In C++, a vector is a dynamic array that can resize itself, allowing you to store and manage collections of data more efficiently than traditional arrays.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.push_back(6); // Add an element to the end
for(int i : vec) {
std::cout << i << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
Introduction to `std::vector`
What is `std::vector`?
`std::vector` is a part of the C++ Standard Library, implemented as a dynamically-sized array capable of expanding and contracting as elements are added or removed. This container is crucial for managing collections of data where the size is not known at compile time, providing both flexibility and efficiency.
Importance of `std::vector` in C++
When compared to traditional arrays, `std::vector` offers enhanced functionality, such as automatic memory management and the ability to store any type of data. Whether for simple applications or complex data processing, `std::vector` stands as a fundamental tool in the C++ programmer's toolkit.
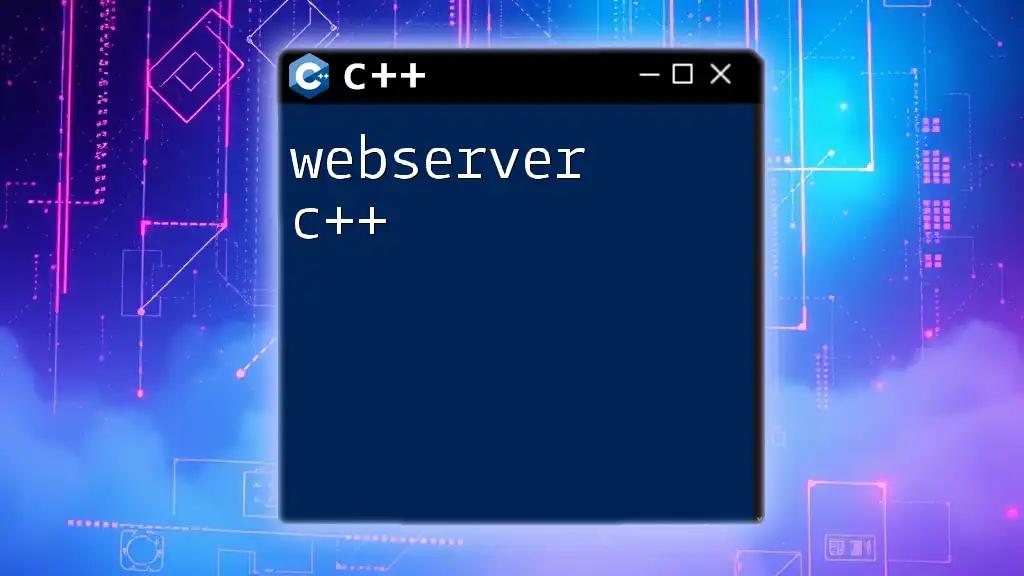
Understanding `std::vector` Basics
Syntax and Declaration
To declare a vector, you’ll follow the syntax: `std::vector<Type> name;`. This simple declaration creates an empty vector capable of holding elements of the specified data type.
Example:
std::vector<int> numbers; // Declares an empty vector of integers
Initializing a Vector
You can initialize `std::vector` in multiple ways:
- Default initialization: This creates an empty vector.
- Initializing with size: This allocates a vector of a specific size filled with default values.
- Initializing with values: You can also initialize a vector with specific values.
Example:
std::vector<int> myVector(10); // Creates a vector of size 10
std::vector<int> myVectorWithValues = {1, 2, 3, 4, 5}; // Vector initialized with specific values
Accessing and Modifying Elements
Accessing Elements
You can access elements in a vector using either the index operator `[]` or the `.at()` method. The index operator provides quick access but does not check for bounds, while `.at()` offers safety by checking if your access is within valid limits.
Example:
myVector[0] = 10; // Using index to modify the first element
int value = myVector.at(0); // Safeguard with .at()
Modifying Elements
Changing values can be effortlessly executed using a loop or directly referencing the index. For instance, applying an increment to every vector element is simplified with range-based loops.
Code Example:
for (auto& element : myVector) {
element += 5; // Increment each element by 5
}
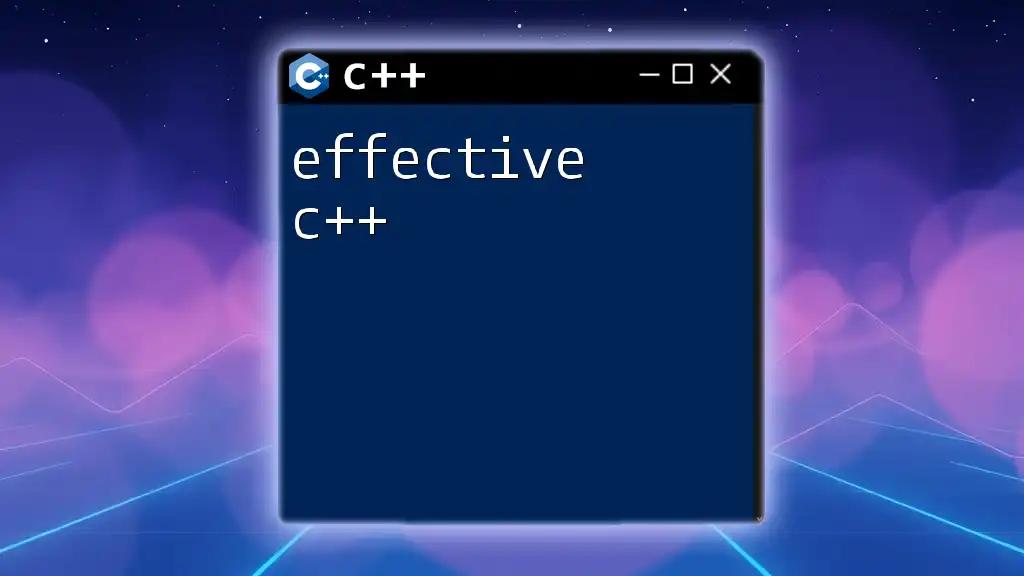
Core Functionalities of `std::vector`
Adding and Removing Elements
Adding Elements
The `.push_back()` method allows you to append items to the end of the vector. This expands the vector size dynamically as necessary.
Example:
myVector.push_back(6); // Adds 6 to the end of the vector
Removing Elements
You can remove items using `.pop_back()` to erase the last element or the `erase()` function for specific positions.
Code Example:
myVector.pop_back(); // Removes the last element
myVector.erase(myVector.begin() + 1); // Removes the element at the second position
Reserved Memory Management
Why and How to Use `reserve()`
Before adding a significant number of elements, you can use `reserve()` to pre-allocate memory. This can prevent frequent overhead from resizing during multiple insertions.
Example:
myVector.reserve(20); // Pre-allocates space for 20 elements
Iterating over a Vector
There are several approaches to iterate through a vector. You can use:
- A traditional for loop for indexed access.
- A range-based for loop for simplicity.
- Iterators for greater control.
Code Example:
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i] << " "; // Print each element using index access
}
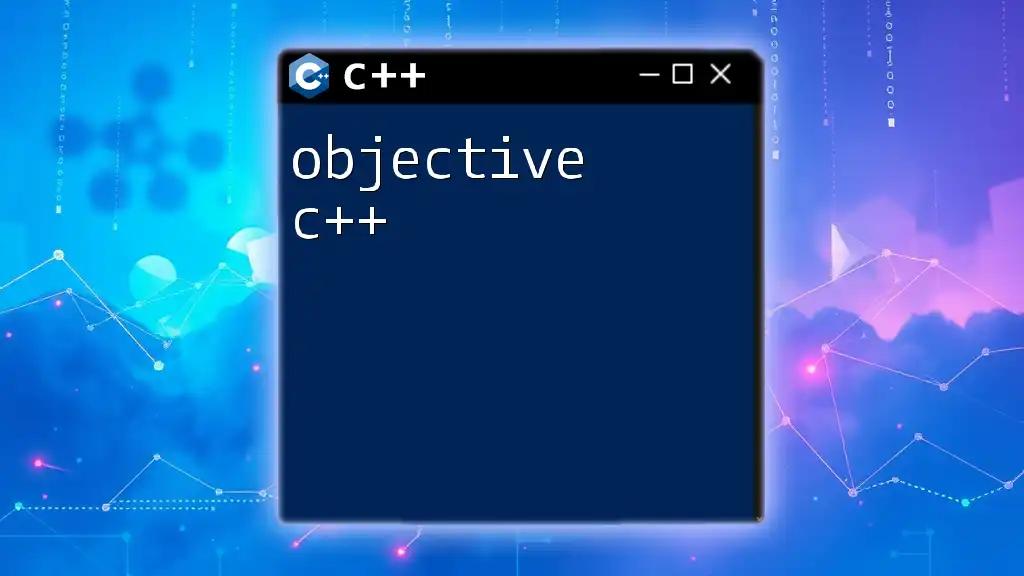
Advanced Features of `std::vector`
Sorting and Manipulating Vectors
Sorting a Vector
With the `std::sort()` function, you can sort the contents of a vector quickly and efficiently. This function utilizes the QuickSort algorithm internally.
Example:
std::sort(myVector.begin(), myVector.end()); // Sorts the vector in ascending order
Reversing a Vector
You can also reverse the order of elements within a vector using the `std::reverse()` function.
Code Example:
std::reverse(myVector.begin(), myVector.end()); // Reverses the vector
Working with Multi-Dimensional Vectors
Defining multi-dimensional vectors (e.g., 2D arrays) is straightforward:
Defining a 2D Vector
Syntax and initialization can be done as follows:
Example:
std::vector<std::vector<int>> matrix = {{1, 2, 3}, {4, 5, 6}}; // A 2D vector
Accessing Elements in 2D Vectors
Accessing elements requires specifying both dimensions.
Example:
int value = matrix[1][2]; // Accesses the element '6'
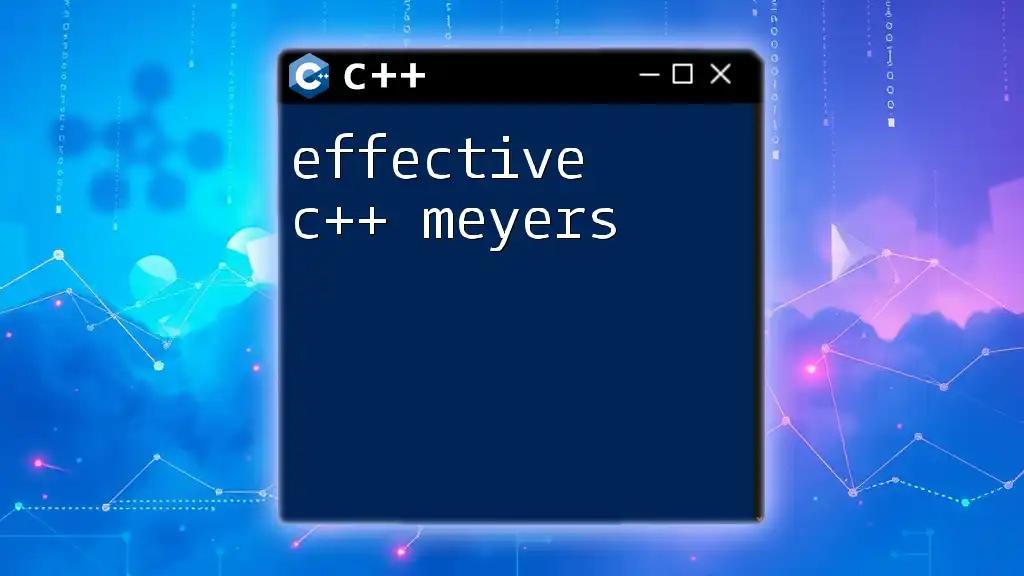
Performance Considerations
When to Use `std::vector` vs Other Containers
Advantages of `std::vector`
- Storage efficiency: Automatically manages memory without fragmentation.
- Fast access times: Provides O(1) time complexity for index-based access.
Disadvantages and Limitations
However, while `std::vector` is potent, remember that excessive resizing can lead to performance hits, particularly when the vector grows pretty large often.
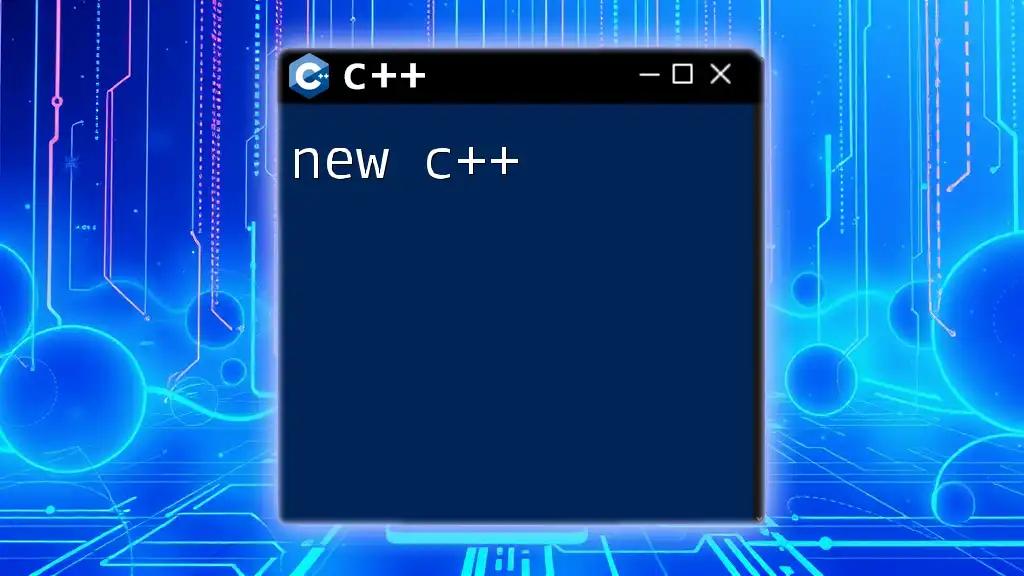
Conclusion
In summary, `std::vector` is an essential tool for any C++ programmer, providing powerful functionalities for handling dynamic sized arrays. By mastering `std::vector`, you'll be equipped to tackle a wide array of programming challenges efficiently. Practice makes perfect; dive into hands-on coding and enjoy the learning journey!
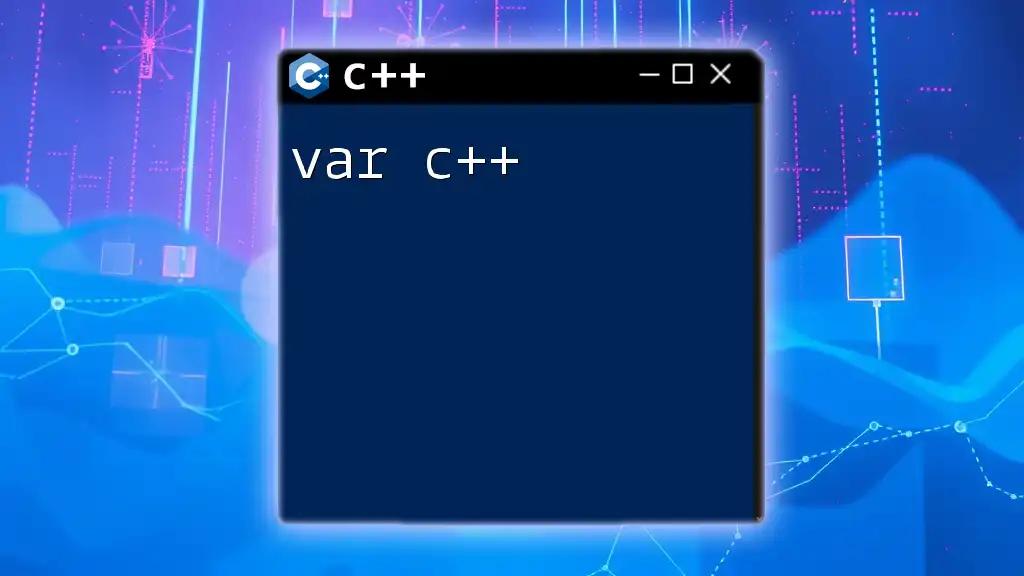
References
- For more in-depth reading, you can explore the [C++ Standard Library Documentation](https://en.cppreference.com/w/cpp/container/vector).
- Check out [Effective STL](https://www.oreilly.com/library/view/effective-stl-50/9780132772374/) for advanced techniques and insider tips on using this versatile container effectively.