In C++, a new array can be created using the `new` keyword followed by the type and size of the array, allowing for dynamic memory allocation.
int* myArray = new int[10]; // Creates a dynamic array of 10 integers
Understanding C++ Arrays
What is an Array?
An array is a collection of elements, all of the same type, arranged in a contiguous block of memory. It allows for efficient storage and retrieval of data, making it essential in various programming tasks. Arrays are particularly useful when you need to manage a fixed-size collection of items, such as storing the scores of students or the prices of products.
Types of Arrays in C++
In C++, arrays can be classified into two main types:
- Static Arrays: These have a fixed size determined at compile time. Once defined, their size cannot be altered, which can lead to inefficiencies if the exact size of the array is unknown during compile time.
- Dynamic Arrays: Defined at runtime using the `new` operator, dynamic arrays can change in size, allowing more flexibility in memory management. They are allocated on the heap memory, which distinguishes them from static arrays.
Understanding the difference between static and dynamic arrays is crucial, especially when considering factors like memory allocation and performance.
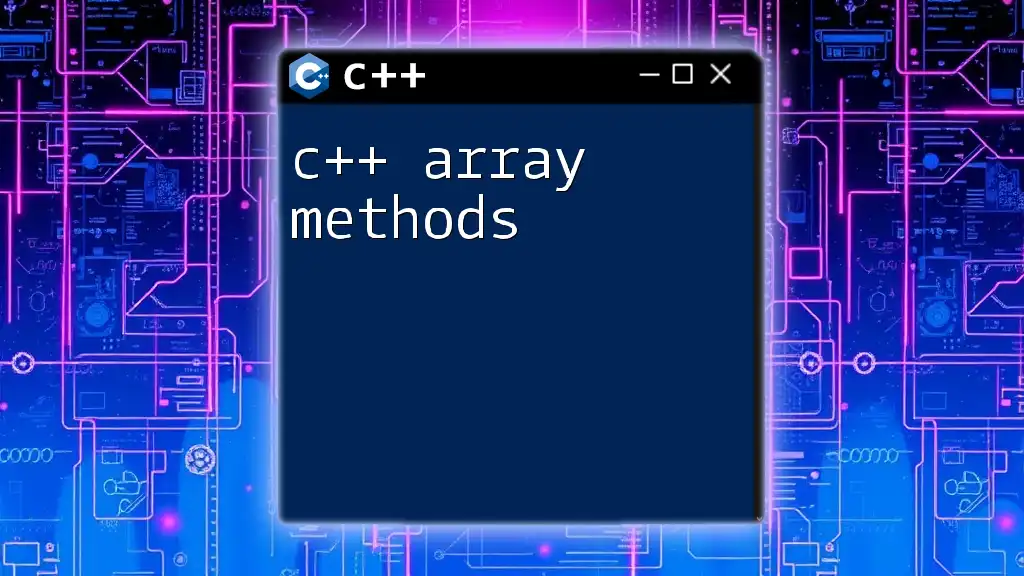
Understanding Dynamic Arrays
Why Use Dynamic Arrays?
Dynamic arrays provide several advantages over static arrays. First and foremost, they offer flexibility. By allowing the size to be specified at runtime, you can adapt to varying amounts of data without wasting memory on unused elements or risking overflow.
Syntax for Creating a Dynamic Array
To declare a dynamic array in C++, you use the following syntax:
data_type* array_name = new data_type[size];
For example, if you want to create a dynamic array of integers:
int* arr = new int[5]; // Creates an array of 5 integers
This single line of code allocates memory for five integers on the heap, providing a dynamic array that can be manipulated during the program's execution.
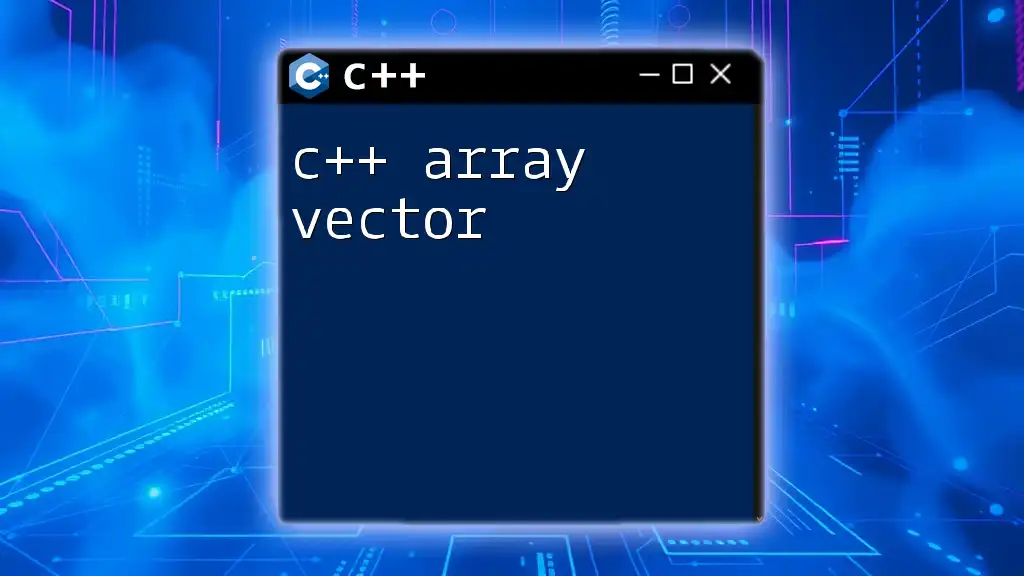
Allocating Memory for Dynamic Arrays
Memory Allocation Process
When you allocate memory for a dynamic array using `new`, it reserves space on the heap, which is separate from the stack space where static arrays reside. This allows for more extensive and flexible memory usage, especially in applications where the data size is unpredictable.
Example of Memory Allocation
Here is an example of how to allocate and initialize a dynamic array in C++:
int size = 5;
int* arr = new int[size];
for (int i = 0; i < size; i++) {
arr[i] = i * 10; // Initializing the array
}
In this code snippet, we first declare the size of the array. We then create an array of five integers and initialize each index with a value that’s ten times its index. This results in an array containing: `0, 10, 20, 30, 40`.
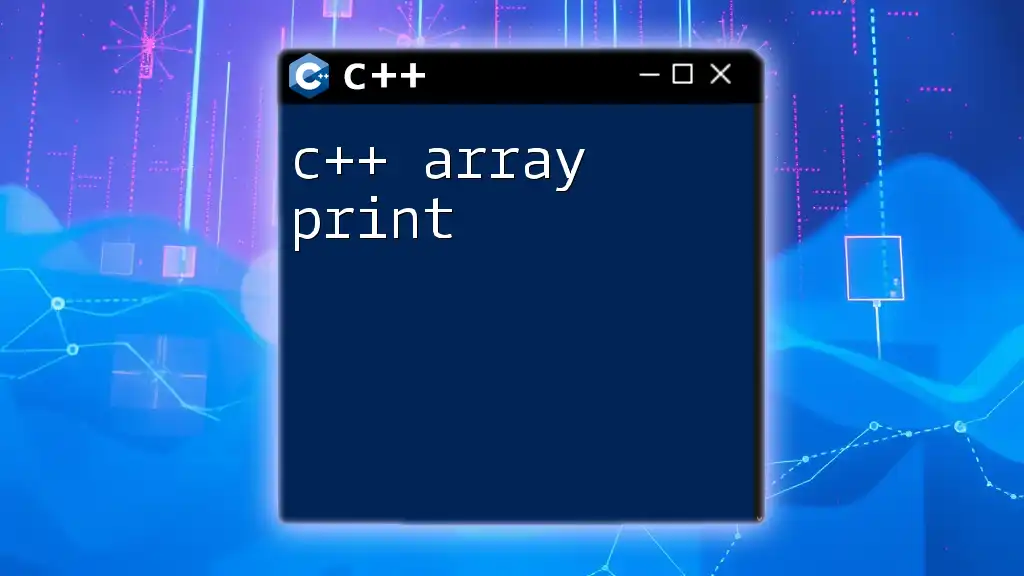
Accessing Elements in New C++ Arrays
Indexing in Arrays
Accessing array elements is straightforward in C++. The array indexing starts at 0, meaning the first element is accessed with `arr[0]`, the second with `arr[1]`, and so on until `arr[size-1]` for the last element.
Looping Through a Dynamic Array
You can utilize a `for` loop to traverse through the dynamic array efficiently. Consider the following example:
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " "; // Output the current element
}
This code snippet prints each element of the array, showcasing how easily you can manipulate and display data stored in dynamic arrays.
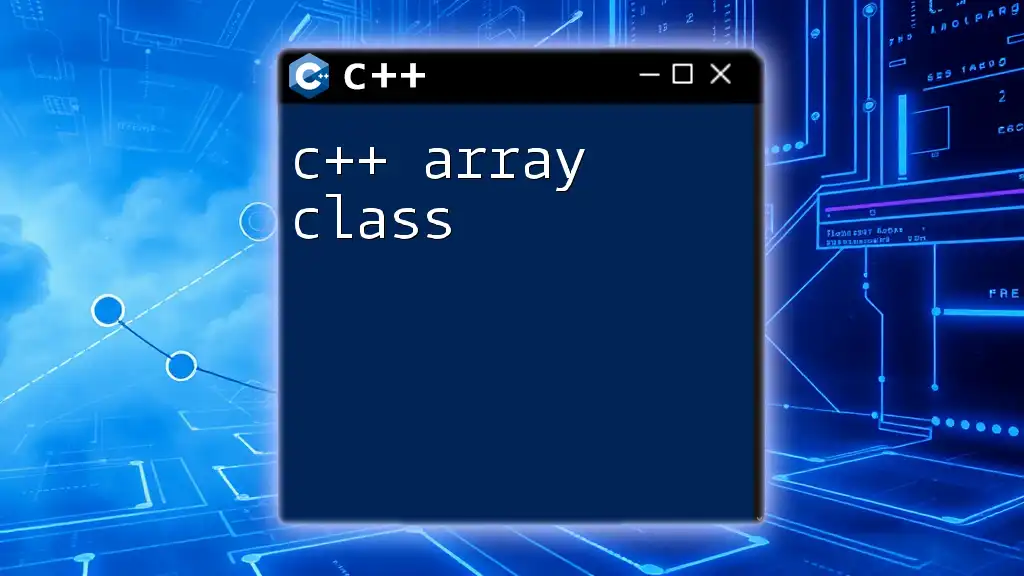
Deallocating Memory for Dynamic Arrays
Importance of Memory Deallocation
While dynamic arrays offer great flexibility, they also require careful memory management to prevent memory leaks—a situation where allocated memory is not freed. This can lead to increased memory usage and ultimately exhaust system resources.
Syntax for Deallocating Memory
When you are done using a dynamic array, it’s crucial to deallocate the memory using the `delete` operator:
delete[] arr; // Deallocating memory for dynamic array
This command frees the memory occupied by the array, reducing the risk of memory leaks in your application.
Example of Proper Memory Management
Here’s a complete example that includes allocating, accessing, and deallocating a dynamic array:
int* arr = new int[5];
// Initializing the array
for (int i = 0; i < 5; i++) {
arr[i] = i * 10;
}
// Using the array
for (int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
delete[] arr; // Always free the memory
In this example, memory management is prioritized by ensuring that `delete[]` is called after the array is used, illustrating good programming practice.
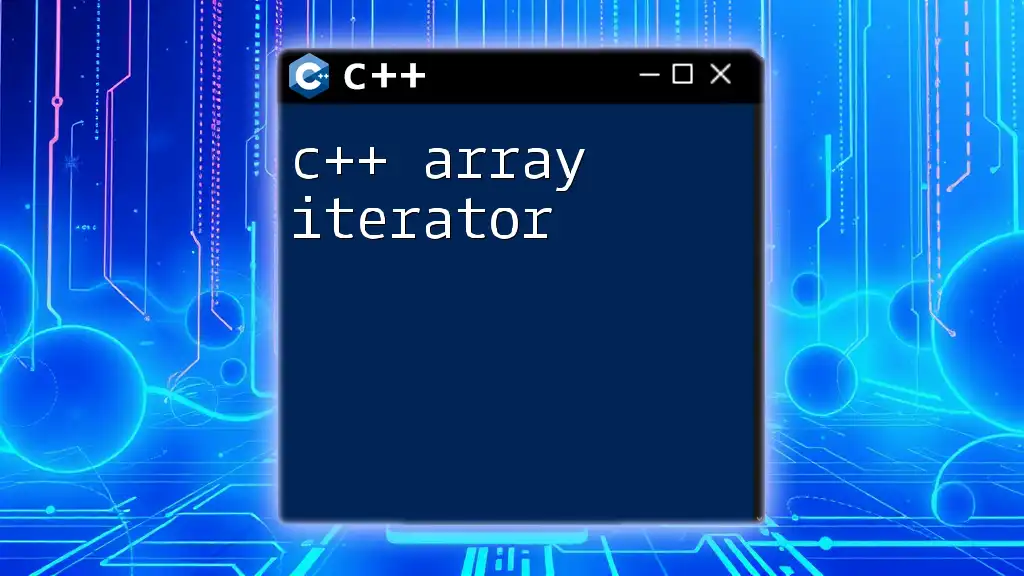
Common Pitfalls with Dynamic Arrays
Memory Leaks
A prevalent issue that arises when using dynamic arrays is memory leaks. This occurs if you forget to call `delete`, leaving allocated memory inaccessible for the remainder of the program. Such leaks can degrade performance over time, especially in programs with prolonged execution.
Out-of-Bounds Access
Accessing an index that exceeds the defined size of your dynamic array can lead to undefined behavior. For example:
std::cout << arr[5]; // Accessing out-of-bounds (Undefined behavior)
The above line tries to access memory beyond the allocated size, which can result in erratic output or program crashes.
Undefined Behavior
Undefined behavior is a fundamental concern in C++. It signifies that the program may produce unpredictable results due to various programming errors, including memory mismanagement, uninitialized variables, or incorrect pointer usage.
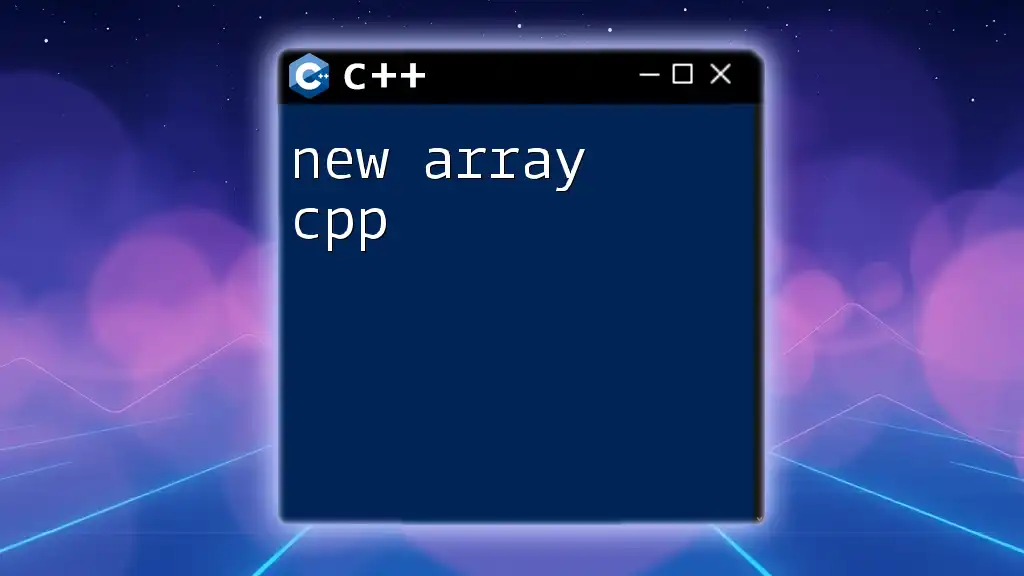
Best Practices for Using New C++ Arrays
Choosing the Right Array Size
When defining the size of a dynamic array, consider factors such as the anticipated maximum number of elements. This helps you avoid excess memory consumption and improve performance.
Using Smart Pointers as an Alternative
Modern C++ provides smart pointers, which automate memory management and significantly reduce the risk of memory leaks. The most common one is `std::unique_ptr`, which manages resources efficiently. Here’s how you can utilize smart pointers for dynamic arrays:
std::unique_ptr<int[]> arr(new int[size]); // Automatically deletes memory
This line of code creates a dynamic array managed by a smart pointer, ensuring that the memory is automatically freed when the pointer goes out of scope, eliminating the need for explicit deallocation.
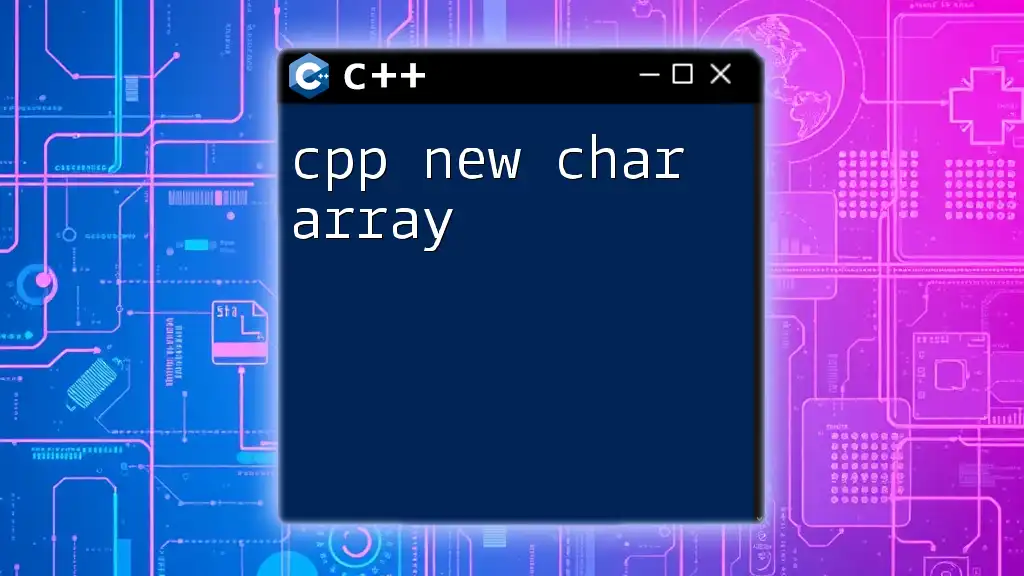
Conclusion
Understanding dynamic arrays—referred to as "new C++ array"—is pivotal for effective memory management in C++. By mastering their syntax, allocation, access patterns, and best practices, you can harness their full potential while safeguarding your programs from common pitfalls. Remember to experiment and practice using dynamic arrays to strengthen your programming skills and enhance your confidence in C++.
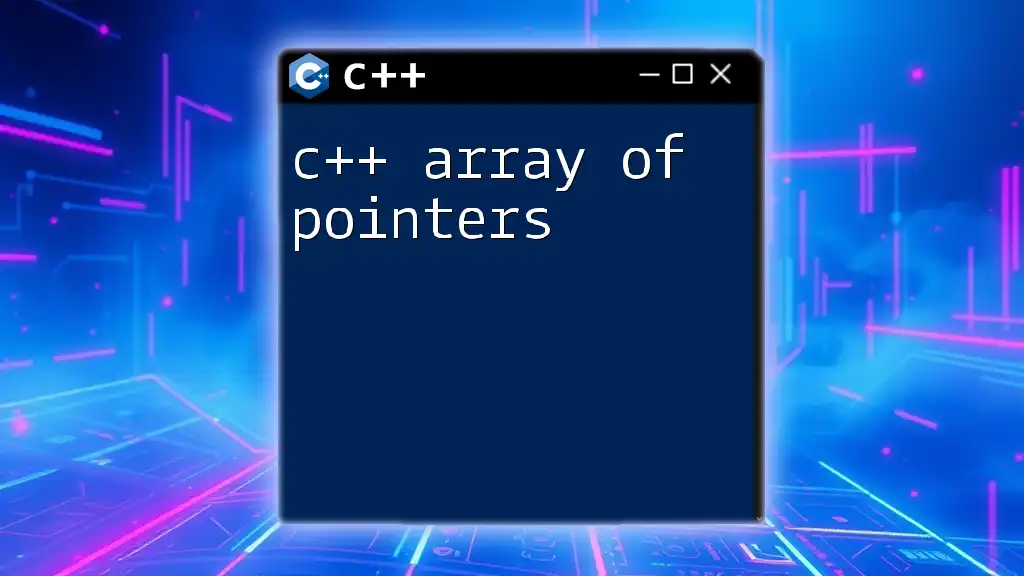
Additional Resources
For further reading, consider exploring official C++ documentation, online tutorials, and recommended programming books. Delving deeper into array manipulation and memory management techniques will empower you to write more efficient, robust, and maintainable C++ applications.