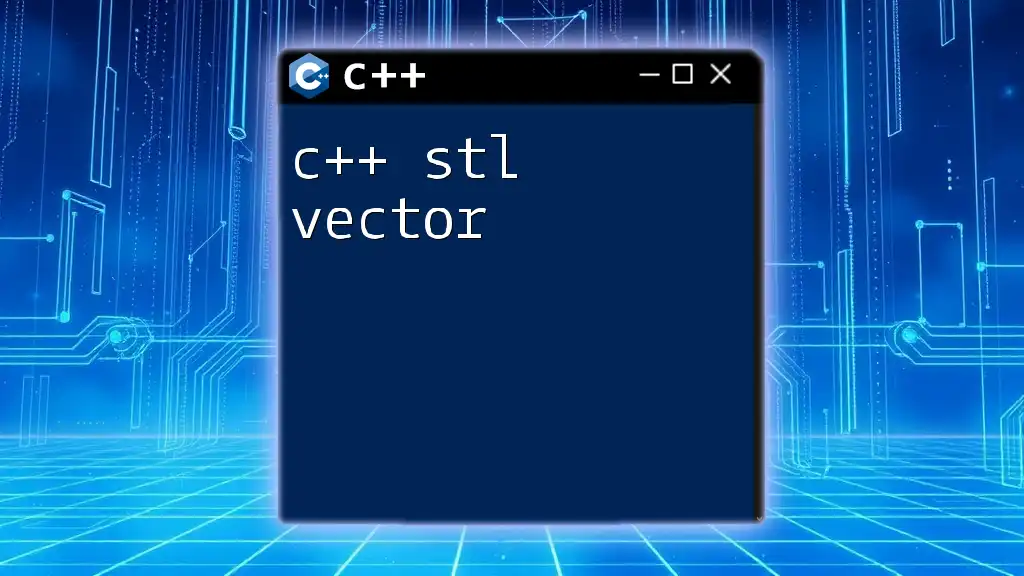
Frequently Asked Questions
What is the advantage of using Protocol Buffers over JSON or XML?
Protocol Buffers offer efficient and compact binary serialization, which results in faster network transmission and reduced storage requirements compared to text-based formats like JSON or XML.
How does `protobuf_generate_cpp` improve my development workflow?
It reduces the need for manual parsing and serialization code generation, resulting in fewer bugs and faster implementation times, while maintaining high performance and compatibility.
Can I use Protocol Buffers in real-time applications?
Yes, Protocol Buffers are well-suited for real-time applications where efficiency and speed are critical, making them a popular choice in high-performance systems.