In C++, you can dynamically allocate a new character array using the `new` operator, allowing you to define its size at runtime. Here's a simple example:
char* myArray = new char[10]; // allocates an array of 10 characters
Understanding Char Arrays
What is a Char Array?
A char array is a sequence of characters stored in contiguous memory locations. In C++, strings can be represented using char arrays, which can be critical in many programming scenarios, especially when dealing with C-style strings. They are essential for representing textual data and often serve as buffers for handling input or output operations.
In programming, you might often encounter situations where you want to manipulate text data without relying on C++ string classes. This is where char arrays become highly relevant.
Declaring and Initializing Char Arrays
Char arrays can be declared both statically and dynamically.
- Static Allocation: This occurs at compile-time and involves defining a fixed size for your array. An example of a static char array declaration is:
char staticArray[10]; // Static allocation for 10 characters
While static arrays are straightforward, they often lack flexibility in terms of size. This leads to the need for dynamic allocation, especially when the required size isn't known until runtime.
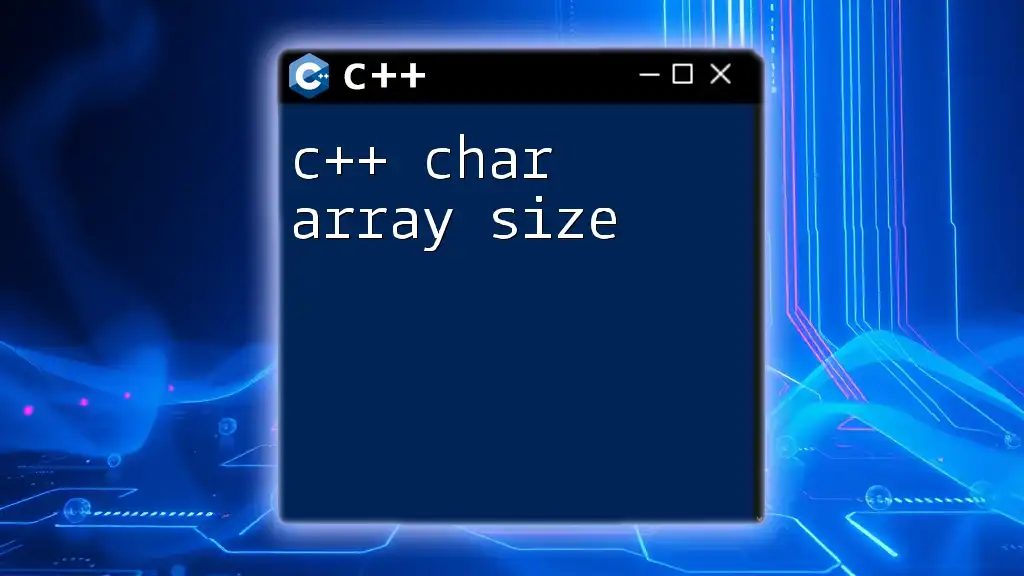
Dynamic Memory Allocation
The Need for Dynamic Memory
Dynamic memory allocation allows programmers to request memory during runtime, providing flexibility. If the size of the data is not known beforehand (for instance, user input), relying solely on static memory can lead to issues. Dynamic char arrays allow you to create an array whose size can be determined while the program is executing.
Using `new` to Allocate a Char Array
To create a dynamic char array, the `new` operator is utilized. This reserves memory specifically for the program’s needs and returns a pointer to the allocated memory. The syntax for using `new` for char arrays is as follows:
char* dynamicArray = new char[size];
In this line, `size` is a variable that signifies how many characters the array should hold. This method is potent, but it also places the responsibility of managing that memory on the programmer.
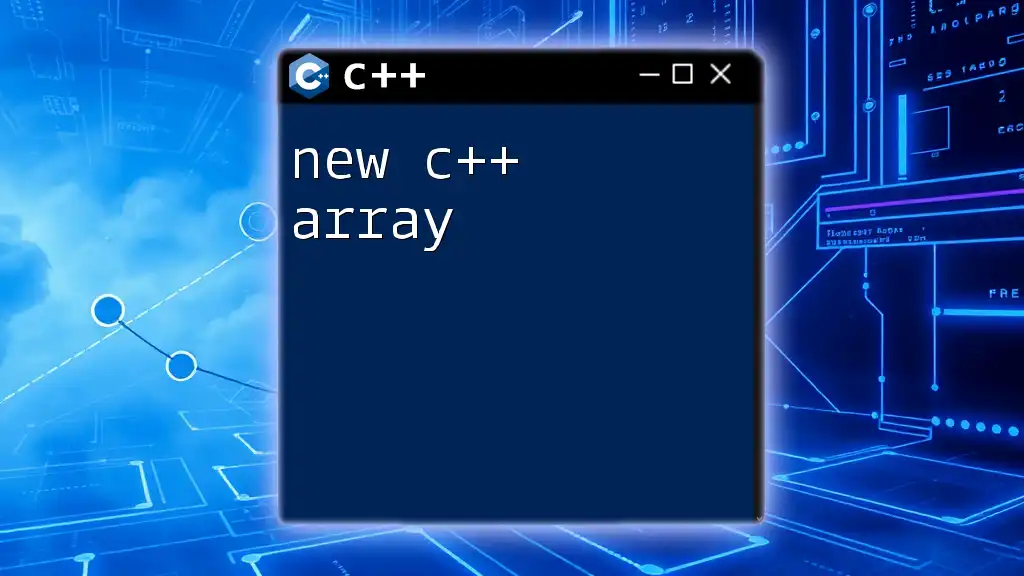
Example: Creating and Using a Dynamic Char Array
Step-by-Step Code Example
To illustrate how to work with a dynamic char array, let’s consider the following full code example:
#include <iostream>
int main() {
int size;
std::cout << "Enter the size of the char array: ";
std::cin >> size;
char* dynamicArray = new char[size];
std::cout << "Dynamic char array created with size " << size << "\n";
// Example of populating the char array
for (int i = 0; i < size; ++i) {
dynamicArray[i] = 'a' + i; // Filling array with letters
}
// Displaying the char array
std::cout << "Contents of char array: ";
for (int i = 0; i < size; ++i) {
std::cout << dynamicArray[i] << " ";
}
std::cout << std::endl;
// Clean up memory
delete[] dynamicArray; // Important to release memory
return 0;
}
Explanation of the Example
- Input Size: The program begins by prompting the user to enter the size of the char array required.
- Array Creation: Using `new`, we allocate a dynamic char array, whose size is based on user input.
- Populating the Array: The program fills the array with consecutive characters starting from 'a'. It makes use of a simple loop to assign values to each index of the dynamic array.
- Displaying Contents: After the array is filled, the content of the char array is displayed to the user.
- Memory Cleanup: Finally, using `delete[]`, the allocated memory is released to avoid memory leaks, which is an essential part of managing dynamic memory.
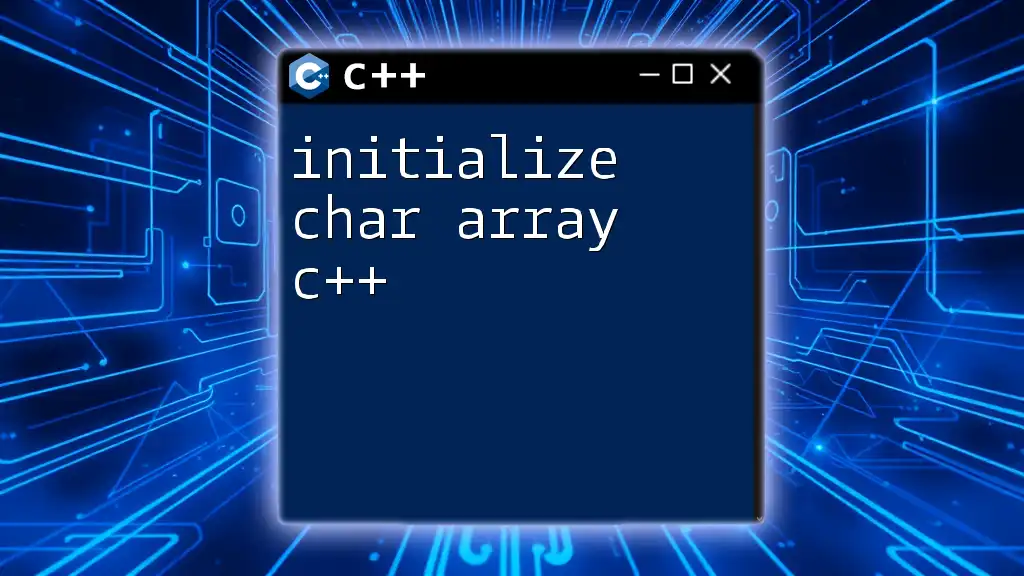
Best Practices for Using Dynamic Char Arrays
Memory Management
An important aspect of working with dynamic memory is ensuring that it is released properly. Always remember to use `delete[]` to free memory allocated by `new`. Failing to do this can lead to memory leaks, which gradually reduce the performance of your application and can lead to crashes when the operating system runs out of allocable memory.
Considering Alternatives
While dynamic char arrays are powerful, they can be error-prone. An excellent alternative is to use `std::string`, which handles memory management internally and provides a more intuitive interface for string manipulation. For many applications, `std::string` is preferred because it simplifies code, reduces the chances of memory management errors, and provides a rich set of functionalities for string handling.
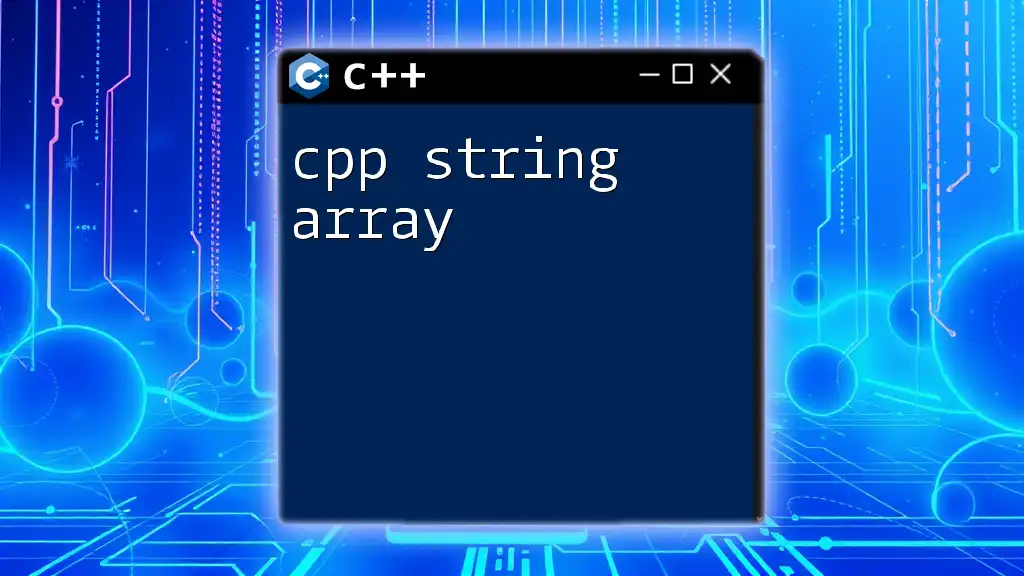
Common Mistakes
Common Pitfalls When Using `new`
- Forgetting to Free Memory: This is one of the most common mistakes when using dynamic allocation. Neglecting to call `delete[]` can lead to memory leaks.
- Buffer Overflows: Writing beyond the allocated size of the array can lead to undefined behavior. It's crucial to ensure that indices used in loops do not exceed the allocated size.
How to Avoid These Mistakes
To guard against these pitfalls, consider:
- Always initializing pointers to nullptr after memory allocation.
- Implementing checks before accessing array elements to ensure they are within bounds.
- Using smart pointers, like `std::unique_ptr`, for arrays to automate memory management and reduce the risk of leaks.
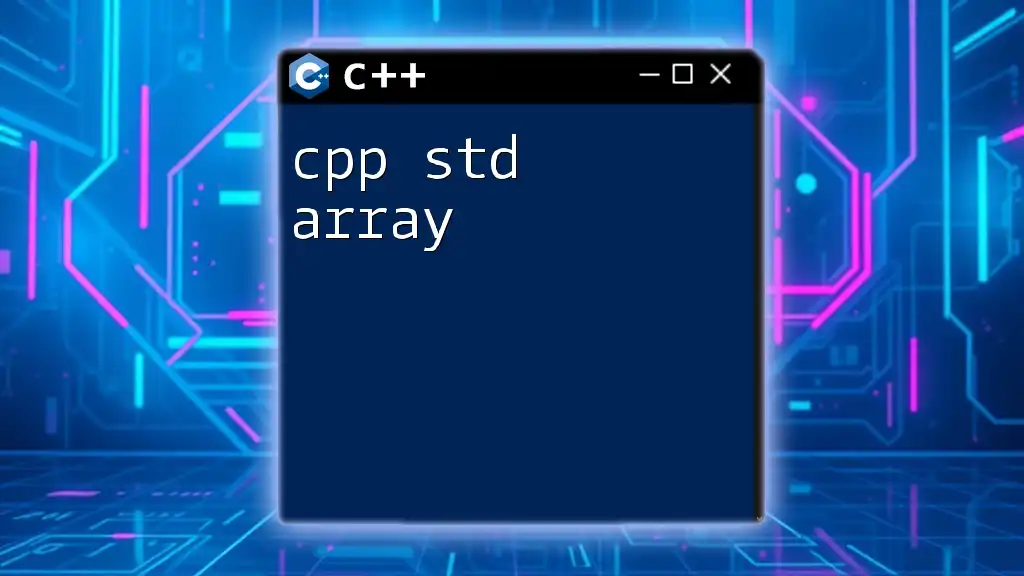
Conclusion
Dynamic char arrays in C++ are a powerful tool for managing memory at runtime. By understanding how to declare, allocate, and manage these arrays, programmers can write efficient and flexible code. Remember, dynamic memory management comes with responsibilities; ensure you allocate and deallocate properly to maintain optimal program performance. C++ provides robust capabilities, but with great power comes great responsibility. Understanding when to use dynamic char arrays versus alternatives like `std::string` is key to becoming a proficient C++ programmer.