"New C++" refers to the modern features and enhancements introduced in C++11 and later versions, enabling developers to write more efficient and expressive code.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Dynamic Memory Allocation
Dynamic memory allocation allows you to allocate memory at runtime, which can be essential in situations where the size of data is not known beforehand. This flexibility provides significant advantages over static memory allocation, where the size must be specified at compile time.
Why Use Dynamic Memory? Dynamic memory is particularly useful for handling variable-sized data structures like arrays, linked lists, or trees. By utilizing dynamic allocation, you can efficiently manage data without wasting memory.
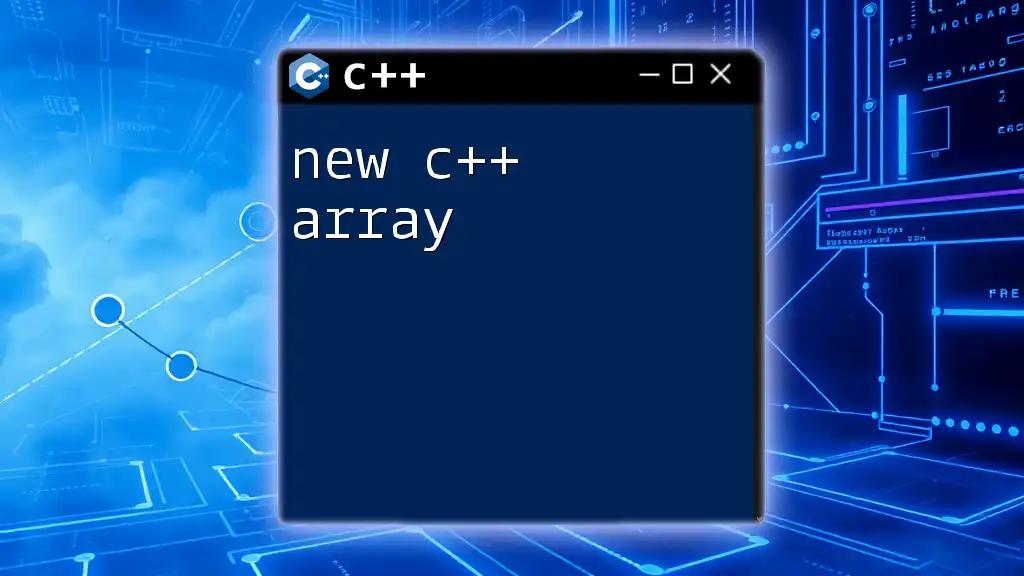
The `new` Operator in C++
The `new` operator in C++ is a powerful tool that facilitates dynamic memory allocation. It not only allocates memory for a variable but also initializes it, making it a convenient choice for developers.
Allocating Single Objects
To allocate a single object dynamically, you can use the following syntax:
Type* pointer = new Type;
For example, to allocate a single integer, you can write:
int* pInt = new int; // Dynamically allocate an integer
This statement allocates memory sufficient to hold an integer and returns a pointer to it, which you can use to manipulate the data.
Understanding Pointers and References
In C++, pointers are variables that hold memory addresses of other variables. When you use the `new` operator, it returns the address of the allocated memory, which is assigned to the pointer. Understanding how pointers work is critical as they allow direct manipulation of memory.
Allocating Arrays
You can also allocate entire arrays dynamically using `new`. The syntax is similar but specifies the size of the array:
Type* pointer = new Type[size];
To allocate an array of integers, for instance, you can do:
int* pArray = new int[10]; // Dynamically allocate an array of 10 integers
Once allocated, you can access and modify the array elements using standard array indexing:
for(int i = 0; i < 10; i++) {
pArray[i] = i * 2; // Initializes array elements
}
This for-loop iterates through the newly allocated array and initializes each element with double the index value.
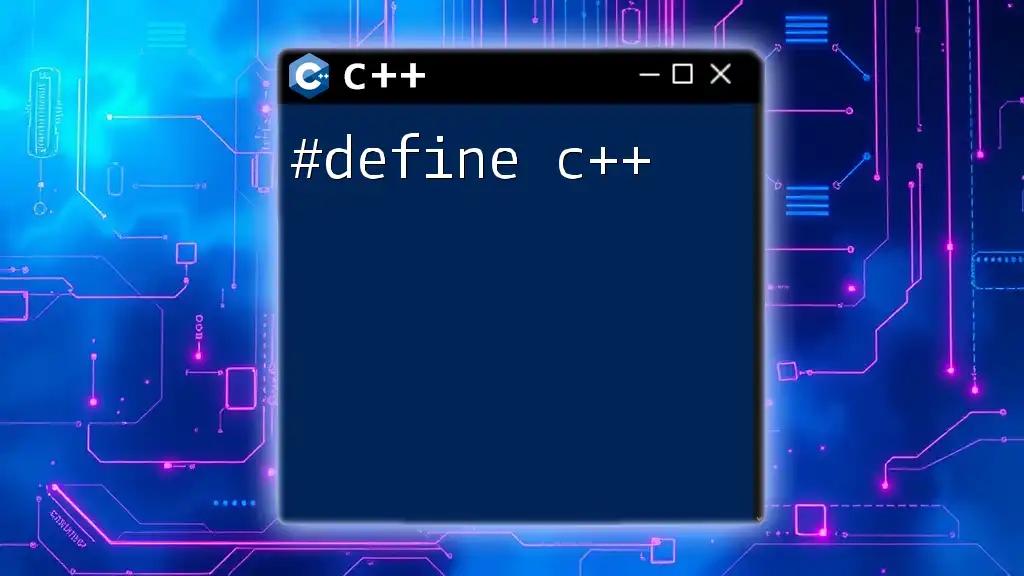
Understanding the `delete` Operator
Memory management in C++ involves not only allocating memory using `new` but also deallocating it using the `delete` operator to avoid memory leaks.
Syntax for Deleting Single Objects
For each object allocated with `new`, you must match it with `delete` to free the memory:
delete pointer; // Deallocates the single object
For the integer allocated earlier, you would use:
delete pInt; // Deallocates the single integer
Syntax for Deleting Arrays
Similarly, when you allocate an array using `new`, you must use `delete[]` to free that memory. For the previously allocated integer array, you would do:
delete[] pArray; // Deallocates the array of integers
Failing to do so can result in memory leaks, where memory remains allocated even when no longer in use.
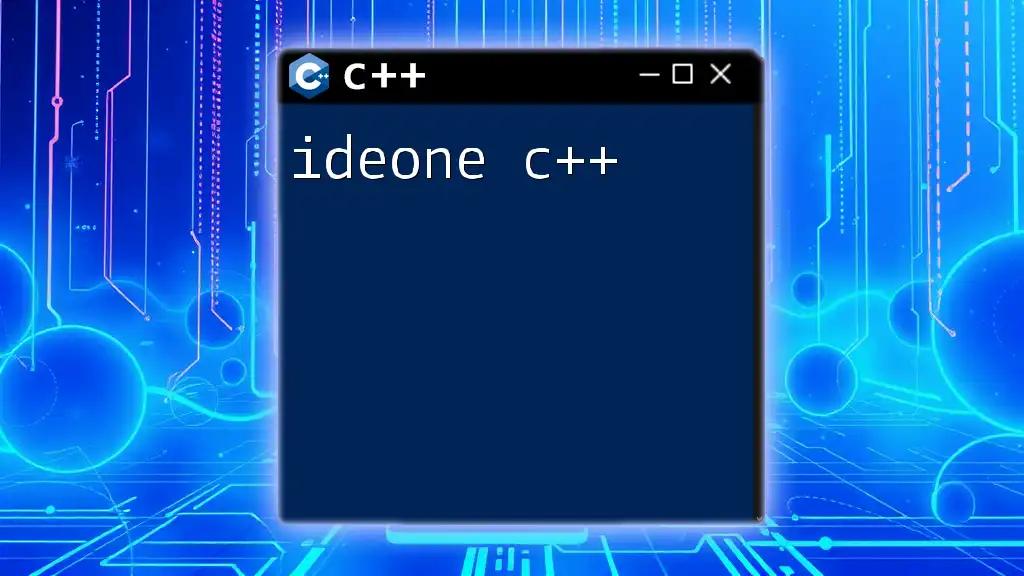
Common Mistakes with `new` and Memory Management
One of the most common mistakes in C++ is related to dynamic memory management, particularly regarding memory leaks and dangling pointers.
Memory Leaks A memory leak happens when allocated memory is not freed. This can occur if you forget to `delete` an allocated object.
Example:
int* leakPointer = new int; // Forgetting to delete can cause memory leak
// Memory is still allocated even when leakPointer goes out of scope
Dangling Pointers A dangling pointer arises when an object is deleted, but the pointer still holds the address of that memory. Accessing this pointer can lead to undefined behavior.
Example of Dangling Pointer:
int* dangPtr = new int;
delete dangPtr; // Dangling pointer now points to freed memory
To avoid these issues, always remember to balance `new` with `delete` and set pointers to `nullptr` after deletion.
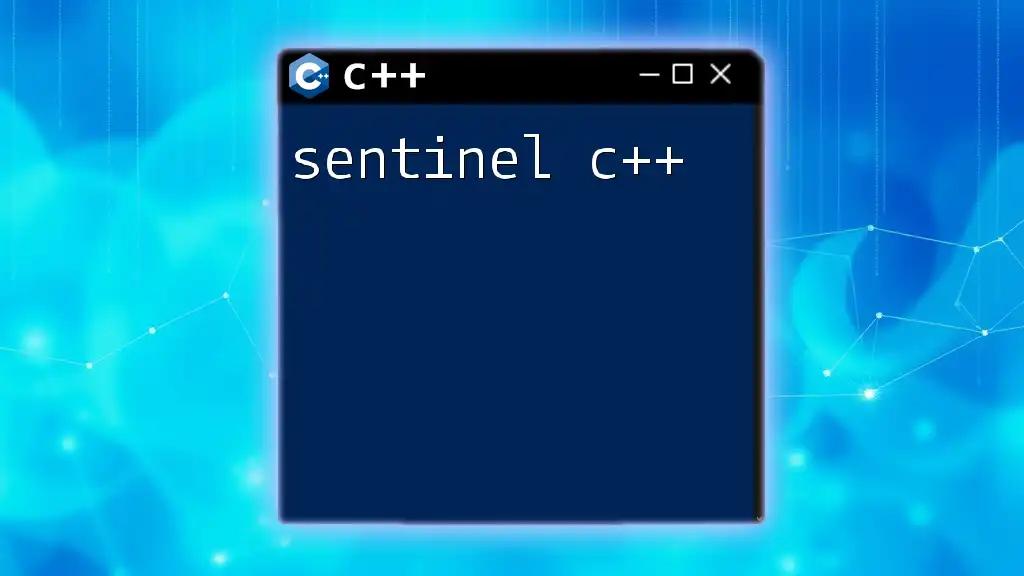
Advanced Usage of `new`
Placement New
Placement new is a specialized form of the `new` operator that allows you to construct an object in a specific memory location. This can be useful in performance-sensitive applications where you want to control memory locations.
Example usage of placement new:
#include <new>
char buffer[sizeof(int)];
int* pInt = new (buffer) int; // Placement new
In this example, `pInt` points to an integer that is constructed in the pre-allocated buffer. Note: When using placement new, you must manually manage the object's lifetime and invoke the destructor when done.
New with Custom Allocators
C++ allows for the use of custom memory allocators, which enable you to define your allocation and deallocation logic. This can lead to improved performance in specific use cases and provide fine-tuned memory management.
Example of integrating a custom allocator:
class CustomAllocator {
public:
void* allocate(size_t size) {
return ::operator new(size); // Use global new for allocation
}
void deallocate(void* pointer) {
::operator delete(pointer); // Use global delete for deallocation
}
};
By creating your allocator, you can optimize memory usage based on the specific needs of your application.
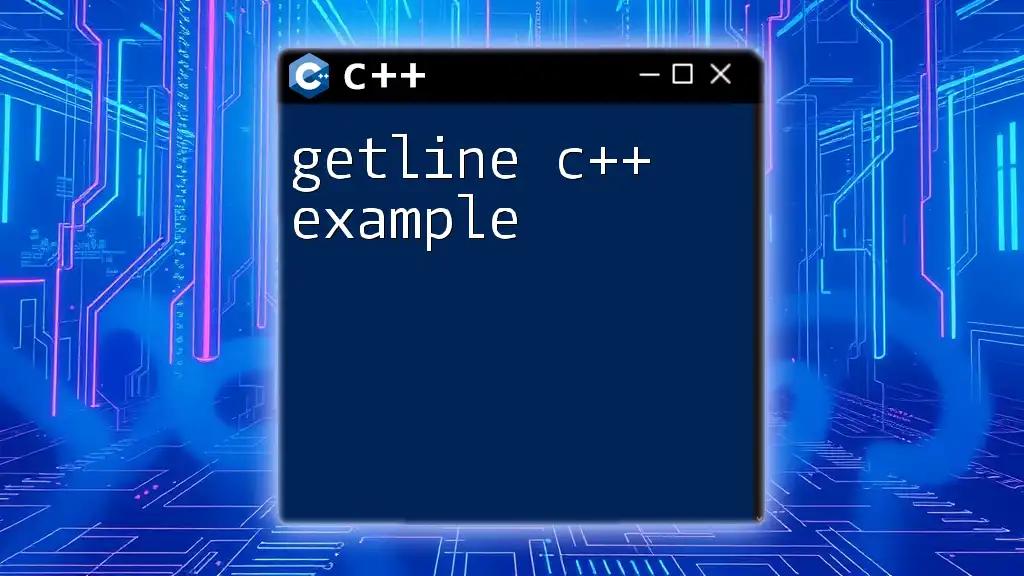
Conclusion
Understanding how to correctly use the `new` operator in C++ is vital for effective memory management and ensuring efficient, performant applications. The interplay between dynamic allocation, pointers, and proper memory deallocation is fundamental to mastering C++. As with any skill, practice is key—invest time into working with dynamic memory to enhance your proficiency in C++ programming.
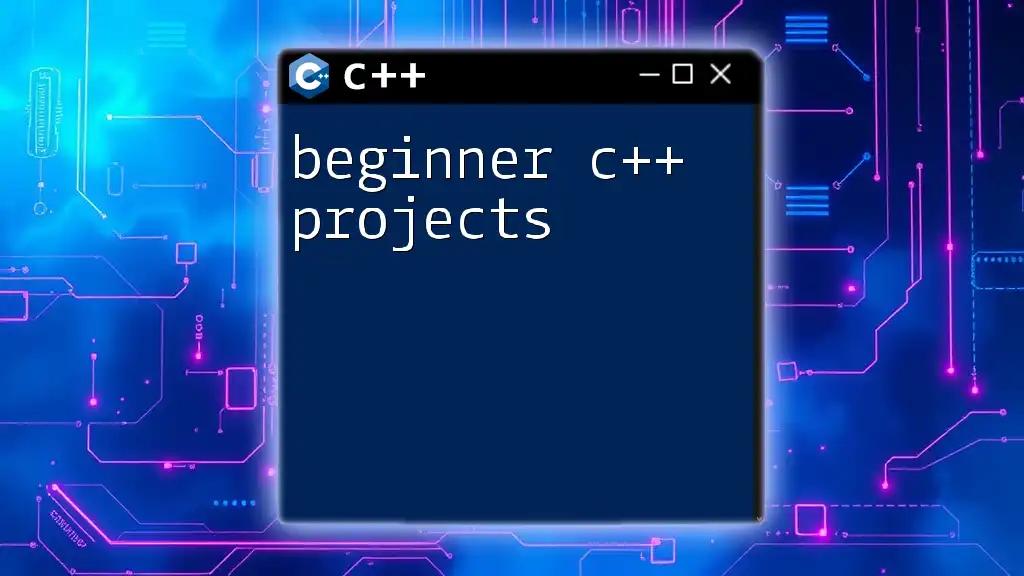
Additional Resources
To further your learning, consider exploring recommended books, online courses, and joining C++ communities. Engaging with professionals can provide insights and answers to challenging questions as you dive deeper into the world of C++.