In C++, `new` is an operator that allocates memory and calls the constructor of the object, while `malloc` is a C library function that allocates raw memory without calling constructors; here's a simple comparison in code:
#include <iostream>
class MyClass {
public:
MyClass() { std::cout << "Constructor called!" << std::endl; }
};
int main() {
// Using new
MyClass* obj1 = new MyClass(); // Allocates memory and calls constructor
// Using malloc
MyClass* obj2 = (MyClass*)malloc(sizeof(MyClass)); // Allocates raw memory, no constructor call
delete obj1; // Cleanup for new
free(obj2); // Cleanup for malloc
return 0;
}
Understanding Memory Management in C++
What is Memory Management?
Memory management refers to the effective handling of computer memory resources in programming. It involves allocating and deallocating memory efficiently to ensure optimal resource usage, preventing memory leaks, and managing memory fragmentation. Understanding memory management is crucial, particularly in languages like C++ where developers have direct control over memory. The two primary types of memory allocation in programming are stack and heap.
- Stack Memory: Automatically managed, used for local variable storage.
- Heap Memory: Manually managed, used when the amount of memory required can't be determined at compile time.
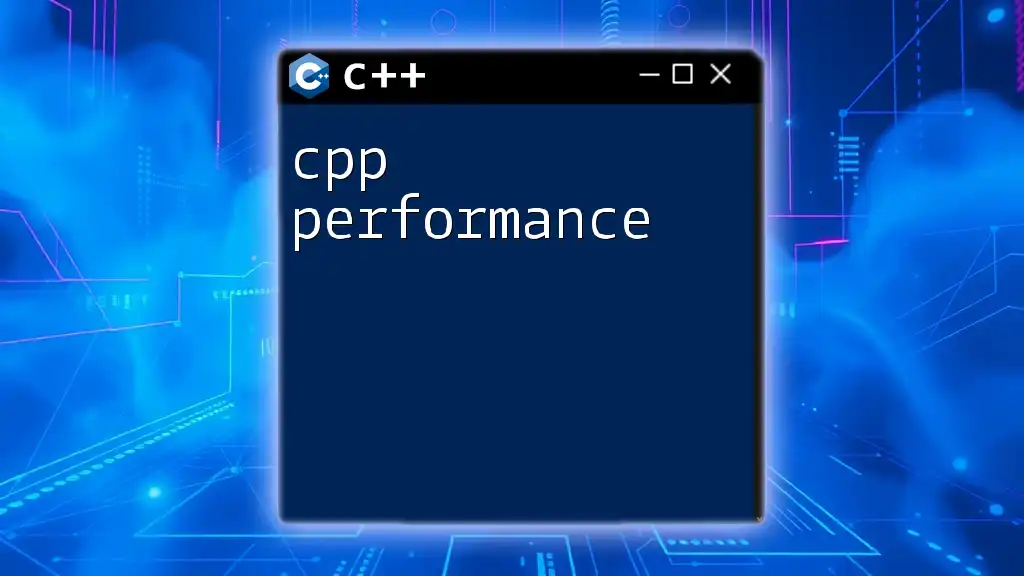
Dynamic Memory Allocation in C++
Introduction to Dynamic Memory Allocation
Dynamic memory allocation allows programs to request memory at runtime whenever required. This is particularly useful when dealing with data structures whose size varies or is unknown during compilation.
Basics of Dynamic Memory Allocation in C++
Dynamic memory allocation enables flexible use of memory. Unlike static memory allocation, which reserves a fixed amount of memory during compile time, dynamic allocation allows developers to handle memory more efficiently as their needs change.
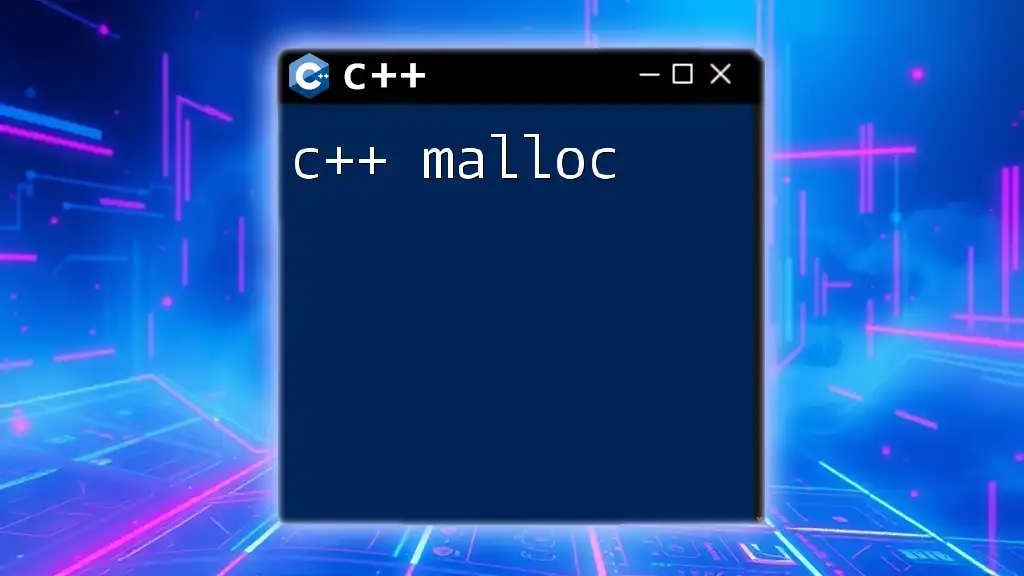
The `new` Operator in C++
What is the `new` Operator?
The `new` operator in C++ is used to allocate memory on the heap. It constructs an object and returns a pointer to the allocated memory.
Allocating Memory with `new`
Here’s how you can use the `new` operator:
int* ptr = new int; // allocate memory for a single integer
*ptr = 42; // assign a value
In this example, memory for an integer is allocated dynamically, and the value `42` is assigned to it. This approach is beneficial because it allows for direct assignments during runtime.
Initializing Objects with `new`
When dealing with user-defined types, you can allocate memory using `new` as follows:
MyClass* obj = new MyClass(); // allocate memory for an object of MyClass
This allocates memory for an instance of `MyClass` and calls the constructor, initializing the object's member variables right away.
Deallocating Memory with `delete`
Memory allocated with `new` must be deallocated using the `delete` operator to prevent memory leaks. Here’s how:
delete ptr; // free the memory allocated to ptr
It’s crucial to deallocate memory to maintain application efficiency and performance.
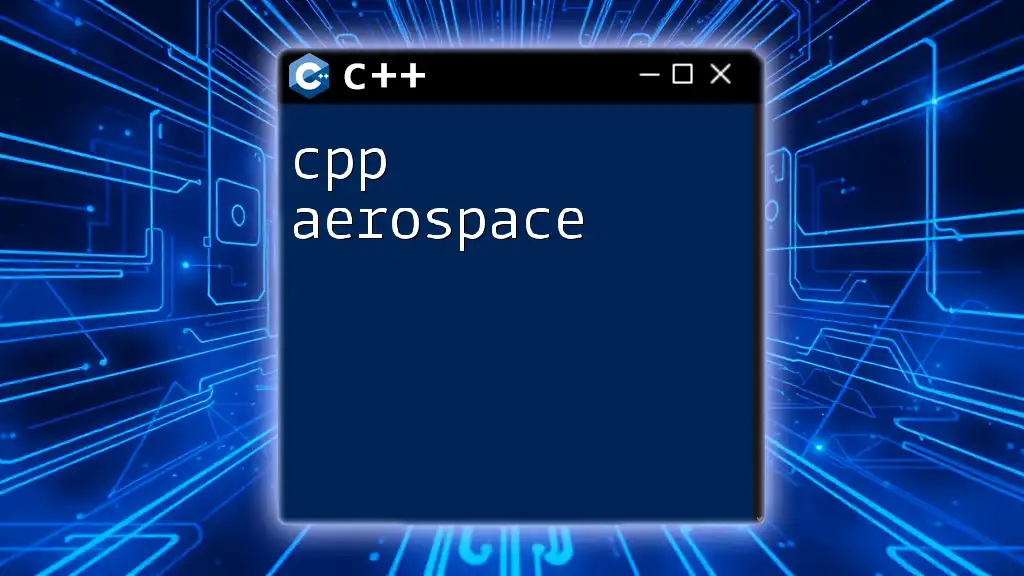
The `malloc` Function in C
What is `malloc`?
`malloc` (memory allocation) is a function in C that allocates memory on the heap. Unlike `new`, which is specifically tailored for C++, `malloc` is a C function and does not call constructors.
Allocating Memory with `malloc`
Using `malloc` is straightforward:
int* arr = (int*)malloc(5 * sizeof(int)); // allocate memory for an array of 5 integers
In this case, `malloc` allocates enough memory for five integers. However, it is essential to remember that `malloc` does not initialize the allocated memory; it may contain garbage values.
Importance of Type Casting
In C++, using `malloc` requires type casting because it returns `void*`. For example:
int* arr = malloc(5 * sizeof(int)); // works in C, but requires type casting in C++
In C, the code above is accepted since C allows implicit conversion of `void*` to other pointer types. In C++, explicit type casting is required.
Deallocating Memory with `free`
To free memory allocated with `malloc`, you use the `free` function:
free(arr); // free the memory allocated for arr
Neglecting to free memory can lead to memory leaks in your applications.
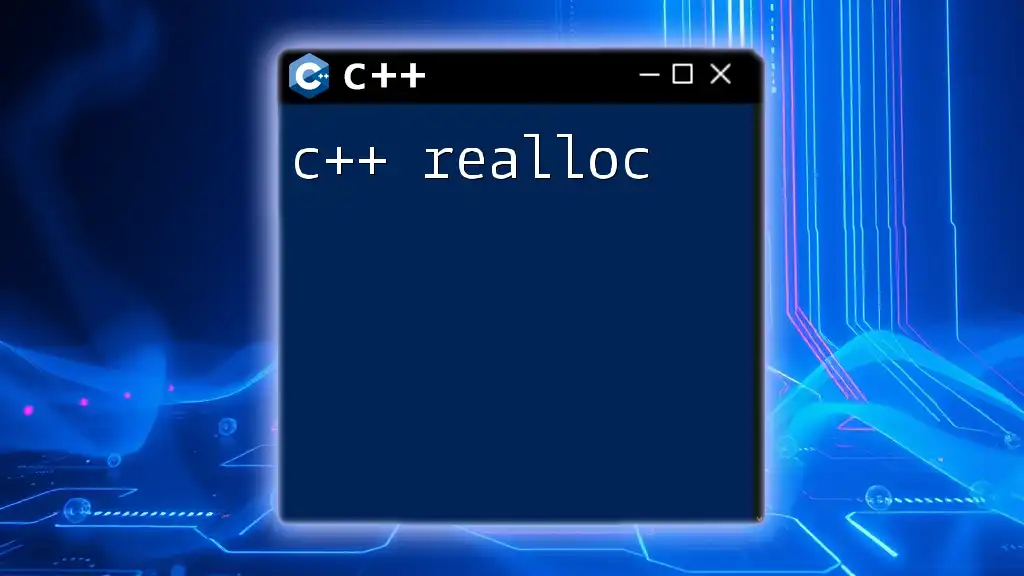
Key Differences Between `new` and `malloc`
Memory Allocation Types
`new`:
- Allocates and constructs memory.
- Calls the constructor for objects, ensuring proper initialization.
`malloc`:
- Allocates memory without constructing or initializing objects.
- Preserves the behavior of C-style memory allocation.
Return Values and Error Handling
The return values from `new` and `malloc` differ significantly in how they represent allocation failures:
- `new`: Returns `nullptr` if memory allocation fails, particularly when used with `(std::nothrow)`.
- `malloc`: Returns `NULL` on failure.
Example of using `new` with error handling:
int* p = new (std::nothrow) int; // returns nullptr on failure
if (!p) {
// handle memory allocation failure
}
Constructors and Destructors
A major distinction between the two comes down to their handling of constructors and destructors:
- `new`: Calls the constructor upon allocation, initializing the object's state.
- `malloc`: Does not call constructors, which means you might end up with uninitialized data.
Overloading and Use Cases
In C++, the `new` operator can be overloaded, allowing customization for memory allocation processes. This makes it a suitable choice for complex applications where memory management demands flexibility and optimization. Meanwhile, `malloc` retains its use in C and situations where C-style allocations are needed.
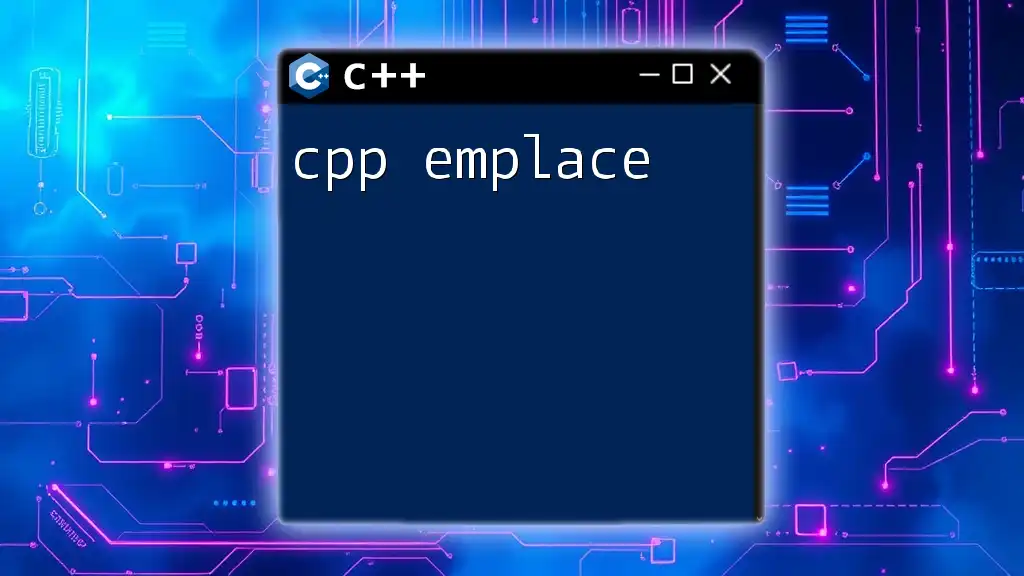
Performance Considerations
Performance of `new` vs `malloc`
When it comes to performance implications, `new` is typically more efficient than `malloc` as it is designed for object-oriented programming. It has mechanisms to improve allocation strategies and potentially reduces overhead.
Fragmentation and Memory Leaks
Both methods can lead to fragmentation and memory leaks if not managed correctly. Memory fragmentation occurs when there is a mismatch between allocated and freed memory blocks, leading to inefficient use of memory resources. Understanding how to use each method loosely or tightly concerning object lifecycles can mitigate such issues.
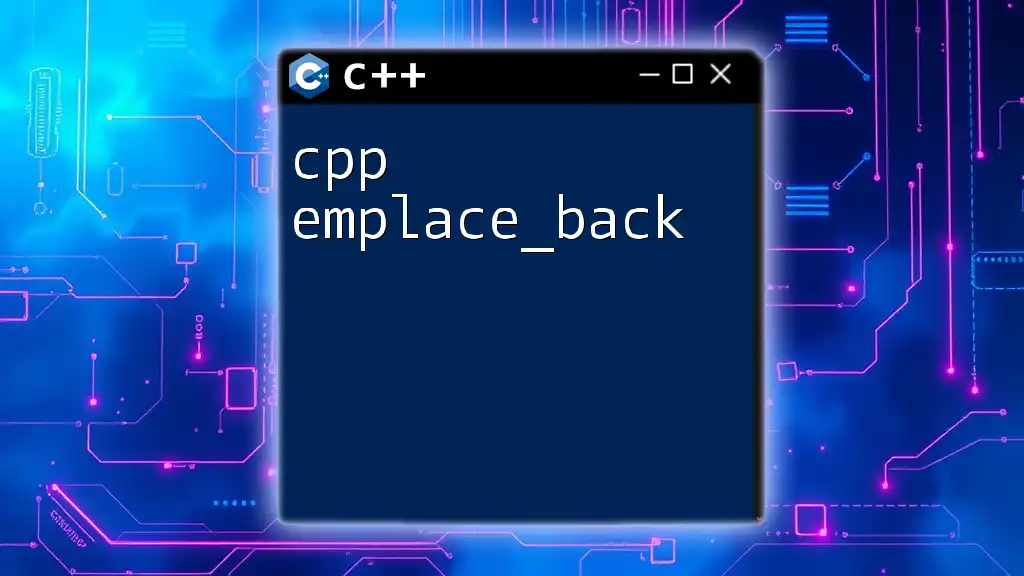
Conclusion
In summary, when considering cpp new vs malloc, it is essential to comprehend their operational differences. While both achieve memory allocation, `new` is tailored for C++ with added convenience in handling object initialization, while `malloc` remains a staple from C for raw memory allocation without constructor interference. By understanding these distinctions, developers can make informed choices that enhance their applications' performance and reliability. Embracing best practices in memory management is vital as you improve your proficiency with these fundamental concepts in C++.